Mastering FlowPane in JavaFX: Common Layout Pitfalls
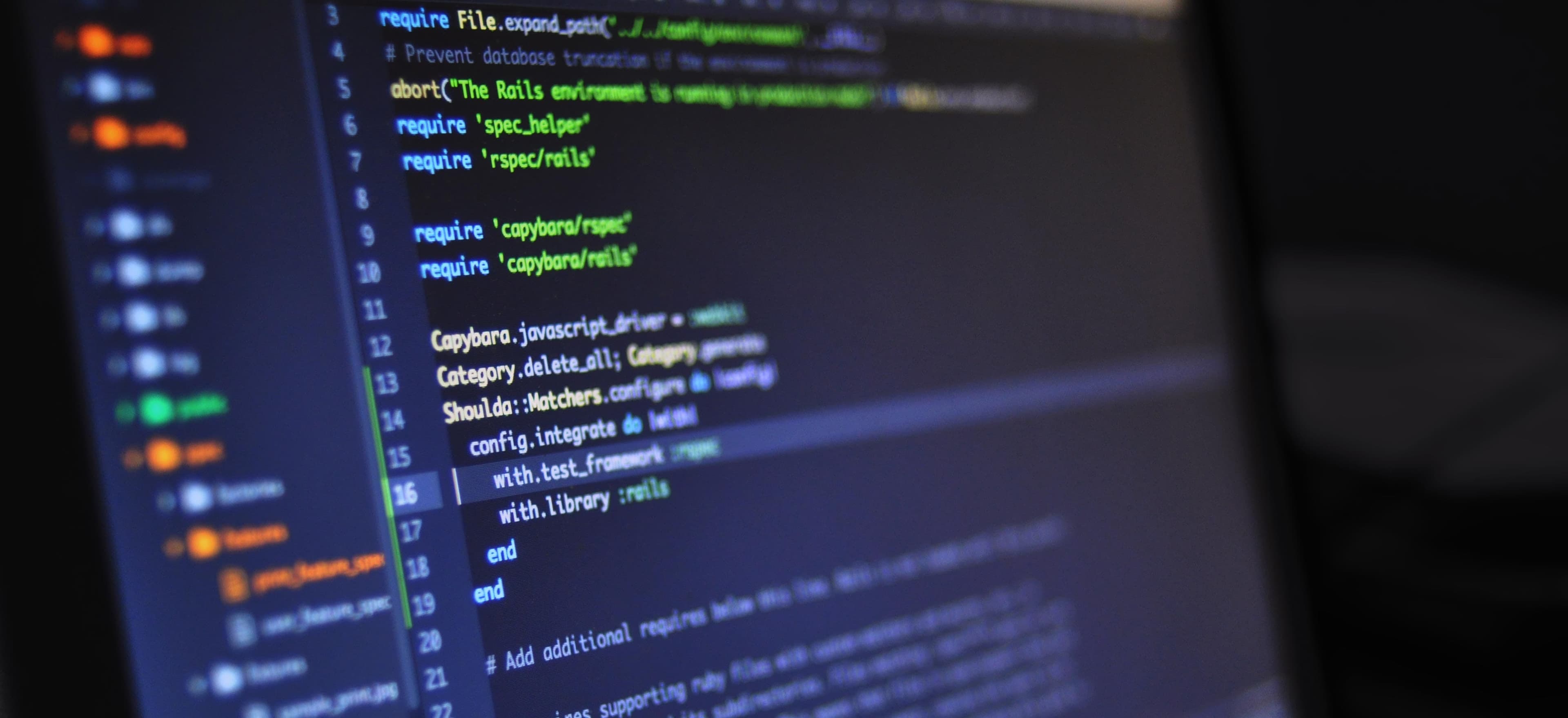
- Published on
Mastering FlowPane in JavaFX: Common Layout Pitfalls
JavaFX is a powerful framework that allows developers to create rich client applications with great flexibility in terms of design and functionality. One of the layout panes that JavaFX provides is the FlowPane. This layout is particularly useful for positioning nodes in a flow, similar to how words wrap in a paragraph. However, mastering FlowPane involves understanding its specific characteristics and potential pitfalls in usage.
In this article, we will delve into the intricacies of FlowPane, explore some common pitfalls, and provide you with best practices to enhance your JavaFX applications.
What is FlowPane?
FlowPane arranges its children in a flow, wrapping them as needed. Its primary attributes are horizontal and vertical gaps, which provide spacing between the nodes. FlowPane is particularly useful for applications where you have dynamic content or variable-sized nodes.
Here’s a simple example to demonstrate how FlowPane operates:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class FlowPaneDemo extends Application {
@Override
public void start(Stage primaryStage) {
FlowPane flowPane = new FlowPane(); // Create a FlowPane
flowPane.setHgap(10); // Set horizontal gap
flowPane.setVgap(10); // Set vertical gap
// Add buttons to the FlowPane
for (int i = 1; i <= 10; i++) {
Button button = new Button("Button " + i);
flowPane.getChildren().add(button);
}
Scene scene = new Scene(flowPane, 400, 200);
primaryStage.setTitle("FlowPane Demo");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Use FlowPane?
FlowPane is a great choice when you want a simple layout that automatically adjusts to the size of the window. It wraps the nodes as per the available horizontal space, making it highly responsive and dynamic.
Common Pitfalls with FlowPane
1. Unrealistic Expectations on Node Sizing
One of the major pitfalls new developers encounter is not being conscious of how FlowPane handles node sizing. Each node can resize differently based on its content, which can lead to unexpected placements.
Example:
If you add buttons with varying lengths, the FlowPane may not align them as you expect.
Button shortButton = new Button("Short");
Button longButton = new Button("This is a long button");
flowPane.getChildren().addAll(shortButton, longButton);
Commentary: To prevent this, ensure that you explicitly set the preferred size of nodes. For example:
shortButton.setPrefWidth(100);
longButton.setPrefWidth(100);
2. Ignoring Gaps
Another common pitfall is neglecting gaps. If you set both horizontal and vertical gaps to zero, the layout may appear crowded, and users might struggle to interact with the controls.
flowPane.setHgap(0);
flowPane.setVgap(0);
Commentary: By defining adequate gaps, you create a more visually appealing interface. A setting of 10 pixels for each gap usually makes for a cleaner layout.
3. Underestimating Dynamic Content Changes
FlowPane will rearrange nodes when new nodes are added or removed. If you do not consider the implications of dynamic changes, the layout might appear unexpectedly.
Example:
Button newButton = new Button("New Button");
flowPane.getChildren().add(newButton);
Commentary: Keep in mind how your FlowPane will behave over time, especially with action events like button clicks that may alter the number of children. Make sure to design with this dynamism in mind.
4. Not Leveraging Binding
Another pitfall is overlooking JavaFX property binding. Not leveraging this feature can make your application less responsive to changes in data or user interactions.
Example:
Label sizeLabel = new Label();
sizeLabel.textProperty().bind(new SimpleStringProperty("FlowPane size: " + flowPane.getChildren().size()));
Commentary: This binding will automatically update the size label whenever the contents of the FlowPane change, delivering a more interactive user experience.
Best Practices
1. Specify Node Sizes
Always specify preferred, min, and max sizes for your nodes when using the FlowPane. This ensures a cohesive look regardless of the underlying data being displayed.
2. Set Gaps
Set both horizontal and vertical gaps to ensure adequate spacing between nodes. This leads to a more user-friendly interface.
3. Use Flexibility Wisely
When designing your application, understand what flexibility you need with your nodes. Using anchors or alignment can be beneficial.
flowPane.setAlignment(Pos.CENTER); // Optional, for center alignment
4. Embrace Dynamic Behavior
Take advantage of the dynamic nature of FlowPane. Utilize listeners or bindings to handle changes. This can improve the responsiveness of your UI significantly.
In Conclusion, Here is What Matters
FlowPane in JavaFX offers a flexible way to layout your application components. However, navigating its quirks requires an understanding of potential pitfalls. By specifying node sizes, setting appropriate gaps, leveraging binding, and embracing the dynamic nature of the layout, you can master FlowPane to create efficient and attractive applications.
Take these lessons into account as you develop your JavaFX projects. For more information and deeper exploration, you may find resources like the official JavaFX documentation and JavaFX tutorial series useful.
Happy coding!