Mastering Java: 10 Hidden Coding Practices You Must Know
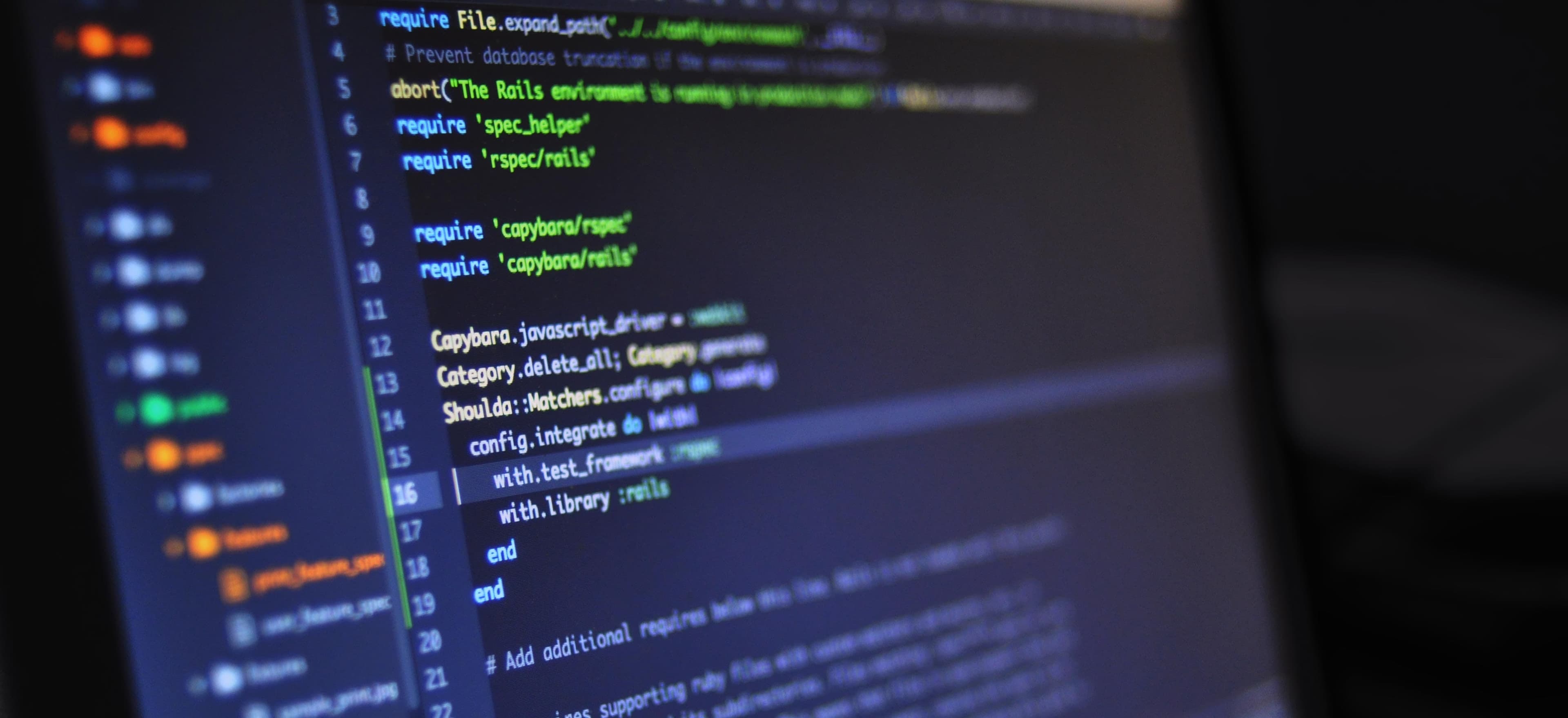
- Published on
Mastering Java: 10 Hidden Coding Practices You Must Know
Java, one of the most robust and versatile programming languages, continues to be a favorite among developers worldwide. Despite its age, it consistently evolves, introducing new features that enhance coding practices. In this blog post, we will explore ten hidden coding practices in Java that can significantly improve your programming efficiency and code quality.
1. Utilize the Enhanced For Loop
The enhanced for loop (also known as the "for-each" loop) simplifies iteration over collections.
Code Example
List<String> colors = Arrays.asList("Red", "Green", "Blue");
for (String color : colors) {
System.out.println(color);
}
Why Use It?
The enhanced for loop not only reduces boilerplate code but also improves readability. It abstracts the complexities of traditional index-based loops and minimizes the chances of errors, such as off-by-one mistakes.
2. Leverage the Try-With-Resources Statement
Java 7 introduced the try-with-resources statement that automatically closes resources.
Code Example
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
Why Use It?
This practice ensures that all resources are closed properly, helping to prevent memory leaks and resource exhaustion. A well-structured resource management pattern can prevent hard-to-trace bugs in larger applications.
3. Use Optional to Handle Nulls
Java 8 introduced Optional
, a container that may or may not hold a non-null value.
Code Example
Optional<String> optionalName = Optional.ofNullable(getName());
optionalName.ifPresent(name -> System.out.println("Hello, " + name));
Why Use It?
Using Optional
reduces the risk of NullPointerException
, making your code cleaner and easier to understand. It emphasizes the importance of checking for nulls, improving code safety.
4. Choose the Right Data Structure
Selecting the appropriate data structure is essential for optimizing performance.
Code Example
Set<String> set = new HashSet<>(Arrays.asList("Apple", "Orange", "Banana"));
// Fast lookup for existence
boolean exists = set.contains("Apple");
Why Use It?
Different data structures offer various time complexities for operations. For instance, using a HashSet
provides O(1) average time complexity for lookups, while a List
might necessitate O(n) in the worst-case scenario. Understanding these nuances can lead to significant performance improvements.
5. Stream API for Functional Programming
The Stream API introduced in Java 8 allows for functional-style operations on collections.
Code Example
List<String> names = Arrays.asList("John", " Jane", "Jack");
names.stream()
.filter(name -> name.startsWith("J"))
.forEach(System.out::println);
Why Use It?
The Stream API encourages a more declarative coding style, enabling shorter and more readable code. It also takes advantage of multi-core architectures for parallel processing, thereby improving performance.
6. Use Immutable Collections
Java offers immutable collection classes, such as List.of()
, Set.of()
, and Map.of()
for thread-safe and unmodifiable collections.
Code Example
List<String> immutableList = List.of("A", "B", "C");
// immutableList.add("D"); // This line would throw UnsupportedOperationException
Why Use It?
Immutable collections guarantee that the data cannot be altered after its creation, making them ideal in multi-threaded applications. This reduces the likelihood of bugs and enhances maintainability.
7. Apply Builder Pattern for Complex Objects
The Builder Pattern is useful for constructing complex objects while avoiding constructors with a long parameter list.
Code Example
public class User {
private final String name;
private final int age;
private User(UserBuilder builder) {
this.name = builder.name;
this.age = builder.age;
}
public static class UserBuilder {
private String name;
private int age;
public UserBuilder setName(String name) {
this.name = name;
return this;
}
public UserBuilder setAge(int age) {
this.age = age;
return this;
}
public User build() {
return new User(this);
}
}
}
// Usage
User user = new User.UserBuilder().setName("John").setAge(30).build();
Why Use It?
The Builder Pattern increases code clarity and promotes immutability. It allows you to create objects in a readable manner, making it easier to handle default values or optional attributes during construction.
8. Use Default Methods in Interfaces
Java 8 allows interfaces to contain default methods, which can provide method implementations.
Code Example
public interface Vehicle {
default void start() {
System.out.println("Vehicle is starting");
}
}
// Implementation
public class Car implements Vehicle {
// Overrides not necessary
}
Why Use It?
Default methods enable adding new functionality to existing interfaces without breaking implementations. They help in achieving backward compatibility while enhancing the interface's capabilities.
9. Annotations for Meta-programming
Java allows the use of annotations, which can be leveraged for various purposes such as documentation (e.g., @Override
) or to configure behaviors.
Code Example
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface Test {
int value() default 0;
}
// Usage
@Test(1)
public void myTestMethod() {
// test logic
}
Why Use It?
Annotations make your code more expressive and provide a way to associate metadata with class members, which can be processed by frameworks and tools, influencing execution during runtime.
10. Use Java's var
for Local Variable Type Inference
Introduced in Java 10, the var
keyword allows for local variable type inference, reducing verbosity.
Code Example
var list = new ArrayList<String>();
list.add("Item 1");
Why Use It?
While explicit typing enhances clarity, var
can make your code more concise without sacrificing readability. However, it should be used judiciously to maintain clarity — especially in complex projects.
The Bottom Line
Mastering Java includes understanding and implementing best practices that enhance code quality and maintainability. By leveraging the hidden coding practices outlined above, you can elevate your Java programming skills and minimize pitfalls in your projects.
For further reading on Java best practices, consider exploring these resources:
Get Started Today
Take these practices into your daily coding routine and observe how they streamline your process, reduce errors, and lead to more robust applications. Happy coding!
Checkout our other articles