Mastering EAR Module Creation in Java EE7: A Newbie's Guide
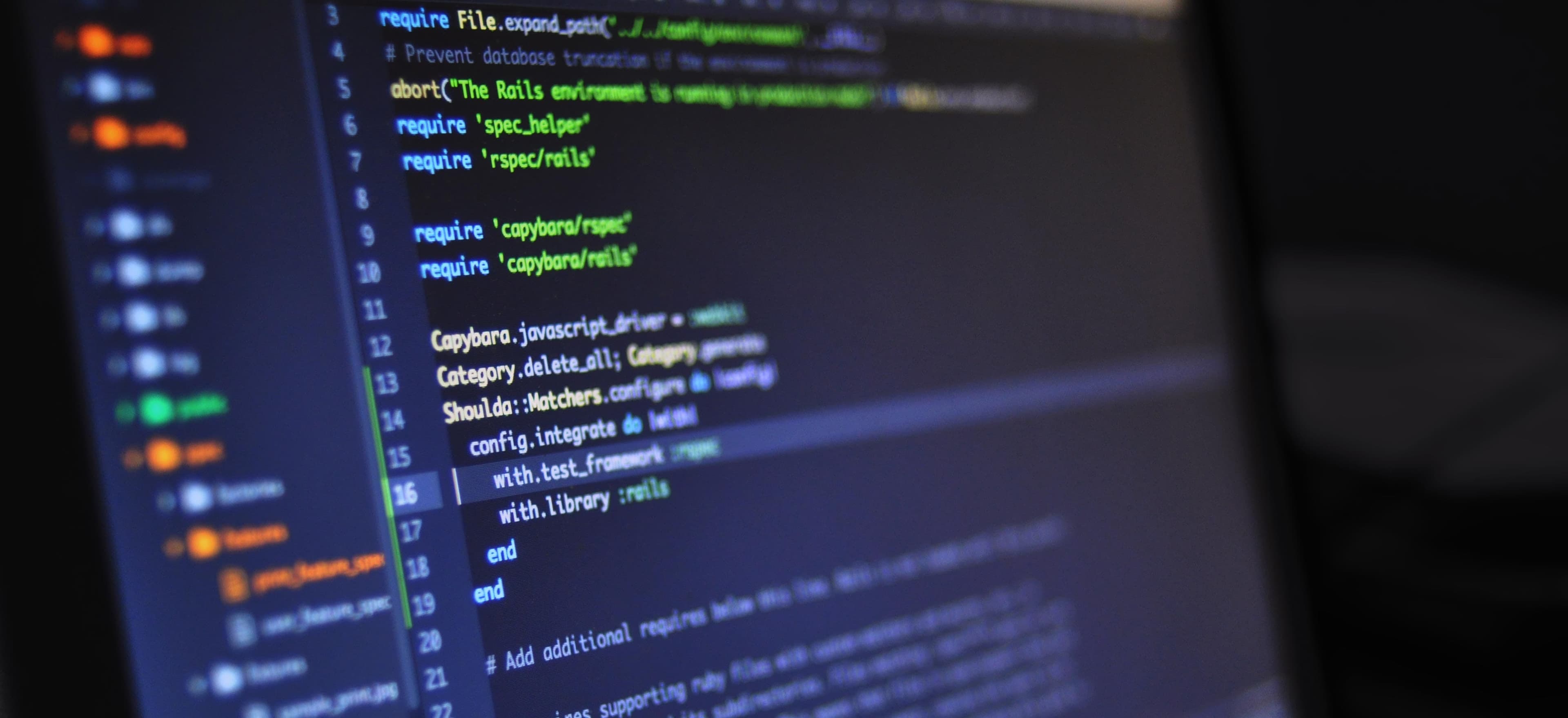
- Published on
Mastering EAR Module Creation in Java EE 7: A Newbie's Guide
Java EE (Enterprise Edition), now known as Jakarta EE, is widely used for building scalable and secure enterprise-level applications. One of the core building blocks of Java EE is the EAR (Enterprise Archive) module. This format is a powerful tool that packages together various Java EE modules like EJB (Enterprise Java Beans) and WAR (Web Application Archive) files into a single deployable unit.
In this blog, we will walk through the process of creating an EAR module with a practical approach, which will help you understand the components involved, the structure, and how to deploy your application effectively.
What is an EAR Module?
Before we delve into creation, let's clarify what an EAR module is. An EAR file is essentially a compressed file structure that aggregates multiple JAR files, and WAR files for deployment to an application server like WildFly or GlassFish. It serves a specific purpose within enterprise applications:
- Modularization: It allows separation of concerns by dividing application components into different modules.
- Simplified Deployment: An EAR file can be deployed as a single unit, simplifying the deployment process for multi-module applications.
- Scoped Resource Management: An EAR can manage resources like connection factories and data sources that can be shared across the modules.
Prerequisites
To get started, you need:
- Java JDK: Make sure you have the latest version installed.
- Java EE 7 SDK: Download and install the Java EE SDK to get access to libraries and tools.
- Integrated Development Environment (IDE): A robust IDE like Eclipse or IntelliJ IDEA is recommended.
- An Application Server: Such as WildFly or GlassFish.
Steps to Create an EAR Module
Step 1: Create a Java Project
Start with creating a new Java project in your IDE.
mkdir MyEarProject
cd MyEarProject
This creates a basic directory structure for our project.
Step 2: Create EJB and Web Modules
You need to create two types of modules: EJB and Web. The EJB module will handle business logic, while the Web module will manage the user interface.
Create the EJB Module
Create a new EJB module named MyEjbModule
.
-
Create the project folder:
mkdir MyEjbModule cd MyEjbModule
-
Create a simple EJB:
package com.example.ejb; import javax.ejb.Stateless; @Stateless public class Calculator { public int add(int a, int b) { return a + b; } }
Why this code?
The
@Stateless
annotation signifies that this bean performs business logic without maintaining a conversation state with the client. It is reusable and can serve multiple clients simultaneously.
Create the Web Module
Now, create another project folder for the Web module named MyWebModule
.
-
Create the project folder:
mkdir MyWebModule cd MyWebModule
-
Create a simple servlet:
package com.example.web; import javax.inject.Inject; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet("/calculate") public class CalculatorServlet extends HttpServlet { @Inject private Calculator calculator; @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { int a = Integer.parseInt(req.getParameter("a")); int b = Integer.parseInt(req.getParameter("b")); int result = calculator.add(a, b); resp.getWriter().write("Result: " + result); } }
Why this code?
The servlet interacts with the EJB using dependency injection, which promotes loose coupling and enhances maintainability. The servlet responds to HTTP requests and forwards them to the EJB for processing.
Step 3: Create the EAR Project
Now, let's create the EAR module that will package these components.
-
Create the EAR project folder:
mkdir MyEarModule cd MyEarModule
-
Create the
application.xml
deployment descriptor:Create a file named
application.xml
undersrc/main/application/META-INF
:<application xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/application_7.xsd" version="7"> <module> <ejb>MyEjbModule.jar</ejb> </module> <module> <web> <web-uri>MyWebModule.war</web-uri> <context-root>/calculator</context-root> </web> </module> </application>
Why this code?
The
application.xml
file is essential as it outlines the modules included within the EAR and their configurations. In this case, we declare our EJB and Web modules with their respective URIs and context roots.
Step 4: Packaging the EAR
Next, you need to package the EAR file using Maven or Gradle. We'll use Maven for simplicity.
-
Add dependencies to
pom.xml
for each module. -
The EAR module
pom.xml
should look like this:<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>MyEarModule</artifactId> <version>1.0-SNAPSHOT</version> <packaging>ear</packaging> <modules> <module>../MyEjbModule</module> <module>../MyWebModule</module> </modules> </project>
Why use packaging?
Specifying
<packaging>ear</packaging>
tells Maven to treat this project as an EAR project and appropriately handle dependencies and builds. -
Run the Maven package command:
mvn clean install
This will compile all source files and produce the EAR file in the
target
directory.
Step 5: Deploy the EAR File
- Locate your
.ear
file inside theMyEarModule/target
directory. - Deploy it to your chosen application server (like WildFly or GlassFish) using the management console or CLI.
Step 6: Test the Application
Once deployed, you can test the application by accessing the web interface. Open your browser and navigate to:
http://localhost:8080/calculator/calculate?a=3&b=4
You should see:
Result: 7
Key Takeaways
Creating an EAR module in Java EE 7 requires thoughtful design but pays off with a robust and manageable structure. You’ve learned how to encapsulate your EJB and web modules into a singular deployable unit, paving the way for more complex applications down the line.
For further reading, consider exploring the Jakarta EE documentation and the Maven for Java EE Project for comprehensive insights.
Start building your Java EE applications with this newfound knowledge, and make enterprise-level development a reality!
Happy coding!
Checkout our other articles