Overcoming Common Pitfalls in Learning Data Structures
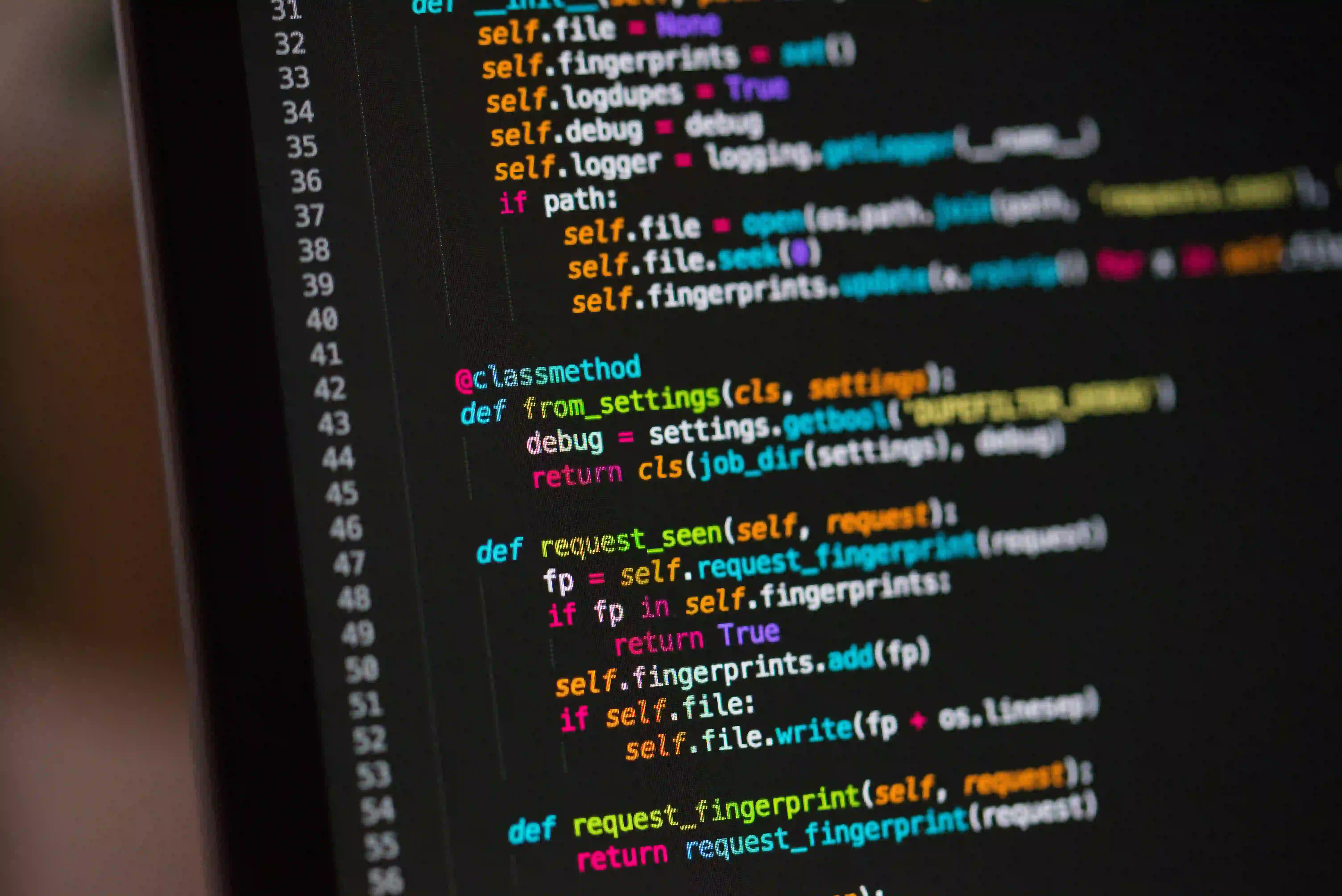
Overcoming Common Pitfalls in Learning Data Structures
Data structures are fundamental components in the world of programming and software development. They store, organize, and manage data efficiently. However, learning them can be a daunting task for many beginners. In this blog post, we will explore common pitfalls when learning data structures, along with strategies to avoid them, ensuring you master these essential concepts with ease.
The Importance of Data Structures
Before diving into potential pitfalls, let's briefly discuss why data structures matter. Efficient data management is crucial in software development. Whether you're handling large datasets or designing algorithms, understanding how to utilize the right data structures can drastically improve performance. By the end of this post, you'll be equipped to tackle data structures head-on.
Common Pitfalls and How to Overcome Them
1. Superficial Understanding
Many learners tend to scratch the surface of data structures, focusing solely on definitions and basic functionalities without grasping their underlying principles. This superficial understanding leads to confusion when faced with real-world problems.
Solution: Deepen Your Knowledge
Instead of merely memorizing what each data structure does, invest time in understanding how they work. Take the time to implement them from scratch. For example, consider implementing a simple linked list.
class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
class LinkedList {
private Node head;
public void add(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
public void printList() {
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
}
In this code, we define a linked list by creating a Node
class and a LinkedList
class. Understanding this implementation allows you to grasp how data is manipulated within the linked list.
2. Ignoring Time and Space Complexity
Another major pitfall is not fully engaging with the concepts of time and space complexity. It’s not enough to know that a particular data structure exists; understanding how it performs under different conditions is crucial.
Solution: Analyze and Compare
As you study, always analyze the time and space complexity of operations:
- Array: O(1) for access, O(n) for insertion (unless at the end).
- Linked List: O(n) for access, O(1) for insertion (if you have a reference).
Here’s an example of analyzing the insertion complexity in an array vs. a linked list:
// Insert an element at the end of an array
public void addToArray(int[] arr, int value, int size) {
if (size >= arr.length) {
System.out.println("Array is full.");
return;
}
arr[size] = value; // O(1) Time Complexity
}
// Insert an element in a linked list
public void addToLinkedList(int value) {
LinkedList list = new LinkedList();
list.add(value); // O(1) Time Complexity
}
When learning these concepts, remember to relate each structure's performance to real-world scenarios. This will help solidify your understanding.
3. Overwhelming Yourself with Too Many Structures
With a plethora of data structures available, it’s easy to feel overwhelmed. Trying to learn all of them at once can lead to frustration and confusion.
Solution: Focus on Fundamentals First
Start with the most commonly used data structures—arrays, linked lists, stacks, queues, trees, and hash tables. Master these before exploring more advanced structures like graphs or tries.
For example, here’s a brief overview of stacks and their operations:
class Stack {
private List<Integer> stack;
public Stack() {
stack = new ArrayList<>();
}
public void push(int value) {
stack.add(value);
}
public int pop() {
if (isEmpty()) {
throw new EmptyStackException();
}
return stack.remove(stack.size() - 1);
}
public boolean isEmpty() {
return stack.size() == 0;
}
}
Understanding the stack structure lays the foundation for more complex data structures like trees and graphs.
4. Neglecting Real-World Applications
Theoretical knowledge is essential, but lacking application can diminish understanding. Many learners forget to apply data structures in real-world scenarios, thus limiting their effectiveness.
Solution: Build Projects
Engage in projects or coding challenges that require you to use different data structures. Sites like LeetCode or Hackerrank offer numerous challenges that can bolster your practical skill set.
For instance, consider implementing a basic directory structure using a tree:
class DirectoryNode {
String name;
List<DirectoryNode> children;
DirectoryNode(String name) {
this.name = name;
this.children = new ArrayList<>();
}
public void addChild(DirectoryNode child) {
children.add(child);
}
}
By simulating a directory tree, you can see how data structures organize information in various software applications.
5. Lack of Practice
Theoretical knowledge is only beneficial with sufficient practice. Many learners may read about data structures but fail to implement and manipulate them consistently.
Solution: Dedicate Time for Coding
Make a habit of solving data structure problems daily. Allocate specific time each week to practice coding exercises.
Consider using the following simple exercise: Implement a queue using two stacks. This challenge reinforces your understanding of both data structures.
class QueueUsingStacks {
private Stack<Integer> stack1;
private Stack<Integer> stack2;
public QueueUsingStacks() {
stack1 = new Stack<>();
stack2 = new Stack<>();
}
public void enqueue(int item) {
stack1.push(item);
}
public int dequeue() {
if (stack2.isEmpty()) {
while (!stack1.isEmpty()) {
stack2.push(stack1.pop());
}
}
return stack2.pop();
}
}
This example reveals how data structures can interact with each other, enhancing your skills in algorithmic thinking and logical design.
A Final Look
Learning data structures is crucial for anyone striving to become a proficient programmer. By avoiding common pitfalls like superficial understanding, ignoring complexity, overwhelming yourself, neglecting real-world applications, and lack of practice, you can navigate these challenges more effectively.
Remember to focus on mastering the fundamentals, engaging in practical applications, and committing to continuous practice. With determination and the right strategies, you can conquer data structures and apply them confidently in your coding journeys.
For further reading on data structures and algorithms, you may find these resources helpful:
Happy coding!