Troubleshooting Common Errors in Spring MVC REST Apps
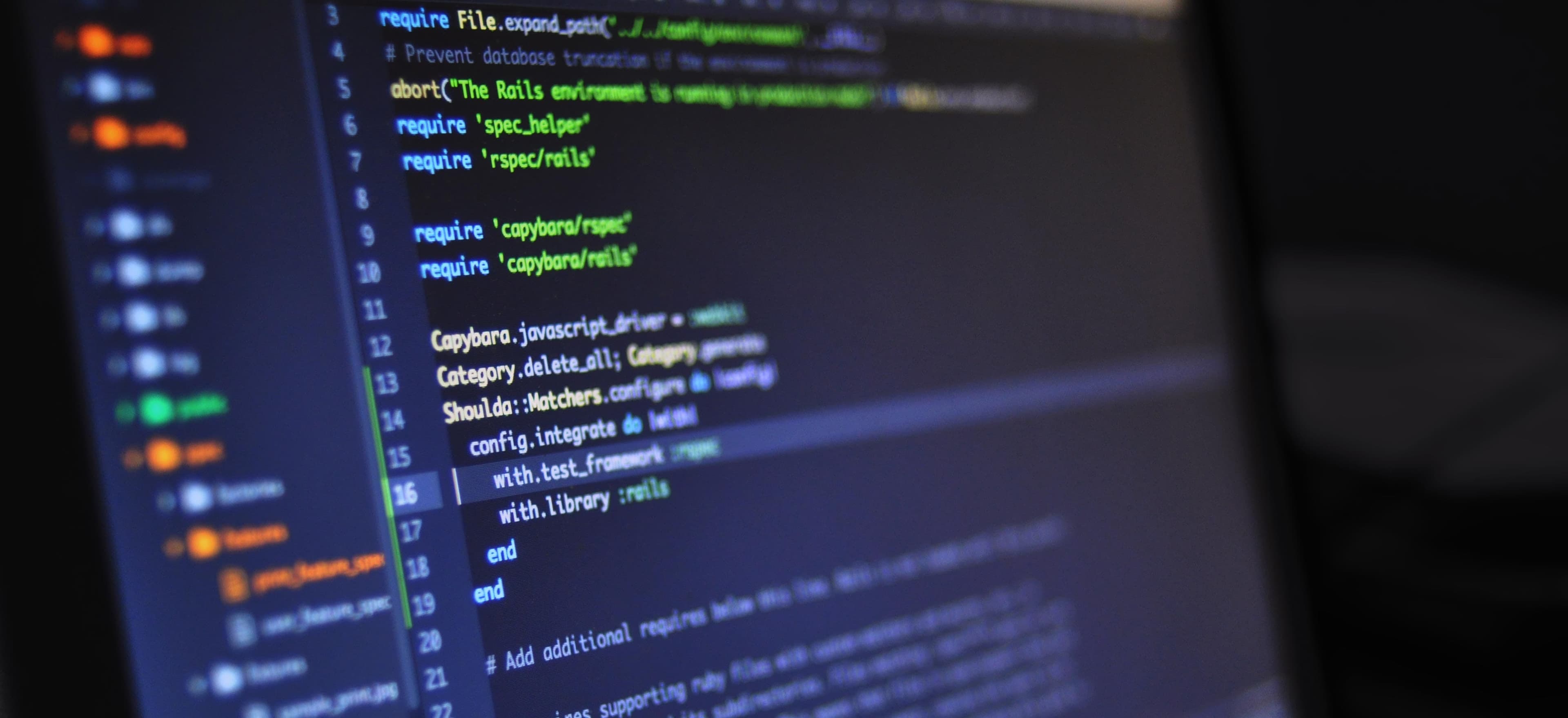
- Published on
Troubleshooting Common Errors in Spring MVC REST Apps
Spring MVC is a powerful framework for building RESTful web applications. However, even seasoned developers can encounter errors. In this blog post, we will delve into common issues faced during Spring MVC REST application development and how to troubleshoot them efficiently.
Table of Contents
- Introduction to Spring MVC
- Common Errors in Spring MVC REST Apps
- 2.1 Missing Request Mapping
- 2.2 HTTP Status 404 Not Found
- 2.3 HTTP Status 500 Internal Server Error
- 2.4 Binding Errors
- 2.5 CORS Issues
- Conclusion
1. Introduction to Spring MVC
Spring MVC is a module of the Spring Framework that helps in building web applications. The core of Spring MVC is based on the Model-View-Controller (MVC) design pattern, which separates the application into three interconnected components. This modularization allows for easier management, testing, and scalability.
To understand how the Spring MVC framework processes a request, consider this very basic controller snippet:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloWorldController {
@GetMapping("/hello")
public String hello() {
return "Hello, Spring MVC!";
}
}
In this example, the @RestController
annotation defines a controller that handles HTTP requests. The @GetMapping
annotation maps HTTP GET requests to the hello()
method. This simple setup gives us a clear entry point to troubleshoot common errors.
2. Common Errors in Spring MVC REST Apps
2.1 Missing Request Mapping
Issue: You may forget to add a required @RequestMapping
or @GetMapping
annotation to your controller methods.
Symptoms: Clients receive a 404 Not Found or an empty response.
Solution: Double-check your controller configuration and ensure that each method has the appropriate mapping annotation.
@GetMapping("/api/data")
public Data getData() {
return new Data();
}
Without this mapping, requests to /api/data
will yield no response.
2.2 HTTP Status 404 Not Found
Issue: A 404 error indicates that the requested resource wasn't found.
Symptoms: When accessing a valid URL doesn't yield the expected data.
Solution: Verify the following:
- Check the URL you're accessing matches the mapping in the controller.
- Ensure that your application is running and listening to the correct port.
@GetMapping("/api/users/{id}")
public User getUser(@PathVariable String id) {
return userService.findById(id);
}
- If
/api/users/123
is accessed, ensure that the route matches your actual controller method.
2.3 HTTP Status 500 Internal Server Error
Issue: A 500 error usually indicates that something went wrong on the server side.
Symptoms: Your API responds with a description of the error, typically due to an unhandled exception or configuration issues.
Solution: To troubleshoot this error, consider:
- Reviewing your server logs for specific error messages.
- Using
@ControllerAdvice
to handle exceptions globally.
Here’s how you can implement a global exception handler:
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR)
public String handleException(Exception ex) {
return "Error occurred: " + ex.getMessage();
}
}
Using @ControllerAdvice
, you can catch exceptions across all controllers and return a consistent error response.
2.4 Binding Errors
Issue: Binding errors typically arise when Spring cannot map incoming request data to an object.
Symptoms: Inadequate or null values are noticed in object fields.
Solution: Check the request format, especially with JSON data. Ensure you have Jackson dependencies for proper JSON handling:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</dependency>
To improve data binding, use annotations in your DTO (Data Transfer Object):
public class User {
@NotNull
private String username;
@Email
private String email;
}
Make sure your request body aligns with these fields. Sending an invalid object structure will lead to binding errors that can be easily traced through the logs.
2.5 CORS Issues
Issue: Cross-Origin Resource Sharing (CORS) issues frequently emerge when your front-end is trying to access your back-end hosted on a different domain.
Symptoms: You may see “No 'Access-Control-Allow-Origin'” errors in your browser console.
Solution: You can resolve this by configuring CORS in your Spring MVC application.
Use the following code snippet to allow CORS in your Spring application:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("http://localhost:3000");
}
}
This allows all endpoints (/**
) to accept requests from specified origins.
3. Conclusion
Troubleshooting common errors in Spring MVC REST applications can seem daunting at times. However, with a systematic approach and an understanding of the probable issues, developers can swiftly tackle these errors. From ensuring your request mappings are set properly to checking for CORS configurations, being methodical is key.
For additional resources on Spring MVC, you can refer to Spring's official documentation or explore error handling in depth through Baeldung's guides.
As always, happy coding! Feel free to leave questions or share your experiences with Spring MVC errors in the comments below.
Checkout our other articles