How to Efficiently Get Time in Milliseconds in Java
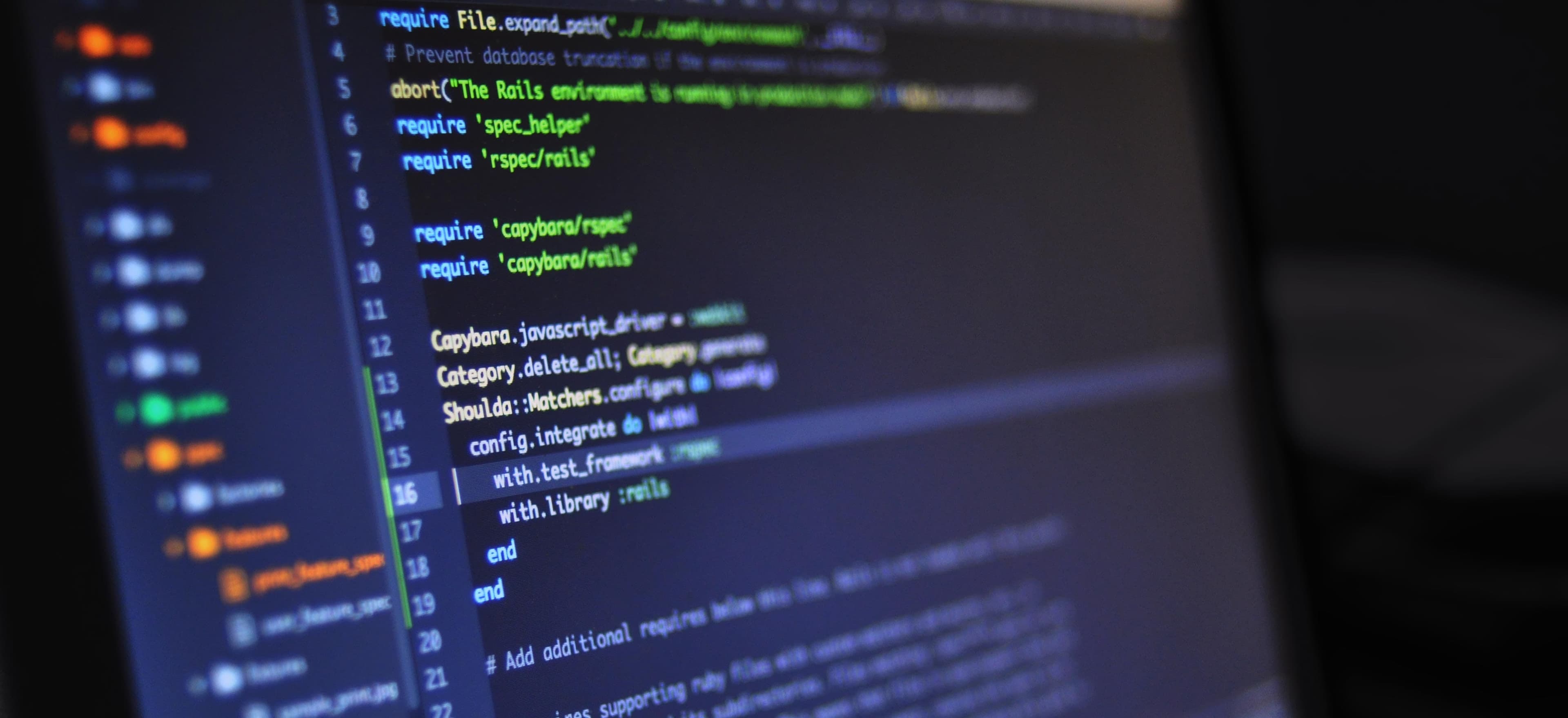
- Published on
How to Efficiently Get Time in Milliseconds in Java
In today's fast-paced world, time management is essential. Whether you are building a web application, handling transactions, or creating animations, knowing how to measure time accurately in Java is crucial. In this blog post, we will explore the ways to get the current time in milliseconds in Java efficiently and understand the nuances involved.
Why Milliseconds are Important
Milliseconds are a standard way to measure time when it comes to performance, precision, and timing. For instance, measuring the time taken for a piece of code to execute helps developers identify bottlenecks. Notably, many systems and APIs require timestamps in milliseconds since the epoch (January 1, 1970, 00:00:00 GMT).
Java Time APIs Overview
Java has several built-in classes that can help you get the current time in milliseconds. The following are the most commonly used ones:
- System.currentTimeMillis()
- Instant.now() (from Java 8 onwards)
Let’s dissect each method and explore the best practices for using them.
Using System.currentTimeMillis()
The simplest and most widely used method to obtain the current time in milliseconds is System.currentTimeMillis()
. Here is a quick example:
public class TimeExample {
public static void main(String[] args) {
long currentTimeInMillis = System.currentTimeMillis();
System.out.println("Current time in milliseconds: " + currentTimeInMillis);
}
}
Commentary on System.currentTimeMillis()
The System.currentTimeMillis()
method retrieves the current time from the system clock in milliseconds since the epoch. It's fast and works seamlessly for basic use cases. However, it’s important to note two limitations:
-
Precision: While it returns milliseconds, the underlying clock may not have that level of granularity. On certain systems, the resolution may vary significantly.
-
Time Zone Issues: Because it provides time based on the system clock, it may be affected by changes in the clock settings or system time updates.
Using Instant.now()
With the introduction of the Date and Time API in Java 8, using Instant.now()
became a more robust alternative. Here is how you can use it:
import java.time.Instant;
public class TimeExample {
public static void main(String[] args) {
long currentTimeInMillis = Instant.now().toEpochMilli();
System.out.println("Current time in milliseconds (Instant): " + currentTimeInMillis);
}
}
Commentary on Instant.now()
The Instant
class provides a more granular and immutable representation of a point in time. A few benefits include:
-
Immutability: An instance of
Instant
cannot be modified, reducing the chance of accidental changes. -
Use of Clocks: The
Instant
API can be used with different clocks, enabling more precise time measurements. -
Compatible with Other APIs: It integrates nicely with Java’s newer frameworks and libraries.
Performance Considerations
Both methods are efficient, but Instant.now()
may incur slight overhead due to its comprehensive functionality. However, for most applications, this is negligible.
Example: Measuring Execution Time
Measuring execution time is a common requirement in performance tuning. Let’s put both methods to the test:
public class ExecutionTimeExample {
public static void main(String[] args) {
long startTime = System.currentTimeMillis();
// Simulate a task
performTask();
long endTime = System.currentTimeMillis();
System.out.println("Execution time in milliseconds: " + (endTime - startTime));
}
public static void performTask() {
try {
Thread.sleep(200); // Simulating a task taking 200 milliseconds
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
In the above example, we simulate a task that sleeps for 200 milliseconds. We capture the start and end time using System.currentTimeMillis()
to measure the execution duration accurately.
For more accurate measurement with a higher resolution, you can consider using Instant
as shown below:
import java.time.Instant;
public class ExecutionTimeExample {
public static void main(String[] args) {
Instant startTime = Instant.now();
// Simulate a task
performTask();
Instant endTime = Instant.now();
long executionTimeInMillis = endTime.toEpochMilli() - startTime.toEpochMilli();
System.out.println("Execution time in milliseconds (Instant): " + executionTimeInMillis);
}
public static void performTask() {
try {
Thread.sleep(200);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
When to Use Which Method
Given the various scenarios, here are some guidelines to decide which method to use:
-
Quick Timestamps: If you’re just logging time or doing simple computations, use
System.currentTimeMillis()
. -
Precise Timing: For performance benchmarking, prefer
Instant.now()
due to its accuracy and the compatibility it offers with other date/time APIs. -
Timezone Aware Applications: If your application requires timezone management, consider working with
ZonedDateTime
along withInstant
for more comprehensive handling.
The Last Word
Understanding how to efficiently obtain time in milliseconds in Java is vital for developers. Whether you choose System.currentTimeMillis()
for its simplicity or Instant.now()
for its robustness, both methods have their place in Java development.
Armed with this knowledge, you can monitor performance, measure execution times, and make informed decisions on your Java applications.
For further reading on the Java Time API and its capabilities, check out the official documentation here and explore more examples related to performance measurement on the Baeldung blog.
By mastering time management in Java, you position yourself to create more efficient and responsive applications while gaining deeper insights into their performance metrics. Happy coding!