Overcoming Common Pitfalls of the Twelve-Factor App
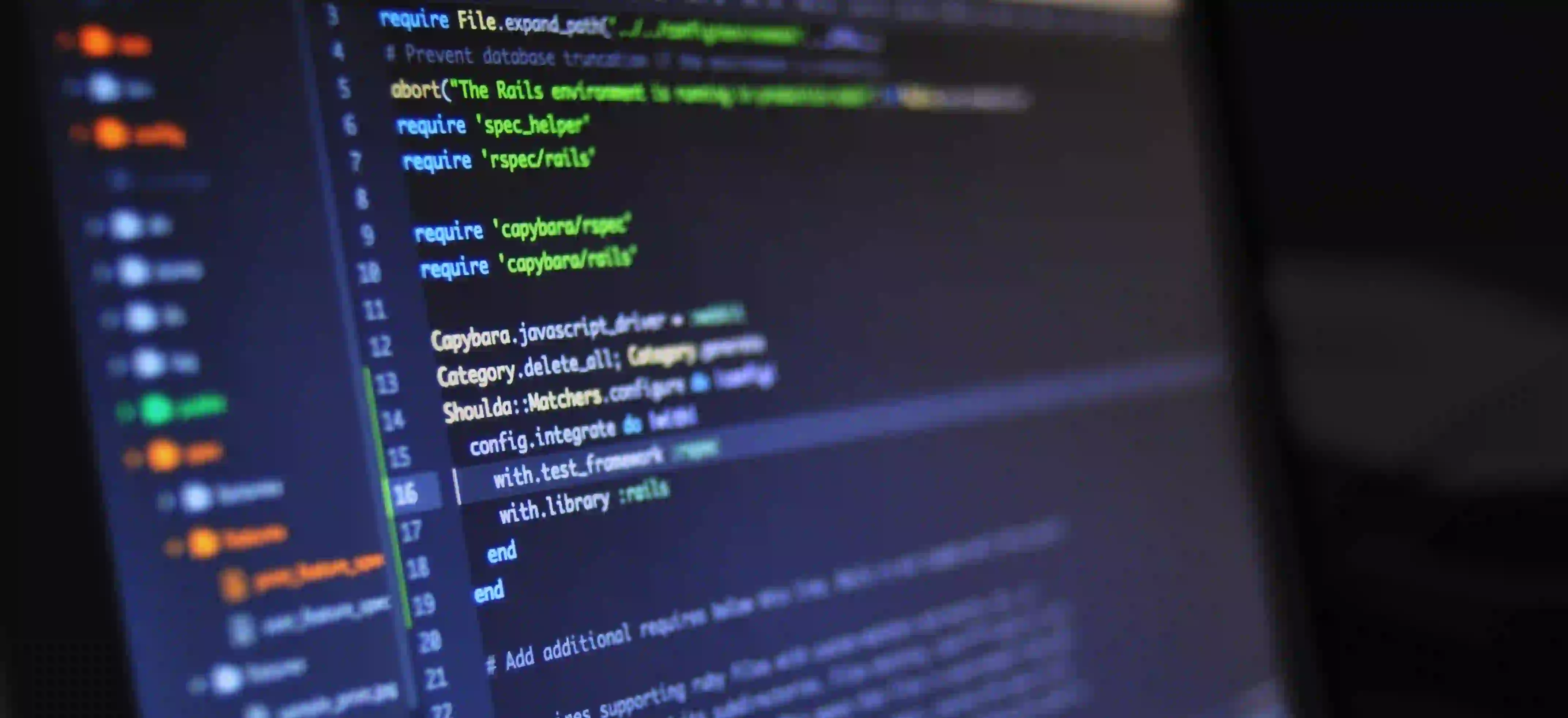
Overcoming Common Pitfalls of the Twelve-Factor App
The Twelve-Factor App is a methodology designed to guide the development of modern web applications. It emphasizes best practices in application design that facilitate scalability, maintainability, and robust deployment processes. Yet, many developers encounter pitfalls when implementing this methodology. This blog post will examine these common challenges and provide solutions to help you overcome them effectively.
What is the Twelve-Factor App?
The Twelve-Factor App is a methodology that offers a set of principles to follow when building cloud-native applications. Every factor is essential, but neglecting any one can lead to complications. Here are the twelve factors:
- Codebase: One codebase tracked in revision control, many deploys.
- Dependencies: Explicitly declaring and isolating dependencies.
- Config: Storing configuration in the environment.
- Backing Services: Treating backing services as attached resources.
- Build, Release, Run: Strictly separating build and run stages.
- Processes: Executing the app as one or more stateless processes.
- Port binding: Exporting services via port binding.
- Concurrency: Scaling out via the process model.
- Disposability: Maximizing robustness with fast startup and graceful shutdown.
- Dev/prod parity: Keeping development, staging, and production as similar as possible.
- Logs: Treating logs as event streams.
- Admin processes: Running administrative tasks as one-off processes.
Understanding the Twelve-Factor App is crucial, but many developers struggle with practical implementation. Let's explore common pitfalls encountered during the development of Twelve-Factor Apps and how to avoid them.
Common Pitfalls and How to Overcome Them
1. Ignoring Dependency Management
Pitfall: Many developers do not explicitly declare dependencies, resulting in inconsistencies across environments.
Solution: Always use a dependency management tool to handle your project's libraries or modules. In Java, tools like Maven or Gradle serve this purpose effectively.
Example with Maven:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>myapp</artifactId>
<version>1.0.0</version>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>2.5.4</version>
</dependency>
</dependencies>
</project>
Using Maven, all project dependencies are defined in the pom.xml
file. This practice isolates dependencies, making your application environment consistent and easier to replicate.
2. Mismanaging Configuration
Pitfall: Storing configuration values hard-coded in your application can lead to environment-specific issues.
Solution: Use environment variables for configuration management. Tools like Spring Boot allow for easy management of configurations using application.properties
or application.yml
.
Example with Spring Boot:
# application.properties
server.port=${SERVER_PORT:8080}
db.url=${DB_URL:jdbc:mysql://localhost:3306/mydb}
By using environment variables, you can configure your application for different environments without changing the codebase. This also aids in security, storing sensitive data outside of source control.
3. Overlooking Disposability
Pitfall: Long-running processes can create problems, especially when it comes to scaling and resource management.
Solution: Design your application processes to be stateless. If necessary, employ a distributed caching solution like Redis to share state across process instances.
Example:
@RestController
public class MyController {
@GetMapping("/data")
public String getData(@RequestParam String userId) {
// Simulating a stateless process
return "Data for user id: " + userId;
}
}
In this example, the controller doesnβt hold onto previous states, allowing for easy scaling and improved robustness.
4. Neglecting Log Management
Pitfall: Treating logs as files, rather than streams, can complicate debugging and monitoring your application.
Solution: Ensure your application outputs logs to standard output (stdout) and utilize a log management solution (e.g., ELK Stack) to analyze log data.
Example with Logback:
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} - %msg%n</pattern>
</encoder>
</appender>
<root level="INFO">
<appender-ref ref="STDOUT"/>
</root>
</configuration>
Using a logging framework allows you to treat logs as event streams, improving the application's observability and maintainability.
5. Ignoring Process Separation
Pitfall: Mixing build and runtime environments can lead to unforeseen issues, particularly with dependencies and configurations.
Solution: Adopt CI/CD (Continuous Integration and Continuous Deployment) practices that enforce the separation of the build, release, and run stages.
Using Jenkins for CI/CD, you might configure your pipeline like this:
pipeline {
agent any
stages {
stage('Build') {
steps {
// Compile and package application
sh 'mvn clean package'
}
}
stage('Deploy') {
steps {
// Deploy to environment
sh 'docker-compose up -d'
}
}
}
}
This separation simplifies troubleshooting and ensures that the application behaves consistently in different environments.
6. Poor Backing Service Management
Pitfall: Treating backing services (like databases, caches, etc.) as hardcoded resources can lead to complications in scaling and maintenance.
Solution: Abstract backing services to treat them as attached resources rather than embedded in your code.
Example configuration for a backing service (database in Spring Boot):
spring:
datasource:
url: ${DB_URL:jdbc:mysql://localhost:3306/mydb}
username: ${DB_USERNAME:root}
password: ${DB_PASSWORD:password}
This approach allows for easy swapping of services or changing configurations without altering application code.
7. Stagnant Dev/Prod Parity
Pitfall: When development and production environments diverge too much, it can lead to unexpected behavior upon deployment.
Solution: Use tools like Docker to ensure all environments are as similar as possible, thereby improving the reliability of deployments.
Example of a simple Dockerfile:
FROM openjdk:11-jre-slim
COPY target/myapp.jar /app/myapp.jar
CMD ["java", "-jar", "/app/myapp.jar"]
By containerizing your application, you can maintain parity between development, staging, and production, reducing the risk of environment-specific issues.
My Closing Thoughts on the Matter
Implementing the Twelve-Factor App methodology doesn't guarantee success, but it significantly reduces the chances of common pitfalls that can derail your project. Awareness and proactive management of dependency, configuration, logging, process separation, backing services, and environmental parity set you on a path to building a scalable, maintainable, and robust cloud-native application.
By emphasizing each of these factors throughout the development lifecycle, you are more likely to create an application that not only meets immediate needs but also scales effectively with your business. For developers eager to dive deeper into this methodology, the official Twelve-Factor App website provides comprehensive resources and examples.
Happy coding!