Unlocking Grails: Overcoming Raw Output Challenges
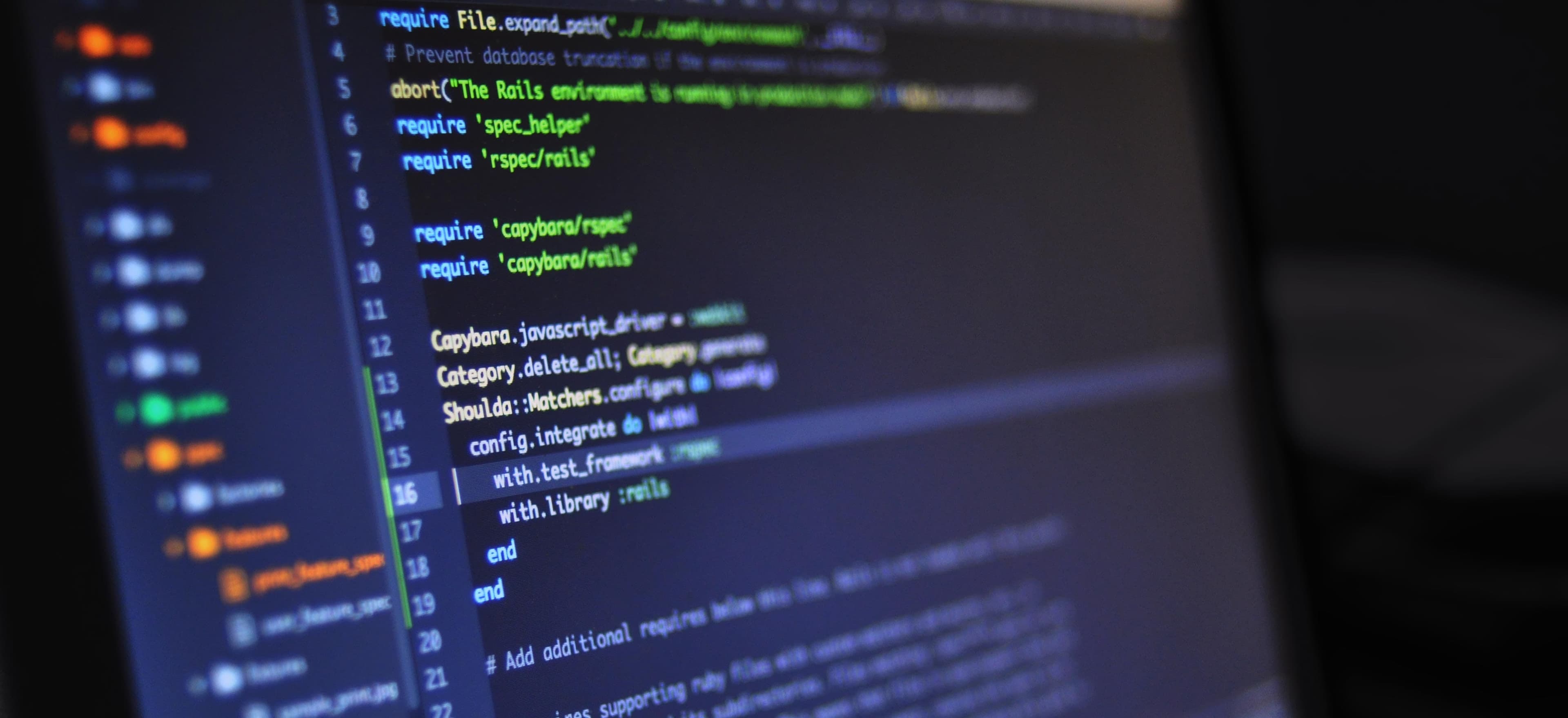
- Published on
Unlocking Grails: Overcoming Raw Output Challenges
Grails is a powerful web application framework which leverages the Groovy programming language and is built on top of the Spring framework. Designed for developer productivity, Grails allows you to build web applications quickly and efficiently. However, working with raw output can sometimes present challenges that require navigating through Grails' capabilities. In this blog post, we will explore these challenges and how to overcome them, ensuring your applications not only function but shine.
Understanding Raw Output in Grails
Raw output generally refers to data rendered directly to the HTTP response without any filtering or processing. While this may be useful in some cases, leaving output unfiltered can lead to security vulnerabilities such as Cross-Site Scripting (XSS) attacks, and can also make it harder to manage and maintain your application.
Here, we will discuss common scenarios where raw output issues arise, their implications, and how to mitigate these challenges using Grails’ built-in features.
Common Challenges with Raw Output
- Security Risks: Raw output can expose your application to potential security vulnerabilities. If user-generated content is rendered without proper escaping, it opens the door for malicious users to inject harmful scripts.
- Loss of Readability: Raw output can sometimes result in an unintelligible mix of HTML and JSON for users who expect clean presentations.
- Maintainability: Keeping a large codebase with raw output makes it cumbersome to manage updates or changes in design without breaking existing functionality.
How to Safely Handle Raw Output
Using GSP for Safe Rendering
Graphical Server Pages (GSP) is the view technology that Grails uses. It automatically escapes output, protecting against XSS attacks.
// Example of GSP rendering a user's name
<g:each in="${users}" var="user">
<p>${user.name}</p> <!-- This is automatically escaped -->
</g:each>
In the above example, ${user.name}
is automatically escaped, ensuring that if a user inputs a script tag in their name, it will be rendered safely as plain text. This is one of the many reasons GSP is a recommended choice for rendering output in Grails applications.
JSON Rendering
When dealing with JSON data, it’s essential to ensure that all data is appropriately serialized. Grails offers built-in methods for rendering JSON, which also handle escaping and data type coercion automatically.
// Example of JSON responses in a controller
def user() {
def user = User.findById(params.id)
render user as JSON // Handles escaping for safe JSON output
}
In this scenario, rendering the user
object as JSON ensures that any special characters are serialized correctly. This method is not only safe but provides a simple way to structure your data.
Implementing HTML Escaping Manually
There may be instances where you control the output and need to sanitize it based on specific use cases. For this, Grails offers many utilities.
import org.apache.commons.lang3.StringEscapeUtils
def userInput = "<script>alert('XSS')</script>"
def safeOutput = StringEscapeUtils.escapeHtml4(userInput)
println safeOutput // Prints: <script>alert('XSS')</script>
Here, we use StringEscapeUtils.escapeHtml4
from Apache Commons Lang to sanitize the input. This approach is effective when you need to provide HTML output but also maintain security standards.
Employing Filters
Another effective strategy to manage raw output and enhance security is to utilize Grails filters. Filters are a powerful way to intercept requests before they reach your controllers.
class SecurityFilters {
def filters = {
all(controller: '*', action: '*') {
before {
// Implement security checks here
}
}
}
}
In the example above, we create a security filter that applies to all actions and controllers. You can implement checks like validating user permissions or sanitizing input/output, providing an extra layer of security across your application.
Taking Advantage of Grails' JSON Views
Starting from Grails 3.x, the framework supports rendering JSON using gson
. This approach enables us to create finely-tuned JSON views without manually constructing JSON structures.
import grails.converters.JSON
def users() {
response.contentType = "application/json"
render User.list() as JSON
}
Using grails.converters.JSON
, we can streamline our JSON serialization, and the framework will handle the intricacies of escaping special characters and handling different data types for us.
Wrapping Up
Grails presents a robust platform for developing web applications, but overcoming the challenges associated with raw output is crucial for building secure, user-friendly, and maintainable applications. By leveraging the advantages of GSP for HTML rendering, utilizing filters, and embracing JSON views, you can ensure that your output remains safe while providing a rich user experience.
For further reading, consider exploring the following resources:
- Grails Documentation
- Grails Security
By adopting best practices and utilizing Grails effectively, you can unlock the full potential of the framework while protecting your applications and user data.
If you liked this article, feel free to share or leave a comment below with your thoughts or questions regarding Grails and raw output handling!