Mastering Asynchronous Processing in Servlet 3.0 Efficiently
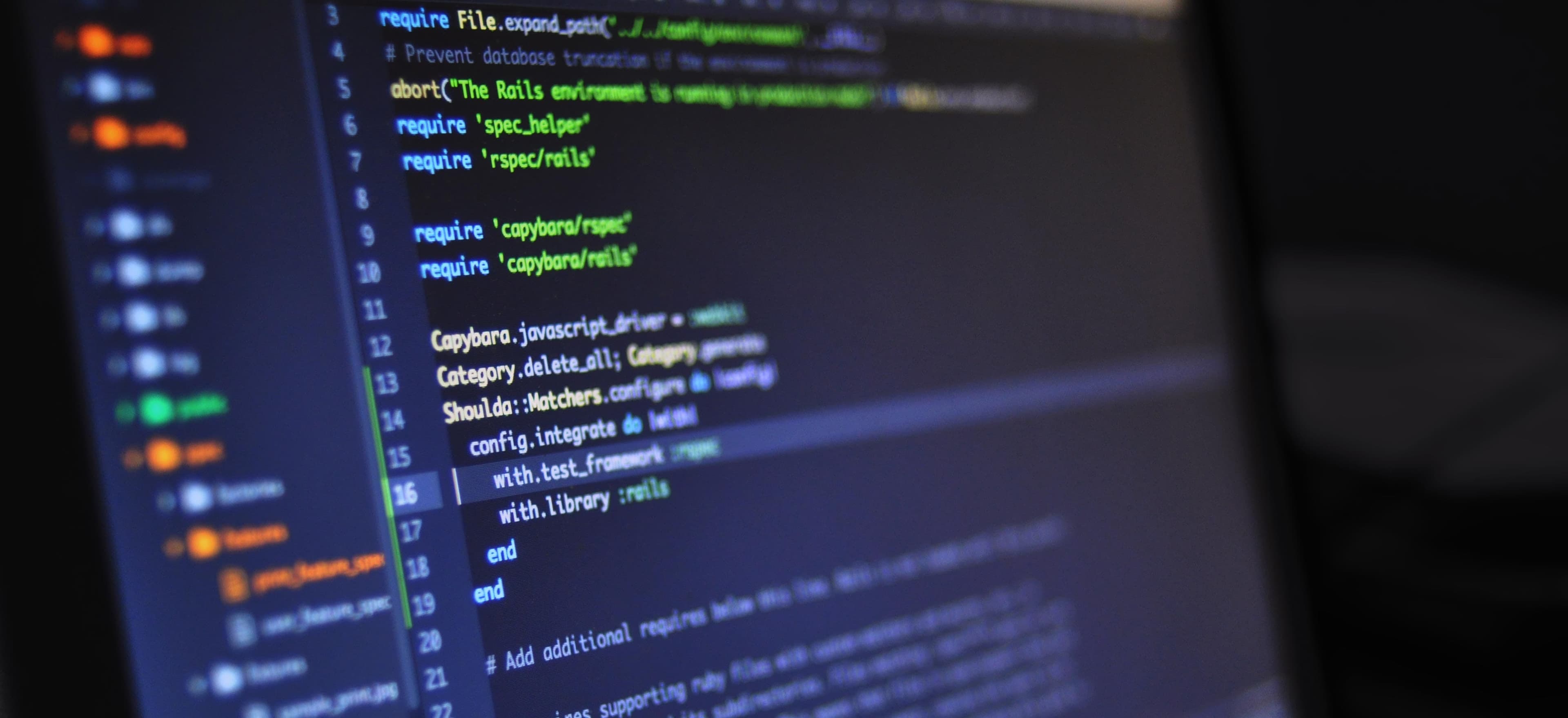
- Published on
Mastering Asynchronous Processing in Servlet 3.0 Efficiently
As web applications have grown more complex, the need for responsive and efficient server-side processing has become increasingly important. One of the pivotal features introduced in Servlet 3.0 is asynchronous processing, which allows developers to handle requests and responses in a non-blocking manner. Mastering this capability can significantly enhance the user experience, especially in applications that rely on long-running tasks.
In this blog post, we will explore the core concepts of asynchronous processing in Servlet 3.0, provide examples, and discuss best practices for effective implementation. Let's dive in!
Understanding Asynchronous Processing
Asynchronous processing in Servlet 3.0 helps to improve application scalability and performance by freeing up threads while waiting for long-running tasks to complete. Traditional servlet processing is synchronous and can result in resource blocks, particularly when handling requests that might involve slow I/O operations, such as database queries or external API calls.
Default Synchronous Model
In a standard servlet lifecycle, when a request is received, a servlet container assigns a thread to handle that request. The thread processes the request synchronously:
public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException {
// Simulate a long-running task
try {
Thread.sleep(5000); // Sleep for 5 seconds
} catch (InterruptedException e) {
e.printStackTrace();
}
response.getWriter().write("Response after long processing!");
}
In the above code, the server thread holds on to the request for 5 seconds, during which it cannot serve any other request.
Transition to Asynchronous Processing
With Servlet 3.0, we can delegate long-running tasks and allow the servlet container to reuse the associated thread. The asynchronous nature provides a way to return control immediately to the servlet container, allowing it to handle other requests while waiting for the long task to complete.
Here’s how to implement asynchronous processing:
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
@WebServlet(urlPatterns = "/asyncServlet", asyncSupported = true)
public class AsyncServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Start asynchronous processing
AsyncContext asyncContext = request.startAsync();
// Set a timeout for the async operation
asyncContext.setTimeout(5000);
// Long-running task
new Thread(() -> {
try {
// Simulate delay
Thread.sleep(5000);
// Complete the response
asyncContext.getResponse().getWriter().write("Response after asynchronous processing!");
} catch (InterruptedException | IOException e) {
e.printStackTrace();
} finally {
asyncContext.complete(); // Completing the async processing
}
}).start();
}
}
Key Code Highlights
- AsyncContext: The
startAsync()
method provides access toAsyncContext
, which manages the lifecycle of the async request. - Timeout Handling: By calling
setTimeout()
, you can define how long the servlet should wait before automatically completing the request. - Thread Management: Wrap the long-running task in a separate thread to ensure the server thread is released immediately.
- Completing the Request: Once the task is finished,
asyncContext.complete()
is called to indicate that the asynchronous processing is complete.
Benefits of Asynchronous Processing
- Improved Scalability: By not blocking threads, you can serve more concurrent requests, as threads can be reused.
- Enhanced User Experience: Users aren't left waiting; long operations can run in the background.
- Better Resource Utilization: Allows efficient use of server resources by freeing threads for other tasks while waiting for external responses.
Challenges and Considerations
While asynchronous processing can provide significant benefits, there are challenges that developers need to keep in mind:
- Error Handling: When working with separate threads, traditional error handling falls short as exceptions cannot be propagated back to the original request.
- Thread Management: Proper management and tuning of threads are essential to avoid resource depletion.
- Clarity and Complexity: Code can become more complex, and developers should maintain clear logic, especially when handling shared resources.
Best Practices for Asynchronous Processing
To efficiently implement asynchronous processing in your Servlet applications, consider the following best practices:
-
Limit Task Duration: Use timeouts wisely to avoid hanging requests. Ensure that your tasks are brief whenever possible.
-
Use Executor Services: Instead of manually creating threads, leverage An
ExecutorService
can provide thread pooling and better management of tasks:ExecutorService executorService = Executors.newCachedThreadPool(); executorService.submit(() -> { // Long running task here });
-
Graceful Exception Handling: Implement error handling strategies to catch exceptions in your asynchronous tasks. Use logging for easier debugging.
-
Identify Long Tasks: Recognize which requests need async processing. Not every task benefits from asynchronous execution; use it judiciously.
-
Maintain State Awareness: When using asynchronous processing, ensure that you manage the state of requests if the server handles multiple influxes of requests simultaneously.
My Closing Thoughts on the Matter
Asynchronous processing in Servlet 3.0 represents a significant enhancement in the Java servlet API, enabling developers to build responsive web applications that can handle heavy loads with grace. By allowing long-running tasks to execute without holding onto server threads, applications can manage resources more efficiently, providing a much-improved user experience.
To further refine your knowledge and implementation of asynchronous processing, explore the official Java EE documentation or dive into specialized literature such as the Java EE 7 Tutorial which covers asynchronous processing thoroughly.
Whether you are developing a new application or optimizing an existing one, mastering asynchronous processing is a key skill for any Java developer looking to enhance their web applications.