Common Pitfalls in Spring Social Twitter Setup
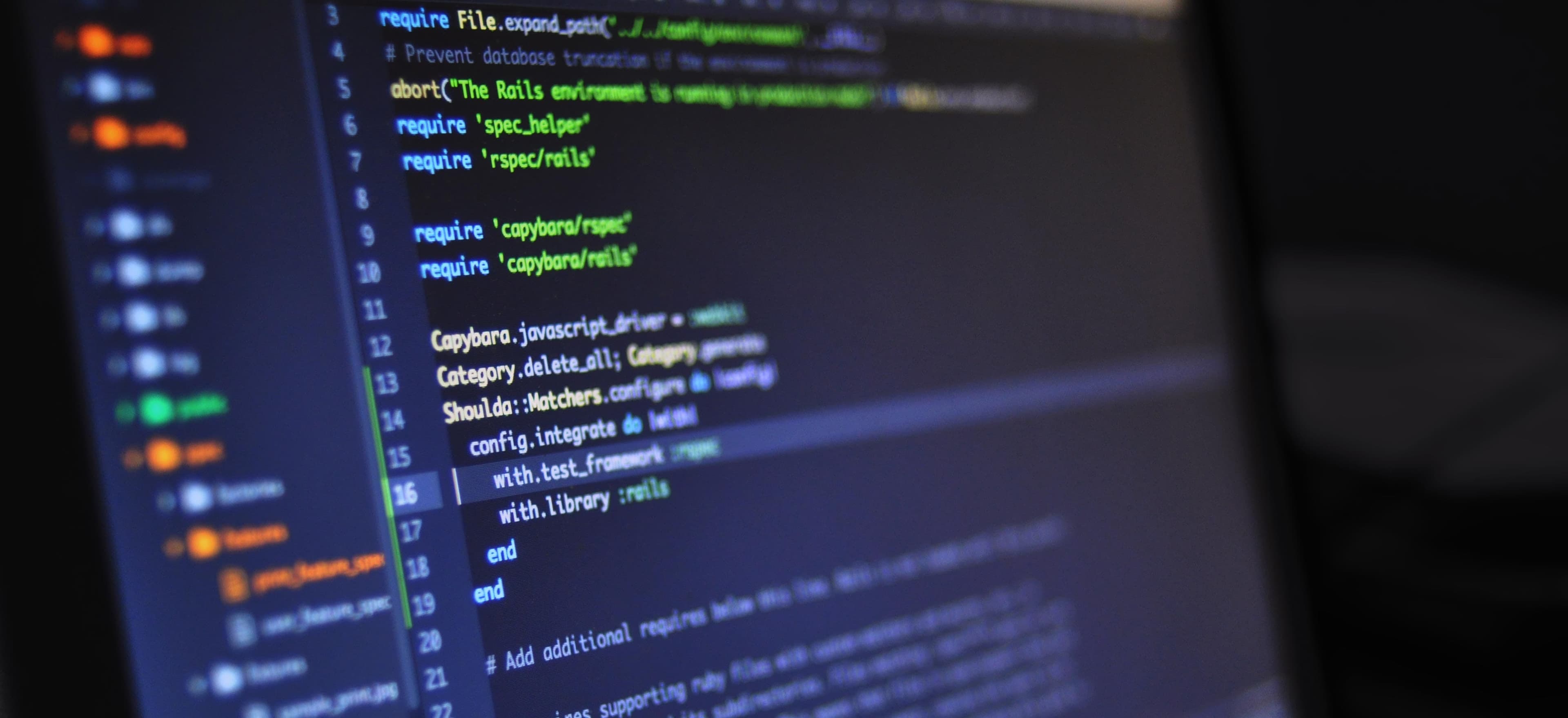
- Published on
Common Pitfalls in Spring Social Twitter Setup
Setting up social media integrations can greatly enhance your application’s functionality. Spring Social Twitter allows developers to connect their applications to Twitter in a straightforward manner. However, there are common pitfalls that developers encounter during this setup. Understanding these challenges will not only save time but also lead to more efficient implementation. In this blog post, we will discuss the common pitfalls when integrating Spring Social with Twitter and provide code snippets to guide you through.
Understanding Spring Social Twitter
Before diving into common pitfalls, let’s briefly discuss what Spring Social Twitter is. Spring Social is an extension of the Spring Framework, designed to provide connectivity to various social media APIs, including Twitter. With Spring Social Twitter, developers can leverage Twitter's unique features – like posting tweets, reading timelines, and even processing direct messages – through a set of high-level services.
Common Pitfalls in Spring Social Twitter Setup
1. Misconfigured Callback URLs
When creating your Twitter app, one of the most critical configurations is the callback URL. This URL is where Twitter will redirect users after they authorize your application to access their account.
Pitfall: Forgetting to set this URL correctly can lead to authentication failures.
Solution: Ensure that the callback URL in your Twitter app settings matches the one your application is using.
@Autowired
private SocialConnectionRepository socialConnectionRepository;
// Define the callback URL
private static final String CALLBACK_URL = "http://yourdomain.com/connect/twitter";
// Use the correct URL in the authorization process
apiBinding.getOAuthOperations().buildAuthorizeUrl(CALLBACK_URL, null);
2. Insufficient Permissions
Twitter has strict rules about the permissions granted to applications. By default, new Twitter apps may not have the permissions needed to perform certain operations.
Pitfall: Missing out on read or write permissions may result in execution failures.
Solution: When creating the app on the Twitter developer portal, ensure that you have requested the necessary permissions.
3. Access Token Mismanagement
The OAuth flow is critical for accessing APIs, and managing access tokens properly is essential for uninterrupted service.
Pitfall: Failing to persist access tokens securely can lead to authentication issues later on.
Solution: Use a database or secure store to save user tokens when they successfully authorize your application.
public void saveAccessToken(String userId, String accessToken) {
// Utilize a secure database for persistence
socialConnectionRepository.createConnectionRepository(userId)
.addConnection(createTwitterConnection(accessToken));
}
private Connection<Twitter> createTwitterConnection(String accessToken) {
// Your code for constructing the Twitter connection
}
4. Not Handling Rate Limits
Twitter API imposes rate limits on the number of calls you can make within a certain time frame.
Pitfall: Exceeding these limits can result in API call failures, breaking the functionality of your application.
Solution: Monitor API call usage and implement a backoff strategy.
try {
twitterService.getUserTimeline();
} catch (TwitterAPIException e) {
if (e.getErrorCode() == 88) { // Rate limit exceeded
// Implement backoff strategy
Thread.sleep(60000); // wait for 60 seconds
}
}
5. Ignoring Error Handling
While Spring Social provides some level of abstraction, it is still essential to handle errors appropriately.
Pitfall: Not implementing error handling can lead to poor user experience and unhandled exceptions.
Solution: Wrap API calls in try-catch blocks to manage exceptions gracefully.
try {
twitterApiBinding.updateStatus("Hello, Twitter!");
} catch (TwitterException e) {
// Log the error and inform the user
logger.error("Failed to update status: " + e.getMessage());
}
6. Not Utilizing Strong OAuth Libraries
The OAuth libraries that Spring Social depends on can be complex.
Pitfall: Misunderstanding how these libraries work can lead to unintended consequences during the OAuth flow.
Solution: Familiarize yourself with the OAuth processes—both from Twitter and Spring Social's perspectives.
7. Not Testing with Different Users
When initially developing your application, it is easy to test it with just your account.
Pitfall: There may be unforeseen issues when other users try to authenticate.
Solution: Always act on feedback from varied users and utilize multiple test accounts.
8. Overlooking Rate Limit Reset Times
Twitter’s API provides a limit reset time alongside rate-limit responses.
Pitfall: Neglecting to check when the rate limit resets can cause unnecessary failures.
Solution: Always check the headers of the API response for rate limit info and adjust your calls accordingly.
9. Failing to Keep Libraries Updated
Using outdated libraries can lead to issues with compatibility and security vulnerabilities.
Pitfall: The lack of updates may result in poor performance and obsolete meetings.
Solution: Regularly check for updates to Spring Social and its dependencies.
10. Not Leveraging the Spring Ecosystem
One of the great things about Spring is that it offers a comprehensive ecosystem.
Pitfall: Not taking advantage of integration with Spring Boot, Spring Security, or databases could lead to a less efficient application.
Solution: Use Spring Boot to simplify configuration. Integrate with Spring Security for better authentication management.
Example of Spring Social Twitter Setup
Here’s a basic example to illustrate how to integrate Spring Social with Twitter correctly.
@Configuration
@EnableSocial
public class SocialConfig extends SocialConfigurerAdapter {
@Override
public void addConnectionFactories(ConnectionFactoryConfigurer registry, Environment env) {
ConnectionFactory<Twitter> connectionFactory = new TwitterConnectionFactory(
env.getProperty("twitter.consumerKey"),
env.getProperty("twitter.consumerSecret"));
registry.addConnectionFactory(connectionFactory);
}
@Override
public void addArgumentResolvers(List<HandlerMethodArgumentResolver> argumentResolvers) {
argumentResolvers.add(new ConnectionFactoryResolver());
}
}
// Service class to handle Twitter operations
@Service
public class TwitterService {
@Autowired
private Twitter twitter;
public void postTweet(String message) {
try {
twitter.timelineOperations().updateStatus(message);
} catch (TwitterException e) {
// Handle error here
}
}
}
Bringing It All Together
Integrating Spring Social with Twitter can significantly elevate your application’s capability to interact with one of the world's largest social media platforms. However, it is crucial to navigate the common pitfalls discussed above to ensure that you set up the integration successfully.
By understanding the essential aspects of managing configuration, permissions, tokens, and error handling, you can streamline your setup process. For further reading on Spring Social and its components, consider exploring the Spring Social Documentation.
Incorporating these tips into your integration process will lead to a robust and resilient Twitter API experience. Be proactive about addressing these pitfalls, and make your social media interactions as smooth and effective as possible. Happy coding!