Speeding Up Your ForkJoin Implementation for Optimal Results
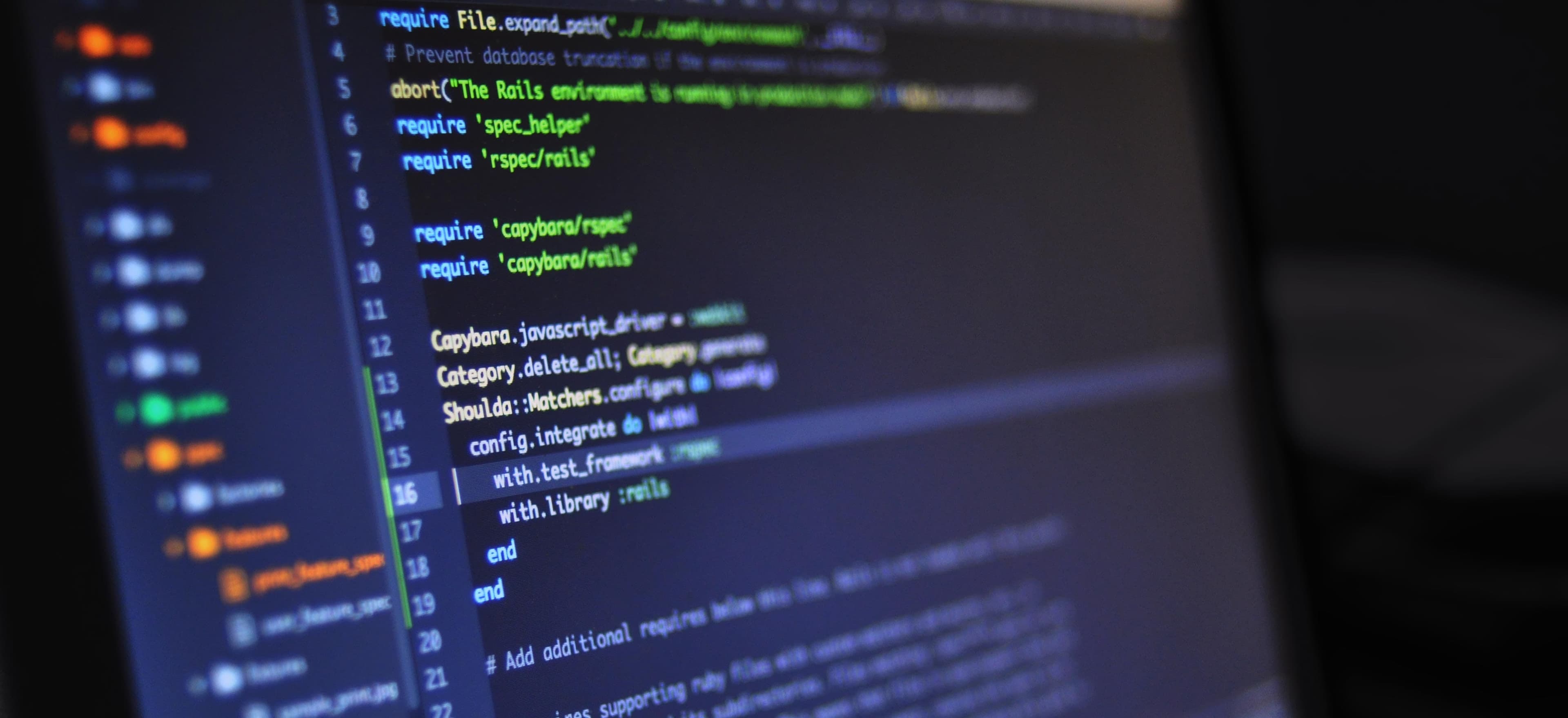
- Published on
Speeding Up Your ForkJoin Implementation for Optimal Results
In the world of modern computing, the need for efficient and responsive applications has never been greater. As developers, we continuously seek ways to optimize our code to fully utilize the capabilities of multi-core CPUs. Enter the Fork/Join Framework, a powerful tool introduced in Java 7 that allows for parallel execution of tasks by breaking them down into smaller subtasks. In this blog post, we will explore how you can speed up your ForkJoin implementations and achieve optimal performance.
Understanding Fork/Join Framework
The Fork/Join Framework is designed for work-stealing algorithms. When a thread cannot find work to do, it can "steal" tasks from other threads in the pool, allowing for better balance and utilization of resources. Here's a brief overview of its components:
- ForkJoinPool: A special implementation of the ExecutorService that helps manage and distribute tasks.
- ForkJoinTask: The base class for tasks that operate within this framework, allowing them to be divided into subtasks.
Why Use Fork/Join?
Before diving into optimizations, let's briefly touch on why you should consider using Fork/Join in your applications:
- Efficiency: It can process large workloads by leveraging multiple CPU cores.
- Scalability: As your application grows, Fork/Join lends itself well to parallel task execution.
For an in-depth understanding of the framework, you can refer to Oracle's documentation on Fork/Join.
Implementing a Simple ForkJoin Task
To begin, let’s look at a simple example of a ForkJoin task. This task will calculate the sum of an array of numbers.
Code Snippet: Summing an Array with ForkJoin
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class SumTask extends RecursiveTask<Integer> {
private static final int THRESHOLD = 10;
private final int[] numbers;
private final int start, end;
public SumTask(int[] numbers, int start, int end) {
this.numbers = numbers;
this.start = start;
this.end = end;
}
@Override
protected Integer compute() {
if (end - start <= THRESHOLD) {
return calculateDirectly();
}
int mid = (start + end) / 2;
SumTask leftTask = new SumTask(numbers, start, mid);
SumTask rightTask = new SumTask(numbers, mid, end);
leftTask.fork();
return rightTask.compute() + leftTask.join();
}
private Integer calculateDirectly() {
int sum = 0;
for (int i = start; i < end; i++) {
sum += numbers[i];
}
return sum;
}
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20};
ForkJoinPool pool = new ForkJoinPool();
SumTask task = new SumTask(numbers, 0, numbers.length);
Integer result = pool.invoke(task);
System.out.println("Total sum: " + result);
}
}
Commentary on Code
-
Task Division: The task is divided based on a threshold (
THRESHOLD
). If the task size is smaller than or equal to this value, it computes the sum directly. This prevents excessive forking and joining when dealing with small datasets, which can be costly. -
Forking and Joining: The left task is forked (executed asynchronously), and the right task is computed in the current thread. We then join the result of the left task. Forking is important here to allow other threads to work on the left task while the current thread processes the right.
-
ForkJoinPool: A
ForkJoinPool
is created and the task is executed usingpool.invoke(task)
, which manages the threading for us.
Optimizing Your Fork/Join Implementation
1. Choose the Right Threshold
Perhaps the most vital part of achieving optimal performance in any Fork/Join implementation is determining the correct threshold. If the threshold is too high, parallelism will be underutilized. If it's too low, you can introduce too much overhead from task management.
- Profile Performance: Experiment with different threshold values in your environment.
- Benchmarking: Use Java Microbenchmark Harness (JMH) or similar tools to measure the effect of various thresholds.
2. Minimize Synchronization
When using Fork/Join, ensure to minimize synchronization in your tasks to avoid the bottlenecking effects of contention.
- Use Local Variables: Rely on local variables instead of shared resources whenever possible.
- Immutable Data Structures: Utilize immutable data structures to avoid locking.
3. Tune ForkJoinPool Parameters
The default configuration of ForkJoinPool
uses a common pool suitable for most applications. However, for specific workloads, you may want to fine-tune the pool size.
ForkJoinPool customPool = new ForkJoinPool(4); // Limit to 4 parallel threads
4. Consider Using ParallelStream
In many cases, using parallelStream()
can provide a quicker implementation without the overhead of managing your ForkJoinTask
. Here's a quick way to sum an array using Streams:
import java.util.Arrays;
public class StreamSum {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int sum = Arrays.stream(numbers).parallel().sum();
System.out.println("Total sum: " + sum);
}
}
- The parallelStream() method allows for parallel processing of elements in the stream, effectively harnessing the Fork/Join framework under the hood.
Advanced Techniques for Higher Performance
5. Adaptive Work Stealing
The Fork/Join framework can adaptively change how it distributes tasks based on workload. Ensuring you're using a version of Java that adheres to the latest JVM optimizations can help in achieving better task scheduling and work stealing.
6. Fine-tuning Granularity
For excessively large problems, high granularity might lead to ongoing task creation overhead. On the other hand, too fine granularity can reduce parallel efficiency. Aim for a balanced approach.
7. Shifting to Other Parallel Libraries
If Fork/Join is causing performance bottlenecks for overhead or complexity, consider using other parallel libraries such as Akka or RxJava, which provide different paradigms for handling concurrent programming.
My Closing Thoughts on the Matter
Optimizing Fork/Join implementations boils down to understanding your specific workload and fine-tuning task division, threshold values, and pool configurations. Careful analysis and constant iteration will lead to achieving optimal performance in your applications.
With the above principles in mind, you can leverage the Fork/Join framework to harness the true power of your multi-core systems, ensuring that your applications remain responsive, efficient, and scalable.
Don’t forget to consider experimenting with alternative approaches and frameworks as you optimize your solutions. Happy coding!
For further insights, feel free to check out Java Concurrency in Practice for valuable strategies and patterns that can be applied in multi-threaded programming.
Checkout our other articles