Overcoming Challenges of Spring Integration with Legacy Systems
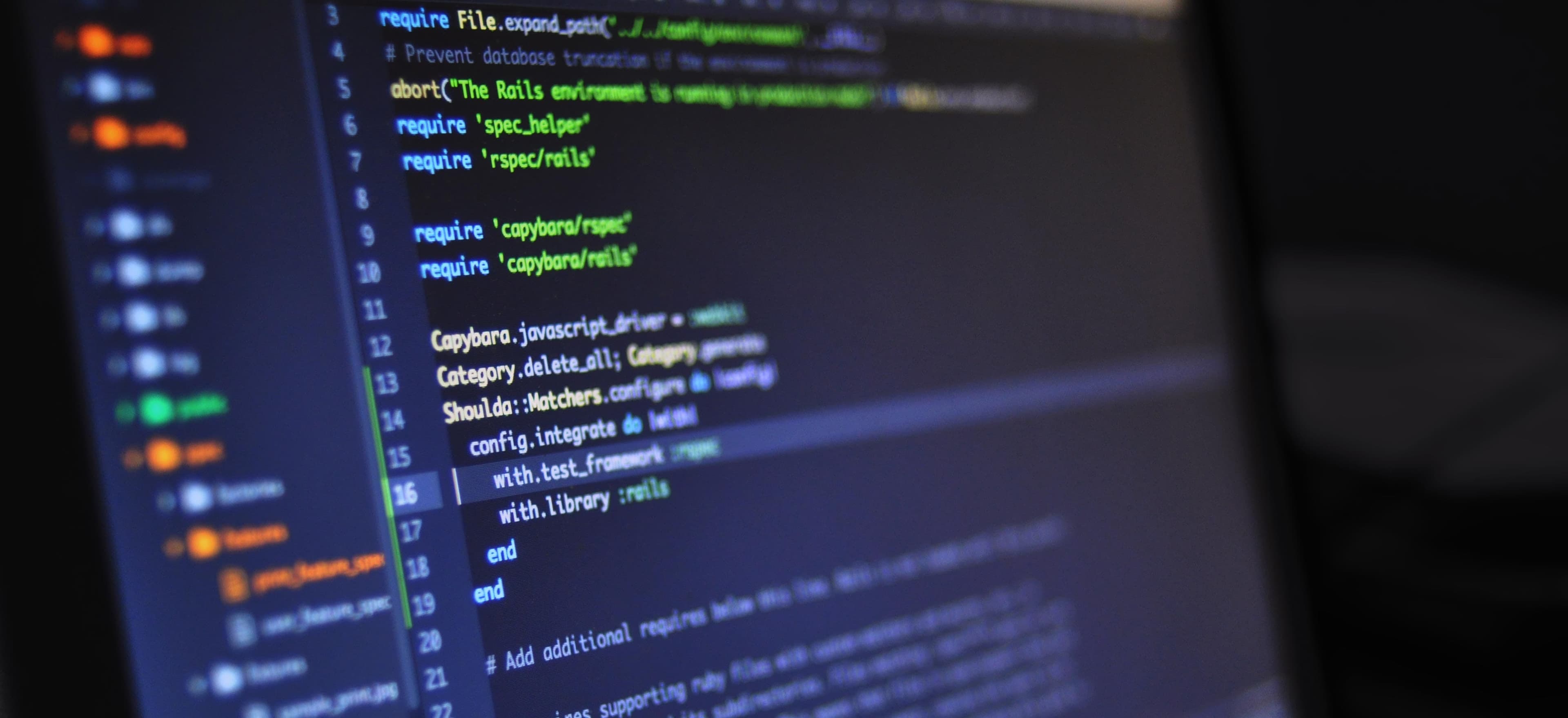
- Published on
Overcoming Challenges of Spring Integration with Legacy Systems
Integration of legacy systems in modern environments is a common challenge faced by many organizations today. As businesses evolve, the need to integrate old systems with new technologies becomes increasingly important for preserving investments and optimizing operations. In this blog post, we'll explore the challenges faced when integrating legacy systems using Spring Integration, along with strategies and code examples to overcome these hurdles.
What is Spring Integration?
Spring Integration is a powerful framework that provides an extension of the Spring programming model to support the integration of enterprise systems. Its main goal is to facilitate the development of integration solutions by using well-defined patterns and abstraction layers. Whether you are dealing with HTTP requests, messaging queues, or file transfers, Spring Integration provides a flexible way to integrate various systems seamlessly.
Common Challenges in Legacy System Integration
When dealing with legacy systems, several challenges can arise:
1. Incompatible Protocols and Formats
Legacy systems often use outdated protocols or data formats, making it difficult to communicate with modern applications.
2. Lack of Documentation
Many legacy systems were implemented years ago and may not have proper documentation. This can make understanding how to interact with the system a daunting task.
3. Limited Scalability
Legacy systems are typically not designed for scalability and may struggle to keep up with the volume of data and traffic.
4. Tight Coupling
Legacy systems may have been developed in a tightly coupled fashion, making it difficult to isolate components when integrating with new technology.
5. Security Concerns
Older systems may not adhere to current security standards, exposing organizations to vulnerabilities during integration.
Strategies to Overcome Integration Challenges
To address these challenges, several strategies can be employed. Below, we outline key approaches while providing code snippets to illustrate them.
Strategy 1: Use Adapters for Communication
Spring Integration provides the concept of adapters to facilitate communication with legacy systems. Adapters can help translate incompatible protocols and formats.
For instance, if your legacy system exposes a SOAP web service, you can use the Spring Web Service module to create an adapter.
@Bean
public WebServiceOutboundGateway legacySystemGateway() {
WebServiceOutboundGateway gateway = new WebServiceOutboundGateway("http://legacy-system-url");
gateway.setRequestFactory(new HttpComponentsMessageFactory());
return gateway;
}
Why this works: By configuring an outbound gateway, we have streamlined the communication process with the legacy SOAP service, handling the underlying complexities for us.
Strategy 2: Utilize Messaging
Using message-driven architectures can help decouple your applications from legacy systems. A message broker, such as RabbitMQ or Kafka, can serve as an intermediary.
@Bean
public IntegrationFlow integrationFlow() {
return IntegrationFlows.from("inputChannel")
.handle(legacySystemGateway())
.get();
}
Why this works: By using an input channel, we've effectively decoupled the input from the output. This allows for retries and buffering, making the system more resilient.
Strategy 3: Transform Incoming Data
Data from legacy systems may require transformation before it is usable. The Spring Integration transformation module provides tools like the Transform
processor.
@Bean
public IntegrationFlow transformFlow() {
return IntegrationFlows.from("legacyChannel")
.transform(new MyCustomTransformer())
.handle("myService", "processData")
.get();
}
Why this works: Applying a custom transformer ensures that any incompatible data formats are converted to a format that the modern system can work with.
Strategy 4: Implement Robust Error Handling
Legacy systems can be unpredictable. Implementing robust error handling mechanisms is essential in an integration flow.
@Bean
public IntegrationFlow handleErrorFlow() {
return IntegrationFlows.from("errorChannel")
.handle(message -> System.out.println("Error occurred: " + message.getPayload()))
.get();
}
Why this works: By listening to an error channel, we can log and respond to integration issues, thus allowing for troubleshooting and recovery.
Strategy 5: Gradual Migration
Attempting to replace a legacy system all at once can be risky. A gradual migration approach, where new features are integrated while the old system remains, can minimize disruption.
- Identify the most critical functions of the legacy system.
- Develop new services using modern technologies that gradually replace these functions.
- Ensure features are consistent during this transition.
Closing Remarks: The Road Ahead for Legacy Integration
Integrating legacy systems with Spring Integration can pose multiple challenges but can be managed with the right strategies. By leveraging the features of Spring Integration, including adapters, messaging, and transformation capabilities, organizations can facilitate seamless communication between old and new technologies.
The key to success lies in understanding your legacy systems' specific challenges and architecting your integration solutions to address those challenges effectively.
For further reading on Spring Integration, consider exploring the Spring Integration documentation for detailed insights and examples.
By accepting the complexity involved in integrating legacy systems and utilizing the appropriate tools provided by frameworks like Spring Integration, enterprises can unlock the value trapped within their historical systems while paving the way for future growth.
Additional Resources
- Spring Integration Samples
- Microservices Patterns: With examples in Java
- RabbitMQ Documentation
Embark on your integration journey today and turn your legacy challenges into opportunities for improvement.
By following these principles, you will be better equipped to tackle the diverse challenges associated with legacy system integration. Happy coding!
Checkout our other articles