Beware the Pitfalls of Resizing Your HashMap
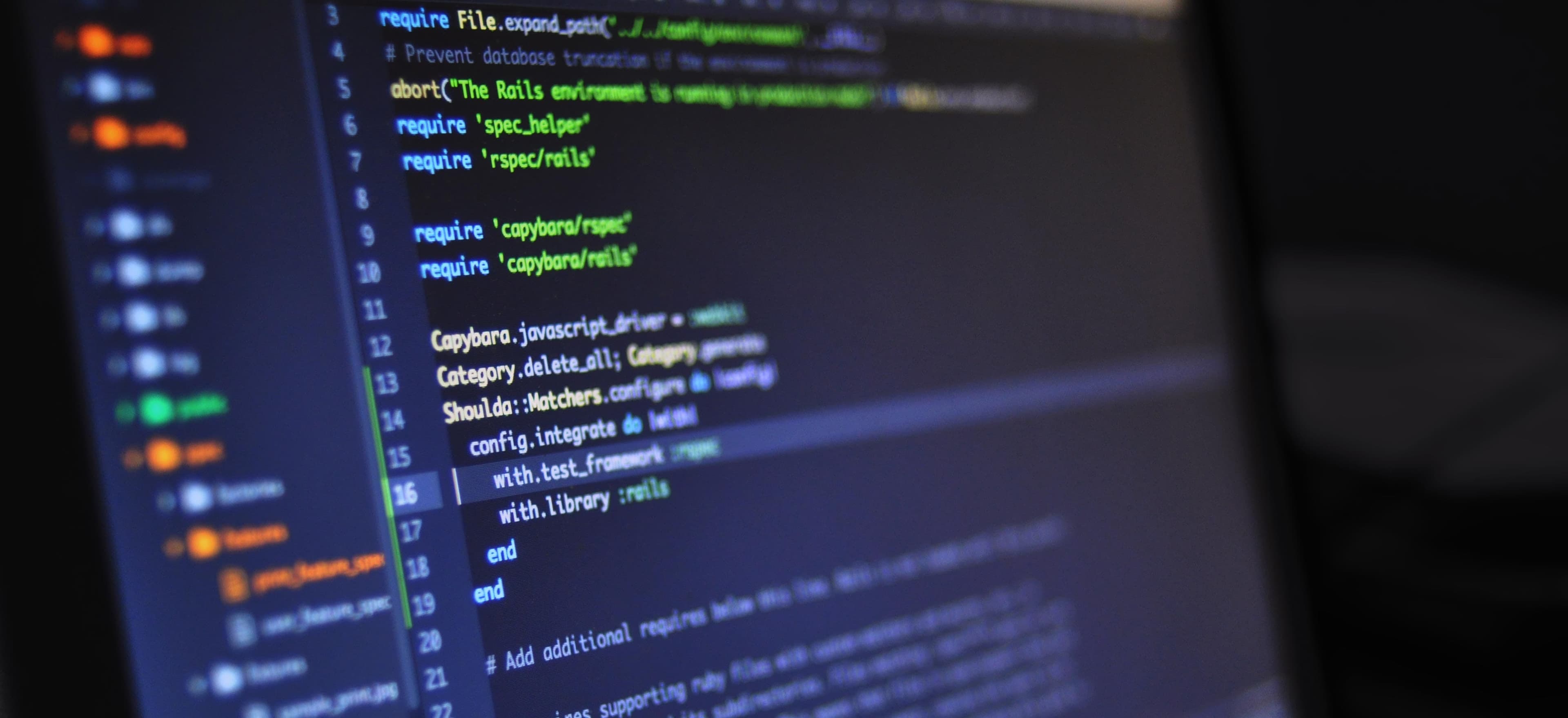
- Published on
Beware the Pitfalls of Resizing Your HashMap
In the vast landscape of Java collections, the HashMap
stands out as one of the most widely used data structures. Its ability to provide fast lookups, insertions, and deletions make it a staple for developers. However, like any powerful tool, its misuse can lead to performance issues and bugs if not handled properly. One of the critical aspects of effectively using a HashMap
is understanding how and when it resizes. In this post, we will explore the pitfalls of resizing your HashMap
and how to mitigate these issues.
What is a HashMap?
A HashMap
in Java is a part of the Java Collections Framework and stores items in key-value pairs. It allows null values and one null key. Internally, it uses an array of lists (buckets) where the keys are hashed to determine their index in the array.
Basic Example
Here's a simple example demonstrating the use of a HashMap
:
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
// Adding elements to the HashMap
map.put("Alice", 30);
map.put("Bob", 25);
map.put("Charlie", 35);
// Retrieving elements
System.out.println("Alice's age: " + map.get("Alice")); // Output: 30
}
}
When Does HashMap Resize?
A HashMap
automatically resizes itself when the number of entries exceeds a certain threshold, known as the load factor. By default, the load factor is set to 0.75. This means that when the number of entries in the HashMap
exceeds 75% of its current capacity, it will resize.
How Resizing Works
When resizing occurs, the following steps are taken:
- A new, larger array is created.
- All existing entries are rehashed and moved to the new array, with their keys recalculated for optimal performance.
This process can be computationally expensive, especially if your HashMap
contains many entries. The operation takes O(n) time complexity, where n is the number of entries in the map.
Pitfalls of Resizing
Performance Overhead
When a HashMap
resizes, the performance impact can be significant. Since all key-value pairs must be rehashed, this can lead to noticeable delays, especially in performance-critical applications.
Solution: To mitigate the performance overhead, you can specify an initial capacity for the HashMap
.
// Initial capacity of 1000 and load factor of 0.75
HashMap<String, Integer> map = new HashMap<>(1000, 0.75f);
This approach reduces the chance of resizing during normal operations, improving performance.
Memory Consumption
A larger HashMap
requires more memory. As you increase the initial capacity to avoid resizing, you might waste memory if your HashMap
does not ultimately hold that many entries.
Solution: To find the right balance, assess the expected number of entries you will store.
Infinite Loop with Concurrent Modifications
Another pitfall arises with concurrent modifications. If a HashMap
is being accessed while resizing occurs (for example, in a multi-threaded environment), it may lead to an ConcurrentModificationException
.
Solution: Use ConcurrentHashMap
for thread-safe operations. Unlike HashMap
, it handles concurrent modifications and allows for safe resizing.
import java.util.concurrent.ConcurrentHashMap;
ConcurrentHashMap<String, Integer> concurrentMap = new ConcurrentHashMap<>();
Predicting Resizing Behavior
Understanding resizing can help avoid unexpected behavior. For instance, if you insert a large number of elements in a loop, the last few operations might trigger resizing, causing performance to dip unexpectedly.
for (int i = 0; i < 10000; i++) {
map.put("Key" + i, i);
}
If the current capacity of the map is small, you will experience significant slowdowns once the resizing trigger occurs.
Load Factor Misunderstanding
A common misconception is that lowering the load factor always yields better performance. While a lower load factor can reduce hash collisions, it also increases memory consumption and the number of buckets.
Solution: Choose a load factor according to your application’s needs. Test various configurations under realistic workloads to find the best setting.
Best Practices
-
Initialize Appropriately: Start with an estimated capacity based on expected entries to minimize resizing.
-
Avoid Frequent Resizing: Perform batch insertions if possible. This way, you reduce the chances of multiple resizes occurring in quick succession.
-
Performance Testing: Assess the performance of your
HashMap
under load. Monitor its resizing behavior to identify potential bottlenecks. -
Utilize Thread-Safe Alternatives: For multi-threaded applications, prefer
ConcurrentHashMap
to eliminate concurrent modification issues.
My Closing Thoughts on the Matter
While the HashMap
provides quick access to collections of data, resizing can present notable performance challenges if not managed properly. By understanding how resizing works, anticipating resizing events, and applying best practices, you can leverage the full potential of HashMap
in your Java applications.
Resizing is an inherent part of the HashMap
architecture, but with a strategic approach, you can avoid its pitfalls and ensure your application runs smoothly.
For more information on Java Collections, check out the Java Collections Framework.
Consider incorporating these lessons into your next project, and watch out for those potential pitfalls. Happy coding!
Checkout our other articles