Common Spring Security Pitfalls in Boot 2.0 Authentication
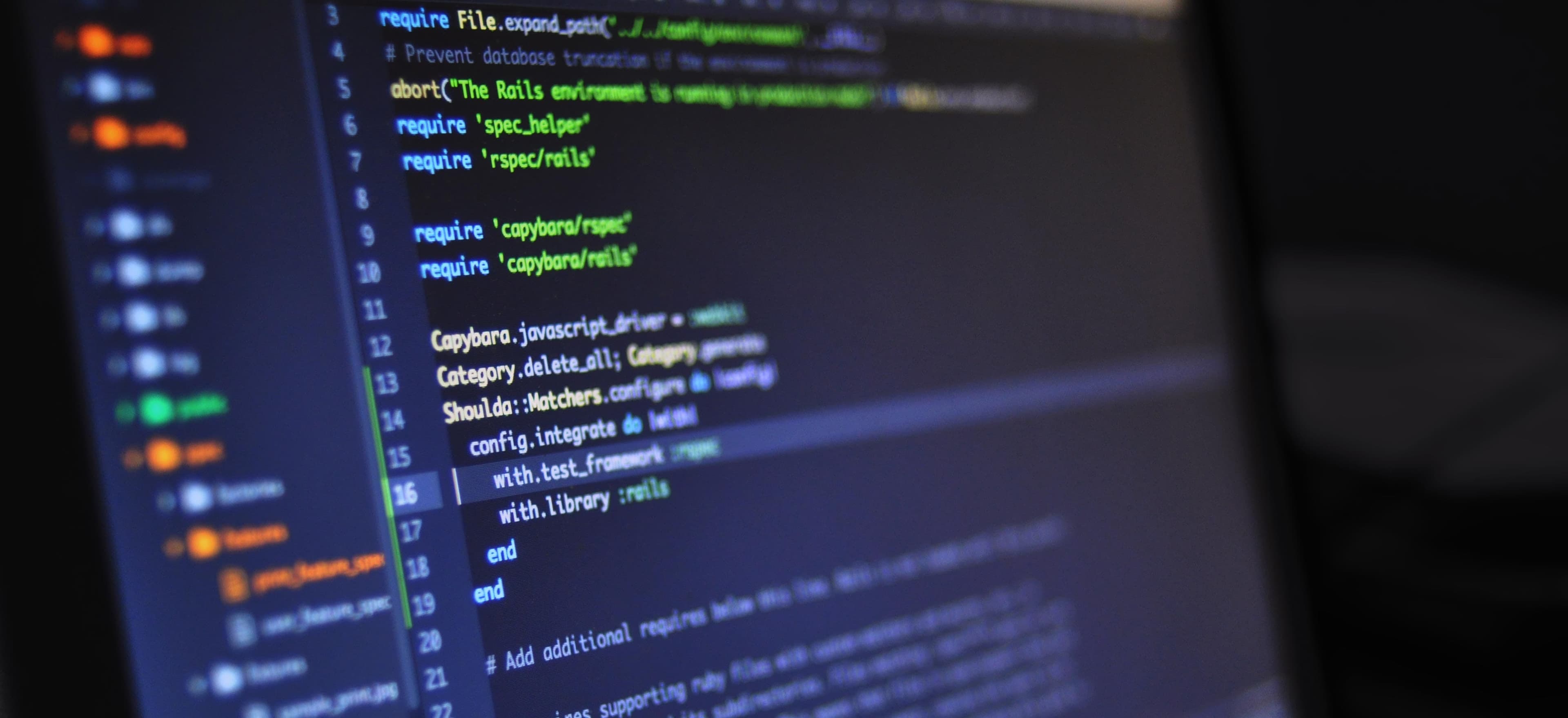
- Published on
Common Spring Security Pitfalls in Boot 2.0 Authentication
Spring Boot 2.0 has revolutionized the way we build Java applications, particularly when it comes to security. However, with great power comes certain pitfalls. In this blog post, we’ll delve into the common spring security pitfalls developers encounter while implementing authentication in Spring Boot 2.0. By understanding these pitfalls, you can ensure a more secure and efficient application.
Understanding Spring Security Boot 2.0
Spring Security is a robust framework that provides a comprehensive security solution for Java applications, including authentication, authorization, and protection against common security exploits. Spring Boot simplifies its configuration and helps developers get started quickly.
Key Features of Spring Security in Boot 2.0:
- Declarative access control
- Authentication and authorization
- CSRF protection
- Session management
- Integration with OAuth 2.0 and JWT
1. Misconfigured CORS Settings
Cross-Origin Resource Sharing (CORS) is crucial when you're building APIs consumed by client-side applications. A common mistake occurs when developers accidentally allow all origins, exposing their API to potential attacks.
Solution
Ensure you understand your CORS configuration. Here is an example of enabling CORS correctly:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**") // Map your specific endpoints
.allowedOrigins("http://yourdomain.com") // Specify allowed origins
.allowedMethods("GET", "POST", "PUT", "DELETE");
}
}
In the above code, we've set up CORS to allow only specific origins and methods. Strive to avoid using allowedOrigins("*")
, as it's akin to opening your doors to anyone.
2. Not Using HTTPS
Another catastrophic mistake is not enforcing HTTPS, which leaves your application susceptible to man-in-the-middle (MITM) attacks.
Why HTTPS Matters
HTTPS encrypts the data transferred between clients and servers, ensuring that sensitive information remains confidential.
Implementation
To enforce HTTPS in a Spring Boot application:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.web.servlet.server.ConfigurableWebServerFactory;
import org.springframework.boot.web.servlet.server.WebServerFactoryCustomizer;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.annotation.Order;
@Configuration
public class HttpsConfig {
@Value("${server.port}")
private int port;
@Bean
@Order(1)
public WebServerFactoryCustomizer<ConfigurableWebServerFactory> webServerCustomizer() {
return factory -> factory.addErrorPages(new ErrorPage(HttpStatus.FORBIDDEN, "/error"));
}
// Uncomment to redirect HTTP to HTTPS
/*
@Bean
public ServletRegistrationBean<RedirectServlet> httpToHttpsServlet(){
return new ServletRegistrationBean<>(new RedirectServlet(), "/*");
}
*/
}
Incorporating HTTP to HTTPS redirection ensures that all your connections are secure.
3. Poor Authentication Credentials Storage
Storing passwords as plain text is a serious flaw that can lead to data breaches. Always hash passwords before storing them in the database.
Best Practices
Utilize a strong hashing algorithm like BCrypt:
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
public class PasswordEncodingExample {
public static void main(String[] args) {
String rawPassword = "userPassword123";
BCryptPasswordEncoder passwordEncoder = new BCryptPasswordEncoder();
// Hash the password
String encodedPassword = passwordEncoder.encode(rawPassword);
// Store `encodedPassword` in your database
System.out.println("Encoded password: " + encodedPassword);
}
}
BCrypt automatically salts the password, which adds an extra layer of security.
4. Inadequate Role-Based Access Control (RBAC)
Role-Based Access Control is essential for managing user privileges effectively. A frequent mistake is overly broad access permissions.
Desired Approach
Define granular roles and permissions, and structure your security configuration like so:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN") // Only ADMIN users can access
.antMatchers("/user/**").hasAnyRole("USER", "ADMIN") // USERS and ADMIN access
.antMatchers("/public/**").permitAll() // Public resources
.and()
.formLogin() // Enables form-based authentication
.permitAll();
}
}
In this configuration, access control is clearly defined, preventing unauthorized access.
5. Not Handling Expired Sessions
Ignoring session management can lead to unauthorized user access, especially in applications where users need to log in frequently. You need to handle expired sessions gracefully.
Handling Sessions
You can customize the session management like this:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.sessionManagement()
.maximumSessions(1) // Limit to one session per user
.expiredUrl("/login?expired=true"); // Redirect to login on expiration
}
}
This configuration ensures that users are appropriately notified upon session expiration.
6. Lack of Logging and Monitoring
Failing to set up logging and monitoring of security-related events can leave your application vulnerable. Always log authentication failures and unauthorized access attempts.
Logging Setup
You can integrate auditing using Spring Security with just a few lines of code:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.web.authentication.AuthenticationFailureHandler;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.failureHandler(authenticationFailureHandler());
}
@Bean
public AuthenticationFailureHandler authenticationFailureHandler() {
return (request, response, exception) -> {
// Log the authentication failure reasons
System.out.println("Authentication failed: " + exception.getMessage());
response.sendRedirect("/login?error=true");
};
}
}
With this, all authentication failures result in a log entry, making it easier to detect and respond to security threats.
The Closing Argument
By understanding these common pitfalls, Spring Boot 2.0 developers can build secure authentication systems with Spring Security. Addressing the problems of CORS misconfiguration, lack of HTTPS, poor password storage, inadequate RBAC, unmanaged session expiration, and missing logging and monitoring is crucial to creating robust and secure applications.
For further reading, consider exploring:
- The Spring Security Reference Documentation
- OWASP Top Ten for understanding common vulnerabilities.
As always, stay updated on security practices to ensure your applications remain resilient against emerging threats. Happy coding!