Common Garbage Collection Issues in Java and How to Fix Them
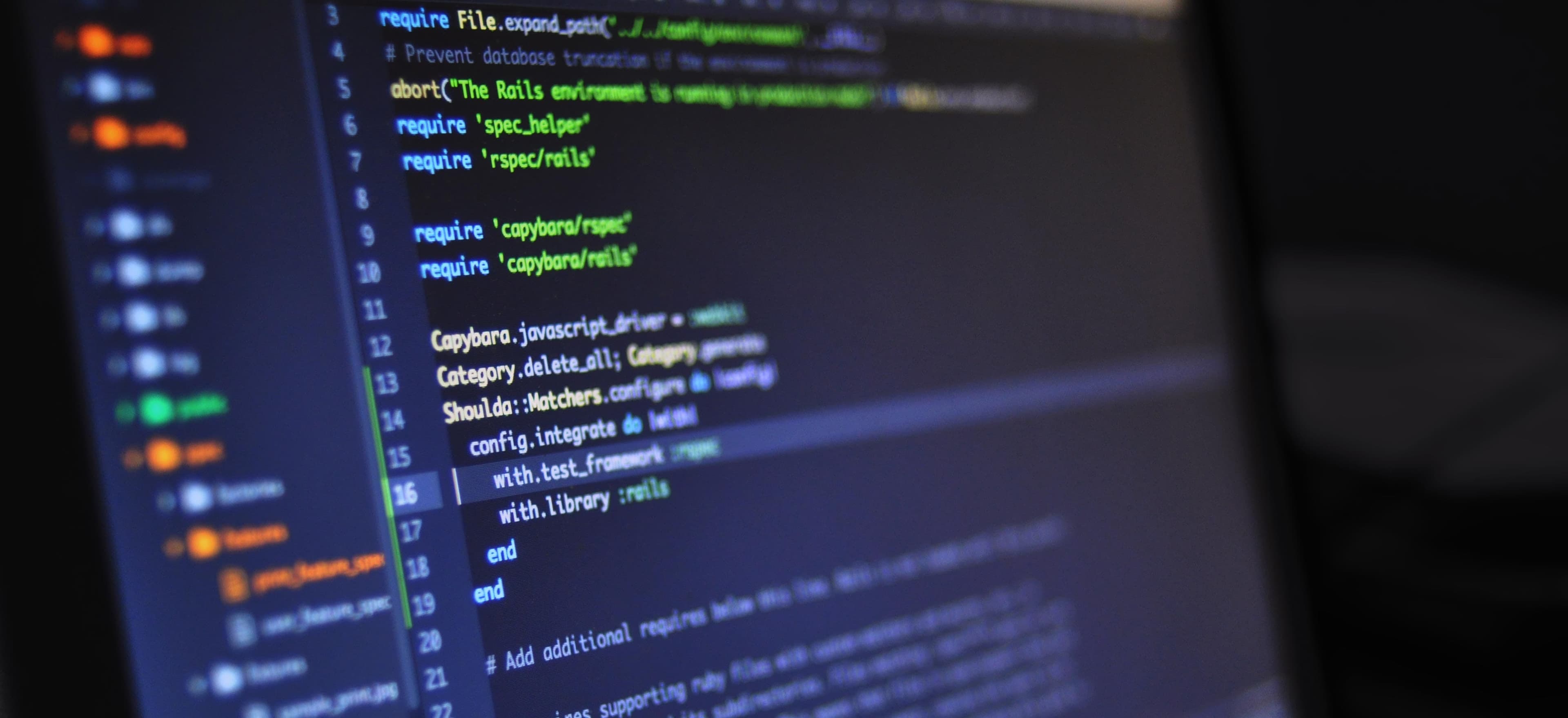
- Published on
Common Garbage Collection Issues in Java and How to Fix Them
Garbage Collection (GC) is an essential feature of Java that manages memory automatically, allowing developers to focus on writing code rather than manually allocating and deallocating memory. However, despite its benefits, garbage collection can lead to performance bottlenecks and various issues if not understood and managed properly. In this blog post, we will explore some common garbage collection problems in Java and how to tackle them effectively.
What is Garbage Collection?
Garbage Collection is the process by which the Java Virtual Machine (JVM) automatically identifies and disposes of objects that are no longer in use, freeing up memory resources. The key advantage of GC is that it reduces memory leaks and improves application stability. However, it comes with its set of challenges, which we will address below.
Common Garbage Collection Issues
1. Long Garbage Collection Pauses
Long GC pauses can significantly affect application performance, leading to slow response times and poor user experience. The main culprits of long pauses include:
- Large Heap Size: The larger the heap, the longer the time taken for garbage collection.
- Wrong GC Algorithm: Using an inappropriate garbage collection algorithm for the application’s needs.
Example Scenario
Imagine an application that holds a large amount of data in memory. The JVM has to traverse this data to identify unreachable objects, which can cause lengthy stops.
Fix
To address this issue, you can:
- Tune Heap Size: Set the minimum and maximum heap size appropriately by using JVM parameters
-Xms
and-Xmx
. Here’s an example:
java -Xms512m -Xmx2048m -jar YourApp.jar
This allocates a minimum of 512 MB and a maximum of 2048 MB of heap space.
- Choose the Right GC: Depending on your application needs (e.g., low-latency systems may benefit from the G1 Garbage Collector), you can specify the garbage collector in your JVM options:
java -XX:+UseG1GC -jar YourApp.jar
2. Memory Leaks
Memory leaks occur when objects that are no longer needed are still referenced, preventing the garbage collector from reclaiming memory. This is a common issue in applications that use collections, listeners, or other resources not properly released.
Example Scenario
Consider a situation where an application uses event listeners but never removes them when they are no longer needed. This situation will lead to memory leaks, as the listeners are still holding references to the objects they "listen" to.
Fix
To avoid memory leaks, always ensure proper cleanup of resources:
- Use Weak References: Might be helpful when dealing with listeners or caches. Here’s an example using
WeakHashMap
:
import java.util.WeakHashMap;
public class WeakReferenceExample {
private WeakHashMap<Key, Value> cache = new WeakHashMap<>();
public void addToCache(Key key, Value value) {
cache.put(key, value);
}
}
In this case, when the Key
is no longer needed, it can be garbage collected, even if it exists in the cache
.
3. Frequent Full GCs
Full GCs can occur when the JVM deems its short-lived space too small to accommodate the allocations, subsequently forcing a collection of both young and old generations.
Example Scenario
In applications that have a high allocation rate, frequent full GCs can lead to performance degradation.
Fix
To minimize full GCs, consider:
- Properly Tuning JVM Parameters: Understanding the
-XX:NewRatio
parameter can help balance the young and old generations. Setting this parameter can ensure the young generation is adequately sized for your application's allocation rate.
java -XX:NewRatio=3 -jar YourApp.jar
This means your old generation will be three times larger than the young generation.
- Profiling and Analyzing Memory Usage: Use tools like Java VisualVM or JConsole to identify memory usage patterns and hotspots in your application.
4. Stop-the-World Events
Stop-the-World (STW) events are pauses during which all application threads are stopped for garbage collection. Frequent or lengthy STW events significantly affect user experience.
Example Scenario
Heavy applications that handle large transactions might cause significant delays during GC, disrupting end-user activity.
Fix
To alleviate STW issues, consider:
- Using Concurrent GC Algorithms: Modern GC algorithms like G1 and ZGC are designed to perform garbage collection more concurrently. Enable G1 GC:
java -XX:+UseG1GC -jar YourApp.jar
- Monitoring Application Behavior: Regularly monitor and profile your application to understand how GC impacts performance and adjust configurations accordingly.
5. Insufficient Memory
When memory is insufficient for your application's requirements, it leads to out-of-memory errors and frequent GC cycles.
Example Scenario
If your application receives a sudden spike in traffic, it may overwhelm the existing heap space.
Fix
- Increase Heap Size: As mentioned earlier, allocating more memory can help. For example:
java -Xmx4096m -jar YourApp.jar
This command increases your maximum heap space to 4096 MB.
- Perform Application Profiling: Tools such as Eclipse Memory Analyzer (MAT) can help identify memory consumption trends and allow targeted optimizations.
Lessons Learned
Garbage collection in Java is a powerful feature that can lead to performance improvements when properly managed. However, it also requires vigilance in tuning and understanding the behaviors of your application.
By addressing common garbage collection issues like long pauses, memory leaks, and frequent full GCs, you can create a more robust and responsive Java application. Regular profiling and monitoring can help you stay ahead of potential issues, ensuring a seamless user experience.
For more insights into Java memory management, consider checking out Oracle's Java documentation or Java Garbage Collection Basics.
Happy coding!