Troubleshooting Guava Cache with Regex Patterns: Common Issues
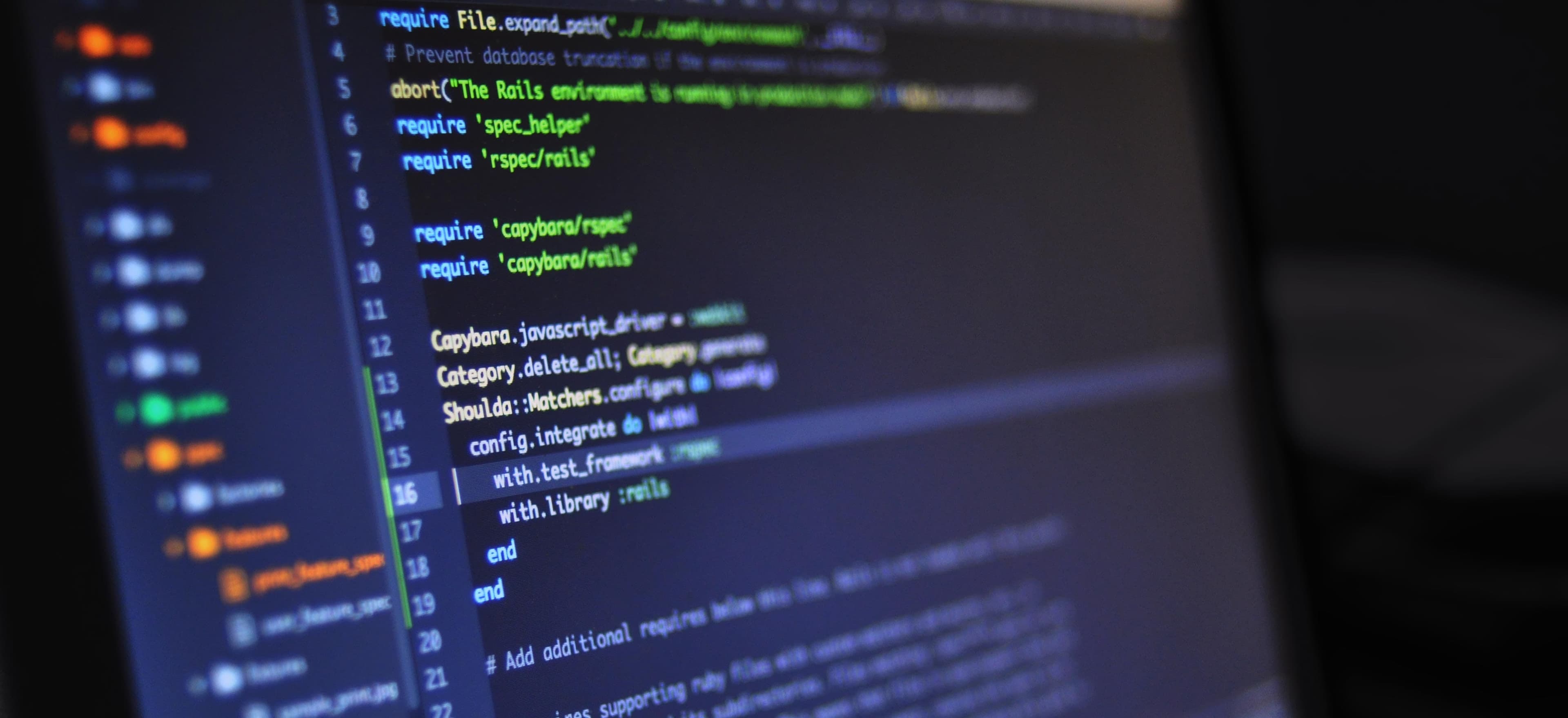
- Published on
Troubleshooting Guava Cache with Regex Patterns: Common Issues
When building Java applications, understanding how to optimize performance is key. One effective way to do this is by using caching mechanisms. Google's Guava library provides an efficient in-memory caching solution that allows developers to store and retrieve objects quickly. However, like any technology, issues can arise when utilizing it—particularly when integrating regular expressions to manage complex cache keys. In this blog post, we will explore common issues that developers face while using Guava Cache with regex patterns and discuss solutions to enhance your application's performance.
What is Guava Cache?
Guava Cache is a part of the Guava library, which offers a simple way to manage a cache of objects. It supports expiration, eviction, and refresh strategies—making it a powerful tool for improving application speed and responsiveness.
Example Usage of Guava Cache
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import java.util.concurrent.TimeUnit;
public class GuavaCacheExample {
public static void main(String[] args) {
LoadingCache<String, String> cache = CacheBuilder.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(100)
.build(new CacheLoader<String, String>() {
public String load(String key) {
return fetchFromDataSource(key);
}
});
// Example of using the cache
String value = cache.getUnchecked("someKey");
System.out.println(value);
}
private static String fetchFromDataSource(String key) {
// Simulate data fetching logic
return "Data for " + key;
}
}
In the code above, we create a LoadingCache
, which automatically loads values upon request. The cache expires entries after ten minutes, allowing stale data to be evicted automatically. It’s crucial to consider performance implications when deciding on cache configurations.
Why Use Regex Patterns in Cache Keys?
Using regex patterns allows for flexible key matching – you can treat similar keys as one. This approach can be particularly helpful when you deal with datasets that have a defined structure and follow predictable patterns. For example, cache patterns can help in scenarios like URL matching or user identifiers that share common attributes.
Common Issues and Solutions
1. Overly Broad Regex Patterns Leading to Collisions
Problem: Using a broad regex pattern can result in cache key collisions, where multiple distinct entities hash to the same key.
Solution: Define regex patterns carefully. Think through all possible values and ensure that your patterns are specific enough. Narrow regex patterns can reduce the likelihood of collisions.
String pattern = "user/[0-9]+"; // Poorly defined pattern leading to collisions
Refine the regex to something more specific:
String refinedPattern = "user/(?<id>[0-9]{1,5})"; // Better definition avoiding collisions
2. Cache Size Limit Configurations
Problem: Setting a maximum size limit for the cache without monitoring can lead to frequent cache evictions.
Solution: Monitor cache usage and adjust the size limit dynamically based on your application's needs. You can log cache hits and misses to gather insights on usage patterns.
Long hits = cache.stats().hitCount();
Long misses = cache.stats().missCount();
// Log for monitoring
System.out.println("Cache Hits: " + hits + ", Cache Misses: " + misses);
3. Difficulty in Updating Cached Values
Problem: When the underlying data changes, stale cache values can mislead your application.
Solution: Use a refresh strategy that keeps the cache up-to-date without stressing your data source. Guava has a built-in feature for asynchronous refresh which can be beneficial.
LoadingCache<String, String> cache = CacheBuilder.newBuilder()
.refreshAfterWrite(5, TimeUnit.MINUTES)
.build(new CacheLoader<String, String>() {
public String load(String key) {
return fetchFromDataSource(key);
}
});
4. Regex Performance Cost
Problem: Complex regex patterns can significantly degrade performance, especially if the regex is applied frequently.
Solution: Avoid using regex for frequent lookups. Instead of regex, consider using simpler string manipulation functions like String.contains()
or String.startsWith()
when appropriate. Always benchmark performance when changing logic.
if (key.startsWith("user/")) {
// Perform action
}
5. Caching Wrong Data Types
Problem: Developers sometimes cache the wrong data types or incompatible objects, leading to ClassCastException.
Solution: Ensure the cached data type matches the expected type throughout the code. Utilize generics in your cache definition.
LoadingCache<String, String> cache = CacheBuilder.newBuilder()
.build(new CacheLoader<String, String>() {
public String load(String key) {
// logic
}
});
6. Thread Safety Issues
Problem: If the cache is accessed from multiple threads, it can lead to inconsistent reads or race conditions.
Solution: Guava's LoadingCache
is inherently thread-safe. However, if you're mutating shared objects returned from the cache, ensure that appropriate synchronization is in place.
String value = cache.getUnchecked("someKey");
// Use synchronized block if modifying:
synchronized (value) {
// Perform modifications
}
Additional Resources
Wrapping Up
Working with Guava’s cache and regex patterns can significantly enhance performance when done correctly. However, it is essential to remain vigilant about potential pitfalls such as collisions, stale data, and performance costs. By optimizing your cache configuration and employing careful regex design, you can ensure a robust caching mechanism that bolsters your application speed and reliability.
In summary, always assess your caching strategies periodically and keep up with best practices. The right balance between regex flexibility and cache performance is key to building scalable and efficient Java applications. Take these troubleshooting tips into account, and you’ll be better equipped to handle issues that arise in your caching layer. Happy coding!
Checkout our other articles