Troubleshooting Thread-Local Storage Issues in Java
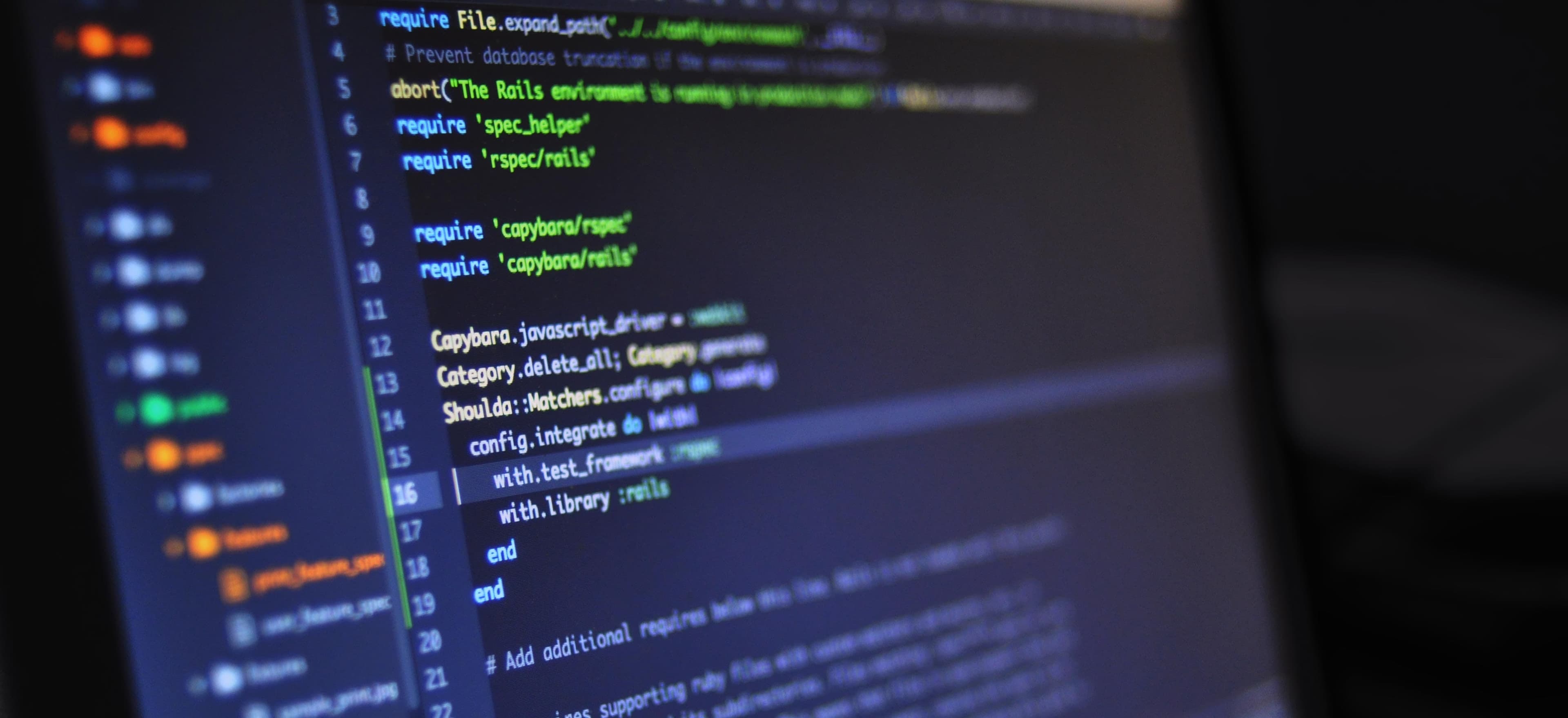
- Published on
Troubleshooting Thread-Local Storage Issues in Java
Thread-local storage is a useful feature in Java, enabling you to store data that is unique to a thread and can be accessed only by that thread. This becomes especially handy when dealing with multi-threaded applications, where data isolation is crucial for avoiding concurrency issues. However, even experienced developers encounter various problems associated with thread-local storage. In this article, we will explore common thread-local storage issues in Java and discuss effective troubleshooting techniques.
Understanding Thread-Local Storage
The ThreadLocal
class in Java allows you to create variables that are accessible by a thread but not by others. Each thread has its own value of the variable, which is initialized as necessary.
Here's a simple example:
public class ThreadLocalExample {
private static ThreadLocal<Integer> threadLocalValue = ThreadLocal.withInitial(() -> 0);
public static void main(String[] args) {
threadLocalValue.set(42);
System.out.println("Main thread value: " + threadLocalValue.get());
new Thread(() -> {
threadLocalValue.set(100);
System.out.println("New thread value: " + threadLocalValue.get());
}).start();
}
}
Code Commentary
- We initialize a
ThreadLocal<Integer>
variable. It starts with a default value of 0 for each thread. - When the main thread sets its value to 42, it retrieves it successfully.
- A new thread sets its own value to 100, showcasing thread isolation.
Common Issues with Thread-Local Storage
While ThreadLocal
can help manage data effectively, it also comes with its share of challenges:
- Memory Leaks: Keeping references to
ThreadLocal
variables may prevent their associated objects from being garbage collected. - Unexpected Null Values: If a thread hasn't initialized its
ThreadLocal
, accessing it may lead to confusion and null values. - Thread Not Reusing: The current thread might be performing work in different contexts, leading to inconsistent or unexpected values.
- ThreadLocal Inheritance: In certain Java designs, child threads do not inherit the parent thread's
ThreadLocal
values directly.
Troubleshooting Techniques
1. Memory Leaks
To avoid memory leaks, ensure that you properly remove ThreadLocal
values when they are no longer needed:
public class MemoryLeakExample {
private static ThreadLocal<Object> threadLocalVariable = ThreadLocal.withInitial(() -> new Object());
public static void doWork() {
Object value = threadLocalVariable.get();
// Perform some operations with the value
// Clean up to prevent memory leaks
threadLocalVariable.remove();
}
}
Why Use remove()
?
- The
remove()
method clears the value for the current thread, hence preventing potential memory leaks. - By clearing the associated data, you allow garbage collection to reclaim objects that are no longer in use.
2. Handling Unexpected Null Values
To handle scenarios where a thread might access a ThreadLocal
variable without initialization, always check for null or use default initialization:
public class NullValueHandling {
private static ThreadLocal<String> threadLocalValue = ThreadLocal.withInitial(() -> "Default Value");
public static void main(String[] args) {
// Not setting threadLocalValue
System.out.println("Thread-local output: " + threadLocalValue.get());
}
}
Why Is Default Initialization Important?
- By using
withInitial()
, you're providing a safe, default value that allows your application to work smoothly even if a value isn't set explicitly.
3. Ensuring Thread Reusability
In cases where a thread works on multiple tasks, it is crucial to encapsulate ThreadLocal
usage within particular task boundaries:
public class ThreadReusabilityExample {
private static ThreadLocal<Integer> threadLocalVariable = ThreadLocal.withInitial(() -> 0);
public static void task1() {
threadLocalVariable.set(10);
// Do work
}
public static void task2() {
threadLocalVariable.set(20);
// Do work
}
public static void main(String[] args) {
new Thread(() -> {
task1();
System.out.println("Task1 value: " + threadLocalVariable.get());
task2();
System.out.println("Task2 value: " + threadLocalVariable.get());
}).start();
}
}
Why Control Thread Locality?
- Properly managing thread locality prevents a collision of values that may lead to unexpected behavior during the execution of different tasks within the same thread.
4. ThreadLocal Inheritance
To share ThreadLocal
variables between parent and child threads, placeholder methods can be designed carefully or use Executors. To illustrate:
public class InheritanceExample {
private static ThreadLocal<String> threadLocalValue = new ThreadLocal<>();
public static void main(String[] args) {
threadLocalValue.set("Parent Value");
Thread childThread = new Thread(() -> {
// Direct access won't work; consider alternatives or custom context management
System.out.println("Child thread value: " + threadLocalValue.get());
});
childThread.start();
}
}
Solution for Inheritance Handling
Consider passing the values explicitly or using additional context management mechanisms (like a context holder class) to maintain the parent-child relationships properly.
Key Takeaways
Thread-local storage in Java offers valuable benefits, especially in multi-threaded applications. However, it also poses specific challenges, such as memory leaks, null-value access, task context management, and inheritance issues. By implementing proper cleanup, embracing default initialization, managing task boundaries, and managing thread relations diligently, we can mitigate these issues effectively.
For further exploration of Java concurrency and best practices, be sure to check out the official Java documentation and other resources for more in-depth knowledge. With just a little care and attention, you can leverage Java's ThreadLocal
mechanisms to build efficient and clean applications.