Understanding the Risks of Shallow Copy in ArrayLists
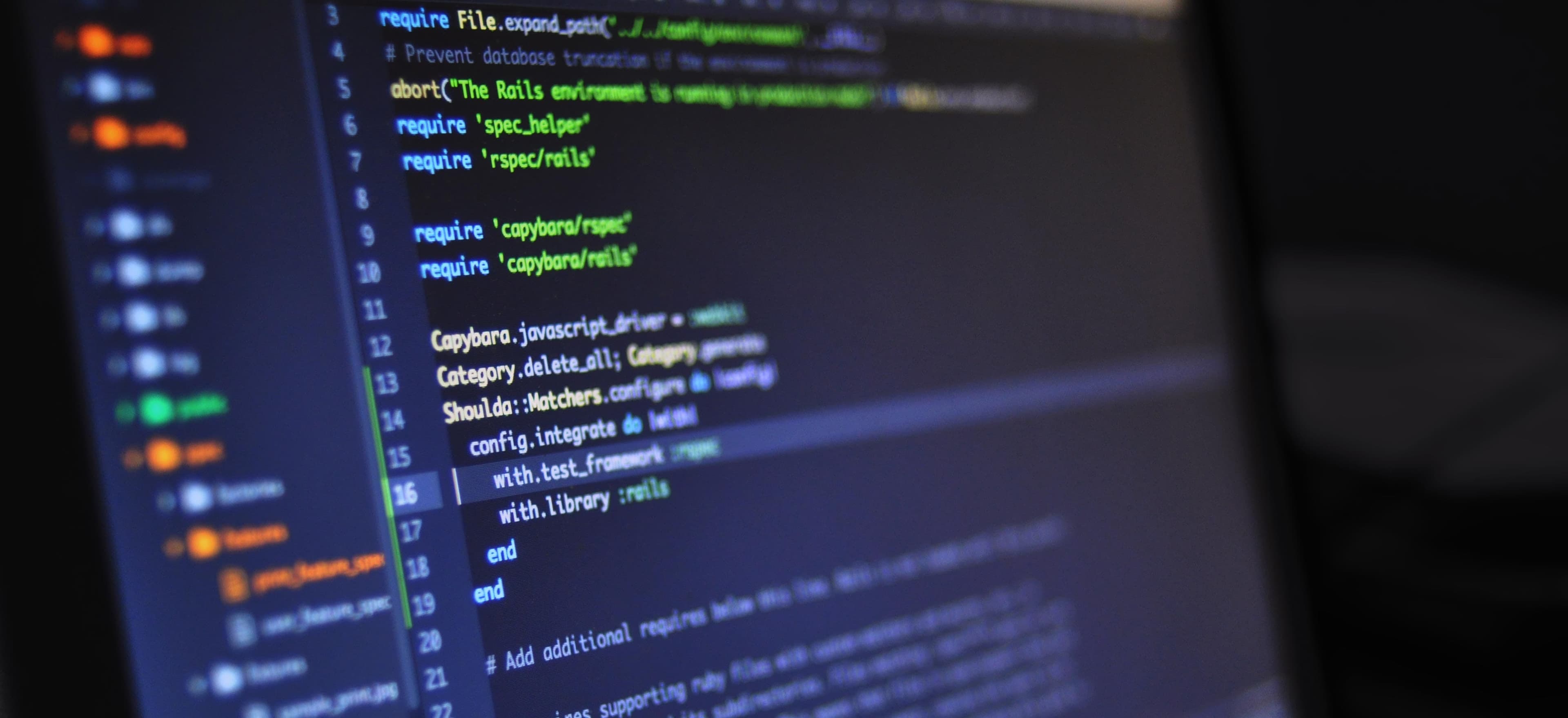
- Published on
Understanding the Risks of Shallow Copy in ArrayLists
Java developers commonly utilize the ArrayList
class as part of the Collections Framework due to its dynamic nature and flexibility. However, when making copies of ArrayList
instances, it's vital to understand the differences between shallow and deep copies, especially when dealing with objects containing mutable state. This blog post will examine the risks associated with shallow copies, provide example code, and advise best practices for cloning ArrayLists
.
What is a Shallow Copy?
A shallow copy of an ArrayList
creates a new list with references to the original list's elements. In simpler terms, while the new list itself is a separate object, the items in the list still point to the same objects as in the original list.
Here’s a concise demonstration of constructing a shallow copy:
import java.util.ArrayList;
class Person {
String name;
Person(String name) {
this.name = name;
}
void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Person{name='" + name + "'}";
}
}
public class ShallowCopyExample {
public static void main(String[] args) {
ArrayList<Person> originalList = new ArrayList<>();
originalList.add(new Person("Alice"));
originalList.add(new Person("Bob"));
// Creating a shallow copy
ArrayList<Person> shallowCopy = (ArrayList<Person>) originalList.clone();
// Modifying the name of the person in the shallow copy
shallowCopy.get(0).setName("Charlie");
// Displaying both lists
System.out.println("Original List: " + originalList);
System.out.println("Shallow Copy: " + shallowCopy);
}
}
Explanation of the Code
In the above code:
- We create an
ArrayList
ofPerson
objects. - We then create a shallow copy using the
.clone()
method. - After modifying the name of a
Person
in the shallow copy, both the original list and the shallow copy reflect this change.
Output
Original List: [Person{name='Charlie'}, Person{name='Bob'}]
Shallow Copy: [Person{name='Charlie'}, Person{name='Bob'}]
This example highlights a significant risk of shallow copying. Changes made to mutable objects in the copied list affect the original list.
The Risks of Shallow Copy in ArrayLists
-
Unexpected Side Effects: As shown in the example, modifying objects in the shallow copy can lead to unintended changes in the original list. This behavior can cause confusion and bugs, particularly when developers expect a completely independent copy.
-
Performance Issues: While shallow copies are generally faster than deep copies, in scenarios where you frequently make changes or require isolation, the overhead of managing unintended side effects can degrade performance. It’s essential to weigh the performance benefits against the risks of state incoherence.
-
Inconsistent State: In applications that rely on shared states, shallow copying can lead to inconsistent states in different parts of the application. This situation complicates debugging and may result in challenging maintenance scenarios.
Contrasting Deep Copy
To mitigate the risks of shallow copying, utilizing a deep copy can be an excellent solution. A deep copy creates a new list and also clones the objects contained within it. Here is an example of how we can achieve that:
import java.util.ArrayList;
import java.util.List;
public class DeepCopyExample {
public static void main(String[] args) {
ArrayList<Person> originalList = new ArrayList<>();
originalList.add(new Person("Alice"));
originalList.add(new Person("Bob"));
// Creating a deep copy
ArrayList<Person> deepCopy = new ArrayList<>();
for (Person person : originalList) {
deepCopy.add(new Person(person.name));
}
// Modifying the name of the person in the deep copy
deepCopy.get(0).setName("Charlie");
// Displaying both lists
System.out.println("Original List: " + originalList);
System.out.println("Deep Copy: " + deepCopy);
}
}
Explanation of the Code
In this illustration:
- We manually create a new
Person
instance for each object in the original list when constructing the deep copy. - Modifying an object in the deep copy does not affect the original list, thereby preserving its state.
Output
Original List: [Person{name='Alice'}, Person{name='Bob'}]
Deep Copy: [Person{name='Charlie'}, Person{name='Bob'}]
Best Practices for Cloning ArrayLists
-
Determine the Need: Before cloning an
ArrayList
, consider whether you need a shallow or deep copy. Assess the nature of the objects and their mutability. -
Implement Custom Cloning: When working with complex objects, consider implementing a custom cloning mechanism, such as the Cloneable interface or providing a copy constructor, to ensure deep copies are made.
-
Utilize Libraries: Consider utilizing libraries like Apache Commons Lang, which provides utilities for cloning objects safely in various ways. For instance:
import org.apache.commons.lang3.SerializationUtils; ArrayList<Person> clonedList = SerializationUtils.clone(originalList);
-
Unit Testing: Test both shallow and deep copies to ensure they meet your application’s requirements. Use unit tests to validate behaviors when using copies to catch potential issues early.
-
Documentation: Clearly document methods that perform cloning to indicate whether a shallow or deep copy is being made. This can help other developers (or your future self) understand any potential risks.
Closing the Chapter
Understanding the risks and behaviors associated with shallow copies in ArrayList
is fundamental for effective Java programming. While the convenience of shallow copies can be tempting, the potential pitfalls often outweigh the benefits, especially when mutable objects are involved.
By evaluating the need for shallow versus deep copies and adhering to best practices, developers can streamline their code while minimizing unexpected behaviors. For further reading on this subject, consider exploring Java Collections Tutorial.
By taking a methodical approach towards managing copies of ArrayList
, we can build more resilient applications and maintain clean code practices.
Checkout our other articles