Overcoming Dependency Conflicts in Maven Builds
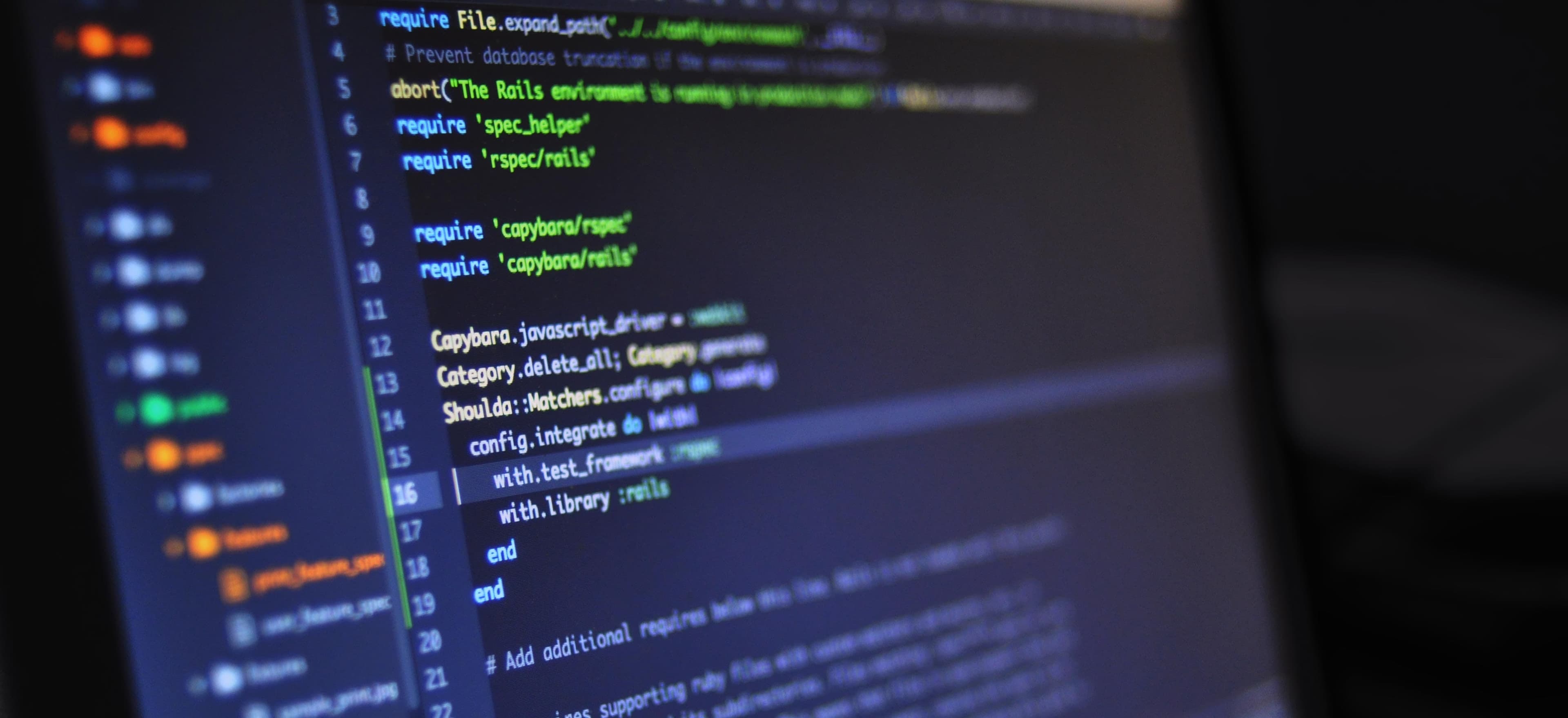
- Published on
Overcoming Dependency Conflicts in Maven Builds
Dependency management is a crucial component of Java development, especially when using a build automation tool like Maven. As projects grow in complexity and require various libraries, the potential for dependency conflicts increases. In this blog post, we will explore the mechanism of dependency conflicts in Maven, why they occur, and how to resolve them effectively.
What Are Dependency Conflicts?
A dependency conflict arises when two or more libraries in your project require different versions of the same dependency. For example, if Library A requires version 1.0 of a library, and Library B requires version 2.0, Maven must choose which version to include in the build. This situation typically leads to a "dependency hell" scenario where the application might break due to the missing features or classes in the chosen version.
Why Do Dependency Conflicts Occur?
There are several reasons why dependency conflicts occur in Maven:
- Transitive Dependencies: Libraries often depend on other libraries. If these dependencies have their own transitive dependencies, conflicts can arise.
- Differing Version Requirements: Different libraries or modules might explicitly declare varying version requirements for the same dependency.
- Poorly Managed Dependencies: Sometimes individual libraries do not manage their dependencies well, leading to conflicting versions.
Finding Dependency Conflicts
Before resolving conflicts, it is essential to identify them. Maven provides a helpful command to visualize dependencies:
mvn dependency:tree
This command generates a tree structure of the project's dependencies, making it easier to spot version conflicts.
Example Output
[INFO] +- org.example:libraryA:jar:1.0:compile
[INFO] | \- org.example:libraryB:jar:1.0:compile
[INFO] | \- com.google.guava:guava:jar:28.0-jre:compile
[INFO] \- org.example:libraryC:jar:2.0:compile
[INFO] \- com.google.guava:guava:jar:27.0.1-jre:compile
From the above output, you can see that libraryA
relies on Guava version 28.0-jre
, while libraryC
relies on 27.0.1-jre
.
Strategies to Overcome Dependency Conflicts
1. Dependency Exclusion
One way to resolve conflicts is by excluding specific transitive dependencies. This tactic can help you keep only the version of the library you want. Here’s how to exclude a dependency:
<dependency>
<groupId>org.example</groupId>
<artifactId>libraryB</artifactId>
<version>1.0</version>
<exclusions>
<exclusion>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
</exclusion>
</exclusions>
</dependency>
Why Use Exclusions?
Exclusions provide control over what gets included in your build. It allows you to retain the core functionality of the main library while removing dependencies that cause conflicts.
2. Declare a Dependency Version
If a project requires a specific version of a library, explicitly declaring that version in the <dependencies>
section can help resolve conflicts. Here’s an example:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>28.0-jre</version>
</dependency>
Why Declare Versions?
By explicitly defining the version you intend to use, you ensure that anyone who builds your project will use the same library versions. This consistency is crucial for avoiding runtime issues.
3. Use a Dependency Management Section
For multi-module projects, you can define versions in a parent POM using the <dependencyManagement>
section:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>28.0-jre</version>
</dependency>
</dependencies>
</dependencyManagement>
Why Use Dependency Management?
This section helps manage dependency versions across modules efficiently. Changes made in the parent POM are propagated to child modules, keeping libraries in sync throughout the project.
4. Upgrade Libraries
Sometimes conflicts are resolved by upgrading the conflicting libraries. This is a more forward-looking solution, ensuring that you benefit from the latest features and performance improvements.
Before upgrading, always check the release notes for breaking changes or deprecated features.
Why Upgrade Libraries?
Using the latest versions frequently improves performance and security. Staying updated is a good practice for long-term project sustainability.
Testing After Resolving Conflicts
After resolving dependency conflicts, thorough testing is essential. Automated tests can verify that your application behaves as expected with the new dependency versions.
Here’s a snippet illustrating a simple JUnit test:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class SampleTest {
@Test
public void testSampleFunction() {
SampleClass sample = new SampleClass();
assertEquals("Expected result", sample.sampleFunction());
}
}
Make sure you run your entire test suite using:
mvn test
Additional Resources
For more detailed information about Maven dependency management, consider visiting the Apache Maven Dependency Management documentation. This resource provides comprehensive insights into how Maven resolves dependencies.
Another great tutorial on dependency issues can be found at Baeldung's Guide to Dependency Management in Maven.
Lessons Learned
Dependency conflicts in Maven can pose significant challenges, but understanding how they arise and the strategies to resolve them can simplify your development process. By following best practices like declaring versions, using exclusions judiciously, managing dependencies through parent POMs, and considering upgrades, you can maintain a clean and efficient build. Always remember to verify your application with robust tests to catch any issues early on.
Overcoming dependency conflicts isn’t just about fixing immediate issues; it is also about establishing good practices to ensure the long-term success of your project. Happy coding!