Mastering GridPane in JavaFX: Common Layout Pitfalls
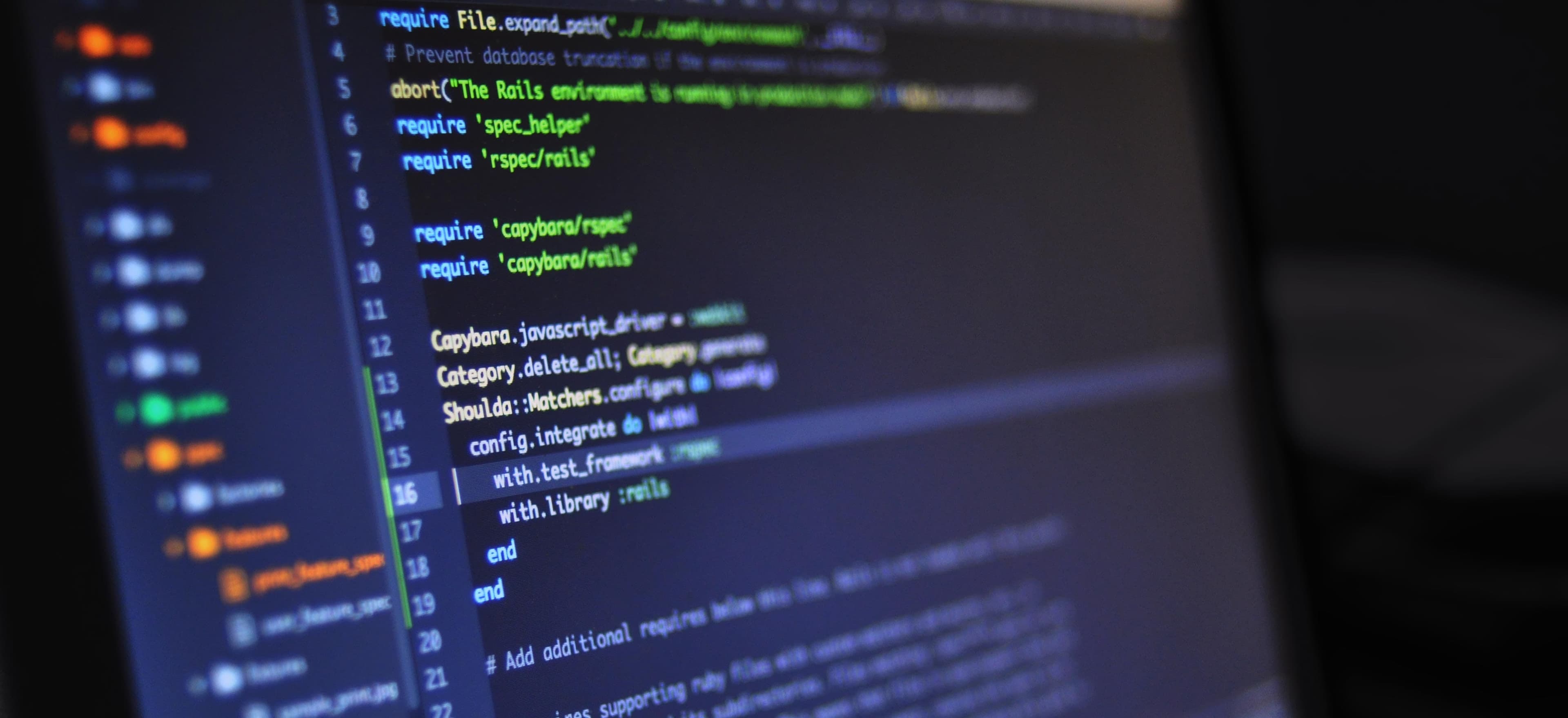
- Published on
Mastering GridPane in JavaFX: Common Layout Pitfalls
JavaFX is a powerful tool for building rich desktop applications in Java. One of its crucial components is the GridPane, which allows developers to arrange UI elements in a flexible grid format. While GridPane offers great flexibility and usability, it can also lead to common pitfalls that developers often encounter. This blog post dives deep into those pitfalls, providing illustrative code snippets and solutions to help you master GridPane.
Table of Contents
- Understanding GridPane
- Common Pitfalls
- Overlap of Nodes
- Improper Column and Row Sizing
- Mismanagement of Attachments and Constraints
- Best Practices
- Example Application
- Additional Resources
Understanding GridPane
GridPane
is a layout container in JavaFX that organizes its children in a grid of rows and columns. This layout manager can dynamically resize based on the content it holds, making it incredibly versatile.
Here’s a simple code snippet demonstrating how to create a basic GridPane
:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class GridPaneExample extends Application {
@Override
public void start(Stage primaryStage) {
GridPane grid = new GridPane();
Button btn1 = new Button("Button 1");
Button btn2 = new Button("Button 2");
grid.add(btn1, 0, 0); // Column 0, Row 0
grid.add(btn2, 1, 0); // Column 1, Row 0
Scene scene = new Scene(grid, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("GridPane Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Use GridPane?
Using GridPane
allows for complex UI structures without losing the simplicity of layout management. It is especially beneficial for forms and applications that need to align elements neatly.
Common Pitfalls
While GridPane makes layout management easier, there are some common pitfalls developers must navigate:
Overlap of Nodes
One common mistake is overlapping nodes, which occurs when multiple elements are added to the same cell without explicit configuration. This can cause one node to obscure another, leading to confusion in the UI.
Example:
grid.add(btn1, 0, 0); // Button 1 in (0,0)
grid.add(btn2, 0, 0); // Button 2 overlaps Button 1
Solution:
Ensure each component is placed in a unique cell, or use ColumnSpan
and RowSpan
for more complex layouts.
GridPane.setColumnSpan(btn2, 2); // Button 2 spans both columns
Improper Column and Row Sizing
GridPane has default sizing behavior that may not always align with your design intentions. Mismanaged width and height can lead to awkward spacing.
Example:
grid.setPrefWidth(200);
grid.setPrefHeight(200);
Solution:
Use column constraints to establish fixed or percentage-based sizes. Here’s how you can do that:
ColumnConstraints col1 = new ColumnConstraints();
col1.setPercentWidth(50);
grid.getColumnConstraints().add(col1);
ColumnConstraints col2 = new ColumnConstraints();
col2.setPercentWidth(50);
grid.getColumnConstraints().add(col2);
This ensures that the two columns will occupy equal space, dynamically adjusting as the window resizes.
Mismanagement of Attachments and Constraints
GridPane allows for fine-tuning using constraints. Not properly managing these constraints can lead to unanticipated layouts, especially when using nested layouts.
Example:
GridPane.setHalignment(btn1, HPos.RIGHT);
GridPane.setValignment(btn1, VPos.BOTTOM);
Solution:
Always double-check your alignment settings for each individual node. Document your choices within the code for easier debugging:
// Aligning Button 1 to the bottom right corner of its cell
GridPane.setHalignment(btn1, HPos.RIGHT);
GridPane.setValignment(btn1, VPos.BOTTOM);
This level of attention helps maintain your layout style long-term.
Best Practices
To effectively use GridPane, consider the following best practices:
-
Plan Your Layout: Before coding, sketch out how your components will relate within rows and columns.
-
Use Column and Row Constraints: Explicitly set constraints to maintain responsive designs, as shown previously.
-
Test Dynamically: Always run your application and test resizing to ensure your layout behaves as expected before deployment.
-
Avoid Nested GridPanes: If your design requires multiple nested layouts, consider using a combination of other layout managers like
VBox
orHBox
that might serve your purpose better.
Example Application
To illustrate, let's create a simple form using GridPane. This form will accept a username and password, demonstrating how to avoid the pitfalls discussed.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.PasswordField;
import javafx.scene.control.TextField;
import javafx.scene.layout.ColumnConstraints;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class LoginForm extends Application {
@Override
public void start(Stage primaryStage) {
GridPane grid = new GridPane();
// Define column constraints
for (int i = 0; i < 2; i++) {
ColumnConstraints columnConstraints = new ColumnConstraints();
columnConstraints.setPercentWidth(50);
grid.getColumnConstraints().add(columnConstraints);
}
// Add labels and inputs
grid.add(new Label("Username:"), 0, 0);
TextField usernameField = new TextField();
grid.add(usernameField, 1, 0);
grid.add(new Label("Password:"), 0, 1);
PasswordField passwordField = new PasswordField();
grid.add(passwordField, 1, 1);
Button loginButton = new Button("Login");
grid.add(loginButton, 1, 2);
// Align button to the right
GridPane.setHalignment(loginButton, HPos.RIGHT);
Scene scene = new Scene(grid, 350, 200);
primaryStage.setTitle("Login Form");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this code, we create a simple login form with labels and fields. Each element is properly placed without overlap, ensuring a clean interface.
Additional Resources
To further enhance your understanding of JavaFX and GridPane, consider these links:
A Final Look
GridPane is a powerful layout tool in JavaFX, but it comes with its set of common pitfalls. By understanding its behavior and adhering to the best practices outlined in this post, you can create robust and responsive applications. Happy coding!
Checkout our other articles