Common Pitfalls in JUnit Testing Spring Services & DAOs
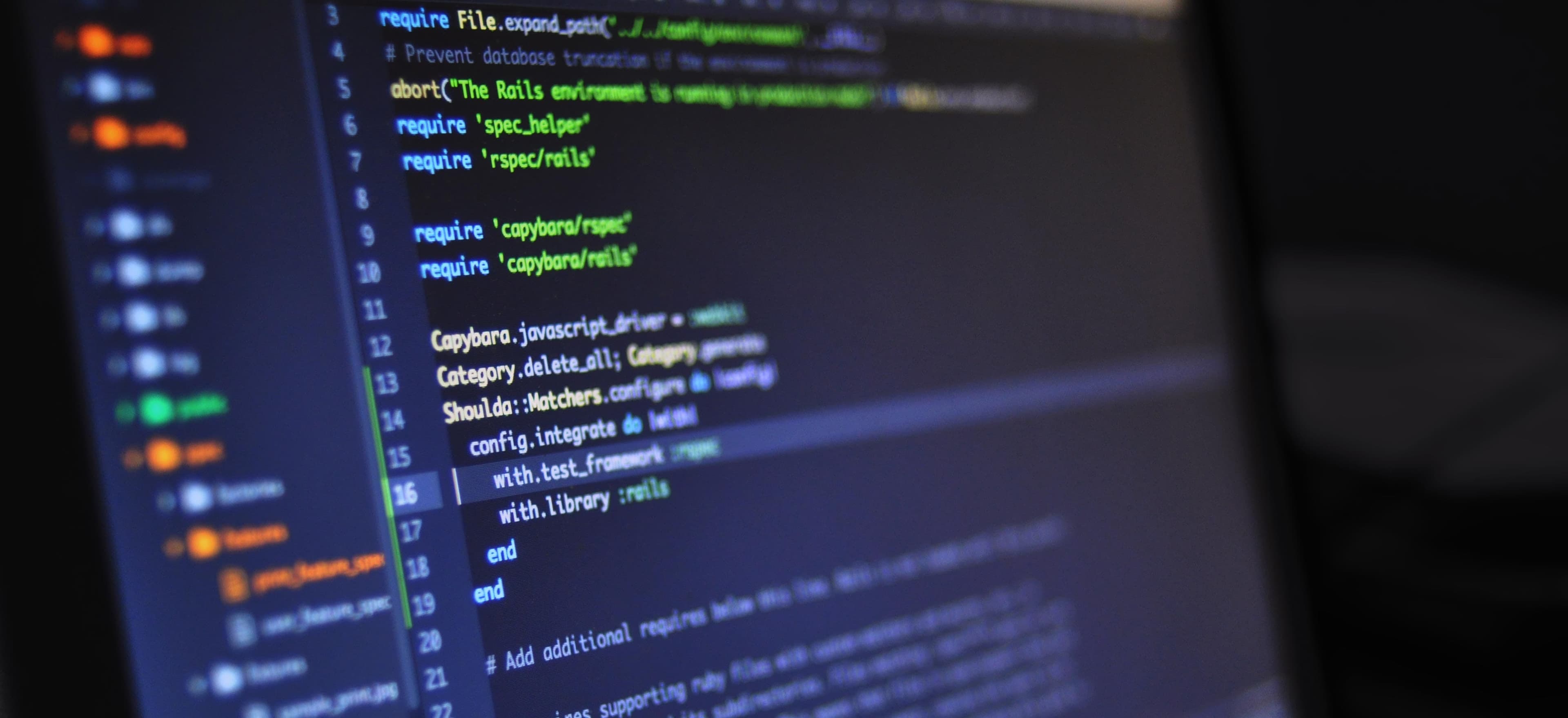
- Published on
Common Pitfalls in JUnit Testing Spring Services & DAOs
When it comes to testing Java applications built using the Spring framework, JUnit has become the go-to testing framework. It is powerful, versatile, and integrates seamlessly with Spring's testing capabilities. However, developers often encounter pitfalls that can lead to flaky tests, incorrect results, or tedious maintenance. In this blog post, we will discuss common pitfalls in JUnit testing for Spring services and Data Access Objects (DAOs) while offering practical solutions to help you write more effective tests.
Understanding the Role of Services and DAOs
Before diving into testing strategies, it's crucial to understand what services and DAOs are.
- Services typically encapsulate business logic and handle interactions between controllers and DAOs.
- DAOs (Data Access Objects) manage database interactions, providing an abstract interface to the underlying persistence layer.
These components interact closely, so testing them effectively ensures application reliability and performance.
Common Pitfalls
1. Not Using Annotations Properly
One of the biggest pitfalls is improper use of annotations. Spring's testing feature relies heavily on annotations like @SpringBootTest
, @MockBean
, and @Autowired
.
Solution
Make sure to use the correct annotations for your context. Here is an example of how to set up a simple test for a service using @SpringBootTest
:
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserServiceTest {
@Autowired
private UserService userService;
@MockBean
private UserRepository userRepository;
@Test
public void whenValidUserId_thenUserShouldBeFound() {
User user = new User(1L, "John Doe");
Mockito.when(userRepository.findById(1L)).thenReturn(Optional.of(user));
User found = userService.getUserById(1L);
assertEquals("John Doe", found.getName());
}
}
Commentary: Using @MockBean
, we can mock the UserRepository
, isolating the UserService
for focused testing. This approach prevents any side effects caused by actual database interactions.
2. Ignoring Configuration
Configuration management in Spring can be tricky. Failure to set up the right context can lead to ApplicationContext
failures or wrong configurations being used.
Solution
Always ensure that your test classes load the configuration they need. Use @ActiveProfiles
to specify the necessary profiles:
@SpringBootTest
@ActiveProfiles("test")
public class UserServiceTest {
// Test code
}
Commentary: By specifying the testing profile, we can configure our data source or any mocking frameworks to use controlled environments, reducing the chance of side effects.
3. Not Using Mocking Properly
Another common issue is the poor use of mocking frameworks. Real database interactions during tests can slow down execution and lead to SQL exceptions.
Solution
Utilize Mockito or any other mocking framework for external dependencies. Here's an example where we mock a DAO method:
@MockBean
private UserRepository userRepository;
@Test
public void whenUserNotFound_thenExceptionShouldBeThrown() {
Mockito.when(userRepository.findById(1L)).thenReturn(Optional.empty());
assertThrows(UserNotFoundException.class, () -> userService.getUserById(1L));
}
Commentary: By mocking the repository, we are controlling input and output, allowing us to focus on testing the service's logic without invoking the actual database.
4. Overlooking Transactional Boundaries
Spring manages transactions effectively, but if transactions are not handled properly in your tests, it could lead to unwanted data persistence.
Solution
Always use @Transactional
in your test classes to roll back the transaction after each test. For example:
@RunWith(SpringRunner.class)
@SpringBootTest
@Transactional
public class UserServiceTest {
// Test code
}
Commentary: Using @Transactional
ensures that any changes made during the test do not persist in the database, facilitating a clean state for each test run.
5. Writing Tests that Depend on Each Other
Creating tests that depend on the results of other tests is another common pitfall. Such tests suffer from fragility and can create confusion in debugging.
Solution
Write each test as a standalone unit. For instance, avoid this approach:
@Test
public void testCreateUser() {
userService.createUser("Jane Doe");
}
@Test
public void testFindUserByName() {
User user = userService.getUserByName("Jane Doe");
assertNotNull(user);
}
Instead, ensure the context is preserved by explicitly creating the user in each test, or utilize mocking:
@Test
public void testFindUser_shouldReturnSameUser() {
User user = new User(1L, "Jane Doe");
Mockito.when(userRepository.findByName("Jane Doe")).thenReturn(Optional.of(user));
User found = userService.getUserByName("Jane Doe");
assertNotNull(found);
}
Commentary: By using mocking for setup, tests become independent, reducing flakiness and making debugging easier.
6. Not Testing Edge Cases
Many developers focus only on happy paths, ignoring edge cases that can lead to unexpected behavior.
Solution
Consider a user's names or emails that might be null or empty. Add tests to handle these cases effectively:
@Test
public void whenCreatingUserWithEmptyName_thenExceptionShouldBeThrown() {
assertThrows(IllegalArgumentException.class, () -> userService.createUser(""));
}
@Test
public void whenUserNameIsNull_thenExceptionShouldBeThrown() {
assertThrows(NullPointerException.class, () -> userService.createUser(null));
}
Commentary: By addressing edge cases, we fortify the application against potential runtime errors.
7. Not Leveraging Parameterized Tests
Parameterized tests can dramatically reduce redundancy in your tests by allowing you to run the same test logic with different inputs.
Solution
Use JUnit's @ParameterizedTest
annotation for repetitive test scenarios:
@ParameterizedTest
@ValueSource(strings = {"", " ", null})
public void whenCreatingUserWithInvalidName_thenExceptionShouldBeThrown(String name) {
assertThrows(IllegalArgumentException.class, () -> userService.createUser(name));
}
Commentary: Parameterized tests allow for checking multiple conditions with ease, enhancing test coverage without escalating code duplication.
The Bottom Line
JUnit testing of Spring services and DAOs is an essential part of your development lifecycle. However, overlooking common pitfalls can lead to fragile and unreliable tests. By implementing the practices outlined in this article, you can enhance your testing strategy, ensuring that your Spring applications are robust, reliable, and maintainable.
For more detailed insights into testing with JUnit and Spring, consider checking out the official Spring testing documentation.
By committing to well-structured testing, you pave the way for higher code quality and a more successful software project. Happy testing!