Common Pitfalls of Lambda Expressions in Java 8
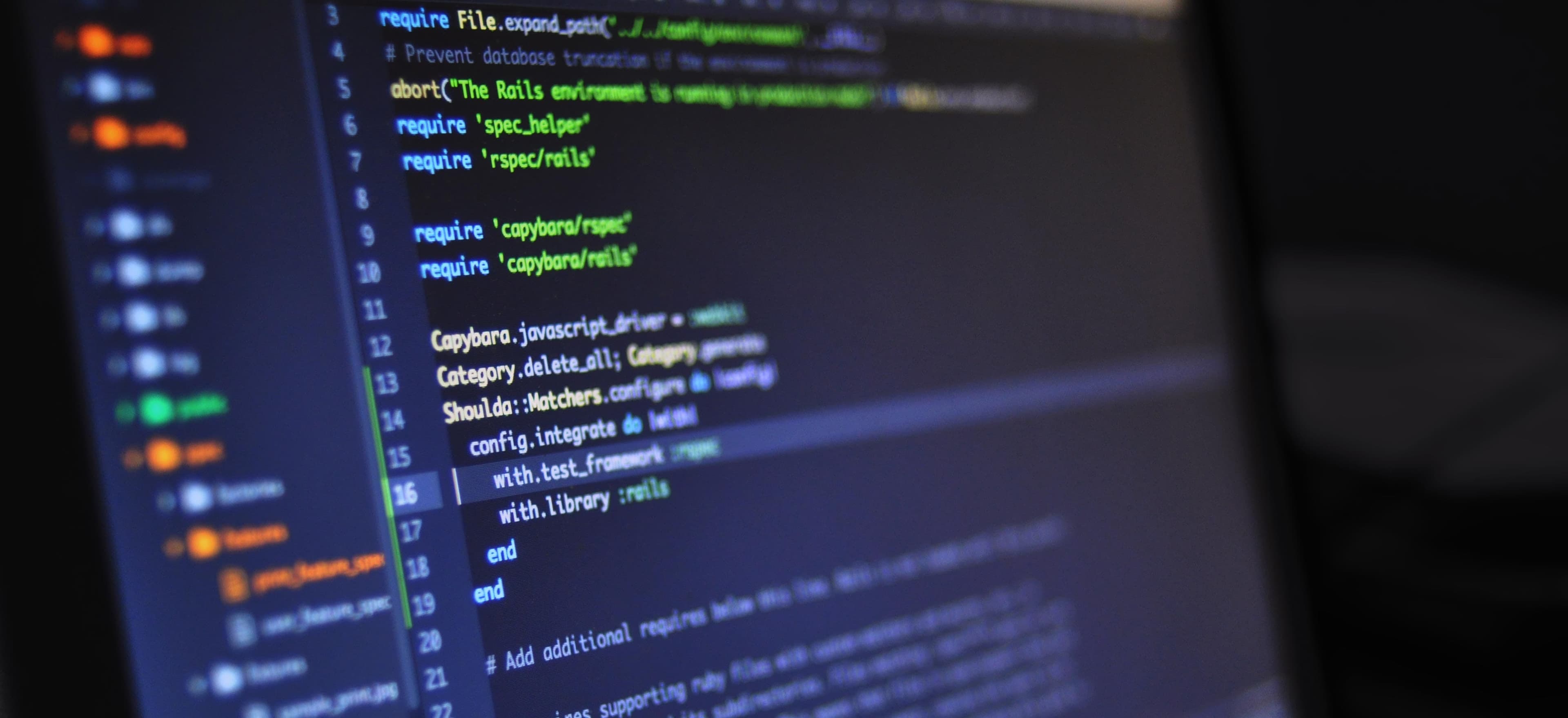
- Published on
Common Pitfalls of Lambda Expressions in Java 8
Java 8 brought a revolutionary change to the Java programming landscape, introducing a plethora of features that facilitate functional programming. Among these, lambda expressions stand out as a common yet powerful tool for writing cleaner and more concise code. However, with great power comes great responsibility. Many developers encounter pitfalls when using lambda expressions. In this blog post, we will explore these common pitfalls and how to avoid them.
What are Lambda Expressions?
Before diving into the pitfalls, it’s essential to clarify what lambda expressions are. A lambda expression in Java is a succinct way to represent an anonymous function. It provides a clear and concise syntax for creating function interfaces.
Here is a fundamental example of a lambda expression:
// Classic usage of a lambda expression
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> {
System.out.println(name);
});
In this snippet, we achieve the same functionality as traditional iteration but with much less code. The syntax allows developers to implement functional interfaces in a clearer way.
Pitfall 1: Type Inference Issues
The Problem
One of the frequent mistakes developers make is mismanaging type inference. While Java does an excellent job of inferring types in most cases, there are situations where it may not infer the expected types correctly.
The Solution
To avoid ambiguity, you should declare the parameter types explicitly when writing lambda expressions.
Example:
// Ambiguous lambda expression – may lead to inference issues
Function<String, String> toUpperCase = s -> s.toUpperCase();
// Clearer with explicit types
Function<String, String> toUpperCaseExplicit = (String s) -> s.toUpperCase();
In this case, explicitly declaring the type helps the compiler and developers understand the intended use better, reducing the margins for error.
Pitfall 2: Capturing Variables
The Problem
Lambda expressions can capture variables from their surroundings, which is both a feature and a potential pitfall. When a lambda expression captures a mutable variable, it can lead to unexpected results, especially in loops.
The Solution
Prefer using final or effectively final variables in your lambda expressions. This practice ensures that the captured variable state isn’t unintentionally modified.
Example:
// Problematic scenario
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
for (String name : names) {
String greeting = "Hello, ";
// Modification of greeting can lead to confusion
greeting += name;
new Thread(() -> System.out.println(greeting)).start();
}
The above code snippet could lead to unexpected behavior, as greeting
changes with each loop iteration. Here’s how we could fix it:
// Correct usage with effectively final variable
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
for (String name : names) {
final String greeting = "Hello, " + name; // now effectively final
new Thread(() -> System.out.println(greeting)).start();
}
This fix ensures that greeting
remains immutable, preventing potential bugs.
Pitfall 3: Overuse of Lambda Expressions
The Problem
While lambda expressions promote concise code, using them excessively can lead to reduced readability, especially when they are lengthy or complex. Sticking to the principle of clarity is paramount.
The Solution
Exercise discretion when opting for lambda expressions. If a lambda becomes too complex or spans several lines, consider extracting it into a named method for clarity.
Example:
// Overly complex lambda expression
List<String> namesSorted = names.stream()
.filter(name -> {
if (name.startsWith("A")) {
return true;
}
return name.length() > 3;
})
.collect(Collectors.toList());
Instead, you could define a method:
// Named method for clarity
private boolean filterCondition(String name) {
return name.startsWith("A") || name.length() > 3;
}
// Using the named method
List<String> namesSorted = names.stream()
.filter(this::filterCondition)
.collect(Collectors.toList());
This change enhances readability and makes your intent clearer.
Pitfall 4: Exception Handling
The Problem
Handling checked exceptions in lambda expressions can be cumbersome. Since lambda expressions cannot throw checked exceptions directly, developers often find themselves struggling with error handling.
The Solution
One common pattern to circumvent this issue is to create a utility method or use a custom functional interface that can handle checked exceptions.
Example:
// Defining a custom functional interface for checked exceptions
@FunctionalInterface
interface ThrowingFunction<T, R> {
R apply(T t) throws Exception;
}
// A utility method to handle exceptions
public static <T, R> Function<T, R> wrap(ThrowingFunction<T, R> throwingFunction) {
return t -> {
try {
return throwingFunction.apply(t);
} catch (Exception e) {
throw new RuntimeException(e);
}
};
}
// Usage of the utility method
List<String> values = Arrays.asList("1", "2", "three");
values.stream()
.map(wrap(value -> Integer.parseInt(value))) // Safely handle the checked exception
.collect(Collectors.toList());
This approach allows you to maintain clean and concise lambda expressions while still managing exception handling logically.
Pitfall 5: Concurrency Issues
The Problem
Lambda expressions are often used in multi-threaded environments, but capturing mutable state can lead to concurrency issues. Not being aware of how shared mutable variables might behave in different threads can lead to inconsistencies.
The Solution
Minimize mutable shared states and use thread-safe constructs like synchronized blocks or volatile variables.
Example:
// Shared mutable state can be problematic in a concurrent environment
List<String> names = Collections.synchronizedList(new ArrayList<>());
Runnable task = () -> {
names.add("New Name"); // This can cause issues if not thread-safe
};
new Thread(task).start();
Instead, prefer immutability or synchronization:
// Using a synchronized list or thread-safe collection
List<String> names = new CopyOnWriteArrayList<>();
Runnable task = () -> {
names.add("New Name"); // Thread-safe
};
new Thread(task).start();
By using thread-safe collections, we can mitigate concurrency issues effectively.
My Closing Thoughts on the Matter
While lambda expressions offer a modern approach to writing cleaner Java code, it’s essential to be aware of these common pitfalls. Understanding type inference, variable capturing, readability, exception handling, and concurrency can significantly enhance your programming experience. By incorporating cautious practices when using lambda expressions, you can unlock their full potential while keeping your code robust and maintainable.
For more extensive Java programming lessons, check out Oracle’s official Java documentation on functional interfaces, or gain further insights into functional programming with Java from Baeldung.
Embrace the power of lambda expressions with caution, and make your Java code even more elegant!
Checkout our other articles