Common Pitfalls when Deploying Spring Boot Apps on Knative
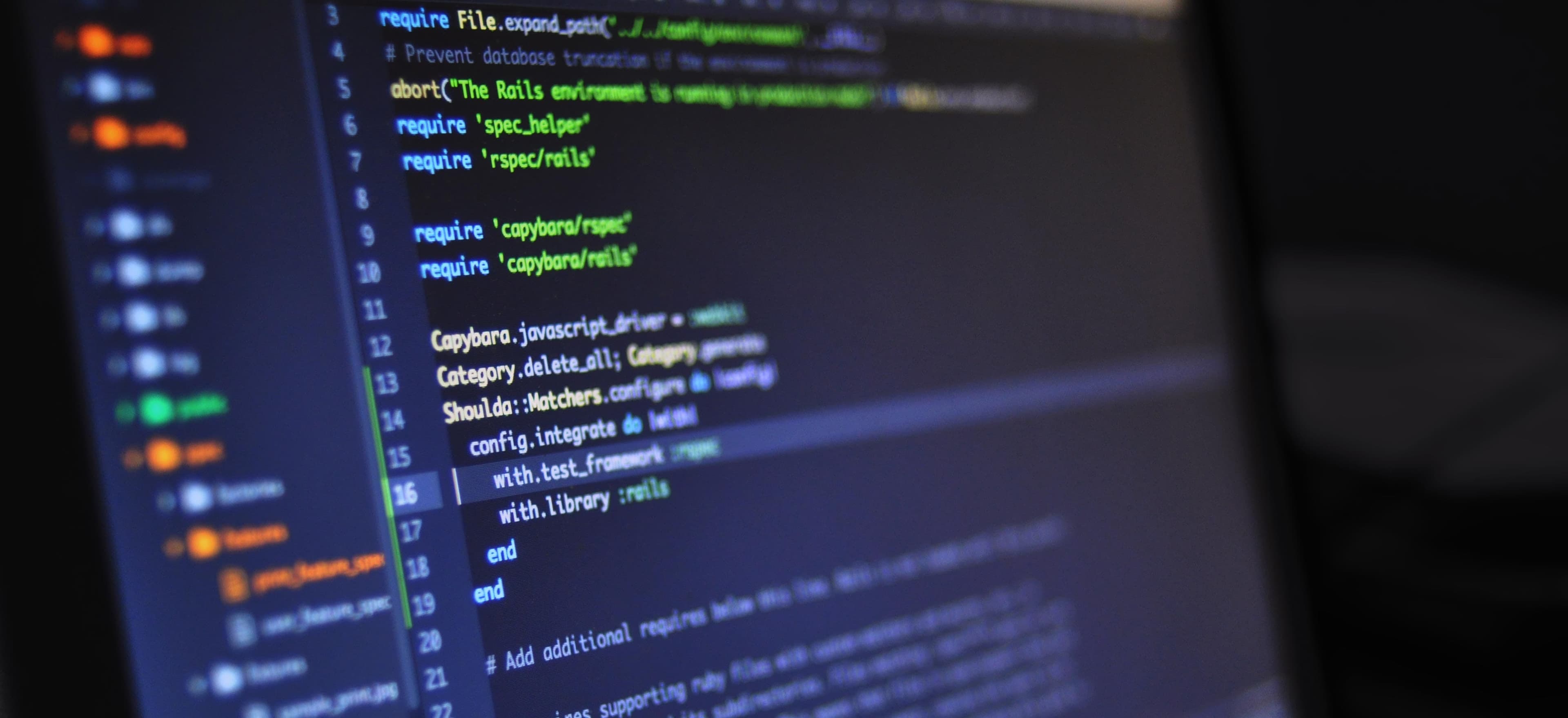
- Published on
Common Pitfalls When Deploying Spring Boot Apps on Knative
As microservices architecture gains momentum, deploying applications efficiently becomes critical. Knative, a Kubernetes-based platform, offers a powerful solution for building, deploying, and managing serverless applications. When combined with Spring Boot, it simplifies microservices development. However, as promising as this combination is, several pitfalls can hinder your deployment experience.
In this blog post, we will explore common challenges developers face when deploying Spring Boot applications on Knative and provide solutions to overcome them. Stay tuned, as we will also include code snippets that demonstrate best practices.
Understanding Knative
Knative consists of three main components:
- Serving: Manages the deployment and scaling of your service.
- Eventing: Enables the building of event-driven architectures.
- Build: Supports creating container images from source code.
Why Use Spring Boot with Knative?
Spring Boot is renowned for its ease of use in creating microservices. It provides:
- Convention over Configuration: Reduces boilerplate code.
- Standalone: Can run a Spring application without needing an application server.
- Rich Ecosystem: Offers extensive libraries and integrations.
When combined with Knative, Spring Boot applications can take advantage of autoscaling features, simplifying deployment and resource management.
Common Pitfalls and Solutions
1. Ignoring the Resource Requests and Limits
One of the initial considerations is defining resource requests and limits for your Spring Boot application. Without these, Knative can struggle to allocate resources efficiently.
Why It Matters
Setting appropriate requests and limits helps prevent issues such as resource starvation, which can lead to performance degradation.
Example Code Snippet
Here’s an example of a deployment.yaml
file with specified resource limits:
apiVersion: serving.knative.dev/v1
kind: Service
metadata:
name: spring-boot-app
spec:
template:
spec:
containers:
- image: your-repo/spring-boot-app
resources:
requests:
memory: "256Mi"
cpu: "500m"
limits:
memory: "512Mi"
cpu: "1"
Commentary: The above snippet defines requests and limits that guide Knative to allocate necessary resources while preventing resource hogging.
2. Not Using a Knative-Optimized Image
Most Spring Boot applications are packaged as JAR or WAR files. However, deploying these directly might result in larger images, leading to longer deployment times.
Why It Matters
The size of your container image directly affects startup time and scaling performance. Smaller images are not only faster but also save on bandwidth costs.
Example Code Snippet
Consider using a multi-stage Docker build:
# Stage 1: Build the application
FROM maven:3.6-jdk-8 AS build
WORKDIR /app
COPY pom.xml .
COPY src ./src
RUN mvn clean package -DskipTests
# Stage 2: Create the runtime image
FROM openjdk:8-jre
COPY --from=build /app/target/spring-boot-app.jar /spring-boot-app.jar
ENTRYPOINT ["java", "-jar", "/spring-boot-app.jar"]
Commentary: This multi-stage build creates a lean runtime image containing only the essentials, effectively reducing startup times.
3. Not Configuring Health Checks
In a Kubernetes environment, health checks are crucial. They ensure that your service is responsive and can handle traffic without issues.
Why It Matters
Neglecting health checks can lead to inadequate monitoring. Knative needs to know whether your application is up and running to route traffic efficiently.
Example Code Snippet
Here's how to configure liveness and readiness probes for your Spring Boot app:
apiVersion: serving.knative.dev/v1
kind: Service
metadata:
name: spring-boot-app
spec:
template:
spec:
containers:
- image: your-repo/spring-boot-app
readinessProbe:
httpGet:
path: /actuator/health
port: 8080
initialDelaySeconds: 5
periodSeconds: 10
livenessProbe:
httpGet:
path: /actuator/health
port: 8080
initialDelaySeconds: 10
periodSeconds: 30
Commentary: Using Spring Boot Actuator, this snippet adds health checks, enhancing the reliability of your application on Knative.
4. Forgetting to Configure Autoscaling
Knative provides autoscaling capabilities based on concurrency or CPU utilization. Not configuring this correctly could lead to inefficient resource usage or degraded performance.
Why It Matters
Setting appropriate autoscaling metrics ensures that your application scales seamlessly according to demand, without incurring unnecessary costs.
Example Code Snippet
Here’s how to configure autoscaling based on concurrency:
apiVersion: serving.knative.dev/v1
kind: Service
metadata:
name: spring-boot-app
spec:
template:
spec:
autoscaling:
minScale: 1
maxScale: 5
containers:
- image: your-repo/spring-boot-app
env:
- name: K_NATIVE_CONCURRENT_REQUSTS
value: "1" # Change this value based on your application needs
Commentary: This snippet dynamically adjusts the number of pod replicas based on the number of requests your app handles concurrently.
5. Hardcoding Configuration Values
Environment variables and configurations should never be hardcoded into your application. Use Spring profiles or external configurations instead.
Why It Matters
Hardcoding values makes your application less flexible and harder to deploy across different environments.
Example Code Snippet
Here is how to specify configurations using application.yml
:
spring:
datasource:
url: ${DATABASE_URL}
username: ${DATABASE_USERNAME}
password: ${DATABASE_PASSWORD}
Commentary: This approach utilizes Spring's support for externalized configuration, enhancing portability across different environments.
Best Practices for Smooth Deployment
- Use CI/CD Pipelines: Automate testing and deployment to catch errors early.
- Monitor Performance: Utilize tools like Prometheus to monitor your Knative service.
- Incremental Rollouts: Employ Blue-Green or Canary deployments for safer releases.
- Emphasize Logging: Implement logging strategies to troubleshoot issues effortlessly.
The Closing Argument
Deploying Spring Boot applications on Knative can unlock exceptional advantages for your development workflow and operational efficiency. However, awareness of common pitfalls ensures that you mitigate challenges before they escalate. By adhering to best practices, you can significantly enhance your deployment experience.
If you are interested in further exploring Spring Boot and Knative, consider checking out the Knative documentation and the Spring Boot reference guide.
Feel free to share your experiences and challenges when dealing with Spring Boot and Knative in the comments below. Happy coding!
Checkout our other articles