Decoding Blue-Green Deployments: Key Pitfalls to Avoid
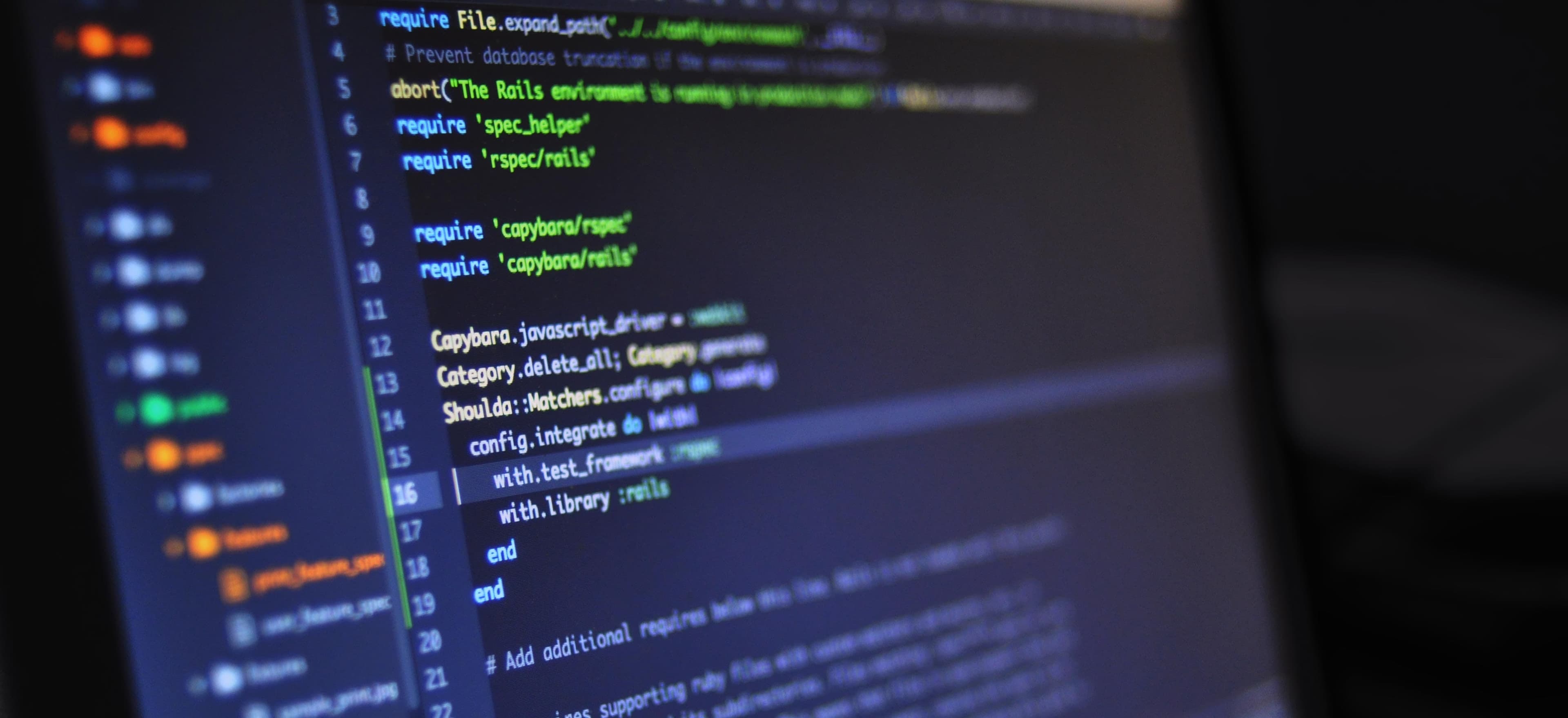
- Published on
Decoding Blue-Green Deployments: Key Pitfalls to Avoid
In the world of DevOps, blue-green deployments have emerged as a game-changer for delivering features quickly and reliably. This approach allows teams to minimize downtime and reduce deployment risk by maintaining two identical environments—one active (blue) and one idle (green). While this strategy offers remarkable benefits, it is not without its challenges. In this blog post, we will decode blue-green deployments and explore the critical pitfalls you should avoid.
What is Blue-Green Deployment?
A blue-green deployment involves creating two environments:
- Blue: The current live environment serving all customer traffic.
- Green: The new version of the application that has been tested and is ready for release.
Once the green environment is fully validated, you can switch traffic from blue to green with minimal downtime. If something goes wrong after switching, rolling back to the blue environment is as simple as redirecting traffic back.
Benefits of Blue-Green Deployments
Before we dive into the pitfalls, let's quickly highlight some of the advantages:
- Reduced risk: Immediate rollback option if issues arise.
- Increased uptime: Seamless transition for users leads to zero downtime.
- Simplified testing: Validate new changes in a live-like environment before going live.
For more information on the advantages of blue-green deployments, you can refer to this link.
Key Pitfalls to Avoid
Although blue-green deployment is a powerful technique, some pitfalls can undermine its effectiveness. Let’s explore some critical issues and how to steer clear of them.
1. Inadequate Testing Before Deployment
The Issue
Testing is crucial when switching between environments. If the green deployment hasn’t undergone thorough testing, this can lead to unpredictable behavior or even downtime when the switch occurs.
The Solution
Conduct extensive testing in a staging environment that mirrors production closely. Consider performance tests, regression tests, and load testing before the final switch. Always validate that the new features work under expected user scenarios.
@Test
public void testFeatureX() {
// Arrange
FeatureX featureX = new FeatureX();
// Act
boolean result = featureX.performAction();
// Assert
assertTrue(result, "FeatureX should perform its action correctly");
}
In this snippet, we’re using a simple unit test to validate a feature. Proper unit tests help ensure that the application functions as intended during deployment.
2. Not Monitoring Both Environments
The Issue
When you deploy in a blue-green setup, you might focus your monitoring efforts solely on the environment that is currently live. This can lead to undetected issues in the green environment.
The Solution
Implement monitoring tools that allow you to track the performance and health of both environments continuously. Use alerts to notify your team about any anomalies in either environment.
For further reading, you can check out monitoring strategies.
3. Ignoring Configuration Drift
The Issue
Configuration drift occurs when your environments start as identical but diverge over time due to updates, patches, or manual changes. This drift can introduce discrepancies that lead to unexpected behavior when switching environments.
The Solution
Use infrastructure as code (IaC) tools to manage and provision your environments consistently. Tools like Terraform or AWS CloudFormation can help maintain parity between environments.
Example (using Terraform):
resource "aws_instance" "web" {
ami = "ami-12345678"
instance_type = "t2.micro"
tags = {
Name = "Blue Environment"
}
}
This Terraform code snippet creates a new AWS EC2 instance. By managing both your blue and green environments via IaC, you mitigate configuration drift effectively.
4. Failing to Train Your Team
The Issue
If your team is not well-versed in blue-green deployment practices, the switch can lead to panic and mistakes. This can nullify the intended benefits and increase the risk of downtime or errors.
The Solution
Invest in training and documentation for your team. Conduct workshops and simulations to practice deployments and rollbacks in a low-stakes environment.
5. Poorly Managed Database Migrations
The Issue
One of the trickiest parts of blue-green deployments is handling database migrations. If your application has dependencies on specific database schemas, inconsistency arises when switching environments.
The Solution
Adopt patterns such as backward-compatible migrations. Always design database changes in a way that both old and new versions of your application can function simultaneously.
Example of a backward-compatible migration:
ALTER TABLE user ADD COLUMN new_email VARCHAR(255);
In this SQL command, we add a new column without dropping or altering existing ones, ensuring that both application versions can access the necessary data.
6. Lack of Rollback Procedures
The Issue
Assuming that everything will go perfectly during deployment can backfire. Lack of a clearly defined rollback plan can stall critical recovery processes.
The Solution
Prepare a detailed rollback plan that outlines how to revert to the previous environment swiftly. Document your processes for quick reference.
7. Network Configuration Problems
The Issue
Network issues often arise when transitioning between blue and green environments, especially regarding load balancers and routing settings.
The Solution
Ensure that your network configuration is optimized for fast switches. Test the routing thoroughly to avoid user traffic being directed to the wrong environment.
Example: Load Balancer Configuration
public void switchTraffic(String targetEnv) {
loadBalancer.setActiveBackend(targetEnv);
System.out.println("Switched traffic to " + targetEnv);
}
This Java function changes the backend environment of a load balancer. Proper configuration guarantees that user traffic is directed correctly during deployment.
The Bottom Line
Blue-green deployments offer an effective strategy for delivering software continuously and minimizing downtime. However, it’s essential to navigate common pitfalls thoughtfully. By ensuring thorough testing, proper monitoring, consistent configurations, team training, efficient database migration, careful rollback planning, and network management, you can harness the full potential of blue-green deployments.
If you're ready to implement or optimize your blue-green deployment strategy, make sure to keep these pitfalls in mind. They can save you a great deal of headache, time, and resources in the long run.
For more on deployment techniques, check out this resource.
By understanding and addressing key pitfalls, your team can successfully execute blue-green deployments, enhancing your development lifecycle and delighting your users with seamless updates.
Checkout our other articles