How to Minimize Major GC Pauses for Better Performance
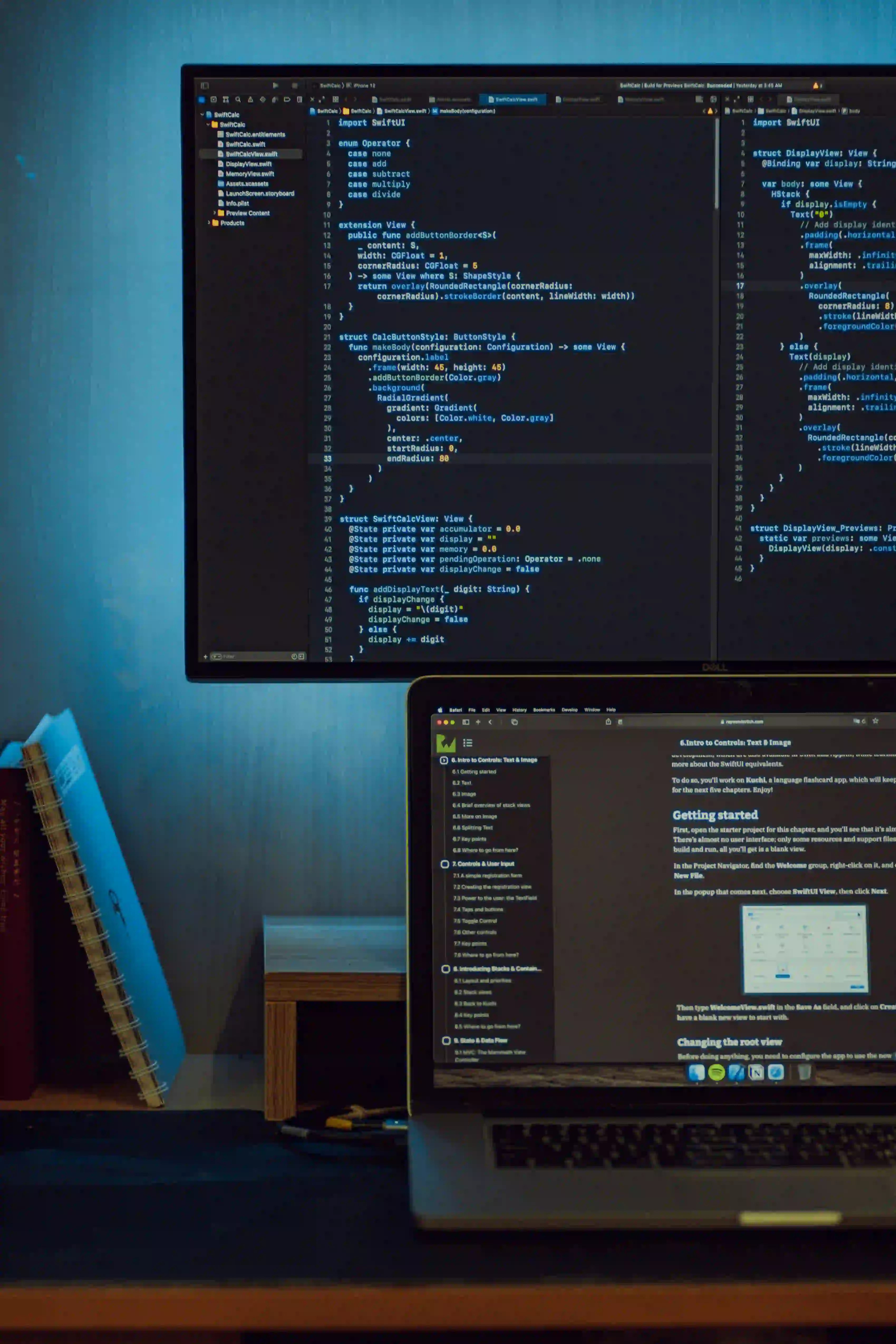
How to Minimize Major GC Pauses for Better Performance in Java
Garbage Collection (GC) is a crucial aspect of Java development that ensures memory management, allowing developers to focus on functionality rather than memory allocation. However, with the power of automatic memory management comes the challenge of GC pauses, particularly major GC pauses, which can significantly impact application performance. In this blog post, we will explore strategies to minimize these pauses, enhance application responsiveness, and improve overall performance.
Understanding Garbage Collection in Java
Before diving into optimization strategies, it's essential to understand how garbage collection works in Java. The Java Virtual Machine (JVM) manages objects in memory, automatically allocating and deallocating resources as needed. There are two main types of garbage collection:
-
Minor GC - This occurs in the Young Generation, which holds short-lived objects. Minor GCs are typically brief, as they primarily deal with objects that are no longer needed.
-
Major GC - This relates to the Old Generation, which holds long-lived objects. Major GCs can result in noticeably longer pauses, affecting application performance.
The goal is to reduce the frequency and duration of major GC pauses, ensuring a smoother user experience.
Strategies for Minimizing Major GC Pauses
1. Optimize Heap Size
One of the most effective ways to reduce major GC pauses is by optimizing heap size. JVM provides parameters to set initial and maximum heap sizes, ensuring that the garbage collector has enough memory to work with.
java -Xms512m -Xmx4096m -jar your-application.jar
Explanation:
-Xms512m
: Sets the initial heap size to 512 MB.-Xmx4096m
: Sets the maximum heap size to 4096 MB.
Having an adequately sized heap reduces the frequency of major GCs. However, be cautious not to allocate too much memory, as it can increase the GC pause time.
2. Utilize Garbage Collector Tuning
The JVM provides several garbage collectors, allowing developers to select the appropriate one based on their application's needs. The two most common collectors are:
- Parallel GC: Suitable for multicore processors.
- G1 GC: Designed for applications requiring low-latency.
java -XX:+UseG1GC -Xmx4096m -jar your-application.jar
Explanation: This command enables the G1 garbage collector, which splits the heap into regions. It prioritizes the collection of regions with the least live data first, reducing major GC pause times.
For more detailed insights on choosing a garbage collector, visit the Oracle documentation on Java Garbage Collection.
3. Minimize Object Creation
Creating too many objects can lead to frequent GCs. To minimize major GC pauses, optimize your code to reduce object creation.
Consider reusing objects instead of creating new instances. For example, using a StringBuilder instead of string concatenation:
StringBuilder sb = new StringBuilder();
for (String item : items) {
sb.append(item).append(",");
}
String result = sb.toString();
Explanation:
- Using
StringBuilder
minimizes the number of intermediateString
objects created. This approach effectively reduces memory pressure and the likelihood of frequent garbage collections.
4. Use the Latest Java Version
Java releases frequently improve garbage collection algorithms. Make it a practice to use the latest available version of Java. New versions often possess enhanced garbage collection strategies and optimizations that can lead to decreased GC pause times.
For instance, Java 11 introduced the Z Garbage Collector (ZGC), aimed at low-latency applications. ZGC can handle heaps of several terabytes with minimal pauses.
5. Analyze Application Performance
Regularly analyze your application's performance using profiling tools. Tools like VisualVM
or JProfiler
can provide insights into memory usage patterns and garbage collection activity.
Example of using VisualVM:
- Launch VisualVM.
- Attach to your running Java application.
- Analyze the heap dumps and garbage collection statistics.
With this data, identify any memory leaks or areas where memory can be optimized.
6. Review and Optimize Data Structures
Choosing the right data structures is essential in minimizing major GC pauses. For example, using an ArrayList
might be more memory-efficient than a LinkedList
due to different memory overheads associated with each structure.
Example:
List<String> list = new ArrayList<>(); // More efficient for random access
In scenarios where object size is minimal, prefer data structures with less overhead.
7. Consider Using Soft and Weak References
Implementing soft and weak references can help manage memory more effectively. Soft references are cleared when the JVM needs memory, while weak references are cleared at the next GC.
import java.lang.ref.SoftReference;
import java.util.HashMap;
public class CacheDemo {
private HashMap<String, SoftReference<Object>> cache = new HashMap<>();
public void put(String key, Object value) {
cache.put(key, new SoftReference<>(value));
}
public Object get(String key) {
SoftReference<Object> reference = cache.get(key);
return reference != null ? reference.get() : null;
}
}
Explanation: Using soft references helps to manage memory by allowing the GC to reclaim memory from objects that are not strongly reachable when the JVM is running low on memory. This approach can potentially reduce the pressure on the heap and mitigate major GC pauses.
To Wrap Things Up
Minimizing major GC pauses in Java is integral to enhancing performance and user experience. By adopting strategies such as optimizing heap size, selecting appropriate garbage collectors, minimizing object creation, and analyzing application performance, you can significantly reduce the impact of garbage collection.
Remember that the balance between performance and memory management differs based on your application type, so it's essential to customize these strategies according to your needs.
For further reading, check out the Java Performance Tuning Guide for in-depth techniques to optimize Java applications.
By applying these principles, you will not only see improvements in application latency and responsiveness but also a better user experience overall. Happy coding!