Fixing AWS CodeBuild Error: Unable to Access Jar File
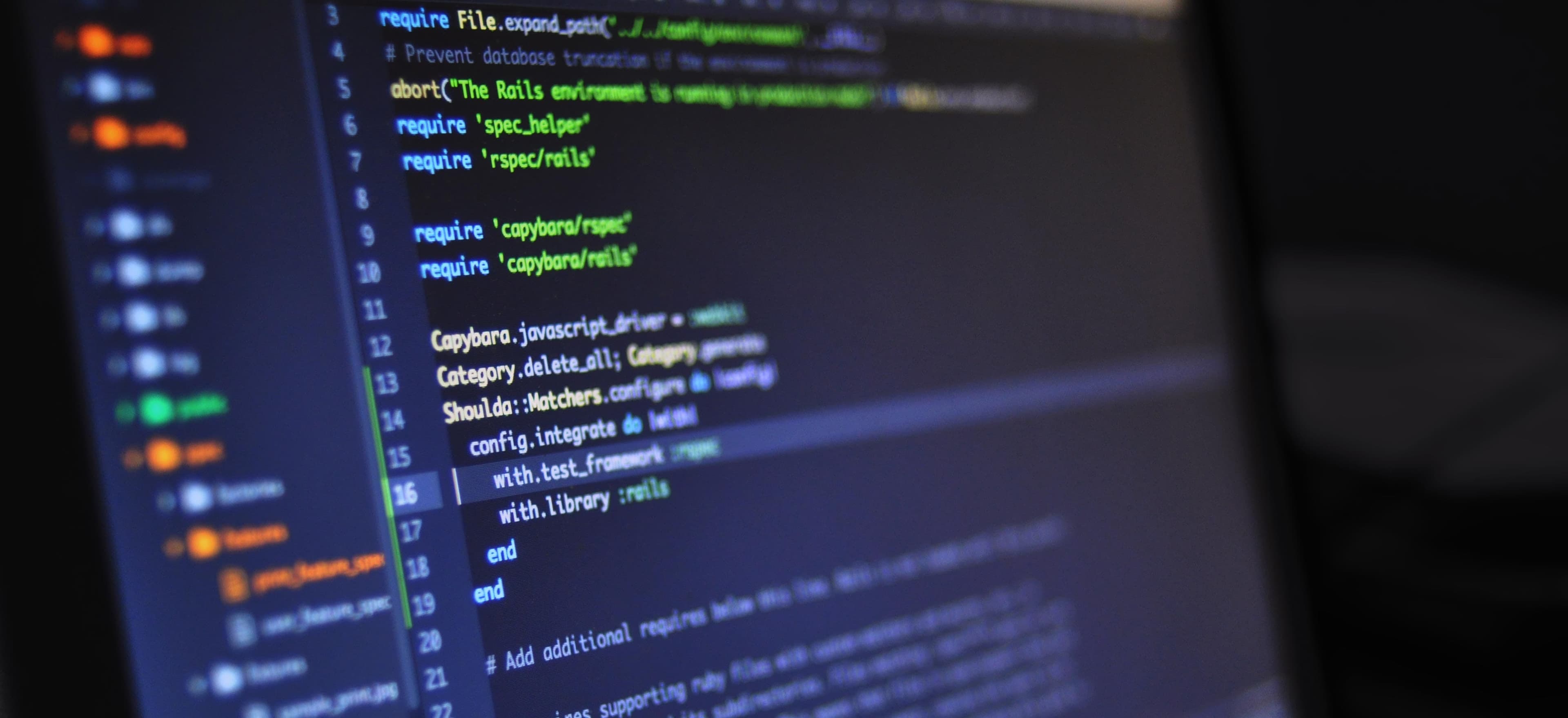
- Published on
Fixing AWS CodeBuild Error: Unable to Access Jar File
AWS CodeBuild is a powerful service for compiling source code, running tests, and producing packaged software. However, developers often encounter various errors during the build process. One common issue is the "Unable to access Jar file" error. This blog post will guide you through understanding this error and provide practical solutions to resolve it.
Understanding the Error
When you see the "Unable to access Jar file" error in AWS CodeBuild, it generally signifies that the build process cannot locate the specified JAR file needed for execution. The source of this problem can often be traced to several common factors, such as incorrect paths, missing files, or misconfigured settings.
Common Causes of the Error
- Incorrect Path to the JAR File: The specified location of the JAR file in your buildspec.yml or project configuration may be incorrect.
- File Not Found: The JAR file was not produced during the build process, meaning there might be a failure earlier in the build pipeline that prevented its creation.
- Permissions Issues: CodeBuild might not have permission to access certain directories or files where the JAR is located.
- Environment Variables: Misconfigured environment variables that define where your JAR files are stored.
Steps to Fix the Error
Step 1: Check the Buildspec.yml Configuration
The buildspec.yml file is crucial in dictating how AWS CodeBuild conducts its operations. Ensure that the path to the JAR file in your commands is accurate.
Here’s a typical example of a buildspec.yml:
version: 0.2
phases:
install:
runtime-versions:
java: openjdk11
build:
commands:
- echo "Building the project..."
- mvn clean package
- echo "JAR built successfully!"
post_build:
commands:
- echo "Running the JAR file..."
- java -jar target/myapp.jar
In this simple example, the JAR file should reside within the target
directory. If the JAR file does not exist here, CodeBuild will throw an error.
Step 2: Validate the Build Process
It's important to ensure that your Maven or Gradle build process is completing without errors.
To check if your JAR file was created, you can add a validation step to your buildspec:
post_build:
commands:
- ls target/
- if [ -f target/myapp.jar ]; then echo "JAR found!"; else echo "JAR not found!"; exit 1; fi
This code snippet lists the contents of the target
directory and verifies if the JAR file exists. If it does not, the build process will exit with an error, helping you to pinpoint the exact location where the build failed.
Step 3: Check File and Directory Permissions
Ensure CodeBuild has the necessary permissions to access your files. Permissions can be a common issue, especially if you're pulling code from an external repository. Verify that the AWS IAM role associated with CodeBuild has permissions to read from the source, write to build artifacts, and perform other necessary actions.
To check your IAM role, navigate to the IAM console, find your role, and review policy permissions. If required, you can modify the policy to grant additional access.
Step 4: Environment Variables
If your workflow involves environment variables to define paths for your JAR files, you need to ensure they are set correctly in your build project. To do this:
- Go to the AWS CodeBuild console.
- Select your project.
- In the build environment section, check your environment variables.
For example:
env:
variables:
JAR_PATH: "target/myapp.jar"
Update your build command to utilize this variable:
java -jar $JAR_PATH
Step 5: Debugging the Build Logs
Sometimes, the best way to identify issues within CodeBuild is through logs. AWS CodeBuild provides detailed logs for every build, and they can be invaluable for troubleshooting.
To access the logs:
- Open the CodeBuild console.
- Click on the build project.
- Select the specific build that failed.
- Navigate to the logs tab.
Look for any error messages or deviations that could lead to your JAR file not being created or found.
Integrating Your Code with CI/CD
If fixing the issue involves updates to your code or build process, consider integrating these changes into a CI/CD pipeline for consistent builds. AWS CodePipeline can help automate the process further by interlinking CodeBuild with version control and deployment tools.
Sample CI/CD Pipeline Configuration
Here’s a simplified overview of what your AWS CodePipeline stages might look like:
version: 0.2
phases:
install:
runtime-versions:
java: openjdk11
pre_build:
commands:
- echo "Preparing the environment"
build:
commands:
- mvn clean package
post_build:
commands:
- echo "Running the JAR"
- java -jar target/myapp.jar
Integrating AWS CodePipeline creates an automated process where your changes are built, tested, and deployed in an efficient flow.
Final Thoughts
Encountering the "Unable to access Jar file" error in AWS CodeBuild can be frustrating, but it is usually a result of simple misconfigurations. By following this guide and applying the steps outlined above, you should be well on your way to resolving the issue.
Don't forget to keep a close watch on build logs and permissions, which are often the keys to diagnosing your build problems effectively.
For more detailed insights into AWS CodeBuild and other services, you can refer to the AWS CodeBuild Documentation. If you have additional questions, feel free to leave comments below. Happy coding!
Checkout our other articles