Finding the Right Test Coverage for Continuous Deployment
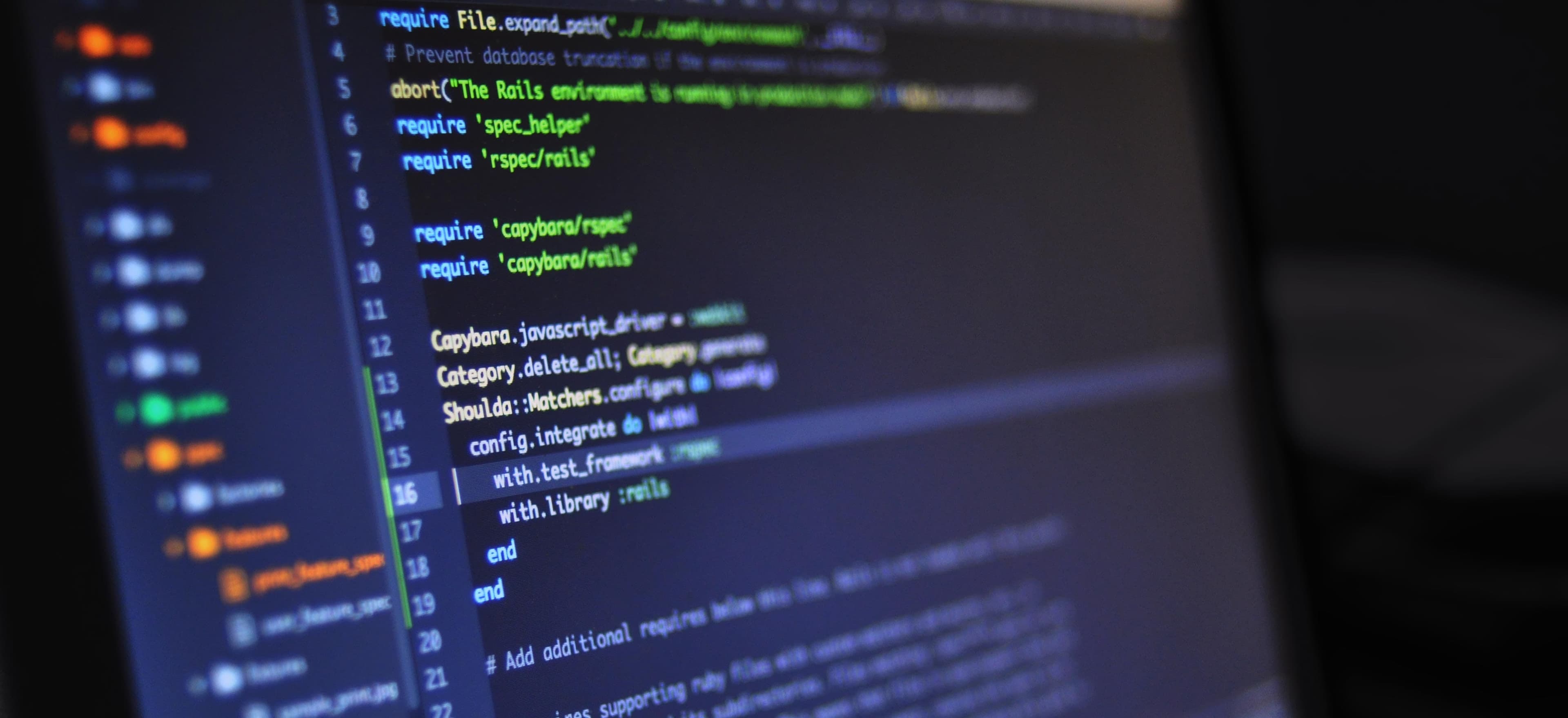
- Published on
Finding the Right Test Coverage for Continuous Deployment
In the fast-paced world of software development, continuous deployment has become a critical practice for many organizations. The ability to deliver small, incremental changes to production enables teams to respond quickly to users’ needs and fix issues as they arise. But how do we ensure that these deployments don't introduce new bugs into production? This is where test coverage comes in. In this blog post, we will explore the importance of test coverage and how to determine the right level for continuous deployment, particularly in Java applications.
Understanding Test Coverage
Test coverage quantifies the degree to which your codebase is tested. It measures the percentage of your code that is executed while tests are running. Proper test coverage is crucial; it directly affects the reliability and maintainability of your code. When adopting continuous deployment, it's essential to maintain a balance between the amount of testing and the speed of delivery.
Types of Test Coverage
-
Line Coverage: This simply measures how many lines of code are executed when running your tests. It can be beneficial but doesn’t tell you if your tests are robust.
-
Branch Coverage: This measures whether both the true and false branches of conditional statements are executed during testing. It provides a better picture than line coverage alone.
-
Function Coverage: This measures if all the functions in your code have been called during testing. While this ensures that functions are executed, it does not guarantee that they perform correctly.
-
Statement Coverage: This ensures every statement in the code is executed at least once. Like line coverage, it can miss potential problems.
For a comprehensive approach, consider a mix of these types of coverage to ensure your application has a solid testing foundation.
Why is Test Coverage Critical for Continuous Deployment?
Continuous deployment aims to provide a smooth, automated transition of code from development to production. High test coverage is crucial for several reasons:
-
Confidence: Effective testing allows developers to implement changes without fear of breaking existing features.
-
Quick Feedback: Tests provide immediate feedback, allowing teams to catch issues early and avoid costly last-minute fixes.
-
Collaboration: With high test coverage, developers can refactor and improve code without concerns about unintended side effects.
Determining the Right Level of Test Coverage
Finding the right balance in test coverage is not straightforward and can depend on various factors including team size, code complexity, and application criticality. Here are some guidelines to consider:
1. Aim for 80% Coverage, but Don't Obsess
A common rule of thumb is to aim for 80% coverage. This figure reflects a balance between ensuring sufficient testing and allowing for efficient development. However, it's essential to recognize that striving for 100% coverage might lead to diminishing returns. The effort spent achieving that last 20% might come at the cost of other critical development practices.
2. Consider Risk Areas
Focus on areas of your code that are critical and prone to bugs. For example, authentication modules, financial calculations, or any business-critical logic should receive more thorough testing.
public boolean authenticateUser(String username, String password) {
if (username == null || password == null) {
throw new IllegalArgumentException("Username and password cannot be null");
}
// Assume we have a method checkCredentials that verifies the user details
return checkCredentials(username, password);
}
Here, you should ensure that this method passes tests for various input scenarios, including valid credentials, invalid credentials, and null values.
3. Use Test-Driven Development (TDD)
Adopting a TDD approach can significantly help in ensuring adequate test coverage. In TDD, tests are written before the actual code, leading to a more testable design.
Example:
@Test
public void testUserAuthentication() {
UserService userService = new UserService();
assertTrue(userService.authenticateUser("validUser", "validPassword"));
assertFalse(userService.authenticateUser("invalidUser", "invalidPassword"));
}
This approach ensures that tests are written constantly, ensuring a high level of code coverage by default.
4. Static Code Analysis Tools
Utilizing tools like SonarQube or JaCoCo can help measure and monitor your test coverage. These tools provide comprehensive insights, enabling teams to visualize code coverage trends over time, and identify critical areas lacking test coverage.
5. Continuous Monitoring and Feedback
With tools integrated into your CI/CD pipeline, you can continuously monitor test coverage. This automatic reporting helps keep the test coverage within desired limits.
Prioritizing Types of Tests
While ensuring code coverage is vital, the focus should not solely be on quantity. Different types of tests (unit, integration, and end-to-end) serve distinct purposes and are important for different contexts.
-
Unit Tests: Test the smallest parts of application logic. They are fast and should cover a significant portion of code.
@Test public void testAddition() { Calculator calc = new Calculator(); assertEquals(5, calc.add(2, 3)); }
-
Integration Tests: Ensure that various components or services work together. They are crucial as your application becomes more modular and integrates with external services.
-
End-to-End Tests: Validate the application flow, focusing on user journeys. Though more complex and time-consuming, they are essential for ensuring the application meets user needs.
Lessons Learned
In an era of continuous deployment, the right level of test coverage is vital not just for functionality, but also for business success. Striving for roughly 80% coverage while emphasizing high-risk areas, adopting TDD, and leveraging modern tools can greatly facilitate this process. Remember, it’s about finding the right balance between the speed of delivery and the quality of your software.
For more in-depth guidance on improving your Java tests, check out JUnit Tutorial and Java Testing Best Practices.
By investing efforts into effective testing and continuous improvement, you'll not only enhance your deployment process but also improve the quality and reliability of your software for users.