Essential Java 8 Tutorials for Overcoming Common Pitfalls
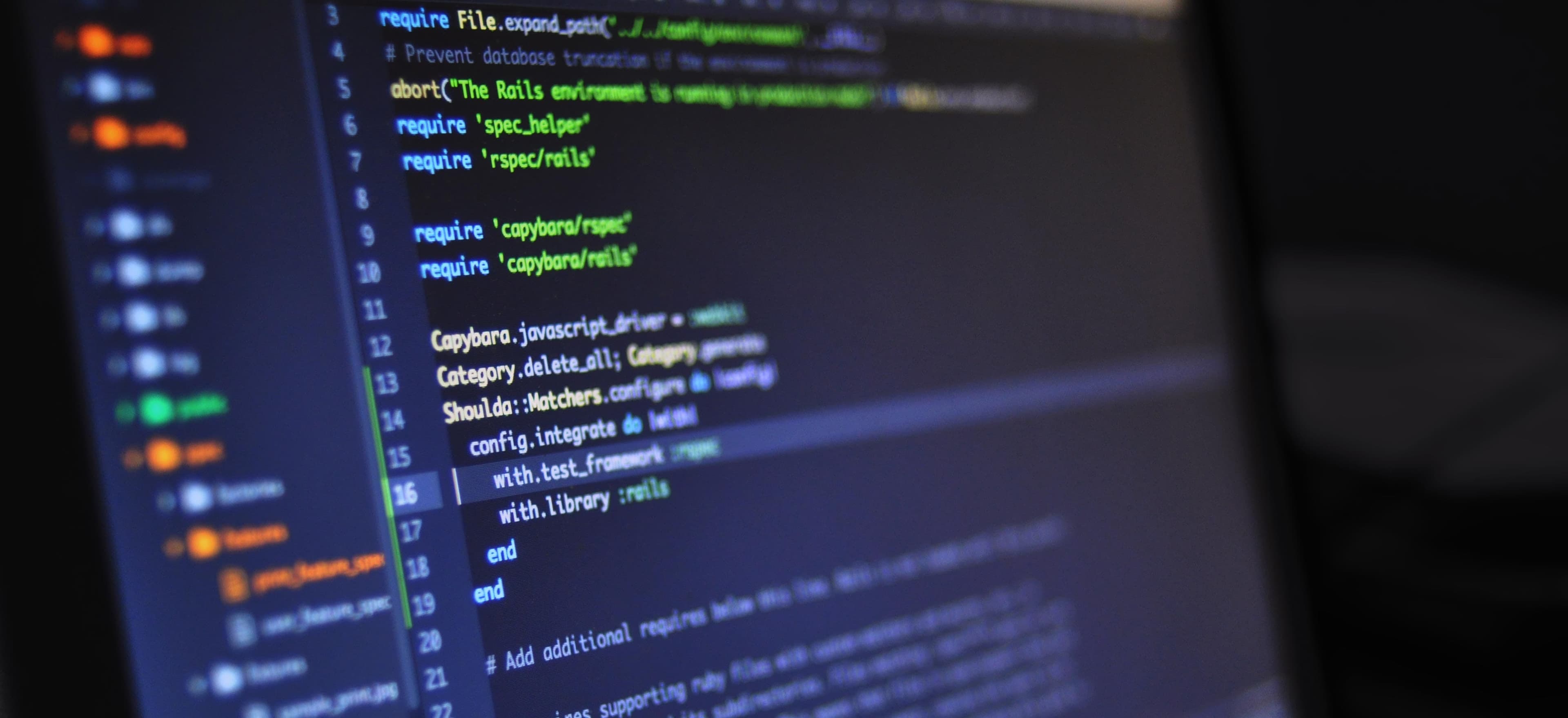
- Published on
Essential Java 8 Tutorials for Overcoming Common Pitfalls
Java 8 has fundamentally transformed the Java landscape, introducing significant features that enhance productivity and code readability. However, with great power comes great responsibility—or, in programming terms, great complexity. This blog post aims to encapsulate essential Java 8 tutorials to help you navigate common pitfalls and master this powerful version of the language.
Understanding Java 8 Features
Before diving into specific tutorials, let's quickly outline the pivotal features that Java 8 introduced:
- Lambda Expressions: These are a new way to express instances of single-method interfaces (functional interfaces).
- Streams API: A new abstraction that allows for functional-style operations on collections.
- Optional Class: A container object which may or may not contain a value, designed to reduce null-checks.
- Default Methods: Method implementations that interfaces can have, promoting backward compatibility.
- New Date and Time API: A comprehensive framework for date and time manipulation.
Now that we have an understanding, let's dig deeper into these features and tackle common pitfalls.
1. Mastering Lambda Expressions
Pitfall: Misunderstanding Scope and Final Variables
Lambda expressions can lead to confusion regarding variable scope. In Java, variables used within a lambda must be effectively final. If you modify a variable that is used inside a lambda, you will encounter a compilation error.
Example Code:
public class LambdaScope {
public static void main(String[] args) {
int number = 5;
Runnable runnable = () -> {
// number++; // Uncommenting this will cause a compilation error
System.out.println("Number: " + number);
};
runnable.run();
}
}
Why It Matters: Understanding scope and mutability ensures that you can leverage lambdas without running into unexpected behavior. Always remember that internal state manipulation could lead to side effects.
Additional Reading
- Java SE 8: The Complete Java Developer Course
2. Utilizing the Streams API
Pitfall: Not Handling Lazy Evaluation Properly
The Streams API operates under the principle of lazy evaluation. This means that intermediate operations are not executed until a terminal operation is invoked. If you don't grasp this concept, you might end up with unprocessed streams.
Example Code:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Lazy evaluation: No operation here yet
numbers.stream()
.filter(n -> n % 2 == 0); // Intermediate operation
// Terminal operation: now the above will execute
long count = numbers.stream()
.filter(n -> n % 2 == 0)
.count();
System.out.println("Even Numbers Count: " + count);
}
}
Why It Matters: Being aware of lazy evaluation helps you chain multiple operations without unnecessary performance costs, ensuring efficiency.
Additional Reading
3. Embracing the Optional Class
Pitfall: Overusing Optionals
While the Optional
class is a fantastic tool to avoid NullPointerExceptions, overusing it can lead to convoluted code and poor performance. It’s crucial to understand when to apply it.
Example Code:
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
String str = null;
Optional<String> optionalStr = Optional.ofNullable(str);
// Safe access
String finalStr = optionalStr.orElse("default");
System.out.println("String Value: " + finalStr); // Output: default
}
}
Why It Matters: Use Optional
primarily for method return types instead of local variables to maintain code clarity and performance.
Additional Reading
- Opt for Optional: A Cautionary Tale
4. Navigating Default Methods
Pitfall: Class Implementation Conflicts
Default methods allow you to add new methods to interfaces without breaking existing implementations. However, conflicts can arise if a class implements multiple interfaces with default methods sharing the same name.
Example Code:
interface InterfaceA {
default void show() {
System.out.println("Interface A");
}
}
interface InterfaceB {
default void show() {
System.out.println("Interface B");
}
}
class ConcreteClass implements InterfaceA, InterfaceB {
public void show() {
InterfaceA.super.show(); // Resolving conflict
}
}
public class DefaultMethodExample {
public static void main(String[] args) {
new ConcreteClass().show(); // Output: Interface A
}
}
Why It Matters: Handling conflicts ensures that the code is clear and maintainable. Override default methods appropriately to specify the desired behavior.
Additional Reading
- Understanding and Using Default Methods in Java 8
5. Working with the Date and Time API
Pitfall: Not Accounting for Time Zones
The new Date and Time API can be daunting. One common mistake is forgetting to consider time zones, which can lead to incorrect date and time calculations.
Example Code:
import java.time.LocalDateTime;
import java.time.ZoneId;
public class DateTimeExample {
public static void main(String[] args) {
LocalDateTime localDateTime = LocalDateTime.now();
ZoneId zoneId = ZoneId.of("America/New_York");
LocalDateTime newYorkTime = LocalDateTime.now(zoneId);
System.out.println("Current Local Date Time: " + localDateTime);
System.out.println("New York Time: " + newYorkTime);
}
}
Why It Matters: The Date and Time API emphasizes accuracy, especially when dealing with global applications. Always be timezone-aware to avoid bugs related to date and time.
Additional Reading
Bringing It All Together
Java 8 introduced myriad powerful features that can facilitate more readable, maintainable, and efficient code. However, with these features come common pitfalls that can trip up developers, especially those new to Java 8 features.
Understanding lambda expressions, leveraging the streams API, utilizing the Optional class, navigating default methods, and working correctly with the Date and Time API are essential skills for any modern Java developer. By mastering these topics, you can mitigate typical pitfalls and write cleaner, more effective code.
As you continue your Java journey, consider diving into the provided additional readings to further enhance your understanding. Happy coding!
Want to stay updated with the latest Java trends? Follow us for more insightful content! 🚀