Overcoming Common Challenges with JasperReports in JSF
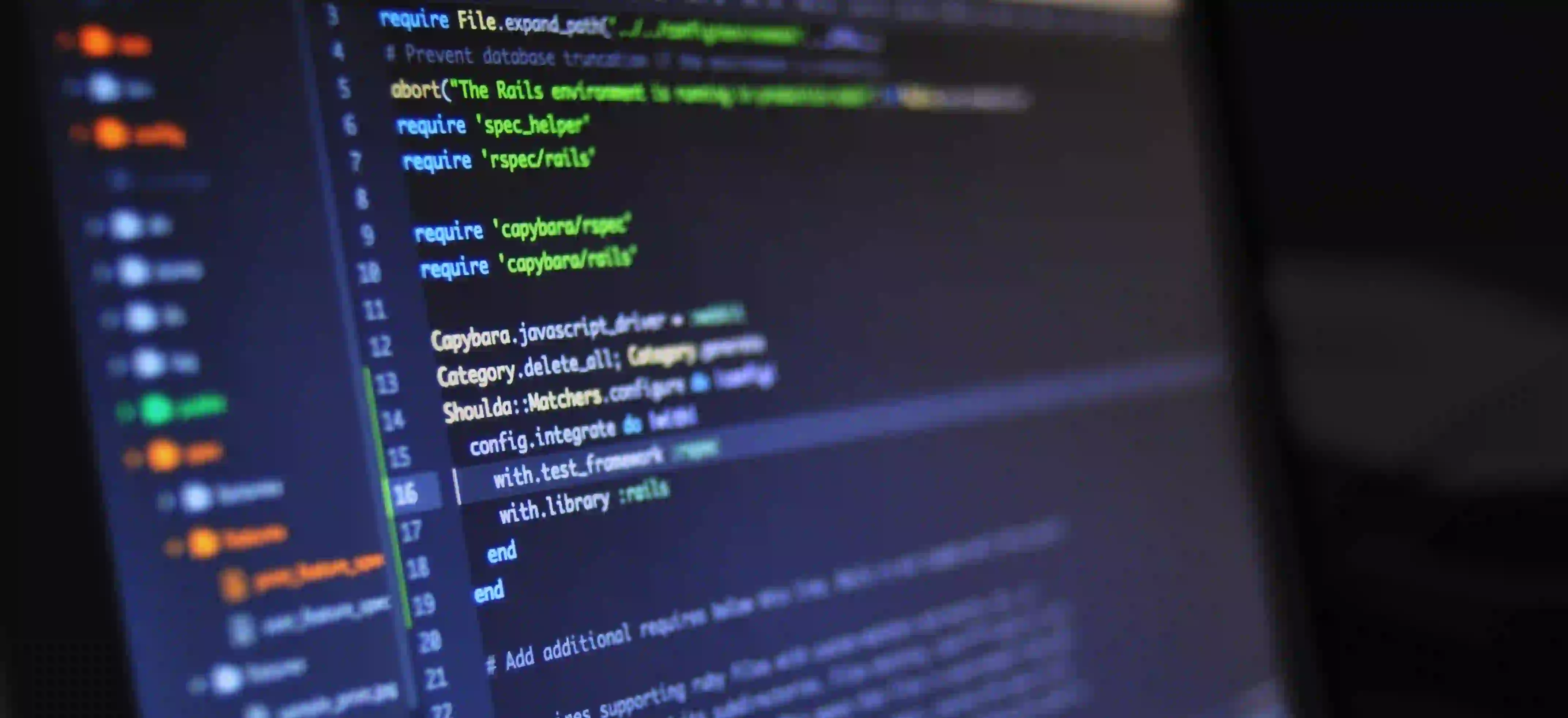
Overcoming Common Challenges with JasperReports in JSF
JasperReports is a powerful reporting tool widely used for generating dynamic reports in Java applications. When you integrate JasperReports with JavaServer Faces (JSF), developers often face various challenges. Java's loosely coupled architecture combined with JSF's component-based model can lead to complexities, particularly when handling report generation.
This blog post will focus on the common challenges encountered when using JasperReports in JSF and explore practical solutions, including code snippets and best practices.
Table of Contents
- Introduction to JasperReports and JSF
- Common Challenges
- Report Execution in the JSF Lifecycle
- Handling Report Parameters
- Exporting Reports in Various Formats
- Solutions to Challenges
- Setting Up Report Execution
- Passing Parameters Cleanly
- Implementing Export Functions
- Conclusion
Let us Begin to JasperReports and JSF
JasperReports is a versatile reporting engine that allows developers to create complex reports in different formats, including PDF, HTML, and Excel. It provides a robust API for report generation and is often used in enterprise applications. To create dynamic and interactive user interfaces, developers often use JSF, a Java specification for building component-based user interfaces for web applications.
When these two technologies are combined, they can create robust reporting solutions. However, developers frequently encounter challenges unique to this integration.
Common Challenges
1. Report Execution in the JSF Lifecycle
One of the most significant challenges when using JasperReports within a JSF application is executing reports smoothly within the JSF lifecycle. The JSF lifecycle is intricate, comprising phases like restore view, apply request values, process validations, update model values, and render response.
Problem
Generating a report typically involves data retrieval and processing that need to occur during specific phases of the JSF lifecycle. If not managed correctly, it can lead to issues such as reports not displaying on the page, data not reflecting correctly, or even encountering state management problems.
2. Handling Report Parameters
Passing parameters to a JasperReport can confuse many developers, especially in a web environment where user input is often necessary. The first step is to ensure that the report parameters match what the report template expects.
Problem
Parameters may not be reaching the report accurately due to possible mismatches in name/value pairs or serialization issues.
3. Exporting Reports in Various Formats
Another challenge is exporting reports in different formats, like PDF or CSV. This can be problematic if you aim to provide users with options for downloading reports directly from the interface.
Problem
Without proper export handling, users can experience difficulties downloading reports, which reduces usability.
Solutions to Challenges
Setting Up Report Execution
To execute JasperReports in JSF effectively, you can define a method in your managed bean that's activated by a user action, such as a button click. Below is an example of how to integrate report generation within the JSF lifecycle effectively:
import net.sf.jasperreports.engine.*;
import net.sf.jasperreports.engine.data.JRBeanCollectionDataSource;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.ViewScoped;
import java.io.OutputStream;
import javax.faces.context.FacesContext;
@ManagedBean
@ViewScoped
public class ReportBean {
private List<YourDataType> dataList; // Collection of your data
private String reportPath = "/path/to/report.jasper";
public void generateReport() {
try {
// Load the Jasper report
JasperReport jasperReport = JasperCompileManager.compileReport(reportPath);
JRDataSource dataSource = new JRBeanCollectionDataSource(dataList);
// Parameter map
Map<String, Object> parameters = new HashMap<>();
parameters.put("ReportTitle", "Your Report Title");
// Fill the report
JasperPrint jasperPrint = JasperFillManager.fillReport(jasperReport, parameters, dataSource);
// Send the report to the client as a PDF
FacesContext facesContext = FacesContext.getCurrentInstance();
facesContext.responseComplete();
facesContext.getExternalContext().setResponseContentType("application/pdf");
OutputStream outputStream = facesContext.getExternalContext().getResponseOutputStream();
JasperExportManager.exportReportToPdfStream(jasperPrint, outputStream);
outputStream.flush();
} catch (Exception e) {
// Handle exceptions
e.printStackTrace();
}
}
}
Commentary
The above code showcases a basic method to generate a PDF report through JasperReports in JSF. The key steps include compiling the report, creating a data source, and filling the report.
Why? It's crucial to ensure that the report is compiled correctly and that the data source is prepared before invoking the fillReport
method. Establishing these steps guarantees that the report reflects accurate data when rendered.
Passing Parameters Cleanly
Handling report parameters in JSF should be straightforward. Here’s an example of how to pass parameters securely from a JSF page:
<h:form>
<h:inputText value="#{reportBean.someParameter}" />
<h:commandButton value="Generate Report" action="#{reportBean.generateReport}" />
</h:form>
In your bean, ensure to retrieve the parameters correctly:
parameters.put("ParameterName", someParameter);
Commentary
Using JSF components to gather parameters keeps the user interface responsive and simple. The parameters must match what JasperReports requires for seamless integration.
Why? Passing parameters directly from JSF components minimizes risk, ensuring user inputs remain sanitized and effectively sent to the report.
Implementing Export Functions
For user convenience, it is essential to provide options for exporting reports in various formats. Below is an example of exporting reports to different formats:
public void exportReport(String format) {
// Logic to generate report as shown above...
if (format.equals("pdf")) {
JasperExportManager.exportReportToPdfStream(jasperPrint, outputStream);
} else if (format.equals("csv")) {
JasperExportManager.exportReportToCsvStream(jasperPrint, outputStream);
}
// Additional formats can be added here...
}
Commentary
This method allows dynamic report export based on the user’s choice. The format parameter determines how JasperReports handles the output.
Why? Providing users with flexible export options enhances user experience while making your application more versatile and appealing.
Wrapping Up
Integrating JasperReports with JSF can present a unique set of challenges concerning report execution, parameter handling, and exporting capabilities. However, with the suggested solutions and implementation guidelines, overcoming these challenges becomes manageable.
By understanding the JSF lifecycle, effectively utilizing managed beans, and setting clear communication between the user interface and the reporting engine, developers can create dynamic, user-friendly reporting applications.
If you're interested in further enhancing your knowledge of JSF and report generation, consider visiting the official documentation for JasperReports. There, you can find more in-depth resources and examples to improve your reporting capabilities.
Happy coding!