Solving N+1 Query Issue in Hibernate Lazy Loading
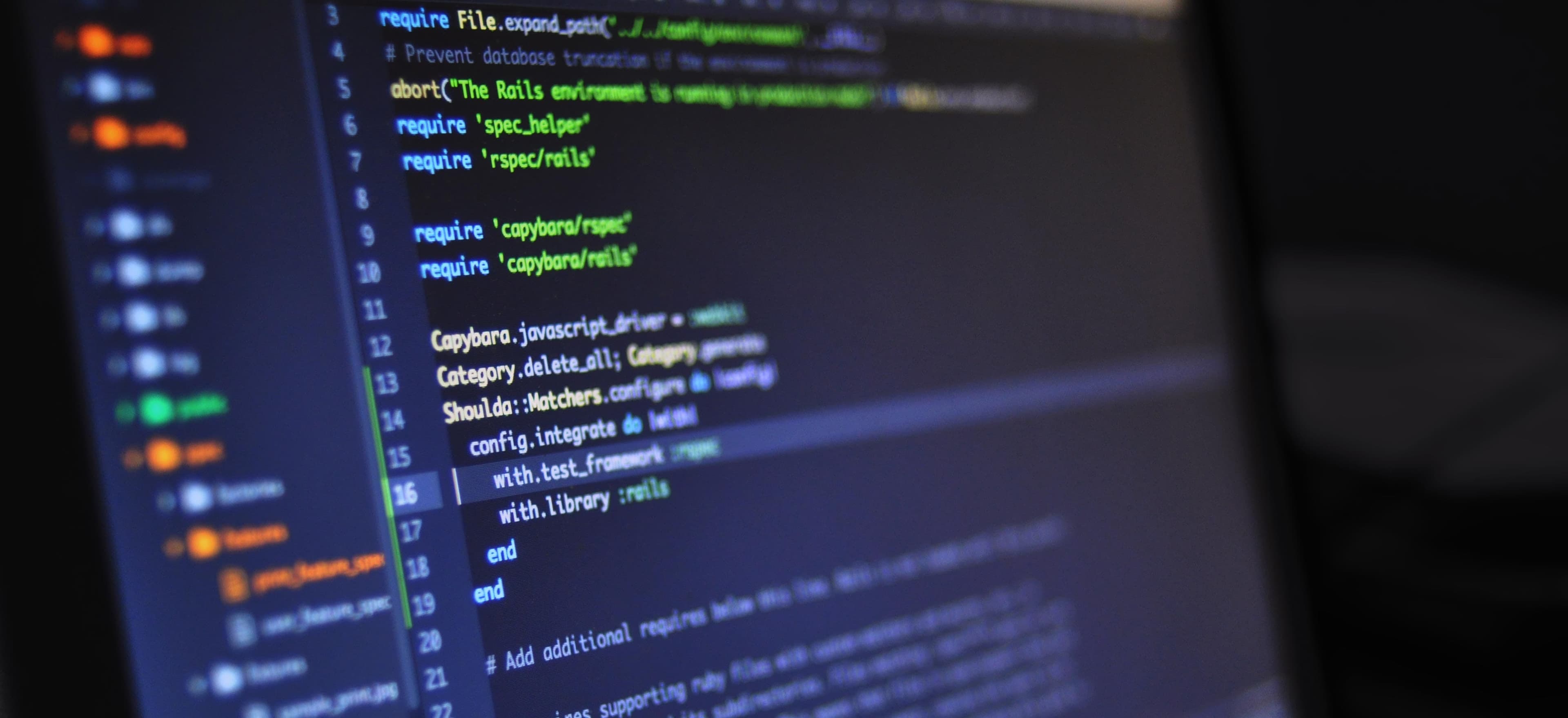
- Published on
Solving the N+1 Query Issue in Hibernate Lazy Loading
When it comes to working with Hibernate, developers often face the challenge known as the N+1 query problem. This can have a significant impact on the performance of your application, particularly in scenarios with multiple relationships between entities. In this blog post, we will explore the N+1 query issue in Hibernate's lazy loading, discuss its implications, and provide several solutions to mitigate or eliminate the problem.
Understanding the N+1 Query Problem
The N+1 query problem occurs when an application makes one initial query to retrieve a list of entities, followed by N additional queries to fetch related entities for each of the N results. This can be particularly detrimental in performance-critical applications, as it leads to increased latency and inefficiency.
A Simple Example
Consider a simple scenario where we have two entities: Author
and Book
. Each author can have multiple books, and we want to fetch all authors along with their associated books.
Imagine the following code snippet:
List<Author> authors = session.createQuery("FROM Author", Author.class).getResultList();
for (Author author : authors) {
System.out.println(author.getName());
// This line will trigger an additional query for each author
System.out.println(author.getBooks().size());
}
In this example, when we access author.getBooks()
, Hibernate generates an additional query for each author to fetch their books, resulting in an N+1 query scenario.
The Impact
The performance hog here is significant. If you have 100 authors, you'd end up executing 101 queries: 1 for fetching all authors and 100 for fetching each author's books. This inefficiency can lead to slower response times and increased database load.
Solutions to the N+1 Query Problem
Fortunately, there are several strategies to circumvent the N+1 query issue when using Hibernate. Below, we dive into some of the most effective methods.
1. Eager Loading
Eager loading retrieves all associated entities in a single query instead of making additional queries for lazy-loaded entities. You can achieve this by using the JOIN FETCH
clause.
Example
You can modify your query as follows:
List<Author> authors = session.createQuery(
"SELECT DISTINCT a FROM Author a JOIN FETCH a.books", Author.class)
.getResultList();
for (Author author : authors) {
System.out.println(author.getName());
System.out.println(author.getBooks().size());
}
Why Use Eager Loading?
- Efficiency: By reducing the number of queries, it minimizes round trips to the database.
- Simplicity: It simplifies code logic since you do not need to manage lazy-loading.
2. Batch Fetching
Batch fetching is another approach you can use, which allows Hibernate to load a set amount of lazily loaded entities in batches instead of one at a time.
Example
You can configure batch fetching in your entity mapping using the @BatchSize
annotation:
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "author")
@BatchSize(size = 10) // Fetch 10 books at a time
private List<Book> books;
}
Why Batch Fetching?
- More Control: You control how many entities are fetched in one go.
- Performance: It reduces the total number of database calls while maintaining flexibility.
3. DTO Projections
Instead of fetching entire entities, you can use Data Transfer Objects (DTOs) to shape the data as needed. This approach can minimize the data transferred over the network.
Example
You can create a DTO model:
public class AuthorDTO {
private String name;
private List<String> bookTitles;
// constructor, getters, setters, etc.
}
Then, you can fetch data like this:
List<AuthorDTO> authors = session.createQuery(
"SELECT new AuthorDTO(a.name, b.title) FROM Author a JOIN a.books b", AuthorDTO.class)
.getResultList();
Why Use DTO Projections?
- Minimal Data Transfer: Only fetch the fields you really need, reducing bandwidth.
- Decoupling: It separates your database layer from your business layer.
4. Hibernate Filters
If you need to limit results based on specific criteria, Hibernate Filters can help manage this complexity without the N+1 query problem.
Example
First, define a filter in your entity:
@Entity
public class Author {
// ...
@OneToMany(mappedBy = "author")
@Filter(name = "bookFilter", condition = "active = true") // Only fetch active books
private List<Book> books;
}
Then, enable the filter in your session:
session.enableFilter("bookFilter");
List<Author> authors = session.createQuery("FROM Author", Author.class).getResultList();
Why Use Filters?
- Conditional Fetching: Allows you to dynamically control what related entities should be loaded.
- Seamless Integration: Works well within your existing Hibernate configurations.
Lessons Learned
The N+1 query problem can be a formidable foe for developers using Hibernate and lazy loading. By understanding how it manifests and employing one or more of the techniques we've discussed, you'll be well on your way to optimizing your application.
When deciding which solution to implement, consider factors like the complexity of your domain model, the performance needs of your application, and the potential impact on code readability. Always measure performance before and after to validate the effectiveness of your approach.
For further reading and more in-depth examples, check out:
- Hibernate Documentation
- Understanding Hibernate Fetch Strategies
Implement these strategies wisely, and you will significantly improve your application's performance while maintaining clean and maintainable code. Happy coding!
Checkout our other articles