Avoiding Null Pointer Exceptions: A Java Developer's Guide
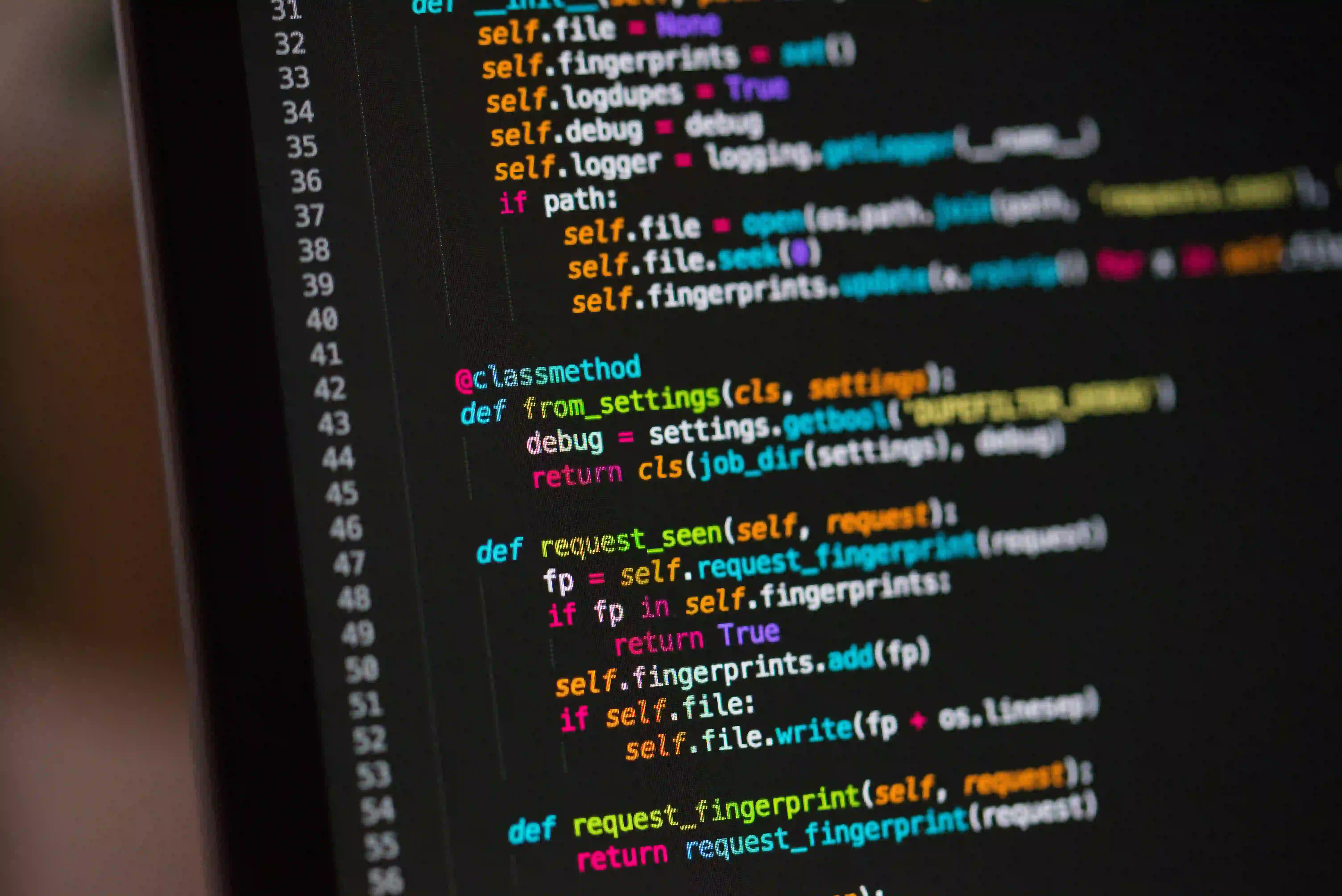
Avoiding Null Pointer Exceptions: A Java Developer's Guide
Null Pointer Exceptions (NPE) – the bane of Java developers everywhere. They pop up unexpectedly and can cause havoc in even the most robust applications. In this blog post, we’ll delve into strategies to avoid these pesky exceptions and improve the overall quality of your code.
Understanding Null Pointer Exceptions
Before we can tackle NPEs, we must first understand them. In Java, a null pointer exception occurs when your code attempts to use an object reference that has not been initialized (i.e., it points to null). This can happen in various scenarios, such as when calling methods or accessing fields of a null object.
Consider the snippet below:
public class Example {
public static void main(String[] args) {
String str = null;
System.out.println(str.length()); // This line throws a NullPointerException
}
}
In this example, invoking the length()
method on the null str
leads to an NPE. This is a common issue that can be easily avoided.
Best Practices to Avoid NPEs
1. Use Optional
One powerful feature that Java 8 introduced is the Optional
class. This class provides a container that can either hold a value or be empty, explicitly indicating that a value might be absent.
Here’s an example:
import java.util.Optional;
public class UserService {
public Optional<User> findUserById(String id) {
// Assume userRepository is a database access object
return Optional.ofNullable(userRepository.findById(id));
}
public void printUserName(String id) {
Optional<User> user = findUserById(id);
user.ifPresent(u -> System.out.println(u.getName()));
}
}
By using Optional
, we explicitly handle the scenario where the User
may not exist. This greatly reduces the likelihood of encountering NPEs.
2. Null Checks
Whenever you deal with potentially null objects, it’s wise to perform null checks before invoking methods or accessing fields. This is a straightforward approach but essential in many cases.
Example:
public class UserProfileService {
private User getUser(String userId) {
User user = userRepository.findById(userId);
if (user == null) {
throw new IllegalArgumentException("User not found");
}
return user;
}
}
In this snippet, we explicitly check if user
is null before trying to access its properties. This not only prevents an NPE but also allows us to handle the error gracefully.
3. Use Annotations
Java provides annotations like @NonNull
and @Nullable
that you can use to indicate whether a method parameter or return value can be null. This will help both you and others understand the intended use of your methods and can also be enforced by static analysis tools.
Using annotations could look something like this:
import org.eclipse.jdt.annotation.NonNull;
import org.eclipse.jdt.annotation.Nullable;
public class ProductService {
public void processProduct(@NonNull Product product) {
// process product
}
@Nullable
public Product findProductById(String id) {
return productRepository.findById(id);
}
}
By adopting these annotations, you provide context for developers who consume your code, helping them avoid potential pitfalls.
4. Constructing Immutable Objects
Immutability helps eliminate many common bugs in Java, including NPEs. When objects are immutable, once they are created, they cannot be changed. This characteristic makes it clear when a variable is supposed to be null or initialized.
Consider the following immutable class:
public final class Address {
private final String street;
private final String city;
public Address(String street, String city) {
this.street = street;
this.city = city;
}
public String getStreet() {
return street;
}
public String getCity() {
return city;
}
}
In this example, the Address
class is immutable. The constructor enforces the requirement that both street
and city
must be supplied, thus preventing null values. For more on immutability in Java, check out this comprehensive guide Java Immutability.
5. Initialization at Declaration
Where possible, initialize your variables at the point of declaration. This ensures that a variable has a meaningful value before it is ever used.
Example:
public class Configuration {
private final String configValue = "default";
public String getConfigValue() {
return configValue;
}
}
By initializing configValue
with a default, we eliminate the possibility of it being null.
Exception Handling for NPEs
Even with all precautions, NPEs may still occur. A suitable way to handle these is through exception handling. Use try-catch blocks to catch NPEs and take appropriate action.
public void printProfile(User user) {
try {
System.out.println(user.getProfile().toString());
} catch (NullPointerException e) {
System.out.println("User profile is not available.");
}
}
While it's better to avoid them in the first place, it’s also important to gracefully handle any NPEs that slip through.
My Closing Thoughts on the Matter
Null Pointer Exceptions are a common occurrence in Java programming, but with careful coding practices, they can be effectively avoided. By using Optional
, performing null checks, leveraging annotations, constructing immutable objects, and initializing variables directly, you can write clearer, more reliable code.
Always remember: the goal is not just to avoid NPEs, but to improve the maintainability and robustness of your codebase.
Additional Resources
For further reading on prevention strategies, you can explore the following links:
By adopting these best practices, you can ensure that your Java applications are less prone to errors, maintainable, and resilient in production. Happy coding!