Understanding FutureTask's toString Method in JDK 10
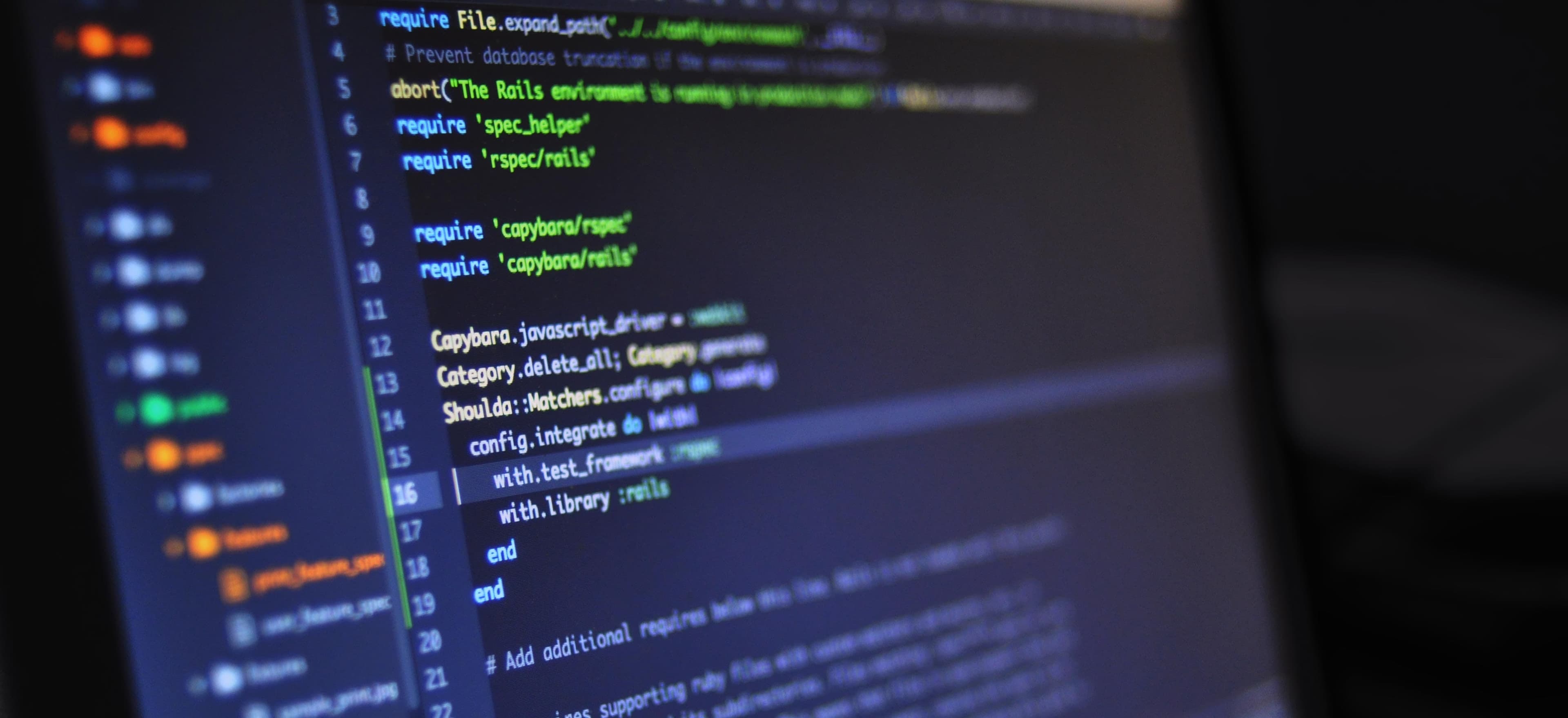
- Published on
Understanding FutureTask's toString Method in JDK 10
Java's concurrency framework has undergone significant evolution over the years, and one of the substantial components in this framework is the FutureTask
class. Introduced in Java 5, FutureTask
provides a mechanism for running tasks asynchronously while allowing you to manage their completion. In this blog post, we will delve into the intricacies of the toString
method in FutureTask
, particularly how it operates in JDK 10.
What is FutureTask?
FutureTask
is a concrete class that implements both the Runnable
and Future
interfaces. This duality makes it a powerful tool for asynchronous task execution.
Here's a quick overview of FutureTask
’s utility:
- Concurrency: It allows tasks to run in parallel with the main thread.
- Cancellation: You can cancel a task and check if it has been completed.
- Result Retrieval: It provides a mechanism to retrieve the result of an asynchronous computation.
Example of Basic FutureTask Usage
Before diving into toString
, let’s review a basic example to illustrate how FutureTask
is structured:
import java.util.concurrent.Callable;
import java.util.concurrent.FutureTask;
public class FutureTaskExample {
public static void main(String[] args) throws Exception {
Callable<String> task = () -> {
Thread.sleep(1000);
return "Task completed!";
};
FutureTask<String> futureTask = new FutureTask<>(task);
Thread thread = new Thread(futureTask);
thread.start();
String result = futureTask.get(); // This will block until the task is completed
System.out.println(result);
}
}
In this snippet, we define a Callable
task that returns a result after a one-second delay. By wrapping the Callable
in a FutureTask
, we can execute it on a separate thread, and we can retrieve the result once it's ready.
How FutureTask's toString Method Works
The toString
method in Java serves a critical role in providing useful information about the object states for debugging purposes. In the context of FutureTask
, it offers insights into the task status, which includes its last-known state and whether the computation is completed, cancelled, or running.
Why Override toString?
The toString
method is overridden to encapsulate vital task information succinctly. In JDK 10, the implementation of toString
returns a string representation of this task in a readable format.
Breakdown of FutureTask's toString Implementation
The implementation of toString
in FutureTask
is structured as follows:
@Override
public String toString() {
String status;
if (isDone()) {
status = (isCancelled() ? "Cancelled" : "Completed");
} else {
status = (isRunning() ? "Running" : "Not started");
}
return String.format("%s[status=%s]", getClass().getName(), status);
}
Commentary on the Code
-
isDone(): This method checks whether the task has finished executing. It returns true if the task has been completed or cancelled.
-
isCancelled(): Used to determine if the task was cancelled before it could complete.
-
isRunning(): This method checks if the task is currently being executed.
-
String Formatting: The use of
String.format
aids in creating a standard output format that includes the class name and the task status.
This method thus effectively presents the task's state in a user-friendly manner, which is crucial for debugging and logging purposes.
Example Usage of toString
Let us explore how toString
can be employed in practical scenarios to enhance our debugging capabilities:
import java.util.concurrent.Callable;
import java.util.concurrent.FutureTask;
public class FutureTaskToStringExample {
public static void main(String[] args) throws Exception {
Callable<String> task = () -> {
Thread.sleep(1000);
return "Hello from FutureTask!";
};
FutureTask<String> futureTask = new FutureTask<>(task);
System.out.println(futureTask); // Initially, it shows "Not started"
Thread thread = new Thread(futureTask);
System.out.println(futureTask); // Shows "Running"
thread.start();
while (!futureTask.isDone()) {
Thread.sleep(200); // Check status every 200ms
System.out.println(futureTask); // Keep printing current status
}
String result = futureTask.get();
System.out.println(result); // Outputs: Hello from FutureTask!
System.out.println(futureTask); // Finally shows "Completed"
}
}
Output Explanation
In this example, we log the status of the FutureTask
at various points during its lifecycle. Initially, it will show "Not started", switch to "Running" once the task begins executing, and, upon conclusion, it will indicate "Completed".
Implications for Developers
Understanding the toString
method’s operation in FutureTask
has practical implications:
- Debugging: It allows developers to easily track task status, promoting efficient debugging.
- Logging: Using proper logging mechanisms, integrating
toString()
provides real-time task lifecycle updates.
Final Considerations
In summary, the toString
method in Java’s FutureTask
class serves as a fundamental tool for developers, summarizing the current status of a task in a human-readable format. With the enhancements brought in JDK 10, it's now more important than ever for developers utilizing concurrency in Java applications to leverage this method for effective task monitoring and debugging.
For more detailed documentation, you can refer to the official Oracle Java Documentation.
Using FutureTask
effectively, along with understanding methods like toString
, can significantly improve the reliability and maintainability of concurrent applications. Whether you're a seasoned Java developer or a beginner, mastering these asynchronous concepts will elevate your coding proficiency. Happy coding!