Struggling with 2FA Implementation in Spring Boot?
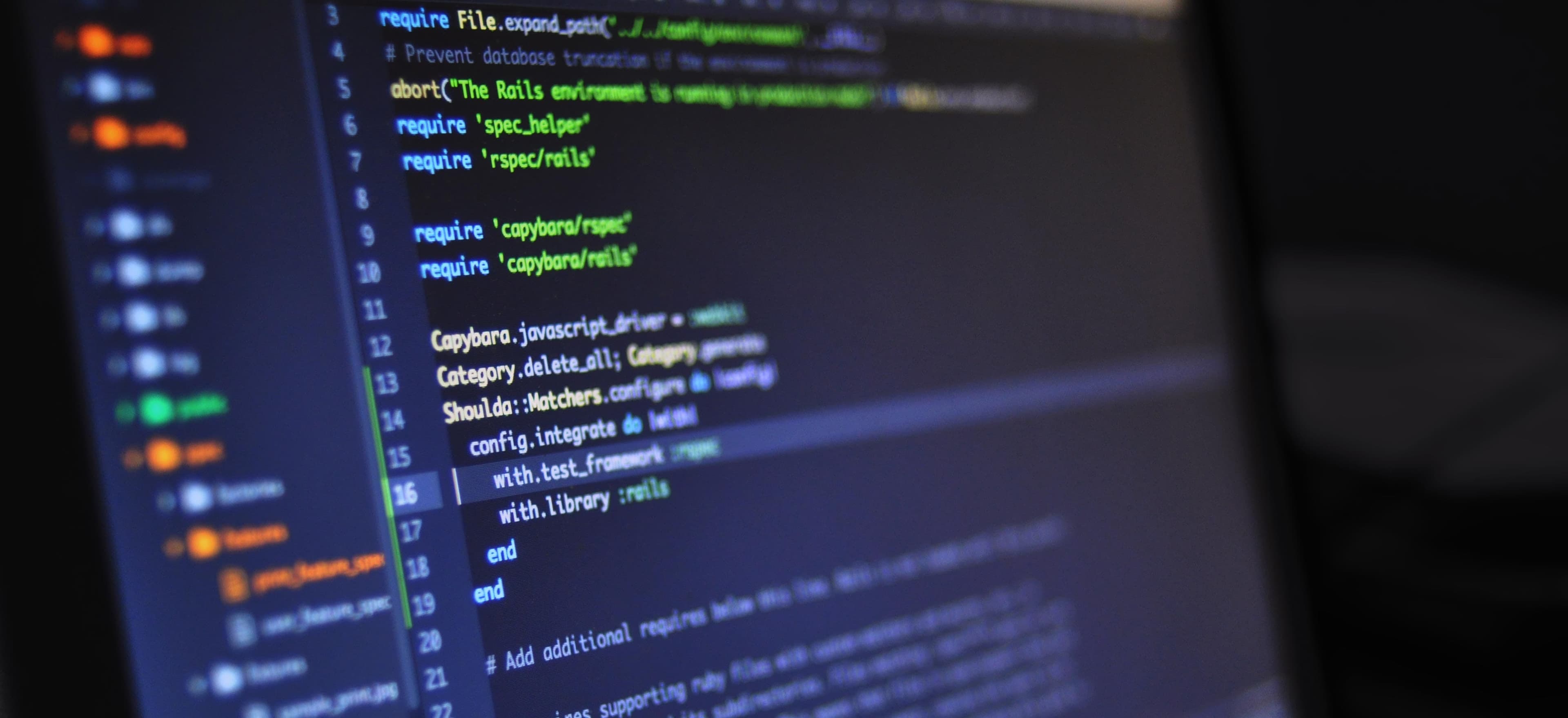
- Published on
Struggling with 2FA Implementation in Spring Boot? Here's Your Ultimate Guide!
Two-Factor Authentication (2FA) has emerged as a cornerstone of secure applications. Whether you're building an enterprise-level system or a small web app, incorporating 2FA can significantly enhance your security posture. This blog post will help you navigate the complexity of implementing 2FA in Spring Boot, enabling your application to not just protect user data, but also instill trust among your users.
What is Two-Factor Authentication?
Two-Factor Authentication adds an additional layer of security beyond just a simple password. It requires two forms of identification from the user — something they know (like a password) and something they have (like a mobile device). This approach greatly reduces the risk of unauthorized access, even if the password is compromised.
Why You Should Implement 2FA
-
Enhanced Security: A password alone is not enough. 2FA ensures that even if an attacker manages to steal a password, they cannot access your account without the second factor.
-
User Trust: Users increasingly expect secure interactions. Implementing 2FA demonstrates your commitment to protecting their data.
-
Regulatory Compliance: Many industries are moving towards stricter regulations around data protection. Implementing 2FA can help ensure compliance with these regulations.
Prerequisites for 2FA in Spring Boot
Before diving into the code, ensure you have the following prerequisites:
- A Spring Boot project set up.
- Basic knowledge of Spring Security.
- An understanding of RESTful web services.
If you haven’t set up a Spring Boot project, check out the official Spring Boot documentation for a straightforward guide on getting started.
The Overview of 2FA Implementation
To implement 2FA in Spring Boot, we will:
- Create a login endpoint.
- Generate a Time-based One-Time Password (TOTP).
- Send the TOTP to the user’s mobile device.
- Verify the TOTP on the server-side.
Step-by-Step Implementation of 2FA
Step 1: Setting Up Your Dependencies
Ensure that your pom.xml
file includes the necessary dependencies for Spring Security and TOTP generation. For example:
<dependencies>
<!-- Spring Boot Starter Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Security -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<!-- Java TOTP -->
<dependency>
<groupId>com.warrenstrange</groupId>
<artifactId>googleauth</artifactId>
<version>1.6.0</version>
</dependency>
</dependencies>
Step 2: Creating User Model and Repository
Create a User
class to represent user data and a UserRepository
interface to manage user data.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
private String secret; // for TOTP generation
// getters and setters
}
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByUsername(String username);
}
Why use these constructs? The
User
entity holds the user data, including the TOTP secret, which will be crucial for our authentication mechanism.
Step 3: Implementing TOTP Generation
To generate TOTP codes, add a service to manage TOTP creation and verification.
import com.warrenstrange.googleauth.GoogleAuthenticator;
@Service
public class UserService {
private final UserRepository userRepository;
private final GoogleAuthenticator gAuth = new GoogleAuthenticator();
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public String createUser(String username, String password) {
User user = new User();
user.setUsername(username);
user.setPassword(password); // Remember to hash the password!
user.setSecret(gAuth.createCredentials(username).getKey());
userRepository.save(user);
return user.getSecret(); // Return the secret for the user to configure their authenticator app
}
public boolean validateTOTP(String secret, int code) {
return gAuth.getTotpPassword(secret) == code;
}
}
What's important here? The
createUser
method generates a unique secret for each user, which needs to be shared with the user for them to configure their TOTP app (like Google Authenticator). ThevalidateTOTP
method checks the TOTP code entered by the user against the generated code.
Step 4: Creating a Login Controller
Next, create a REST controller that allows users to log in and verify their TOTP.
@RestController
@RequestMapping("/auth")
public class AuthController {
private final UserService userService;
public AuthController(UserService userService) {
this.userService = userService;
}
@PostMapping("/login")
public ResponseEntity<String> login(@RequestBody LoginRequest loginRequest) {
Optional<User> userOpt = userService.findByUsername(loginRequest.getUsername());
if (userOpt.isPresent() && passwordMatches(loginRequest.getPassword(), userOpt.get().getPassword())) {
return ResponseEntity.ok("Please enter your TOTP code");
}
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).body("Invalid credentials");
}
@PostMapping("/verify")
public ResponseEntity<String> verify(@RequestBody VerificationRequest verificationRequest) {
User user = userService.findByUsername(verificationRequest.getUsername()).orElse(null);
if (user != null && userService.validateTOTP(user.getSecret(), verificationRequest.getCode())) {
return ResponseEntity.ok("Login successful!");
}
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).body("Invalid TOTP code");
}
}
Step 5: Testing Your Implementation
Once you've implemented the code, deploy your application and make a request to the /auth/login
endpoint. If successful, prompt the user to enter a TOTP code. Upon receiving the TOTP, call the /auth/verify
endpoint to confirm the user's identity.
Here’s a sample of what your login request might look like:
{
"username": "testuser",
"password": "password123"
}
And the TOTP verification request:
{
"username": "testuser",
"code": 123456
}
Final Thoughts
The implementation of Two-Factor Authentication in Spring Boot is not just a technical requirement; it's a necessity in today's digital landscape. By following the aforementioned steps, you can significantly enhance the security of your application.
The above implementation is a basic version. In a production scenario, consider:
- Hashing user passwords securely.
- Using a proper shared secret sharing mechanism.
- Implementing rate limiting and lockout mechanisms for repeated failed login attempts.
For more in-depth guidance, check out Spring Security documentation and two-factor authentication best practices.
By incorporating these enhancements, you will not only secure your application but also provide peace of mind to your users. Happy coding!