Mastering the Adapter Pattern: Bridging Java Class Gaps
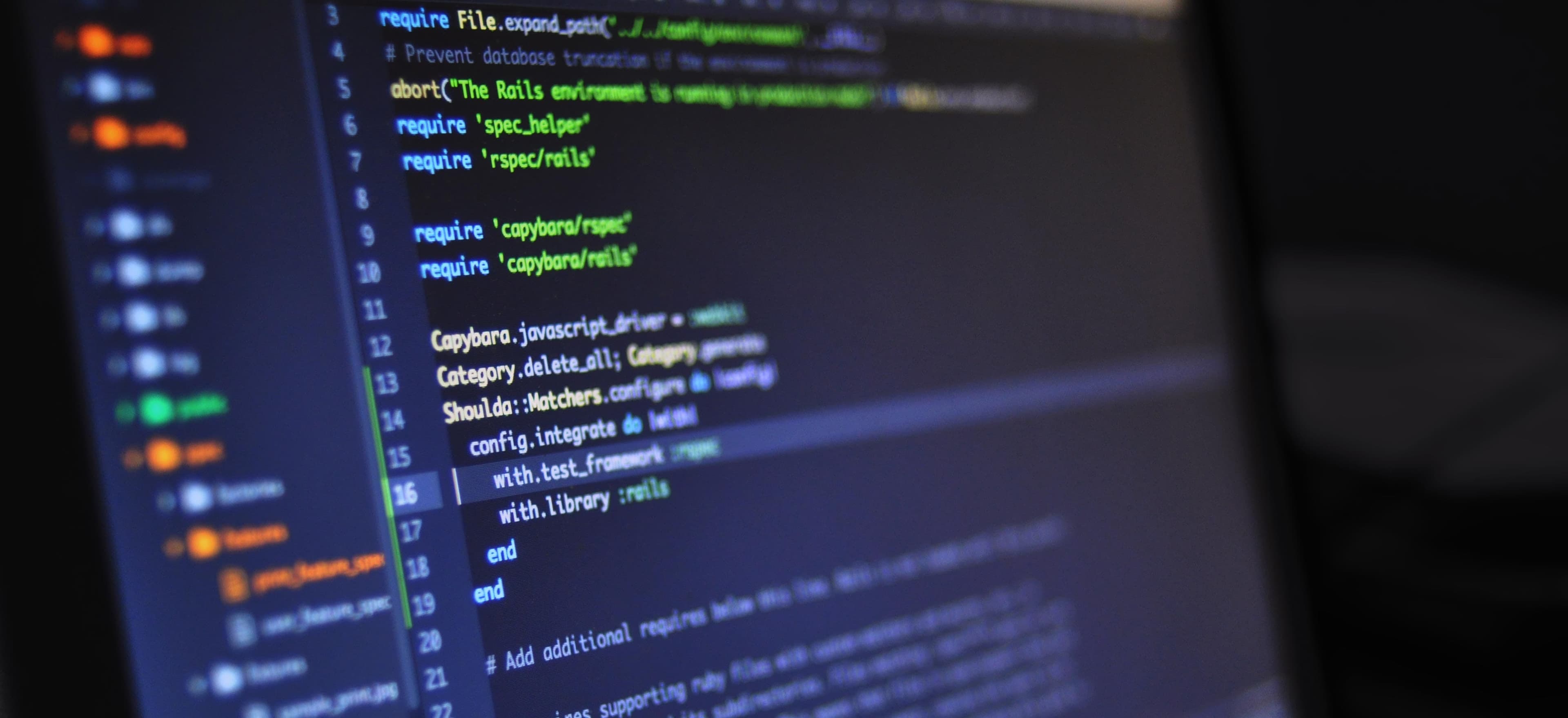
- Published on
Mastering the Adapter Pattern: Bridging Java Class Gaps
In software design, the concept of design patterns has gained much attention, and one of the most useful patterns in object-oriented programming is the Adapter Pattern. This pattern allows incompatible interfaces to work together, thus enabling classes to interact seamlessly. Utilizing the Adapter Pattern is particularly beneficial in Java, where the landscape of libraries and frameworks is comprehensive.
In this blog post, we will explore the Adapter Pattern in Java, including its structure, implementation, and practical examples. Whether you are a seasoned developer or a novice just getting your feet wet, mastering this pattern can significantly improve your coding architecture and flexibility.
What is the Adapter Pattern?
The Adapter Pattern serves as an intermediary that translates one interface into another expected by the client. Think of it as a translator that allows two parties speaking different languages to communicate effectively.
When to Use the Adapter Pattern
The Adapter Pattern can be particularly useful in the following scenarios:
- You have a large codebase with existing classes that need to interact with new classes.
- You need to integrate third-party libraries that do not match your existing interfaces.
- You want to create a reusable class that can work with different interfaces.
Structure of the Adapter Pattern
Components of the Adapter Pattern
- Target Interface: This is the expected interface that the client will utilize.
- Adaptee Class: This class has an interface that you need to adapt.
- Adapter Class: This class implements the Target Interface and uses an instance of Adaptee to fulfill the interface contract.
Implementation Example
Let's dive into a concrete example of the Adapter Pattern in Java.
Step 1: Define the Target Interface
// The Target Interface that client code expects
public interface MediaPlayer {
void play(String audioType, String fileName);
}
Step 2: Create the Adaptee Class
// The Adaptee - a class that plays audio files in a specific format
public class AudioPlayer {
public void playMp3(String fileName) {
System.out.println("Playing MP3 file. Name: " + fileName);
}
public void playWav(String fileName) {
System.out.println("Playing WAV file. Name: " + fileName);
}
}
Step 3: Create the Adapter Class
// The Adapter - adapts the AudioPlayer to work with MediaPlayer interface
public class AudioPlayerAdapter implements MediaPlayer {
private final AudioPlayer audioPlayer;
public AudioPlayerAdapter(AudioPlayer audioPlayer) {
this.audioPlayer = audioPlayer;
}
@Override
public void play(String audioType, String fileName) {
if ("mp3".equalsIgnoreCase(audioType)) {
audioPlayer.playMp3(fileName);
} else if ("wav".equalsIgnoreCase(audioType)) {
audioPlayer.playWav(fileName);
} else {
System.out.println("Invalid audio type: " + audioType);
}
}
}
Step 4: Client Code
Finally, we can utilize our adapter to play different audio types seamlessly.
public class Main {
public static void main(String[] args) {
MediaPlayer player = new AudioPlayerAdapter(new AudioPlayer());
player.play("mp3", "song1.mp3"); // Output: Playing MP3 file. Name: song1.mp3
player.play("wav", "song2.wav"); // Output: Playing WAV file. Name: song2.wav
player.play("avi", "movie.avi"); // Output: Invalid audio type: avi
}
}
Explanation of Code
- We first create an interface called
MediaPlayer
as our target interface. - The
AudioPlayer
class serves as the adaptee, implementing specific functionality to handle audio files. - The
AudioPlayerAdapter
class wraps around theAudioPlayer
object, converting requests for audio playback into the appropriate calls onAudioPlayer
. - In our
Main
class, we instantiate the adapter to seamlessly play audio, demonstrating versatility while maintaining the expected interface.
Advantages of the Adapter Pattern
- Separation of Concerns: The Adapter Pattern decouples the client from the details of the adaptee classes. This promotes cleaner and more maintainable code.
- Reusable Code: By enabling classes to interact through a common interface, you can have reuse and flexibility across different components.
- Flexible Architecture: Changes in one component do not necessitate changes in others. This adaptability is crucial in software development.
Common Pitfalls to Avoid
While the Adapter Pattern brings many benefits, there are some common pitfalls:
- Overusing Adapters: Aim for clarity and simplicity; don't create an adapter if it's unnecessary.
- Chaotic Interfaces: Ensure that your interfaces remain cohesive and focused. Adapting too many incompatible classes into one can lead to messy code.
- Poor Naming: Make sure to name your classes clearly to indicate their roles as adapters. Clarity aids in understanding your code.
Bringing It All Together
Mastering the Adapter Pattern can significantly enhance your Java applications by bridging gaps between incompatible interfaces, thus promoting flexibility and maintainability. By following the principles outlined in this post, you can effectively implement this pattern in your projects.
For further reading, you might want to explore the following resources:
- Design Patterns: Elements of Reusable Object-Oriented Software - A foundational text on design patterns.
- Refactoring Guru - Adapter - A comprehensive explanation and examples of the Adapter Pattern.
By leveraging the Adapter Pattern, you can ensure that your Java projects remain adaptable, maintainable, and ready for future growth. Happy coding!
Checkout our other articles