How to Run Eclipse IDE with Multiple Java Versions
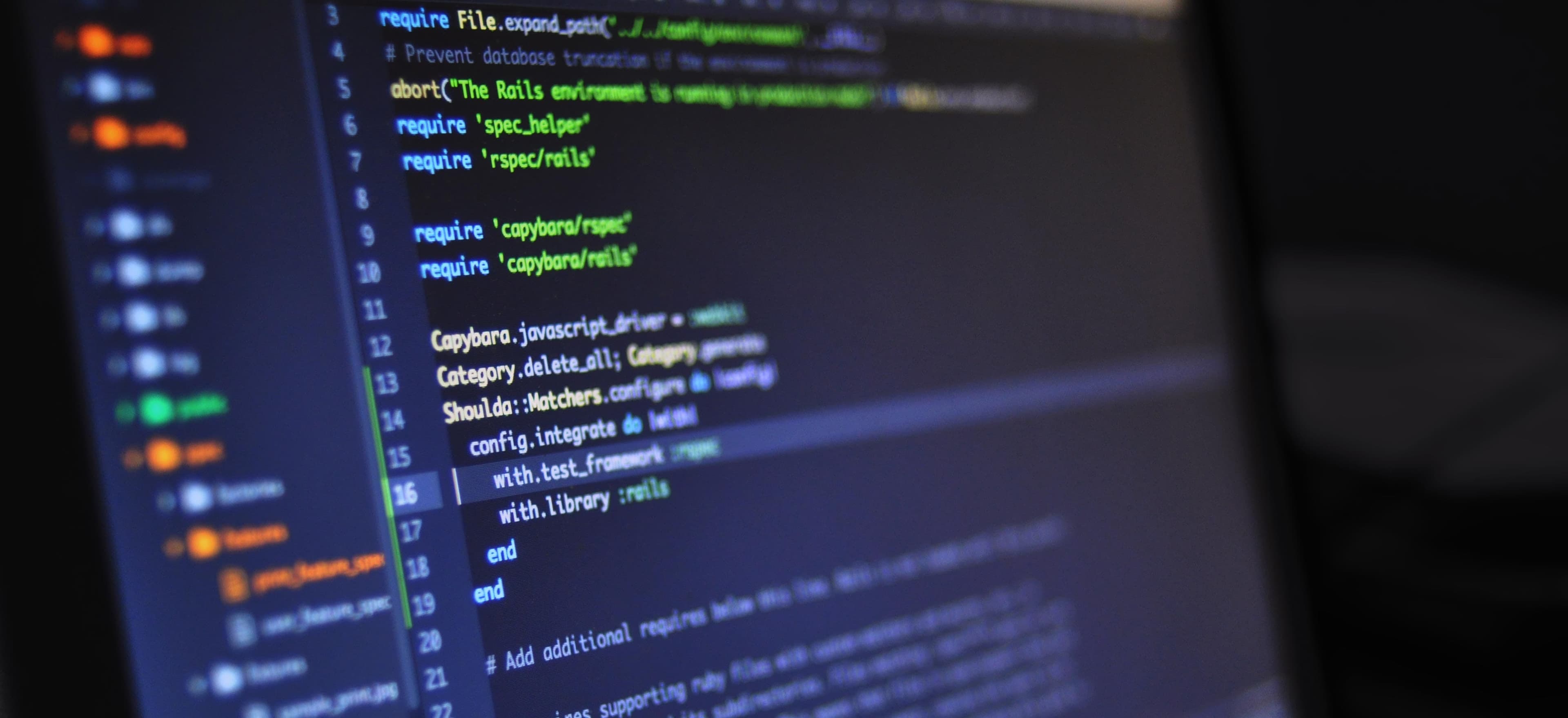
- Published on
How to Run Eclipse IDE with Multiple Java Versions
Java is one of the most widely-used programming languages in the world today. Developers often require different Java versions for different projects. Whether it's for compatibility reasons, new features, or specific library dependencies, using multiple versions makes good sense. Eclipse IDE provides a flexible way to manage various Java Development Kits (JDKs), enabling you to switch between them seamlessly. This blog post will walk you through the steps to configure Eclipse IDE to run with multiple Java versions.
Why Use Multiple Java Versions?
Before diving into the specifics, let’s discuss some reasons for using multiple Java versions:
- Project Compatibility: Some applications may rely on features found only in older versions.
- New Features: With continuous updates, newer Java versions often have valuable enhancements.
- Testing: It's crucial to test your Java application across various versions to ensure reliability and performance.
Prerequisites
You will need the following before you begin:
- Eclipse IDE installed on your system. You can download it here.
- Multiple Java versions installed on your machine. You can obtain the OpenJDK or Oracle JDK from their respective websites.
Step 1: Install Java Versions
First, ensure that you have the required Java versions installed. Use the command below to check your installed Java versions in a terminal or command prompt:
java -version
You can download the latest JDK releases from:
Install these JDK versions according to the provided instructions.
Step 2: Set Up Your Environment Variables
Setting your environment variables correctly is vital for Eclipse to recognize installed JDKs.
For Windows
- Press
Windows + R
, typesysdm.cpl
, and hit Enter. - Click on the Advanced tab and then on Environment Variables.
- In the System Variables section, find the
JAVA_HOME
variable. Modify it to point to the JDK folder you want to use.
For Linux/macOS
Edit your shell configuration file like .bashrc
, .bash_profile
, or .zshrc
and add:
export JAVA_HOME=/path/to/your/jdk
Make sure to refresh your terminal or run source ~/.bashrc
(or the appropriate file) afterwards.
Step 3: Configure Eclipse to Recognize Java Installations
Open Eclipse IDE, and follow these steps:
- Navigate to
Window
->Preferences
. - Expand the
Java
section and click onInstalled JREs
.
Adding a New JRE/JDK
- Click on Add -> Select Standard VM.
- Click Next.
- In the JRE home field, browse to your desired JDK installation folder (e.g.,
C:\Program Files\Java\jdk-11.0.10
). - Click Finish.
You can now see the added JRE in the list.
Step 4: Create a New Java Project
Now let's create a Java project and specify which Java version to use:
- Go to
File
->New
->Java Project
. - In the project dialog, name your project and select the appropriate JRE from the Use default location section.
This ensures your new project uses the Java version you selected.
Example of an Eclipse Project Setup
When creating a project, structure it well. Here's a simple structure example:
MyJavaProject
│
├── src
│ └── Main.java
└── lib
Sample Code Implementation in Main.java
public class Main {
public static void main(String[] args) {
System.out.println("Hello, Java World!");
}
}
This code simply prints a greeting message to the console.
Step 5: Switching Java Versions for Existing Projects
If you need to switch the Java version for an existing project:
- Right-click on your project in the Project Explorer.
- Go to
Properties
. - Click on
Java Build Path
. - Under the
Libraries
tab, remove the existing JRE and then click Add Library. - Select JRE System Library, then choose Workspace default JRE or Alternate JRE, and continue with the instructions.
Step 6: Clean and Build Your Project
Once you have selected the appropriate JDK, clean and rebuild your project to ensure that everything is functioning smoothly. You can do this by:
- Going to
Project
in the menu bar. - Selecting
Clean...
and rebuilding your project.
Useful Eclipse Plugins
Using plugins can enhance your experience in Eclipse. Here are a couple of recommendations:
- EclEmma: A code coverage tool that helps ensure your tests cover all necessary lines of code.
- Spring Tools 4: If you’re working with Spring projects, this plugin can make your life easier.
Debugging Across Different Java Versions
Debugging can differ across versions due to various enhancements or deprecated features.
Simple fixes for debugging issues:
- Ensure Compatibility: Make sure library dependencies are compatible with the Java version you are running.
- Check Compiler Compliance Level: In Project Properties -> Java Compiler, ensure the compliance level is set correctly.
Final Thoughts
Setting up multiple Java versions in Eclipse is straightforward, and it allows you to maintain compatibility with different project requirements. By following these steps, you can easily switch between Java versions, ensuring that your code runs correctly regardless of the JDK being used.
Now that you are equipped with the knowledge of configuring and managing multiple Java versions in Eclipse, you can tackle any project confidently. For more resources and to explore potential Java libraries and frameworks, check out the Oracle Java documentation or the Eclipse Community.
Happy coding!
Checkout our other articles