Common Pitfalls When Using Spring MVC 4 Quickstart
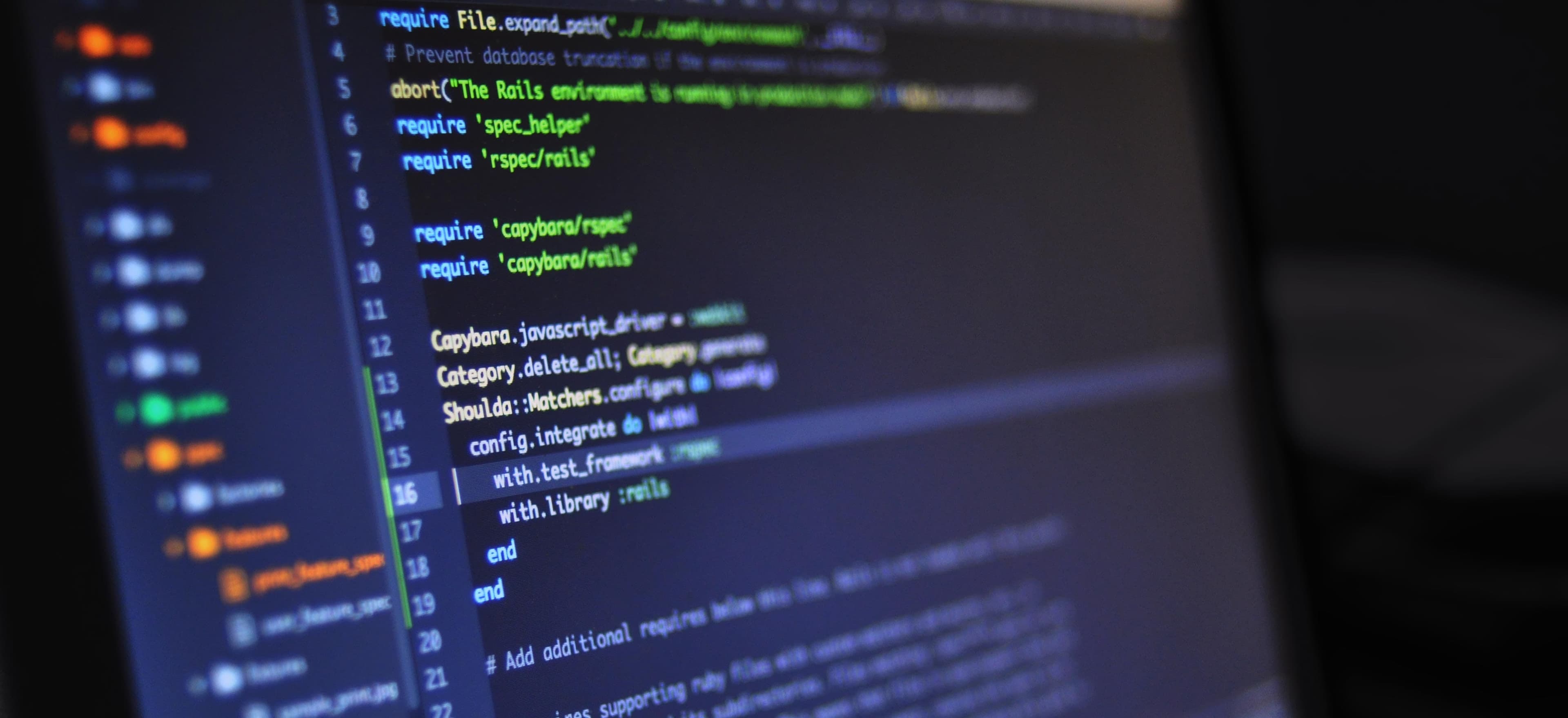
- Published on
Common Pitfalls When Using Spring MVC 4 Quickstart
Spring Framework is a versatile and powerful tool for building Java applications. Among its various modules, Spring MVC provides a robust framework for developing web applications. However, when starting with Spring MVC 4, developers often encounter some common pitfalls that can lead to frustration. In this post, we will explore these pitfalls and offer actionable solutions.
What is Spring MVC?
Spring MVC is a model-view-controller (MVC) architecture and framework that provides a way to develop web applications in a loosely coupled and maintainable manner. It constructs a clean separation of concerns, enabling developers to manage application components effectively.
Common Pitfalls
1. Misconfiguration of DispatcherServlet
The first hurdle most beginners face is the misconfiguration of the DispatcherServlet
. This servlet is the heart of the Spring MVC framework, responsible for routing requests to appropriate handlers.
Solution
Make sure that you have correctly defined the DispatcherServlet
in your web.xml
. Here is an example:
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
Why: Failing to configure this servlet correctly will lead to 404 errors or requests not being handled at all.
2. Ignoring @Controller
Annotations
Another common mistake is neglecting to use the @Controller
annotation on your controller classes. This annotation is crucial for Spring to recognize them as controllers.
Solution
Always annotate your controller classes like so:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HomeController {
@RequestMapping("/")
public String index() {
return "index"; // View name
}
}
Why: Without @Controller
, Spring will not treat the class as a handler for web requests, leading to a lack of response to client requests.
3. Poorly Configured View Resolvers
View resolvers are essential for determining how views are resolved. Many developers underestimate their importance.
Solution
Ensure that you have configured a view resolver in your Spring configuration:
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/" />
<property name="suffix" value=".jsp" />
</bean>
Why: A misconfigured view resolver will cause org.springframework.web.servlet.ViewResolver
to fail to locate your JSP files, resulting in view-related errors.
4. Ignoring the Model Attribute
When sending data from your controller to the view, developers sometimes forget to add model attributes.
Solution
Always use model attributes in your controller methods:
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloWorldController {
@GetMapping("/hello")
public String hello(Model model) {
model.addAttribute("message", "Hello, World!");
return "hello"; // View name
}
}
Why: Adding model attributes is essential for transferring data to the view correctly, without which your JSPs or HTML templates may lack the required data.
5. Not Handling Exceptions Properly
Ignoring error handling can lead to poor user experiences. Spring MVC allows you to manage exceptions centrally using @ControllerAdvice
.
Solution
Define a global exception handler:
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR)
public String handleAllExceptions(Exception ex) {
// Log error messages
return "error"; // View name for error page
}
}
Why: Handling exceptions allows you to display user-friendly error messages instead of the standard error pages, enhancing user experience.
6. Overusing the @RequestMapping
Annotation
Using @RequestMapping
on every method can lead to cluttered code.
Solution
Utilize method-specific annotations like @GetMapping
, @PostMapping
, @PutMapping
, and @DeleteMapping
to keep your code clean:
@GetMapping("/users")
public String listUsers(Model model) {
model.addAttribute("users", userService.getAllUsers());
return "userList"; // View name
}
Why: Using specific annotations increases code readability and makes your intentions clear.
Best Practices for Using Spring MVC 4
1. Use Dependency Injection
Utilize Spring's dependency injection capabilities to manage your service layers. This will improve testability and reduce coupling.
Example:
@Service
public class UserService {
// User service code
}
@Controller
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
}
2. Adhere to RESTful Principles
If you're building a RESTful API, make sure to adhere to best practices around HTTP methods and response codes.
3. Enable CORS
If you're developing SPAs or mobile applications, enabling Cross-Origin Resource Sharing (CORS) will facilitate seamless API consumption.
import org.springframework.web.bind.annotation.CrossOrigin;
@CrossOrigin(origins = "http://example.com")
@Controller
public class CrossOriginController {
// Your methods here
}
4. Testing Your Application
Always write unit tests for your controllers and services to ensure your code is functioning as expected. Utilizing frameworks like JUnit and Mockito alongside Spring Test can help streamline your testing process.
5. Stay Updated
Spring MVC is part of the larger Spring ecosystem, which receives frequent updates. Make sure to keep your dependencies current to leverage security updates and new features.
In Conclusion, Here is What Matters
Spring MVC is a powerful framework that can significantly simplify Java web development. However, starting with it comes with its own set of challenges. By being mindful of common pitfalls like misconfigurations, ignoring model attributes, and neglecting exception handling, you can streamline your development processes significantly.
Above all, remember that learning is iterative; mistakes are part of the journey. By understanding the ‘why’ behind these pitfalls, you will be better equipped to build robust applications. If you want to dive deeper into Spring MVC, consider checking out the Spring MVC Documentation and the Spring Guides for practical examples and best practices.
Embrace the challenges, and happy coding!
Checkout our other articles