Keycloak vs OAuth 2.0: Which One Secures Your APIs Best?
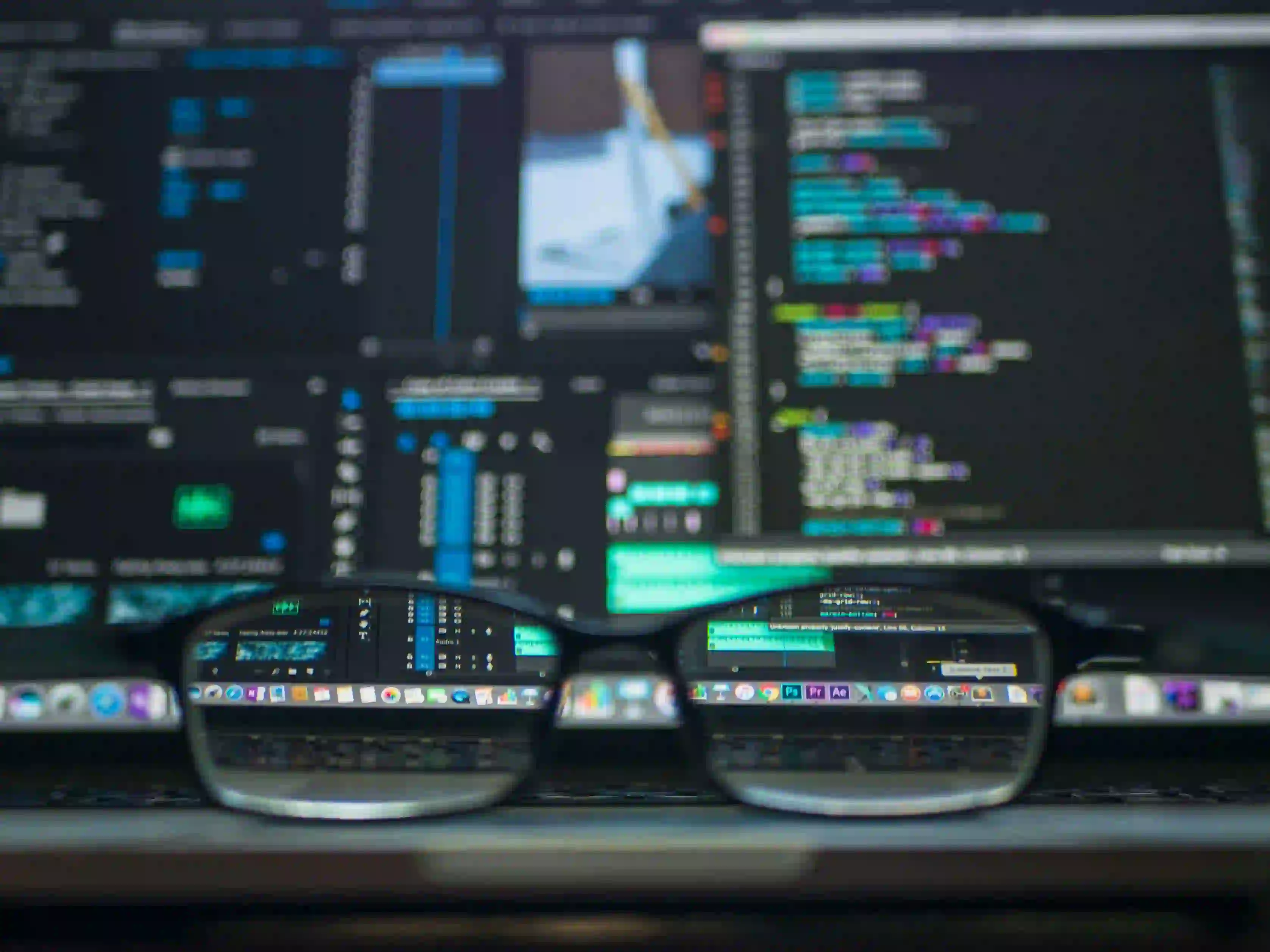
Keycloak vs OAuth 2.0: Which One Secures Your APIs Best?
In the modern world of web applications and APIs, security is a paramount concern. Two well-known solutions for managing authentication and authorization are Keycloak and OAuth 2.0. However, it can be confusing to understand the distinction between the two, as they often overlap in functionality. In this article, we will explore each technology, how they work, and detail their strengths and limitations when it comes to securing your APIs.
Understanding OAuth 2.0
OAuth 2.0 is an open standard for access delegation. This protocol allows third-party services to exchange web resources on behalf of a user. In simpler terms, OAuth 2.0 grants tokens to users that give limited access to their account, thus enabling secure API requests without sharing passwords.
Here’s a basic flow of how OAuth 2.0 works:
- Authorization Request: The client requests authorization from the user.
- Authorization Grant: The user grants permission.
- Access Token Request: The client exchanges the grant for an access token.
- Access Token Response: The server responds with an access token.
- Resource Access: The client uses the access token to request protected resources.
Use Cases for OAuth 2.0
- APIs: OAuth 2.0 is primarily used for API access management.
- Single Sign-On (SSO): It can be integrated with login mechanisms, allowing users to log in once to access multiple applications without needing to re-enter their credentials.
Implementation Example
Let’s take a look at how you might implement a simple OAuth 2.0 authorization flow in your API using Node.js.
const express = require('express');
const axios = require('axios');
const app = express();
// Endpoint to start the OAuth flow
app.get('/login', (req, res) => {
const authorizationUrl = 'https://authorization-server.com/auth';
res.redirect(authorizationUrl); // Redirecting user to authorize
});
// Callback endpoint that handles redirects from the authorization server
app.get('/callback', async (req, res) => {
const { code } = req.query;
// Exchange authorization code for access token
const response = await axios.post('https://authorization-server.com/token', {
code,
client_id: 'YOUR_CLIENT_ID',
client_secret: 'YOUR_CLIENT_SECRET',
grant_type: 'authorization_code',
});
const accessToken = response.data.access_token;
res.json({ accessToken }); // Send back the access token
});
app.listen(3000, () => console.log('Server running on port 3000.'));
Commentary
In this example, we have implemented a simple authorization flow with an express server. Users are redirected to the authorization server, where they log in and approve access. The server then exchanges the authorization code for an access token which can be used to make API requests. This demonstrates the simplicity of handling user authentication and API security with OAuth 2.0.
Understanding Keycloak
Keycloak is an open-source identity and access management tool. Unlike OAuth 2.0, which is a protocol, Keycloak is an implementation that provides built-in support for user authentication and authorization. Furthermore, Keycloak supports OAuth 2.0, OpenID Connect, SAML, and other authentication methods.
Features of Keycloak
- User Federation: Keycloak can integrate with existing user databases.
- Single Sign-On: It provides SSO across multiple applications.
- Social Login: Users can log in using social media accounts.
- Admin Console: A user-friendly interface allows easy management of users and roles.
Implementation Example
Here’s how you’d configure Keycloak to secure an API in a Java application.
Maven Dependency
To include Keycloak in your Java application, add the following dependency in your pom.xml
:
<dependency>
<groupId>org.keycloak</groupId>
<artifactId>keycloak-spring-boot-starter</artifactId>
<version>xx.xx.xx</version>
</dependency>
Configuration
import org.keycloak.adapters.springsecurity.KeycloakConfiguration;
import org.keycloak.adapters.springsecurity.config.KeycloakSecurityComponents;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.web.authentication.logout.LogoutSuccessHandler;
@Configuration
@EnableWebSecurity
@KeycloakConfiguration
@ComponentScan(basePackageClasses = KeycloakSecurityComponents.class)
public class SecurityConfig extends KeycloakWebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
super.configure(http);
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.logout()
.logoutSuccessHandler(new LogoutSuccessHandler() {
@Override
public void onLogoutSuccess(HttpServletRequest request, HttpServletResponse response,
Authentication authentication) throws IOException, ServletException {
// Custom logout handling can go here
}
});
}
}
Commentary
Using Keycloak in a Spring application is straightforward. By extending KeycloakWebSecurityConfigurerAdapter
, you can easily define which endpoints require authentication. The integration with Spring Security allows seamless protection of your API resources.
Key Differences: Keycloak vs OAuth 2.0
-
Nature:
- OAuth 2.0 is a protocol for authorization.
- Keycloak is a full-fledged authentication and authorization server.
-
Implementation Complexity:
- OAuth can be complex to implement on your own.
- Keycloak simplifies user management and comes with a user interface.
-
Protocols Supported:
- OAuth 2.0 focuses strictly on authorization.
- Keycloak supports multiple protocols, including OAuth 2.0, SAML, and OpenID Connect.
-
Deployment:
- OAuth 2.0 can be implemented in various environments with varying complexities.
- Keycloak requires their server but can be easily deployed in various formats like Docker or Kubernetes.
Summary: Which is Right for You?
If your primary need is to delegate access to your APIs and you are comfortable with handling the complexities of different OAuth flows, then implementing OAuth 2.0 yourself may be the right route. However, if you seek a more comprehensive solution that includes user management, SSO, and support for various authentication methods, then Keycloak is a better fit.
Final Thoughts
Both Keycloak and OAuth 2.0 have their roles in securing API requests and managing access. Your choice comes down to your specific application requirements, team skill levels, and the complexity of integration you expect to face. By understanding the strengths and weaknesses of both solutions, you can make an informed decision that best meets your security needs.
For more detailed tutorials, you can explore Keycloak Documentation and the OAuth 2.0 Framework.