Common Pitfalls in Java Records: What You Need to Know
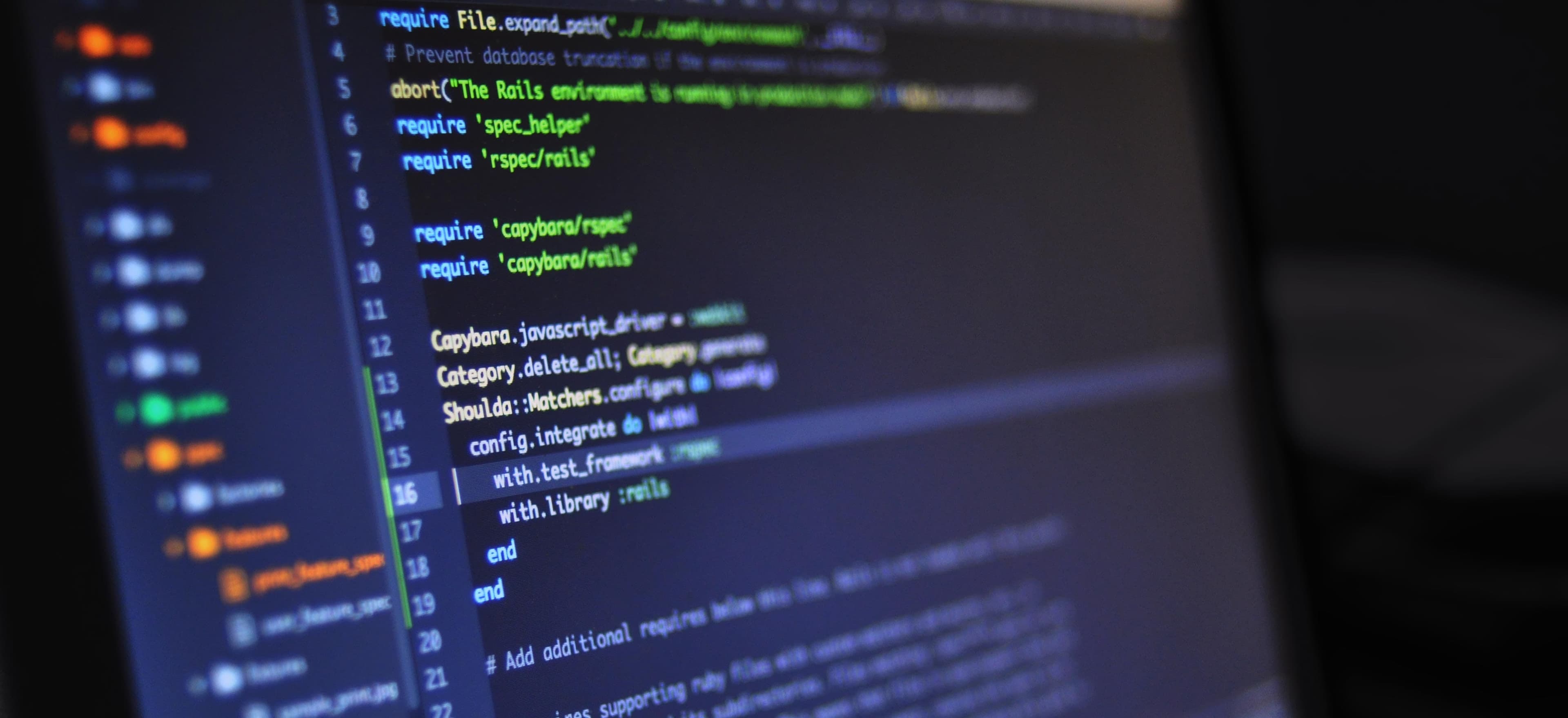
- Published on
Common Pitfalls in Java Records: What You Need to Know
Since the introduction of Java 14, records have emerged as a powerful, concise way to model immutable data in Java. They streamline the creation of classes that are primarily used to store data, reducing boilerplate code significantly. Despite their advantages, there are several common pitfalls that developers may encounter when using Java records. In this blog post, we will explore these pitfalls, offer solutions, and present best practices to ensure that you leverage Java records effectively.
What are Java Records?
Java records are special types of classes designed for immutable data models. They provide a way to create simple data containers that can be easily instantiated and accessed. Here’s a basic example to illustrate:
public record Person(String name, int age) {}
In this example, Person
is a record that holds a name and age. This single line replaces the traditional boilerplate code required to create a class with fields, constructors, getters, and toString
, equals
, and hashCode
methods.
Key Benefits of Using Records
- Reduced Boilerplate: Automatically generates getters,
toString
,equals
, andhashCode
methods. - Immutable by Default: Promotes immutability, enhancing data integrity.
- Compact Syntax: Offers a cleaner, more readable syntax compared to traditional classes.
Common Pitfalls in Java Records
Despite their simplicity, there are pitfalls that developers should be wary of. Let's dive into the most common issues.
1. Misunderstanding Immutability
Pitfall: One of the biggest misconceptions about records is their immutability. While the fields in a record are immutable, if those fields reference mutable objects, the state of the record can change.
Example:
import java.util.List;
public record Team(String name, List<String> members) {}
public class Main {
public static void main(String[] args) {
List<String> members = new ArrayList<>();
Team team = new Team("Dev Team", members);
// Modifying the list after the record is created
members.add("Alice");
System.out.println(team); // This will show the updated list
}
}
Solution: Always use immutable collections like List.of()
for fields that should not change.
import java.util.List;
public record Team(String name, List<String> members) {
public Team {
// Use immutable copy of members
members = List.copyOf(members);
}
}
2. Default Constructor Limitation
Pitfall: Records do not have a default constructor. This means you can't create an instance without providing the required fields. Attempting to use a default constructor will lead to a compilation error.
Example:
public record User(String username) {}
// Compilation error: cannot create instance without username
Solution: Always provide the required information or use optional parameters and validation via factory methods.
public record User(String username) {
public static User createDefault() {
return new User("defaultUser");
}
}
3. Serialization Issues
Pitfall: Java records can sometimes experience issues with serialization. When a record is serialized and then deserialized, problems may arise if the version does not match.
Solution: Ensure you manage your serialVersionUID properly.
import java.io.*;
public record SerializablePerson(String name, int age) implements Serializable {
private static final long serialVersionUID = 1L;
}
4. Inheritance and Subclassing
Pitfall: Records cannot extend other classes aside from java.lang.Record
. This limitation may cause issues when developers expect records to behave like traditional classes.
Example:
public record Manager(String name, int age) extends Employee { /* Error */ }
Solution: Prefer composition over inheritance by creating interfaces or using other design patterns.
5. Overusing Records
Pitfall: It can be tempting to use records for every data structure. However, using them for complex data structures with behavior can lead to code smells and reduced clarity.
Solution: Use records for simple data-carrying scenarios only. Complex logic should still leverage conventional classes.
6. Mixing Mutable and Immutable Fields
Pitfall: Mixing mutable and immutable types in records could lead to unintended side effects.
Example:
import java.util.ArrayList;
import java.util.List;
public record Session(String title, List<String> participants) {
public Session {
participants = new ArrayList<>(participants); // This allows modification
}
}
Solution: Always ensure that fields are either fully immutable or are wrapped in defensive copies to prevent unintended modifications.
public record Session(String title, List<String> participants) {
public Session {
participants = List.copyOf(participants);
}
}
7. Lack of Custom Behavior
Pitfall: Records are not designed to hold behavior or complex logic, which can lead to unnecessary complexity if you try to override methods or add custom behaviors.
Solution: Keep records simple and consider using traditional classes when behavior is needed.
Final Thoughts
Java records represent a significant improvement in how developers can model immutable data in their applications. However, with great power comes great responsibility. Understanding the pitfalls associated with records is crucial for effective usage.
For more detailed insights into Java records, visit the official Java Tutorials. Familiarizing yourself with the Java Language Specification can also enhance your understanding of how records operate.
Incorporating the best practices outlined in this guide will ensure that you utilize Java records to their fullest potential, helping to write clean, efficient, and maintainable code. Next time you use records, keep these common pitfalls in mind, and you'll set your Java applications up for success!
Additional Resources
- Java Language Documentation
- Java Tutorials - Working with Records
- Effective Java (3rd Edition) by Joshua Bloch
Happy coding!