Overcoming Data Bottlenecks in Spark Cluster Computing
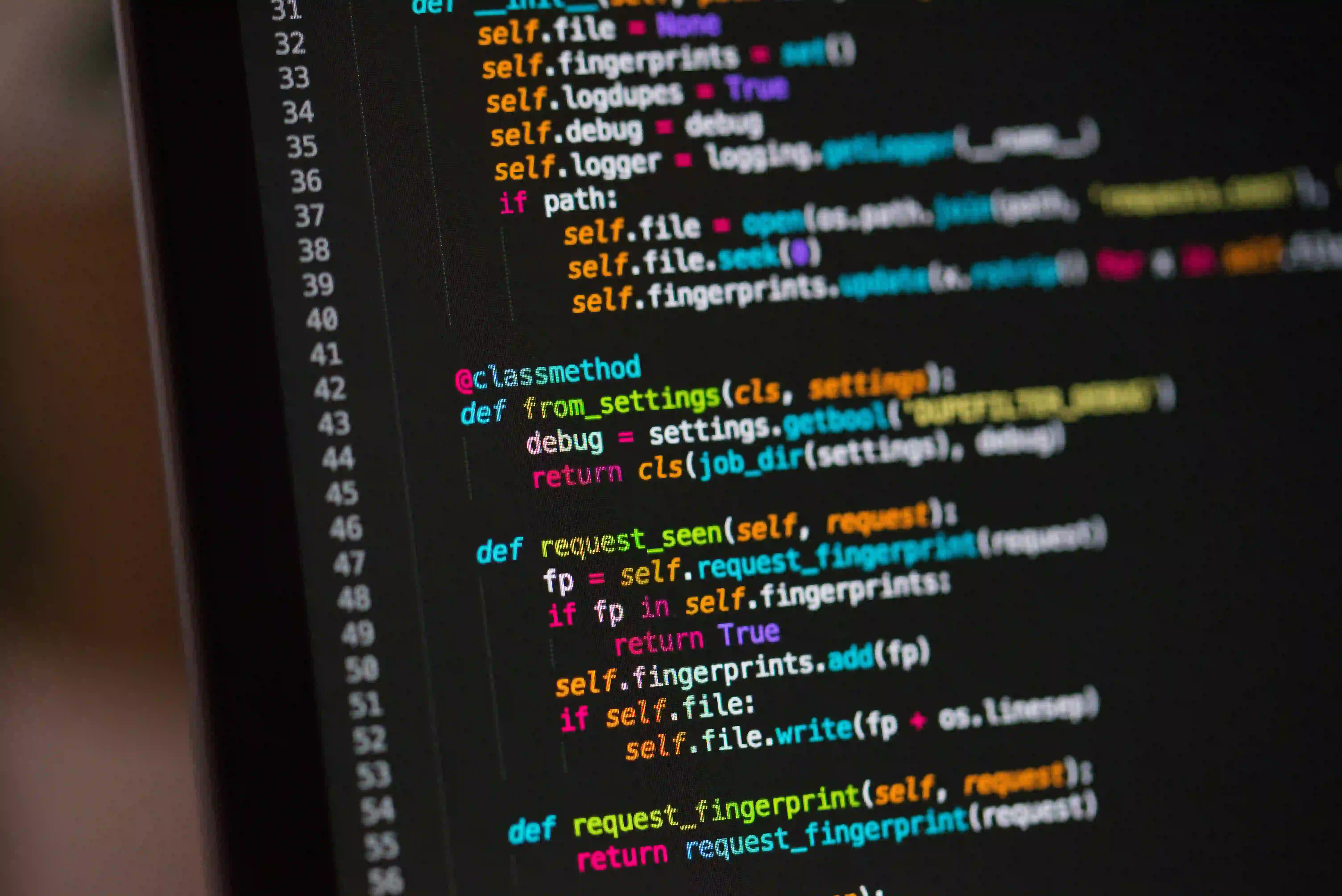
Overcoming Data Bottlenecks in Spark Cluster Computing
Apache Spark is a powerful open-source distributed computing system designed for processing large datasets quickly. However, like any system, it is susceptible to performance bottlenecks, especially relating to data movement and processing. Identifying, understanding, and overcoming these data bottlenecks is crucial for maintaining the efficiency of your Spark applications. This blog post delves into common causes of data bottlenecks and provides actionable strategies for overcoming them.
Understanding Data Bottlenecks
A data bottleneck occurs when the data transfer, processing, or storage components of a system slow down the overall performance. In the context of Spark, this can manifest in several ways:
-
Ineffective data shuffling: Data shuffling is one of the primary culprits in Spark. When data is redistributed across partitions during transformations (like
groupBy
andjoin
), it can cause network congestion and increase disk I/O operations. -
Skewed data distribution: Uneven data distribution across nodes can lead to some nodes being overloaded while others are underutilized, causing delays.
-
Insufficient resources: Insufficient memory or CPU resources can hinder performance, particularly in memory-intensive operations.
-
Poorly optimized transformations: Some Spark operations may inherently be more resource-intensive. Using inefficient data structures or algorithms can exacerbate data bottlenecks.
Analyzing Performance with Spark UI
Before implementing changes, it's important to identify where bottlenecks are occurring. Spark provides a built-in web UI that offers insights into job execution. You can analyze stages of your job, the tasks associated with each stage, and levels of task parallelism.
To access the Spark UI, navigate to http://<spark-master-node>:4040
. Here, you can examine:
- Job durations and task times
- Shuffle read/write metrics
- Task distribution across executors
Strategies to Overcome Data Bottlenecks
1. Optimize Data Serialization
Serialization is crucial as it determines how data is converted to a format suitable for storage or transmission. Spark supports two serialization libraries: Java serialization and Kryo serialization.
Why Kryo? Kryo is faster and results in smaller payloads than Java serialization. Here’s how to enable Kryo serialization in your Spark application:
import org.apache.spark.SparkConf;
import org.apache.spark.api.java.JavaSparkContext;
// Setting up the spark configuration
SparkConf conf = new SparkConf()
.setAppName("KryoSerializationExample")
.set("spark.serializer", "org.apache.spark.serializer.KryoSerializer");
JavaSparkContext sc = new JavaSparkContext(conf);
2. Partitioning Data Efficiently
Partitioning your data effectively reduces the amount of data shuffled across the network. Use repartition
and coalesce
functions thoughtfully.
Repartition Example:
JavaRDD<String> data = sc.textFile("input.txt");
JavaRDD<String> repartitionedData = data.repartition(10); // Increase to 10 partitions
Why is this important? More partitions allow Spark to perform tasks concurrently, although you must balance between too many partitions (increased overhead) and too few (inefficient resource usage).
3. Avoiding Data Skew
Data skew occurs when some partitions hold significantly more data than others. You can detect skew by examining task execution times through Spark UI and noticing disparities.
To mitigate data skew, consider the following techniques:
-
Salting: Adding a "salt" value to the key can distribute the data more evenly across partitions.
-
Using Approximate Algorithms: For operations like counting unique items, approximate algorithms like HyperLogLog can reduce the data sent for processing.
4. Using Broadcast Variables
When working with large datasets that are frequently used in operations, broadcasting can minimize data transfer across nodes.
Broadcasting a dataset ensures that each executor has a copy of the data in memory, eliminating the need to transfer data over the network repeatedly.
Broadcasting Example:
Map<String, Integer> locations = new HashMap<>();
locations.put("NY", 1);
locations.put("CA", 2);
JavaSparkContext sc = new JavaSparkContext(...);
Broadcast<Map<String, Integer>> broadcastLocations = sc.broadcast(locations);
// Using broadcasted variable
JavaRDD<Data> dataWithLocations = data.map(row -> {
Integer locationId = broadcastLocations.value().get(row.getLocation());
// additional processing...
});
5. Tuning Memory Management
Memory overhead can lead to performance bottlenecks. Adjusting Spark's memory configurations can alleviate pressure on the application’s execution.
Some configurations to consider:
spark.executor.memory=4g
spark.driver.memory=2g
spark.memory.fraction=0.6
- spark.executor.memory: Amount of memory to use per executor process.
- spark.driver.memory: Amount of memory to use for the driver process.
- spark.memory.fraction: Fraction of (heap space) used for execution and storage.
6. Optimize Data Locality
Data locality refers to the location of data relative to the computation performed on it. Optimizing your data locality means minimizing the need for data to be shuffled across the network.
-
Use HDFS or S3: Use a distributed storage system so that data is more likely to be present on the same nodes processing it.
-
Leverage Cache: Frequently accessed datasets can be cached in memory using
persist()
, drastically reducing the time spent in future computations.
JavaRDD<String> cachedData = data.cache(); // Cache the RDD
7. Effective Use of Aggregations and Joins
Join operations can be expensive, especially if not optimized. Consider:
-
Broadcast Joins: When one dataset is small enough to fit in memory, broadcast it to avoid shuffling the larger dataset.
-
Reduce the Datasets Before Joins: Perform filter operations on datasets before joining them.
The Closing Argument
By understanding the various aspects of data bottlenecks in Apache Spark and adopting the techniques discussed, you can significantly enhance the performance of your Spark applications. From optimizing data serialization to leveraging broadcast variables, a comprehensive approach will not only improve your current Spark jobs but will also equip you to handle future large-scale data challenges.
For more information on specific techniques, take a look at the official Apache Spark documentation for in-depth topics and performance tuning guidelines.
Happy Sparking!