Understanding Java's Path Methods: Avoiding Common Confusions
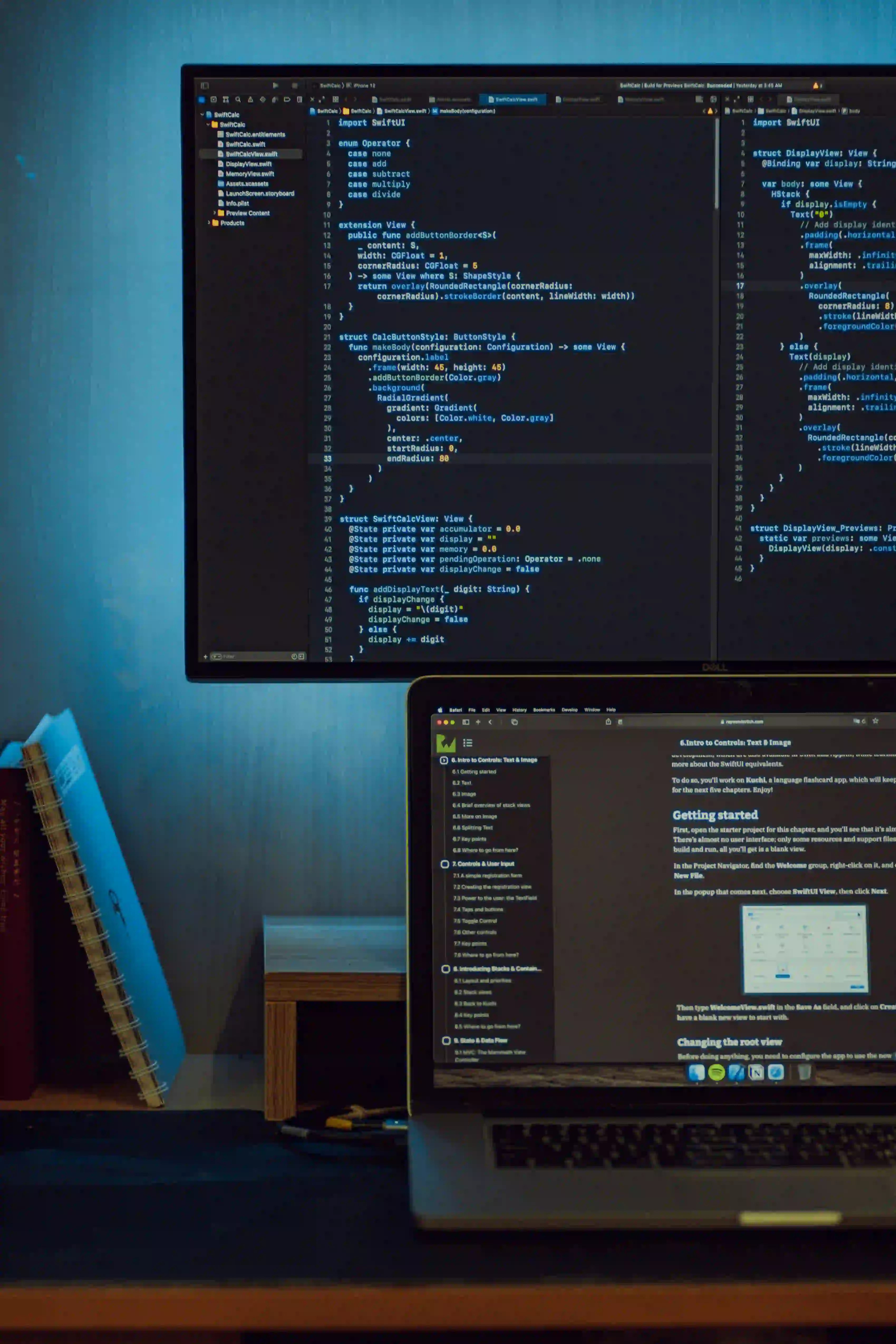
Understanding Java's Path Methods: Avoiding Common Confusions
When working with file systems in Java, the java.nio.file.Path
class plays a crucial role. However, its methods can be confusing for both new and experienced developers. This blog post will demystify the Path
methods, explore their functionalities, provide clear examples, and help you avoid common pitfalls.
What is a Path?
In Java, a Path
instance represents a file system path and can be used to handle file system operations. Essentially, it abstracts the details of file paths and provides a useful API that makes handling files easier and more efficient.
The Path
class is part of the NIO (New I/O) package, which was introduced in Java 7 to improve file handling. For more details about the NIO package, you can refer to the Java NIO documentation.
Creating a Path
Creating a path is a straightforward operation using the Paths
class as follows:
import java.nio.file.Paths;
import java.nio.file.Path;
public class PathExample {
public static void main(String[] args) {
// Creating a Path object for a specific directory
Path path = Paths.get("C:/example/directory/file.txt");
System.out.println("Path: " + path);
}
}
In this code snippet, we use Paths.get()
to create a Path
object that represents a file path. Adjust the string argument as needed for your file system.
The Basic Methods of Path
The Path
class offers methods for path manipulation. Let's explore some of the most commonly used methods.
1. Getting the File Name
To extract the file name from a path, use the getFileName()
method:
Path path = Paths.get("C:/example/directory/file.txt");
System.out.println("File Name: " + path.getFileName());
Why: This method returns the name of the file or directory represented by this path, which is often necessary for display or logging.
2. Getting the Parent Directory
The getParent()
method provides the parent directory of the path:
Path path = Paths.get("C:/example/directory/file.txt");
System.out.println("Parent Directory: " + path.getParent());
Why: Knowing the parent directory is essential for various file operations, such as traversing the file structure.
3. Normalizing Paths
To eliminate redundant elements in a path, use normalize()
:
Path path = Paths.get("C:/example/./directory/../file.txt").normalize();
System.out.println("Normalized Path: " + path);
Why: Normalization helps in standardizing paths, avoiding errors due to extra .
or ..
components.
4. Resolving Paths
Paths can be resolved using resolve()
:
Path basePath = Paths.get("C:/example/directory");
Path resolvedPath = basePath.resolve("file.txt");
System.out.println("Resolved Path: " + resolvedPath);
Why: This method appends the specified path to the current path, which is useful for constructing paths dynamically.
Common Confusions with Path Methods
While working with Path
methods, developers often encounter subtle pitfalls. Let’s address some common confusions.
Relative vs. Absolute Paths
Often, developers misinterpret relative paths. Relative paths depend on the current working directory, while absolute paths start from the root.
To create an absolute path, ensure you start from the root:
Path absolutePath = Paths.get("C:/example/directory");
Path relativePath = Paths.get("directory/file.txt");
System.out.println("Absolute Path: " + absolutePath.toAbsolutePath());
System.out.println("Relative Path: " + relativePath.toAbsolutePath());
Why: Understanding the difference is key to file operations. If you intend to manipulate the file system effectively, knowing how paths relate to each other is crucial.
The Difference between toString() and asUri()
Using toString()
converts a Path
to its string representation. However, using toUri()
creates a URI out of the path, which can lead to confusion.
Path path = Paths.get("C:/example/directory/file.txt");
System.out.println("String Representation: " + path.toString());
System.out.println("URI Representation: " + path.toUri());
Why: A URI (Uniform Resource Identifier) may be required in some cases, especially when integrating with web services or databases.
Advanced Path Manipulations
File Systems
To understand how Path interacts with different file systems, consider using the FileSystems
class:
import java.nio.file.FileSystems;
System.out.println("Default File System: " + FileSystems.getDefault());
Why: Using this class allows you to understand the current file system, which can be critical when dealing with diverse environments.
Path Comparison
Comparing paths can also create confusion. Use the equals()
method:
Path path1 = Paths.get("C:/example/directory/file.txt");
Path path2 = Paths.get("C:/example/directory/file.txt");
System.out.println("Paths are equal: " + path1.equals(path2));
Why: It’s important to understand that paths can point to the same location but are not identical in the absolute sense (e.g., different casing or different representations).
Dealing with Exceptions
When working with paths, always be prepared to handle potential exceptions, especially when dealing with file systems.
import java.nio.file.Files;
import java.nio.file.NoSuchFileException;
try {
Files.readAllLines(path);
} catch (NoSuchFileException e) {
System.out.println("File not found: " + e.getMessage());
} catch (IOException e) {
System.out.println("IO error occurred: " + e.getMessage());
}
Why: Exception handling is crucial in robust applications, ensuring that file operations perform seamlessly without crashing the application.
Summary
Understanding and using Java's Path
methods proficiently is essential for any developer working with files. By mastering these techniques, avoiding common pitfalls, and implementing robust exception handling, you can enhance your application's performance and reliability.
For a more thorough exploration, consider reading official resources like the Java Tutorials on the NIO package.
Feel free to experiment with the examples given here, modify them as per your requirements, and integrate them into your applications. Happy coding!