Common Pitfalls in Setting Up Storm with Kafka and Elasticsearch
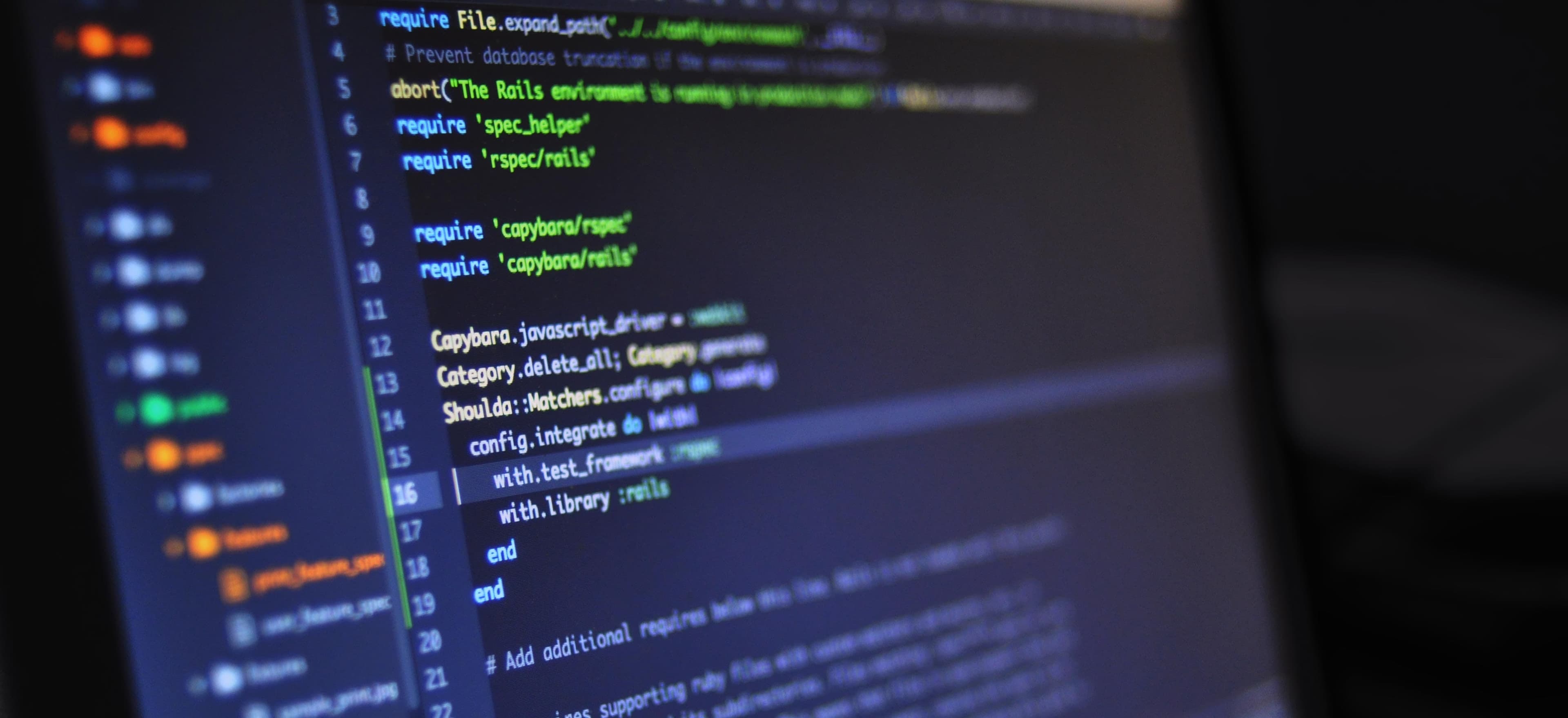
- Published on
Common Pitfalls in Setting Up Storm with Kafka and Elasticsearch
The integration of Apache Storm with Apache Kafka and Elasticsearch is a powerful architecture for real-time data processing and analytics. However, while setting this up, many developers encounter common pitfalls that can lead to performance bottlenecks, data loss, and increased complexity. This blog post will guide you through the common mistakes and provide sensible solutions to ensure a smooth implementation.
Understanding the Architecture
Before diving into the pitfalls, it’s essential to understand how Storm, Kafka, and Elasticsearch interact.
- Apache Storm is a distributed real-time computation system used for processing streams of data.
- Apache Kafka serves as a distributed messaging system that reliably transmits data between systems.
- Elasticsearch is a distributed search and analytics engine, allowing for querying and visualizing large volumes of data.
This trio is often used for building scalable real-time analytics applications. However, improper configuration can lead to significant issues.
Common Pitfalls and Solutions
1. Underestimating Data Serialization
Pitfall: One of the first mistakes developers make is not selecting the appropriate data serialization format. Common formats include JSON, Avro, and Protocol Buffers. JSON is easy to use but can be inefficient in terms of performance and bandwidth.
Solution: Use a more efficient serialization format such as Avro or Protocol Buffers for better performance, especially when dealing with high-throughput systems.
import org.apache.avro.Schema;
import org.apache.avro.generic.GenericData;
import org.apache.avro.io.DatumWriter;
import org.apache.avro.io.Encoder;
import org.apache.avro.io.EncoderFactory;
import org.apache.avro.io.ReflectDatumWriter;
import org.apache.avro.specific.SpecificDatumWriter;
Schema schema = new Schema.Parser().parse(new File("user.avsc"));
GenericData.Record user = new GenericData.Record(schema);
user.put("name", "John Doe");
user.put("age", 25);
// Use a SpecificDatumWriter for serializing objects.
DatumWriter<GenericData.Record> writer = new SpecificDatumWriter<>(schema);
Encoder encoder = EncoderFactory.get().binaryEncoder(outputStream, null);
writer.write(user, encoder);
encoder.flush();
By choosing the right serialization format, you improve both performance and serialize/deserialize times, which is crucial in real-time systems.
2. Poor Resource Allocation
Pitfall: Podding resources inadequately can either lead to inefficient processing or increased costs. Developers often oversubscribe resources, leading to degraded performance during peak load times.
Solution: Always benchmark your application and monitor it under load. Use performance metrics to allocate CPU and memory resources accurately. Apache Storm offers various metrics to help monitor the performance.
# storm.yaml
storm.workers.cpu: 2
storm.workers.memory: 2048
Adjust the above settings based on your application needs after thorough load-testing.
3. Ignoring Backpressure Management
Pitfall: Backpressure is a crucial concept in streaming applications. Failure to manage backpressure may result in resource exhaustion as your application tries to process an influx of events it cannot handle.
Solution: Use Storm’s built-in features to handle backpressure. Monitor processing time, queue sizes, and adjust the number of parallel tasks dynamically.
@Override
public void declareOutputFields(OutputFieldsDeclarer declarer) {
declarer.declare(new Fields("outputField"));
}
4. Insufficient Error Handling
Pitfall: In a distributed system, errors are inevitable. Ignoring error handling mechanisms can result in data loss or system crashes.
Solution: Implement robust error handling and consider leveraging Kafka’s ability to replay events in the case of a failure.
public void processMessage(String message) {
try {
// process the message
} catch (Exception e) {
// log error and potentially send message back to Kafka for reprocessing
log.error("Failed to process message: " + message, e);
}
}
In this example, if processing fails, the error is logged, and corrective action can be taken later.
5. Failing to Tune Elasticsearch
Pitfall: Not tuning Elasticsearch can lead to slow queries and poor performance when indexing data.
Solution: Optimize shard sizes, configure refresh intervals, and set appropriate replicas depending on the type of data.
PUT /my_index
{
"settings": {
"index": {
"refresh_interval": "30s",
"number_of_shards": 1,
"number_of_replicas": 1
}
}
}
Tuning these settings based on the use case improves the performance of both indexing and querying.
6. Ineffective Schema Management
Pitfall: Changing data schemas on the fly can break your application unless managed properly. Torza the data can lead to incompatibility issues down the line.
Solution: Use schema registries, such as Confluent Schema Registry, for Kafka. Ensure that all producers and consumers are aware of schema changes.
# Register a new schema
curl -X POST -H "Content-Type: application/vnd.schemaregistry.v1+json" \
--data '{"schema": "{\"type\":\"record\",\"name\":\"User\",\"fields\":[{\"name\":\"name\",\"type\":\"string\"},{\"name\":\"age\",\"type\":\"int\"}]}"}' \
http://localhost:8081/subjects/users/versions
7. Ignoring Data Retention Policies
Pitfall: Not setting data retention policies for Kafka topics can lead to unbounded growth of data, resulting in system instabilities.
Solution: Define retention times based on the business needs, using either time-based or size-based retention.
# Set retention time to 7 days
bin/kafka-topics.sh --zookeeper localhost:2181 --alter --topic my_topic --config retention.ms=604800000
The Bottom Line
Integrating Apache Storm with Kafka and Elasticsearch can dramatically enhance your real-time analytics capabilities. However, it is essential to be cautious of common pitfalls that can hinder this potential. By understanding the architecture, properly managing data serialization, resource allocation, backpressure, error handling, and tuning Elasticsearch, you will build a robust system that meets your data processing needs.
For further exploration on these technologies, check out the Apache Storm documentation and the Kafka documentation.
By avoiding these pitfalls, you set the foundation for a scalable, resilient, and responsive data processing architecture in your organization.