Mastering Spring MVC: Common Configuration Pitfalls
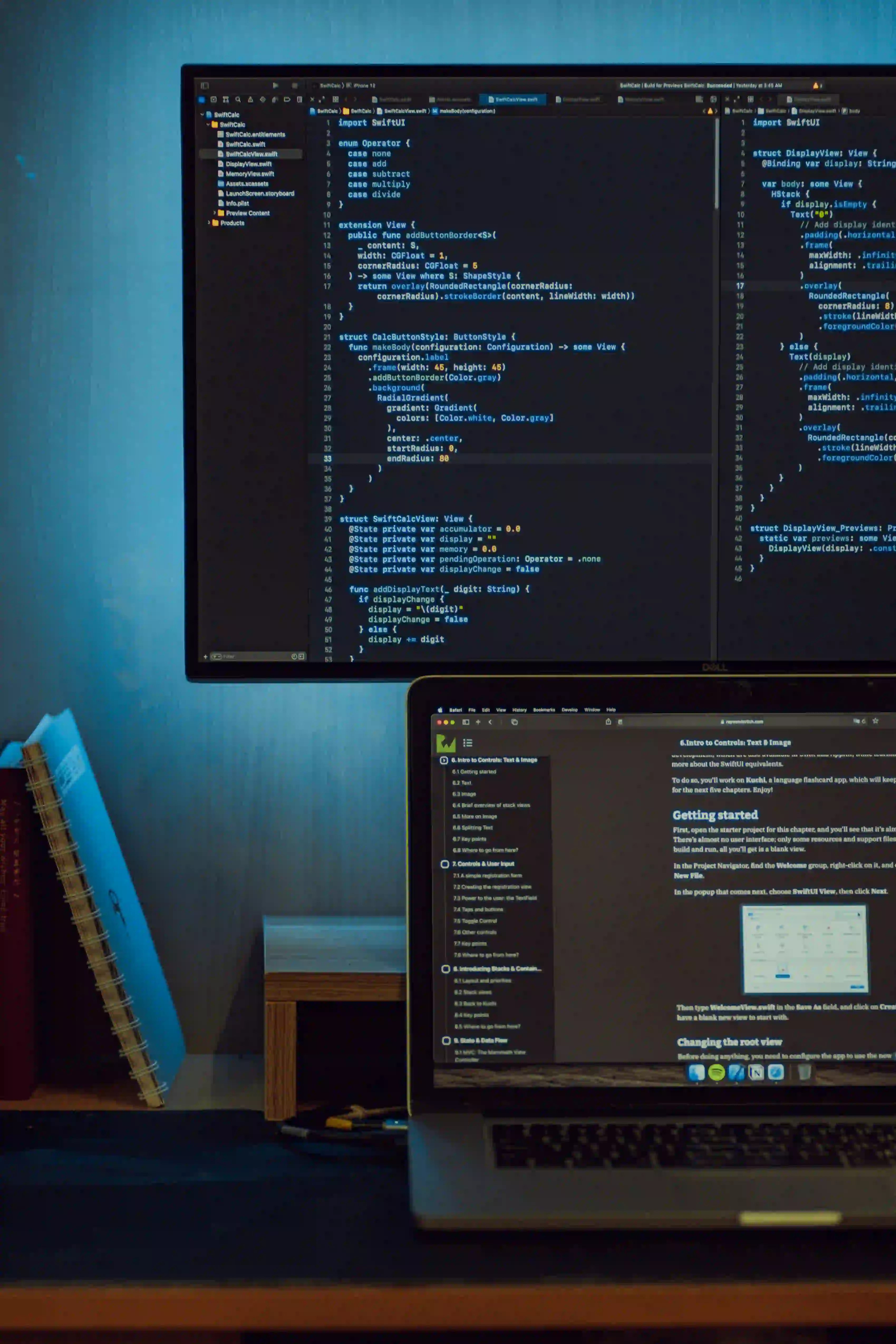
Mastering Spring MVC: Common Configuration Pitfalls
Spring MVC is a powerful framework for building web applications in Java. It offers a flexible architecture, great scalability, and a broad ecosystem of tools. However, misconfigurations can lead to frustrating bugs and hours of wasted time. In this blog post, we will explore common configuration pitfalls in Spring MVC and how to avoid them, ensuring a smoother development experience.
Table of Contents
- Understanding Spring MVC Configuration
- Common Configuration Pitfalls
- Incorrect DispatcherServlet Configuration
- Missing Component Scanning
- Overlooking View Resolvers
- Not Properly Configuring the Context
- Best Practices to Avoid Pitfalls
- Conclusion
Understanding Spring MVC Configuration
Before diving into the pitfalls, it is crucial to understand how Spring MVC operates. The architecture is based on the Model-View-Controller (MVC) design pattern, dividing application logic into three interconnected components:
- Model: Represents the data or business logic
- View: Represents the user interface
- Controller: Handles user inputs and interacts with the model.
The configuration is typically done in XML or through Java-based configurations, such as annotations.
Example of Basic Spring MVC Configuration
Here's a simple setup using Java-based configuration:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
@Configuration
@EnableWebMvc
public class WebConfig {
// Configuration methods here
}
The @EnableWebMvc
annotation is crucial as it enables Spring's MVC features, configuring default settings for handling requests.
Common Configuration Pitfalls
1. Incorrect DispatcherServlet Configuration
One of the most essential parts of a Spring MVC application is the DispatcherServlet
. It acts as a front controller that routes requests to the corresponding handlers (controllers). An incorrectly configured DispatcherServlet
can cause a cascade of problems.
Pitfall: Not specifying the correct mapping in the web.xml
.
Correct Configuration:
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
Why: The url-pattern
must match the incoming requests. If it is not set to /
, routing may not occur correctly, leading to 404 errors.
2. Missing Component Scanning
Component scanning is crucial for Spring to detect and register your controllers, services, and other components automatically. Forgetting to set up component scanning results in a Non-Existent Bean error.
Pitfall: Omitting component scanning in your configuration.
Correct Configuration:
@Configuration
@EnableWebMvc
@ComponentScan(basePackages = "com.example.application")
public class WebConfig {
// Other configurations
}
Why: The component scan (@ComponentScan
) tells Spring where to look for annotated components. If not included, Spring won't be aware of your beans, and you’ll end up with a fresh build but no controllers mapped.
3. Overlooking View Resolvers
View Resolvers are vital in Spring MVC as they determine how to resolve view names returned by controllers. A missing or misconfigured View Resolver can lead to fragments not being correctly displayed.
Pitfall: Not defining a correct View Resolver.
Correct Configuration:
@Bean
public InternalResourceViewResolver viewResolver() {
InternalResourceViewResolver resolver = new InternalResourceViewResolver();
resolver.setPrefix("/WEB-INF/views/");
resolver.setSuffix(".jsp");
return resolver;
}
Why: This configuration specifies to Spring where to find JSP files. If the prefix or suffix is incorrect, the application will throw view not found errors.
4. Not Properly Configuring the Context
In a Spring MVC application, the context is a critical component. It can be the source of numerous issues if not configured correctly.
Pitfall: Missing or incorrectly defining the ApplicationContext
.
Correct Configuration:
<context:component-scan base-package="com.example.application"/>
<context:annotation-config/>
Why: The component-scan
and annotation-config
are necessary to allow Spring to discover and instantiate your beans. If ignored, the application will lack the context needed for dependency injection.
Best Practices to Avoid Pitfalls
-
Read the Documentation: The Spring MVC Documentation is comprehensive and offers immense guidance on configuration nuances.
-
Use Annotations: Utilizing annotations like
@Controller
,@RequestMapping
, and@Autowired
simplifies the configuration process and reduces XML complexity. -
Consistent Naming Conventions: Use clear and consistent naming conventions across your controllers and services to avoid confusion.
-
Unit Testing: Test your controllers independently using JUnit or other testing frameworks to catch configuration issues early in the development phase.
-
Environmental Profiles: Leverage Spring Profiles to keep your configurations clean when working in different environments (development, testing, production).
The Closing Argument
Spring MVC is a highly efficient framework when configured correctly, but common pitfalls can obtain frustrating outcomes. By understanding these pitfalls and following best practices, you can enhance your development experience and create reliable applications.
If you're new to Spring MVC or looking to refine your existing skills, consider exploring Spring Guides, which offer practical examples and scenarios. Remember, mastering the configuration process is crucial for a successful Spring MVC application.
By keeping an eye out for these common issues and implementing the right practices, you can tap into the full potential of Spring MVC and focus on creating robust, efficient applications. Happy coding!