Improving Builder Pattern Flexibility with Additional Parameters
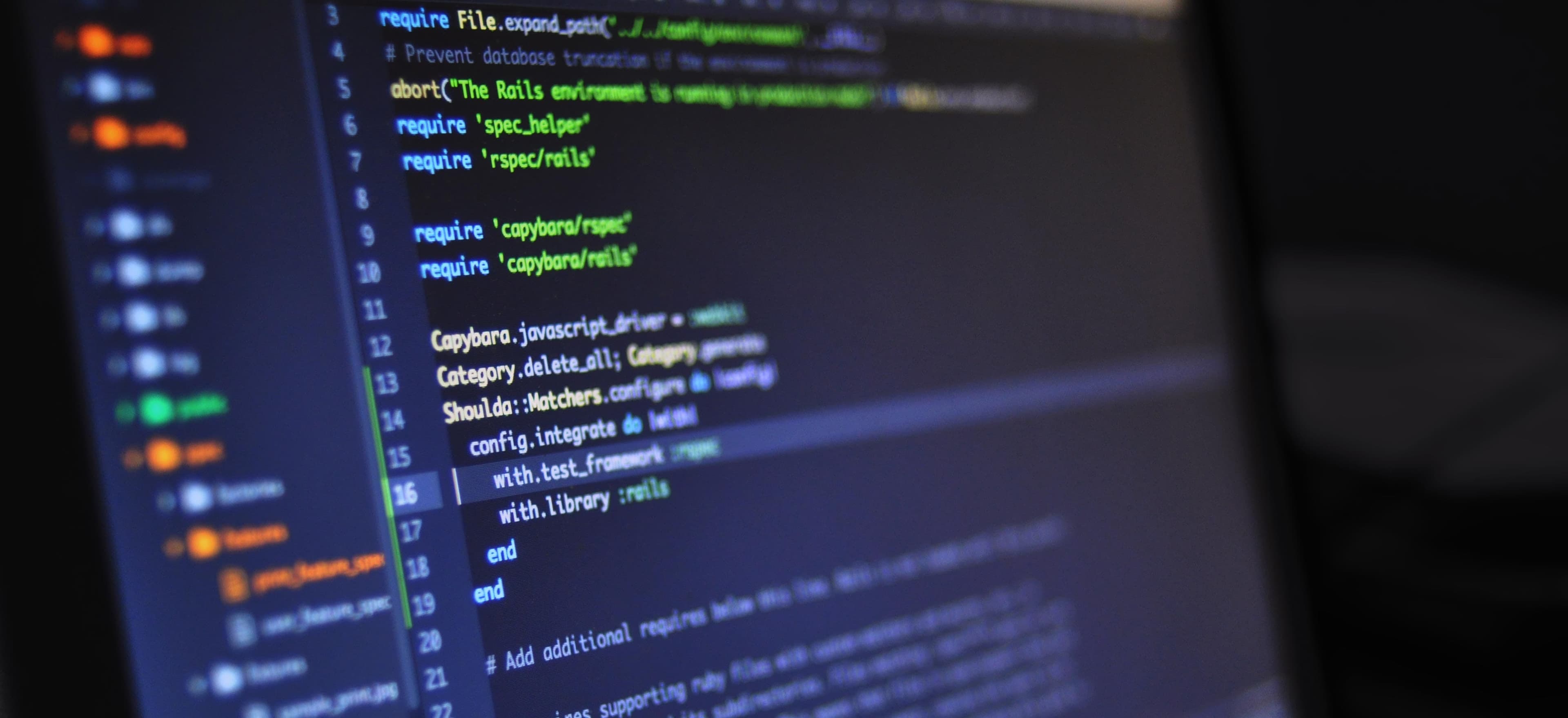
- Published on
Improving Builder Pattern Flexibility with Additional Parameters
The Builder Pattern is a fundamental design pattern in software engineering often used to simplify the process of constructing complex objects. In Java, this pattern is particularly valuable when working with mutable objects and enhances code readability and maintainability.
In this blog post, we will explore how to improve the flexibility of the Builder Pattern by incorporating additional parameters. This will enable us to create more configurable objects with less repetitive code. Throughout the post, we will work with a practical example to clarify the concepts discussed.
Understanding the Builder Pattern
Before diving into multiple parameters, let’s review the essence of the Builder Pattern. The pattern separates the construction of a complex object from its representation. This allows the same construction process to create various representations.
Here is a basic structure of the Builder Pattern in Java:
public class User {
private final String name;
private final String email;
private final int age;
private User(UserBuilder builder) {
this.name = builder.name;
this.email = builder.email;
this.age = builder.age;
}
public static class UserBuilder {
private String name;
private String email;
private int age;
public UserBuilder setName(String name) {
this.name = name;
return this;
}
public UserBuilder setEmail(String email) {
this.email = email;
return this;
}
public UserBuilder setAge(int age) {
this.age = age;
return this;
}
public User build() {
return new User(this);
}
}
}
In the example above, we have a User
class with a nested static class UserBuilder
. The builder provides methods to set different fields of the User
object. The build method creates the user instance using the builder's values.
Pros and Cons of the Builder Pattern
Before we improve its flexibility, let’s consider the pros and cons of the Builder Pattern:
- Pros:
- Clear and concise object construction.
- Easy to read and maintain.
- Reduces constructor overloading.
- Cons:
- Additional classes increase complexity.
- Can become verbose with many parameters.
Enhancing Flexibility with Additional Parameters
Now, let’s enhance our user builder by allowing more flexibility through additional parameters. When creating an object, sometimes you may want to provide additional information that doesn't strictly belong to its initial state.
Let's modify our UserBuilder
:
public class User {
private final String name;
private final String email;
private final int age;
private final String role;
private User(UserBuilder builder) {
this.name = builder.name;
this.email = builder.email;
this.age = builder.age;
this.role = builder.role;
}
public static class UserBuilder {
private String name;
private String email;
private int age;
private String role = "User"; // Default role
public UserBuilder setName(String name) {
this.name = name;
return this;
}
public UserBuilder setEmail(String email) {
this.email = email;
return this;
}
public UserBuilder setAge(int age) {
this.age = age;
return this;
}
public UserBuilder setRole(String role) {
this.role = role;
return this;
}
public User build() {
return new User(this);
}
}
}
Code Explanation
-
Additional Parameters: We added a
role
parameter to our builder. This allows users to specify roles such as "Admin", "User", etc., during object creation. -
Default Value: We assigned "User" as the default role. This ensures that the builder can be useful in both scoped and more complex use cases without instantly forcing every caller to provide a role.
-
Fluency: Users can still chain method calls, maintaining readability.
Usage Example
Below is an example that demonstrates the use of the enhanced UserBuilder
:
public class Main {
public static void main(String[] args) {
User adminUser = new User.UserBuilder()
.setName("John Doe")
.setEmail("john.doe@example.com")
.setAge(30)
.setRole("Admin")
.build();
User regularUser = new User.UserBuilder()
.setName("Jane Doe")
.setEmail("jane.doe@example.com")
.setAge(25)
.build();
System.out.println("Admin User: " + adminUser);
System.out.println("Regular User: " + regularUser);
}
}
Output
This code, when executed, will create two users with different roles, showcasing the flexibility in object creation.
Further Enhancements: Optional Parameters
Sometimes, you might want even more flexibility by making certain parameters optional. We can achieve this through method overloading and Service Objects.
Here's how to achieve that with our UserBuilder
:
public static class UserBuilder {
private String name;
private String email;
private int age;
private String role = "User"; // Default role
public UserBuilder setName(String name) {
this.name = name;
return this;
}
public UserBuilder setEmail(String email) {
this.email = email;
return this;
}
public UserBuilder setAge(int age) {
this.age = age;
return this;
}
// Overloaded method to allow users to create a user without setting a role
public UserBuilder setRole() {
this.role = null; // Indicates no specific role has been set
return this;
}
public User build() {
if (role == null) {
this.role = "User"; // Default to user if not set
}
return new User(this);
}
}
Explanation of Optional Parameters
-
Overloaded Method: The
setRole()
method can now be called without parameters, indicating that the caller does not want to set a specific role. -
Nullable Role Handling: If the role is not set, the builder will default to "User." This provides a guideline to maintain defaults while keeping constructors versatile.
Lessons Learned
The Builder Pattern, when enhanced with additional and optional parameters, becomes a powerful tool for managing complex object creation in applications. It allows for clear, maintainable, and flexible object construction, alleviating many common design pitfalls.
To further your understanding of design patterns in Java, consider consulting resources such as Refactoring Guru - Builder Pattern and Oracle Documentation.
In conclusion, next time you need to design complex objects, remember the Builder Pattern’s flexibility can handle evolving requirements. Happy coding!