Common Pitfalls When Using Base64 in Java 8
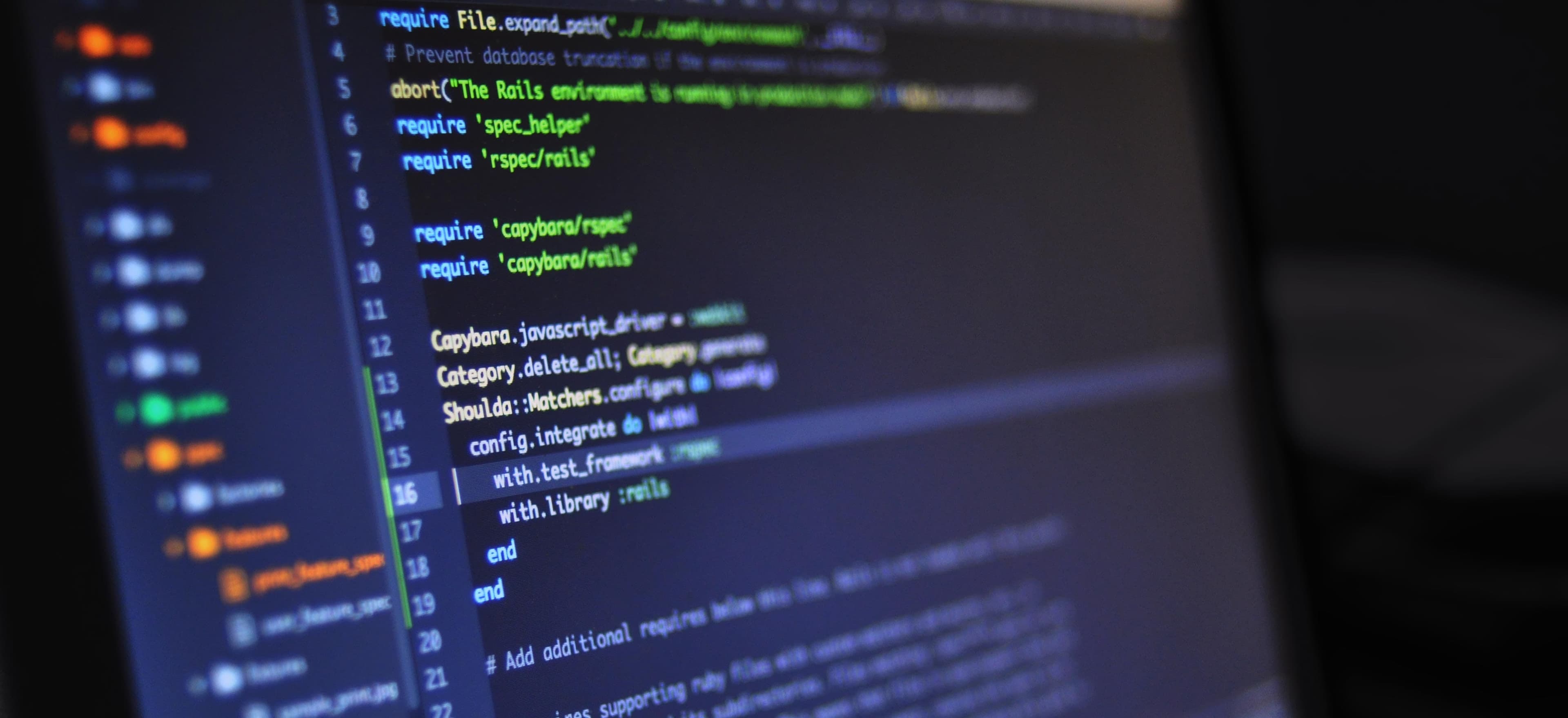
- Published on
Common Pitfalls When Using Base64 in Java 8
Base64 encoding is a method of converting data into a text format, making it easier to transmit over mediums that are designed to deal with text. Java 8 has included a robust implementation of Base64 encoding and decoding, but it's essential to understand the potential pitfalls involved when using it. In this blog post, we'll explore these pitfalls while providing clear code snippets and commentary to help you avoid common issues.
What is Base64 Encoding?
Base64 is an encoding scheme that represents binary data in an ASCII string format by translating it into a radix-64 output. It's commonly utilized in data transmission and storage, especially when binary data must be included in text-based formats such as XML or JSON.
Java 8 introduced a new Base64 class in the java.util
package, significantly simplifying the process of encoding and decoding Base64. Nonetheless, there are common mistakes developers encounter. Let’s dive into them.
1. Encoding and Decoding Data Inappropriately
One frequent pitfall is misunderstanding the distinction between encoding and decoding. Encoding converts raw binary data into a Base64 string, while decoding does the conversion in reverse.
Example:
import java.util.Base64;
public class Base64Example {
public static void main(String[] args) {
// Original data
String originalInput = "Hello World!";
// Encoding
String encodedString = Base64.getEncoder().encodeToString(originalInput.getBytes());
System.out.println("Encoded: " + encodedString);
// Decoding
byte[] decodedBytes = Base64.getDecoder().decode(encodedString);
String decodedString = new String(decodedBytes);
System.out.println("Decoded: " + decodedString);
}
}
Why This Is Important
Failing to appropriately encode or decode data can result in unexpected outputs and errors. If you attempt to decode something that wasn't properly encoded, you will receive a IllegalArgumentException
.
2. Ignoring Character Encoding
Character encoding can lead to additional bugs when working with Base64. The default character set may not always align with the bytes being encoded.
Example:
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class CharacterEncodingExample {
public static void main(String[] args) {
String originalInput = "Hello World!";
// Encoding with a specific character set
String encodedString = Base64.getEncoder()
.encodeToString(originalInput.getBytes(StandardCharsets.UTF_8));
System.out.println("Encoded: " + encodedString);
// Decoding
byte[] decodedBytes = Base64.getDecoder().decode(encodedString);
String decodedString = new String(decodedBytes, StandardCharsets.UTF_8);
System.out.println("Decoded: " + decodedString);
}
}
Why This Is Important
Ignoring character encoding can lead to garbled strings. Always specify the character set explicitly to avoid ambiguity and ensure that the original string is accurately reproduced after decoding.
3. Handling Large Data Sets
Sometimes Base64 encoding is applied to large files such as images or PDFs. It’s essential to be mindful of memory consumption, especially when handling large datasets.
Example:
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.nio.file.Files;
import java.util.Base64;
public class LargeFileEncoding {
public static void main(String[] args) {
try {
// Read the file into a byte array
File file = new File("largeFile.jpg");
byte[] fileContent = Files.readAllBytes(file.toPath());
// Encode the byte array into a Base64 string
String encodedString = Base64.getEncoder().encodeToString(fileContent);
System.out.println("Encoded Size: " + encodedString.length());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why This Is Important
Encoding large files can significantly increase memory usage. For large files, consider streaming the file contents instead of loading everything into memory at once. You can utilize Base64.Encoder
for continuous encoding of data chunks.
4. Incorrectly Handling Line Breaks
One often overlooked aspect is the treatment of line breaks when encoding data. The Base64 specification allows for wrapping lines at every 76 characters. While this is not mandatory, it may be expected in certain applications.
Example:
import java.util.Base64;
public class LineBreaksExample {
public static void main(String[] args) {
String originalInput = "This is a long string that exceeds the 76 characters "
+ "limit, so we need to check how Base64 encoding handles it.";
String encodedString = Base64.getMimeEncoder().encodeToString(originalInput.getBytes());
System.out.println("Encoded with line breaks:\n" + encodedString);
}
}
Why This Is Important
When transmitting Base64 data through certain mediums, such as email, wrapping may be expected. Using the Base64.getMimeEncoder()
method allows proper handling of line breaks.
5. Forgetting to Handle Exceptions
It is crucial to handle exceptions properly, especially during decoding. If the Base64 string is not valid, decoding will throw an IllegalArgumentException
.
Example:
import java.util.Base64;
public class ExceptionHandlingExample {
public static void main(String[] args) {
String malformedBase64 = "InvalidBase64String";
try {
Base64.getDecoder().decode(malformedBase64);
} catch (IllegalArgumentException e) {
System.out.println("Decoding failed: " + e.getMessage());
}
}
}
Why This Is Important
Failing to handle exceptions can lead to crashes or unexpected behavior in your application. Always encapsulate your Base64 operations in try-catch
blocks to maintain robustness.
A Final Look
Base64 encoding is a powerful tool in Java 8, but it comes with its set of challenges. By being aware of the common pitfalls outlined in this article, you can enhance your proficiency in using Base64 effectively.
For further reading, check the official Java documentation on Base64 and explore the various methods available for encoding and decoding operations.
Additionally, a good understanding of character encoding and the implications of data integrity will ensure your usage of Base64 enhances, rather than hinders, your applications. So the next time you find yourself dealing with Base64 in Java, remember this guide and avoid these common traps. Happy coding!
Checkout our other articles