Common Pitfalls When Using Apache Cassandra with Java
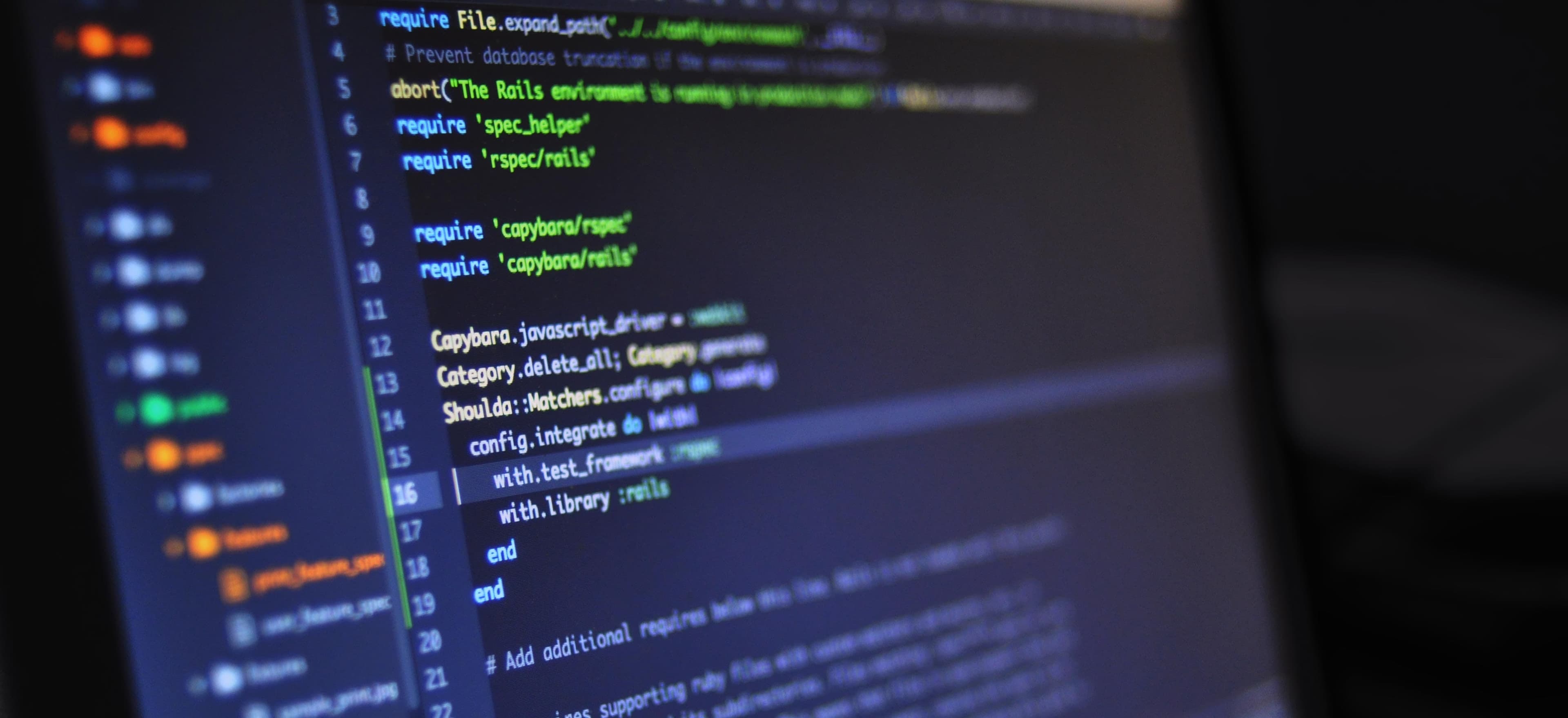
- Published on
Common Pitfalls When Using Apache Cassandra with Java
Apache Cassandra is a powerful distributed database known for its high scalability and availability. While it is an excellent choice for handling large volumes of structured data across many commodity servers, it comes with its own set of challenges when used in conjunction with Java. In this blog post, we will explore common pitfalls developers encounter and how to avoid them.
Table of Contents
- Understanding Cassandra’s Architecture
- Connection Management
- Data Modeling
- Query Execution
- Error Handling
- Best Practices for Using Cassandra with Java
- Conclusion
Understanding Cassandra’s Architecture
Before diving into the pitfalls, it is crucial to have a solid understanding of Cassandra’s architecture. Cassandra is designed to handle enormous amounts of data across many servers, providing high availability without a single point of failure.
- Nodes and Clusters: A Cassandra cluster comprises multiple nodes. Each node is identical, making it easy to scale horizontally by adding more nodes.
- Replication: Data is replicated across multiple nodes to ensure reliability.
- Partitioning: Data is distributed across the cluster based on a partition key, allowing for efficient data retrieval.
For a deeper understanding of Cassandra’s architecture, you can refer to the official documentation.
Connection Management
One of the most common pitfalls when working with Cassandra in Java is improper connection management. Many developers fail to leverage the built-in connection pooling mechanism offered by the DataStax Java Driver.
Example Code for Setting Up a Connection
import com.datastax.oss.driver.api.core.CqlSession;
public class CassandraConnection {
private static CqlSession session;
public static void connect() {
session = CqlSession.builder()
.withKeyspace("my_keyspace")
.build();
}
public static void close() {
session.close();
}
}
Why this Matters: Opening and closing connections frequently can lead to performance bottlenecks. By using the CqlSession
for connection pooling, you significantly reduce latency.
Pitfalls:
- Not using connection pooling: Repeatedly opening connections can exhaust resources.
- Failing to close connections: Neglecting to close connections can lead to memory leaks and eventual application crashes.
Data Modeling
Cassandra requires a different approach to data modeling compared to traditional relational databases. Many developers make the mistake of applying relational principles, which can lead to inefficient reads and writes.
Data Model Example
CREATE TABLE users (
user_id UUID PRIMARY KEY,
name text,
email text,
created_at timestamp
);
Why this Matters: In Cassandra, the data model is designed around how you intend to query the data. By using a primary key effectively, you can optimize the read and write patterns of your application.
Pitfalls:
- Improperly defining partition keys: This can lead to wide partitions and adversely affect performance.
- Not denormalizing data: In Cassandra, it is often beneficial to store related data in the same table.
Query Execution
When executing queries, developers typically overlook the importance of query performance. Understanding how queries interact with the underlying architecture is crucial for efficient data access.
Prepared Statements Example
import com.datastax.oss.driver.api.core.cql.SimpleStatement;
public void executeQuery(String userId) {
SimpleStatement statement = SimpleStatement.newInstance(
"SELECT * FROM users WHERE user_id = ?", userId);
session.execute(statement);
}
Why this Matters: Prepared statements can help optimize query performance by allowing the database to cache execution plans.
Pitfalls:
- Not using prepared statements: Executing raw statements can lead to performance degradation.
- Ignoring consistency levels: Misunderstanding how consistency levels work can lead to unexpected behaviors.
Error Handling
Another frequent oversight is error handling. In a distributed system like Cassandra, network failures and timeouts are common. Thus, handling these exceptions gracefully is critical to maintain the application's stability.
Example for Handling Errors
try {
session.execute(statement);
} catch (Exception e) {
System.err.println("Query execution failed: " + e.getMessage());
// Implement retry logic or fail gracefully
}
Why this Matters: Proper error handling ensures that your application remains resilient in the face of transient errors, thus improving user experience and reliability.
Pitfalls:
- Failing to handle transient errors: Not implementing retry logic can result in lost operations.
- Ignoring timeouts: Not setting appropriate timeout values can lead to very slow responses or application hangs.
Best Practices for Using Cassandra with Java
-
Utilize Connection Pooling: Always use the DataStax Java Driver to manage connections effectively. This reduces latency and resource exhaustion.
-
Design for Read Patterns: Consider how you'll access data when designing your tables. Write your data models to suit the application's read patterns.
-
Prefer Prepared Statements: They are more efficient than regular statements and protect against SQL injection.
-
Employ Error Handling: Implement robust error-handling mechanisms, including retries and logging critical failures.
-
Monitor Performance: Use tools like DataStax OpsCenter to monitor the performance of your Cassandra cluster and identify bottlenecks.
-
Stay Updated: Keep your Cassandra and Java driver versions up to date to leverage improvements and new features.
Closing Remarks
Using Apache Cassandra with Java can be incredibly rewarding, but it requires careful consideration of its unique architecture and behavior. By avoiding common pitfalls related to connection management, data modeling, query execution, and error handling, developers can create robust and efficient applications that scale effectively.
Whether you are a seasoned developer or a newcomer to Cassandra, understanding and applying these best practices will go a long way in enhancing your overall application performance.
For further reading, check out the Cassandra Documentation and the DataStax Java Driver. Happy coding!
Checkout our other articles