Debugging Drools: Mastering Trace Output for Smooth Rules
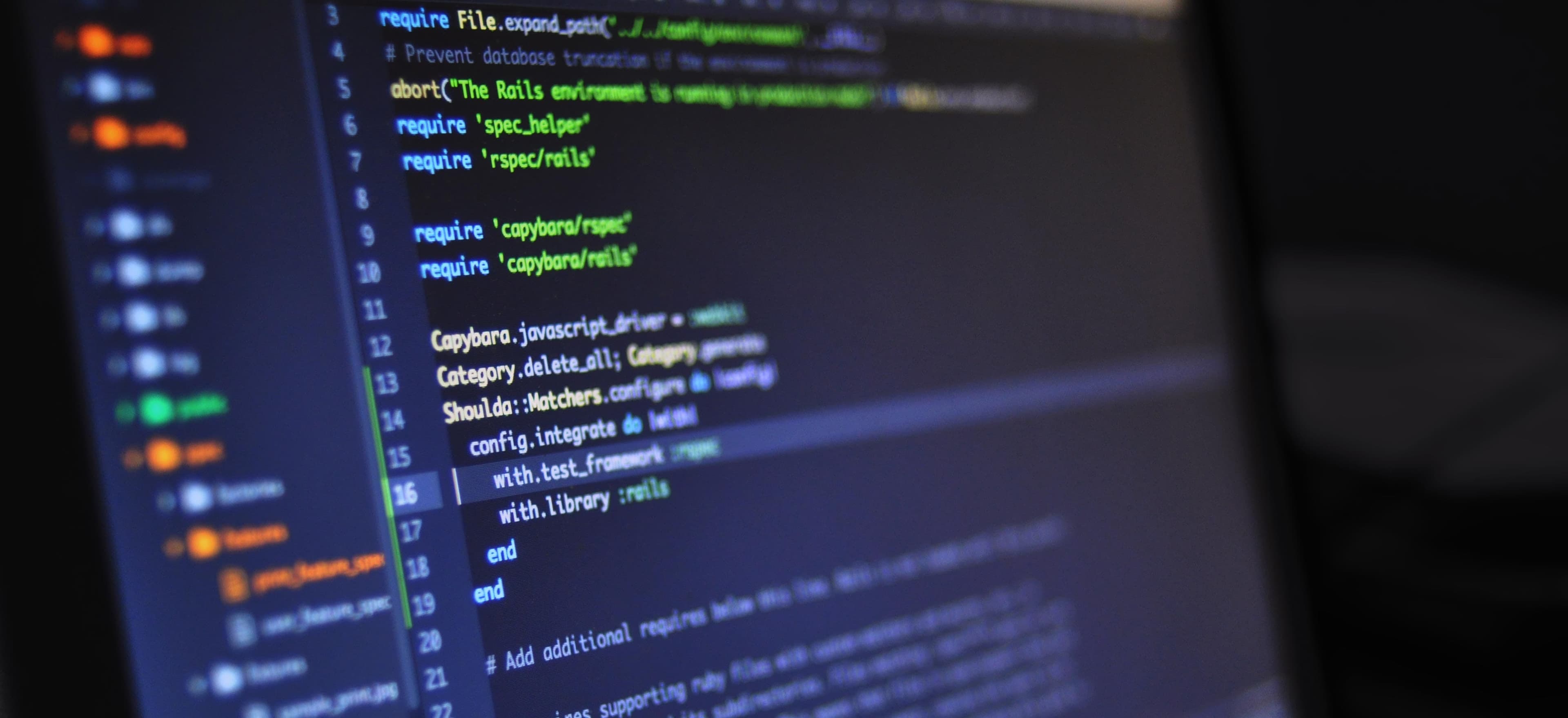
- Published on
Debugging Drools: Mastering Trace Output for Smooth Rules
In the world of enterprise application development, business rules management is crucial. One of the most powerful tools available for this is Drools, a business rules management system (BRMS) that provides a forward and backward chaining inference based rules engine. While Drools is robust and feature-rich, debugging can sometimes become the bane of developers' existence. In this blog post, we will dive deep into how to master trace output for smooth rule execution and debugging.
What is Drools?
Drools is an open-source project by JBoss that allows developers to define or execute business rules in a natural language format. The rules can have complex conditions and actions, making it easier for domain experts to understand and modify the rules without needing to comprehend intricate code.
Importance of Debugging
Debugging is a critical process in software development, particularly when dealing with complex rule engines like Drools. A missed condition or erroneous action can result in unintended behaviors, leading to malfunctions in business processes. Thus, it is essential to use the right tools and techniques for debugging.
Setting Up Trace Output
Drools provides a mechanism called "trace output" that helps in debugging by providing a step-by-step execution log of how rules are processed. Here's how to set up and use trace output for debugging:
1. Configuration
To enable trace output, you will need to configure the logging settings in your log4j.xml
or log4j.properties
configuration file. Here is an example of what that might look like:
<logger name="org.drools" level="DEBUG"/>
This will allow you to see detailed logging information for Drools, including rule activations, evaluations, and firings.
2. Writing Rules with Annotations
When writing rules, include practical annotations and comments. This provides context for anyone who reads the rule later on. It helps in collaboration and simplifies debugging. For example, consider the rule below:
rule "Discount for Premium Customers"
when
customer: Customer(type == "Premium")
then
customer.setDiscount(0.20);
System.out.println("Applied 20% discount for Premium customer: " + customer.getName());
end
Why This Code?
In this sample, we check if a customer is of type "Premium" and apply a discount accordingly. The log message helps track the execution flow during debugging.
3. Use the Knowledge Session API
You can utilize the Drools Knowledge Session API to listen for events and manage the rules execution context. This is where you enable and capture trace output:
KieSession kieSession = kieContainer.newKieSession();
kieSession.addEventListener(new DebugAgendaEventListener());
kieSession.addEventListener(new DebugWorkingMemoryEventListener());
Why This Code?
Adding a DebugAgendaEventListener
allows you to see events related to agenda manipulation, like when rules are activated. The DebugWorkingMemoryEventListener
provides insights on what facts are added or removed in the working memory.
4. Running the Kie Session
When you run your KieSession
, you can insert facts, fire rules, and observe the output.
kieSession.insert(new Customer("John Doe", "Premium"));
kieSession.fireAllRules();
Why This Code?
Inserting a customer fact and firing all rules will initiate the matching process in the Drools engine. It is essential to run this to identify if the rules are firing as expected.
Viewing Trace Output
The trace output you configured will provide detailed insights into the rule execution. You will be able to see:
- When rules are activated.
- The conditions that caused them to fire.
- Any actions that were executed.
Example Output
DEBUG: Rule Discount for Premium Customers activated
DEBUG: Applying discount for customer: John Doe
This output significantly aids in understanding the flow of execution, highlighting which rules were triggered and confirming whether the expected actions occurred.
Common Debugging Scenarios
Scenario 1: Rule Not Firing
Sometimes a rule may not fire as anticipated. This can occur due to a variety of reasons such as incorrect conditions or missing facts. To troubleshoot:
- Check log entries to ensure the conditions are satisfied.
- Verify that facts are correctly inserted into the session.
Scenario 2: Unexpected Behavior
If rules are firing, but the behavior isn’t what you expect, inspect the actions for any logical errors. This often involves:
- Reviewing the actions to ensure they’re implementing business logic correctly.
- Running through the debug output to audit the execution flow.
Advanced Topic: Dynamic Rule Changes
Drools supports dynamic rule changes, meaning you can re-evaluate and change rules while a session is running. This is powerful but can complicate debugging. To manage this:
- Keep your rules modular.
- Use clear naming conventions and documentation.
- Include trace logging to track when rules are added or removed.
The Closing Argument
Debugging in Drools doesn’t have to be daunting. By mastering the trace output and understanding how to effectively utilize logging and event listeners, you can enhance both your debugging skills and your ability to write smooth, functioning rules.
For further reading, consider visiting the Drools user guide and community forums for tips and tricks. Mastering Drools will not only improve your debugging skills but also enable you to design more robust business logic for your applications.
Happy debugging!
Checkout our other articles