Mastering Java Logging: Common Pitfalls to Avoid
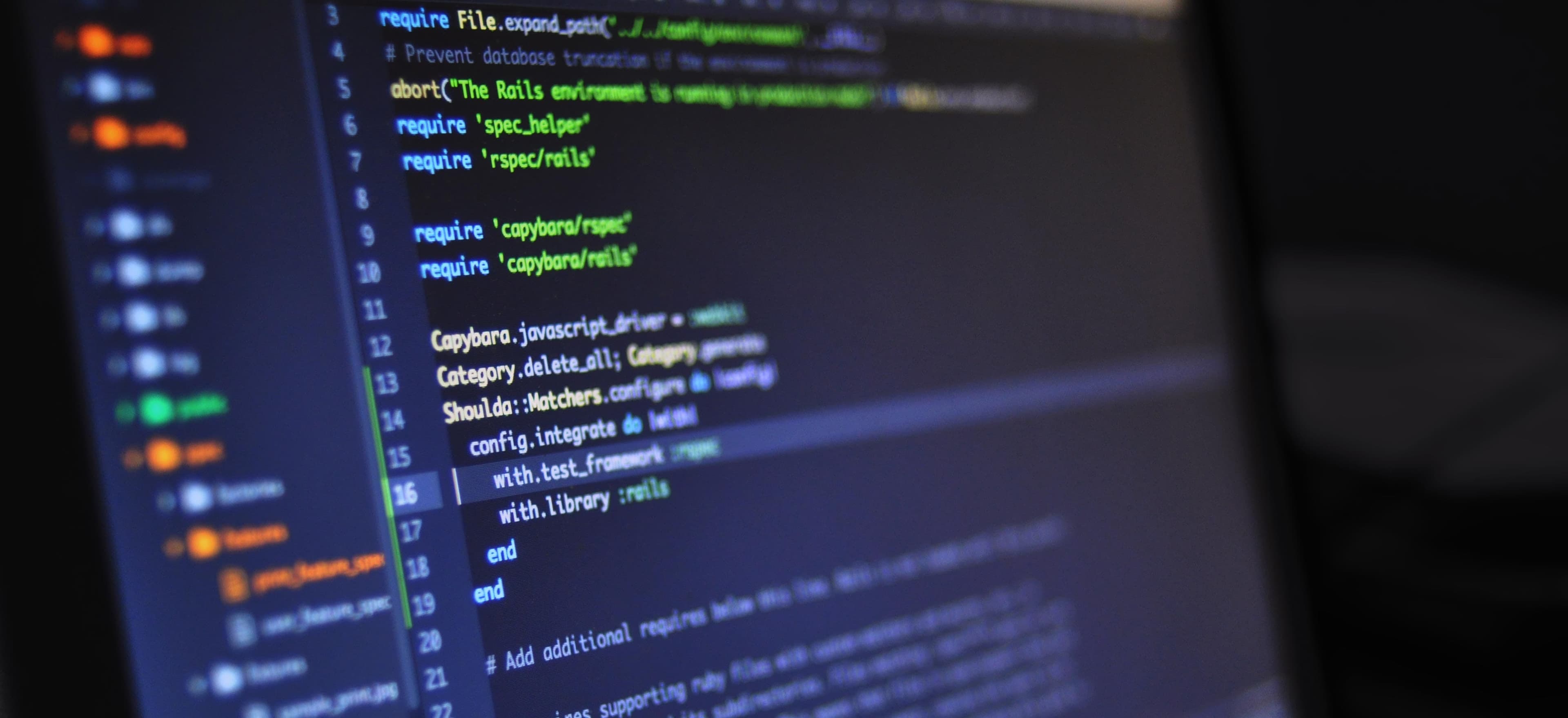
- Published on
Mastering Java Logging: Common Pitfalls to Avoid
Logging is an essential activity in software development, particularly in production environments. It enables developers to track the flow of an application, debug issues, and maintain performance metrics. However, mastering Java logging is not just about adding a logging framework; it requires a solid understanding of the potential pitfalls and best practices. In this post, we’ll explore common mistakes developers make with Java logging and how you can avoid them.
Why Is Logging Important?
Before we dive into pitfalls, let’s briefly discuss the importance of logging:
- Debugging: Logs provide valuable insights into the application's behavior and help pinpoint issues.
- Monitoring: Logs can serve as a real-time monitoring tool, helping teams understand application performance and health.
- Audit Trails: Logging creates an audit trail that can help with compliance and security audits.
Common Pitfalls in Java Logging
1. Over-logging
What It Is: Over-logging involves logging too much information, often leading to bloated log files that are hard to navigate.
Why to Avoid: Excessive logging can slow down your application and make it difficult to find relevant information when troubleshooting.
How to Avoid:
- Use different logging levels (DEBUG, INFO, WARN, ERROR) appropriately.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class Example {
private static final Logger logger = LoggerFactory.getLogger(Example.class);
public void process() {
logger.debug("Processing started"); // Useful for debugging
// Main logic here
logger.info("Process completed successfully"); // Informational
}
}
In the code above, DEBUG
is used for tracing a process, while INFO
conveys an important milestone in the execution.
2. Ignoring Contextual Information
What It Is: Failing to include contextual information in logs can lead to difficulties when diagnosing problems.
Why to Avoid: Without context, logs may lack clarity and can make the troubleshooting process tedious.
How to Avoid:
- Always include relevant identifiers such as user IDs, transaction IDs, or session information in your logs.
public void processTransaction(String transactionId) {
logger.info("Processing transaction with ID: {}", transactionId);
// Logic here
}
3. Not Configuring Log Levels
What It Is: Not configuring your logging framework properly can lead to either too much or too little information being logged.
Why to Avoid: Improper configuration can either flood your log files or omit crucial information, both of which are problematic.
How to Avoid:
- Utilize configuration files to control log levels easily across different environments (development, production).
Example configuration for logback.xml:
<configuration>
<appender name="CONSOLE" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} - %msg%n</pattern>
</encoder>
</appender>
<root level="INFO">
<appender-ref ref="CONSOLE"/>
</root>
</configuration>
4. Not Using Parameterized Logging
What It Is: Concatenating strings to create log messages can significantly impact performance.
Why to Avoid: String concatenation occurs regardless of the log level being used, which can lead to unnecessary performance overhead.
How to Avoid:
- Use parameterized logging to defer string interpolation until necessary.
logger.error("Failed to process transaction with ID: {}", transactionId);
In this case, the message is constructed only if the error level is enabled.
5. Not Handling Exceptions Properly
What It Is: Failing to log exceptions or logging them without detailed context.
Why to Avoid: Incomplete logging can complicate diagnosis and impede debugging efforts.
How to Avoid:
- Always log exceptions with informative messages and stack traces.
try {
// Some logic that may throw an exception
} catch (Exception e) {
logger.error("An error occurred while processing the transaction", e);
}
6. Hardcoding Log Levels
What It Is: Embedding log level definitions directly in the code rather than using an external configuration can lead to inflexible applications.
Why to Avoid: Changing log levels requires code changes and redeployment, which can be cumbersome and error-prone.
How to Avoid:
- Keep your log levels in external configuration files.
7. Ignoring Logging Best Practices
What It Is: Not adhering to industry-standard practices can lead to inconsistency and ambiguity in logging.
Why to Avoid: Inconsistent logs make it difficult for teams to extract information quickly.
How to Avoid:
- Use a unified logging framework (like SLF4J, Log4j, or Logback) and adhere to a consistent format for log entries.
8. Not Rotating Log Files
What It Is: Failing to implement log rotation can lead to unmanageable log file sizes.
Why to Avoid: Large log files can consume disk space quickly, leading to storage issues.
How to Avoid:
- Implement log rotation in your logging framework configuration.
Example configuration for logback.xml:
<appender name="FILE" class="ch.qos.logback.core.rolling.RollingFileAppender">
<file>logs/myApp.log</file>
<rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy">
<fileNamePattern>logs/myApp.%d{yyyy-MM-dd}.log</fileNamePattern>
<maxHistory>30</maxHistory> <!-- Keeps logs for 30 days -->
</rollingPolicy>
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} - %msg%n</pattern>
</encoder>
</appender>
9. Failing to Monitor Logs
What It Is: Setting up logging but not actively monitoring the logs can lead to missed critical issues.
Why to Avoid: Ignoring logs means you may be unaware of issues until they manifest as failures.
How to Avoid:
- Utilize monitoring tools that can parse logs and alert you to issues.
Key Takeaways
Mastering Java logging is crucial for the health of your applications. By avoiding common pitfalls such as over-logging, ignoring context, and failing to handle exceptions properly, you can enhance your application’s reliability and debuggability.
For further reading on logging frameworks, check out the SLF4J documentation or the Logback documentation.
Incorporating the best practices discussed will improve not only your logging strategy but also your application's maintainability and performance.
Happy logging!