Overcoming Common Pitfalls When Using Groovy Traits
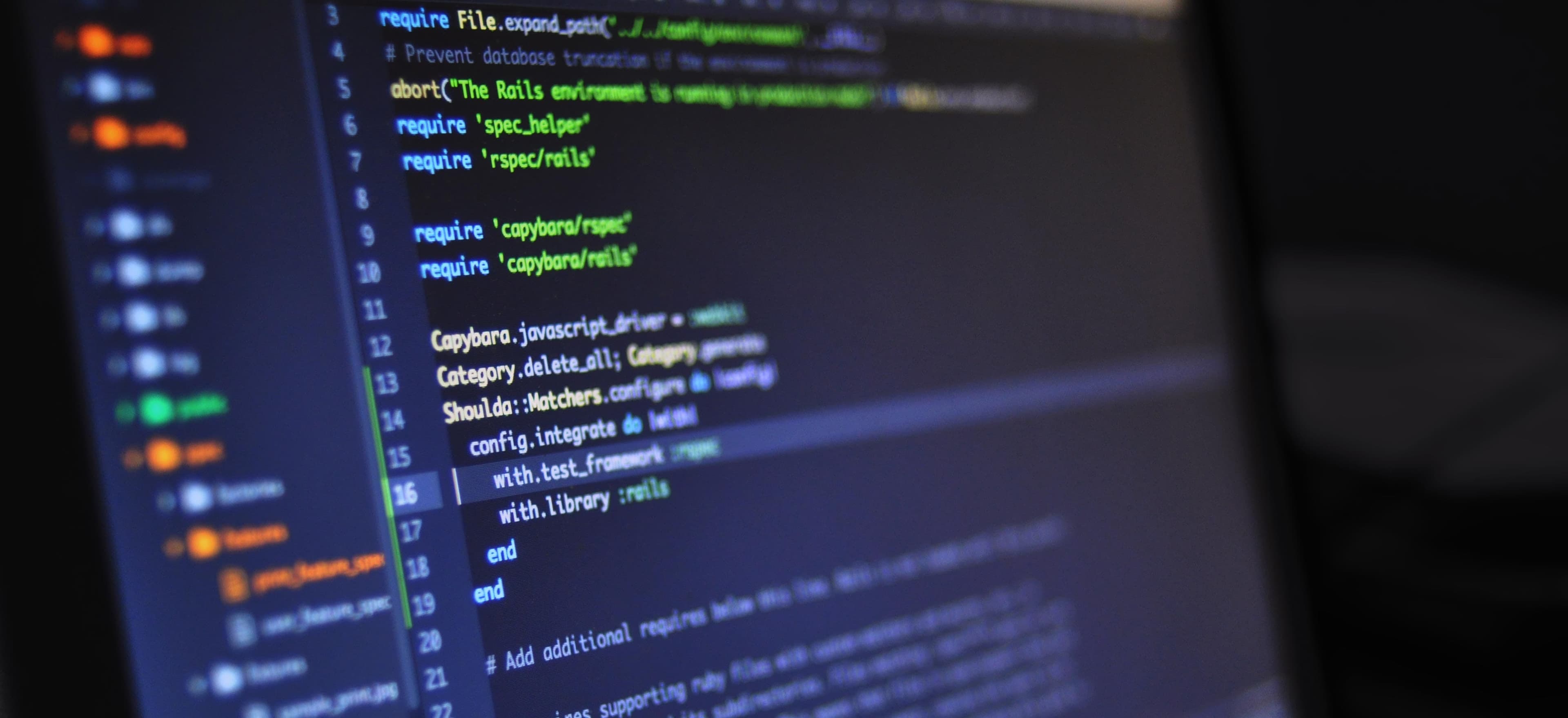
- Published on
Overcoming Common Pitfalls When Using Groovy Traits
Groovy, a powerful and dynamic language for the Java platform, simplifies many common programming tasks and introduces features like traits that enhance code reusability and maintainability. However, using Groovy traits comes with its own set of challenges. In this blog post, we will explore common pitfalls associated with Groovy traits and provide strategies to overcome them.
Understanding Traits in Groovy
Before we dive deep into the pitfalls, it's important to revisit what traits are. Traits allow you to define reusable functionality that can be composed into classes. Unlike traditional inheritance, traits provide a mechanism for extending behavior without tightly coupling classes.
trait GroovyTrait {
String sayHello(String name) {
return "Hello, ${name}!"
}
}
In this example, GroovyTrait
defines a simple method that can be incorporated into any class, enhancing code reuse and modular design.
Common Pitfalls When Using Traits
1. Confusing Traits with Interfaces
One of the most significant misunderstandings regarding traits is confusing them with interfaces. While both can define methods, traits can also provide concrete implementations.
Solution: Use traits for shared functionality rather than just method signatures. This allows you to implement behavior that can be directly used in classes.
trait LoggingTrait {
void log(String message) {
println "Log: ${message}"
}
}
class MyClass implements LoggingTrait {
void doSomething() {
log("Doing something important.")
}
}
In this snippet, MyClass
benefits from the LoggingTrait
, which provides actual implementation for the log functionality.
2. Diamond Problem
When multiple traits that include conflicting method implementations are mixed into a single class, it can lead to the notorious "diamond problem." This occurs when a class inherits from two traits with the same method signature, leading to ambiguity about which method to invoke.
Solution: Explicitly specify which trait method to use. Groovy provides a way to resolve this by using the withTrait
method or overriding the method to clarify intent:
trait TraitA {
String greet() {
return "Hello from Trait A"
}
}
trait TraitB {
String greet() {
return "Hello from Trait B"
}
}
class MyClass implements TraitA, TraitB {
@Override
String greet() {
return super.TraitB.greet() // Specify which greet to use
}
}
// Usage
def myInstance = new MyClass()
println myInstance.greet() // Prints: Hello from Trait B
In this example, MyClass
explicitly overrides the greet
method, resolving the diamond problem by specifying which trait’s method is called.
3. State Management and Traits
Traits can have fields, but they share state across all implementations. If multiple classes use the same trait, they might inadvertently maintain conflicting states.
Solution: Avoid using mutable state in traits. Instead, pass necessary data through constructor parameters or method arguments when possible.
trait CounterTrait {
int count = 0 // Not ideal
void increment() {
count++
}
}
class CounterA implements CounterTrait {}
class CounterB implements CounterTrait {}
// Usage
def counter1 = new CounterA()
def counter2 = new CounterB()
counter1.increment()
counter2.increment() // Both instances share the same state which can lead to confusion
Instead, consider using method parameters to handle the state:
trait CounterTrait {
int increment(int counter) {
return counter + 1
}
}
class CounterA implements CounterTrait {
void printCount() {
int counter = 5
println "Counter A: ${increment(counter)}"
}
}
class CounterB implements CounterTrait {
void printCount() {
int counter = 10
println "Counter B: ${increment(counter)}"
}
}
// Usage
new CounterA().printCount() // Outputs: Counter A: 6
new CounterB().printCount() // Outputs: Counter B: 11
4. Performance Considerations
Using traits may introduce some performance overhead, especially if you're working in performance-sensitive applications. Each trait incurs a certain cost during method resolution, possibly affecting the overall execution speed.
Solution: Limit the use of traits to scenarios where they provide significant enhancements in code reuse and clarity. Always benchmark and profile if traits mildly impact performance.
Best Practices for Using Groovy Traits
-
Limit Trait Use to Shared Behavior: Traits are designed for shared traits and functionality, not general-purpose methods. Use them judiciously.
-
Keep Traits Stateless: Avoid mutable fields in traits to prevent hidden dependencies and conflicting states.
-
Use
@Trait
Annotation: Consider using the@Trait
annotation for clear conveyance of intent when defining a trait. -
Document Trait Behavior: Clear documentation is crucial. Because traits can be composed in multiple ways, ensure that their behavior and purpose are well documented.
-
Test Traits Independently: Write unit tests for traits to ensure that they work as expected irrespective of how they are composed into classes. This aids in verifying their functionality across different contexts.
The Closing Argument
Groovy traits can significantly enhance your programming experience by promoting code reuse and modular design. However, as we've discussed, they come with their own challenges that can lead to pitfalls if not approached correctly. By understanding these pitfalls and following best practices, you can leverage the power of traits effectively while minimizing potential issues.
For more information on Groovy traits and design patterns, you might find these resources helpful:
- Groovy Traits Official Documentation
- Understanding Object-Oriented Design in Groovy
Embracing Groovy traits appropriately can lead to cleaner, more maintainable code. Happy coding!
Checkout our other articles