Struggling with JUnit? Tips to Simplify ADF Unit Testing!
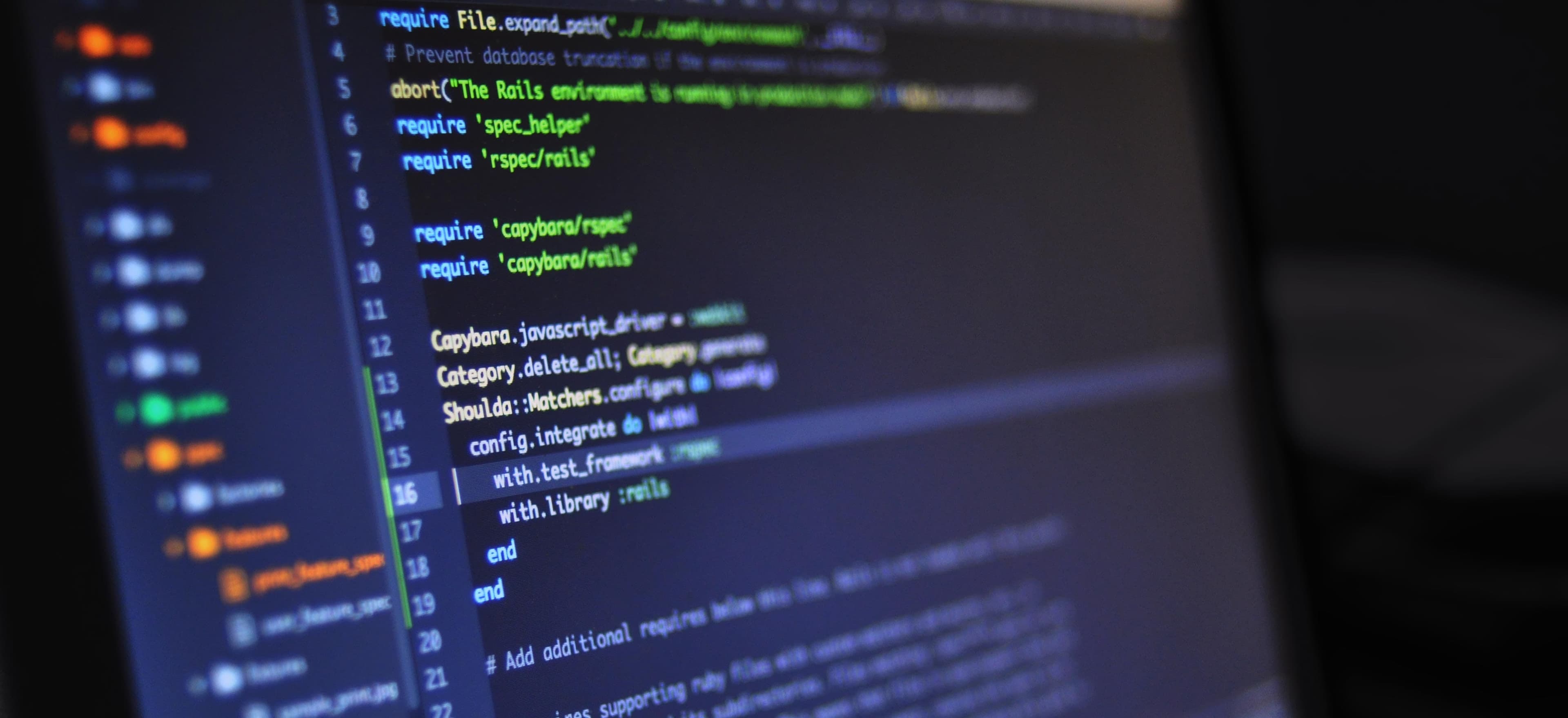
- Published on
Struggling with JUnit? Tips to Simplify ADF Unit Testing!
Unit testing is an essential part of software development, especially when working with Java applications. When it comes to Oracle ADF (Application Development Framework), JUnit serves as a powerful tool to ensure that your application behaves as intended. Despite its capabilities, developers often find unit testing in ADF challenging. This blog post aims to provide tips and best practices to simplify ADF unit testing with JUnit, ensuring that you can write effective and maintainable tests with less hassle.
What is JUnit?
JUnit is a widely-used testing framework in the Java ecosystem, providing a structured way to write and run tests. It is designed around the principle of “test-first” development, supporting the creation of unit tests for individual components. Its ease of use makes JUnit a go-to choice for many developers.
Learn more about JUnit through the JUnit 5 User Guide.
Challenges in ADF Unit Testing
Unit testing an ADF application can be tricky due to its complex architecture. Here’s a checklist of common challenges:
- Heavy Dependency on the ADF Framework: ADF applications often rely on various ADF components and configurations that can be cumbersome to mock.
- Complex Object Lifecycles: ADF’s data binding and lifecycle management can make it challenging to isolate units of work.
- Integration with other Oracle tools: ADF applications may integrate with databases and other services, which brings additional complexity.
Tips to Simplify ADF Unit Testing with JUnit
To simplify these challenges, let’s go through actionable tips that can facilitate effective ADF unit testing.
1. Use Mocks and Stubs
To overcome the heavy dependencies, consider using mocking frameworks such as Mockito. Mocks help isolate the unit under test by simulating its dependencies.
import static org.mockito.Mockito.*;
public class MyServiceTest {
private MyService myService;
private Dependency mockDependency;
@Before
public void setUp() {
mockDependency = mock(Dependency.class);
myService = new MyService(mockDependency);
}
@Test
public void testMyServiceMethod() {
when(mockDependency.someMethod()).thenReturn(someValue);
myService.myMethod();
verify(mockDependency).someMethod();
}
}
Why? The above code creates a mock for a dependency (Dependency
) and allows you to specify its behavior without needing the actual implementation. This drastically reduces the setup complexity.
2. Use the JUnit Lifecycle Annotations
JUnit provides annotations that help manage life cycles—like @Before and @After. Use these to set up your tests efficiently.
@Before
public void init() {
// Set up initial test environment
}
@After
public void cleanUp() {
// Clean up resources
}
Why? These annotations help centralize common setup and teardown activities, making your code DRY (Don’t Repeat Yourself) and more maintainable.
3. Test Individual Components
Whenever possible, break down tests to target individual components, such as service layers or business logic, rather than entire ADF pages.
public class ProductServiceTest {
private ProductService productService;
@Before
public void setUp() {
productService = new ProductService();
}
@Test
public void testCalculateDiscount() {
double discount = productService.calculateDiscount(100, 10);
assertEquals(90, discount, 0);
}
}
Why? Focusing on individual components allows for faster execution and easier debugging, as you can isolate faults effectively.
4. Integration Tests with Arquillian or Spring
While unit tests focus on individual components, integration tests can be important for verifying interactions with the ADF framework itself. Tools like Arquillian or Spring Test can help create comprehensive integration tests.
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath:test-context.xml")
public class UserServiceIntegrationTest {
@Autowired
private UserService userService;
@Test
public void testUserCreation() {
userService.createUser("testUser");
User user = userService.findUser("testUser");
assertNotNull(user);
}
}
Why? Integration tests like the one above ensure that various components interact correctly in a production-like environment. They can catch issues such as configuration problems that unit tests might miss.
5. Use Dependency Injection
Utilize Spring's dependency injection framework to manage dependencies effectively in your tests. This can facilitate clean and maintainable test setups.
@SpringBootTest
public class OrderServiceTest {
@Autowired
private OrderService orderService;
@Test
public void testPlaceOrder() {
orderService.placeOrder(orderDetails);
assertTrue(orderService.isOrderPlaced());
}
}
Why? Spring manages the lifecycle of your beans and injects dependencies, making it easier to swap out components for testing.
6. Test Exception Handling
Don't forget to test how your application handles exceptions. This is crucial for building robust ADF applications.
@Test(expected = CustomException.class)
public void testExceptionHandling() {
myService.callMethodThatThrowsException();
}
Why? Testing exception handling safeguards your application against unexpected failures and ensures your error-handling logic works as intended.
7. Continual Learning and Community Support
The ADF community and resources can provide invaluable support. Join forums like Oracle's ADF forums, or explore resources such as the Oracle ADF Documentation to keep up with best practices.
Key Takeaways
Unit testing in ADF can initially seem daunting due to the framework's complexity. However, by implementing these simplified strategies, developers can create effective tests that ensure their applications run without issues.
Remember, unit testing will not only catch bugs early in development but also contribute to better software design, among other benefits like improved project documentation. The more you practice and learn, the better you will get. Embrace the process, and happy testing with JUnit!
By incorporating these best practices and leveraging the capabilities of JUnit, you can transform your approach to ADF unit testing from a struggle into a streamlined, efficient process.
Checkout our other articles