Boosting Android Performance: Optimize ParseArray Usage
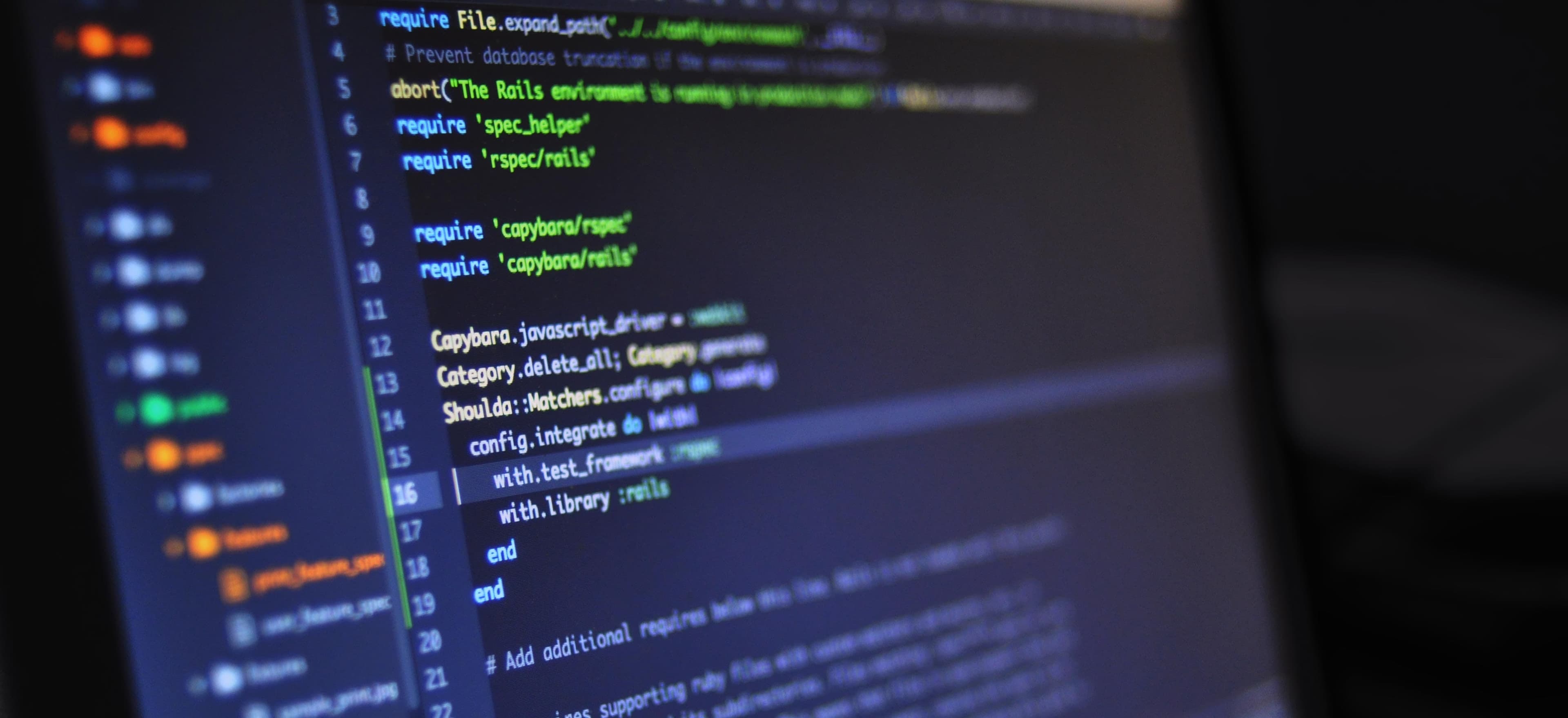
- Published on
Boosting Android Performance: Optimize ParseArray Usage
In the world of Android development, optimizing performance is always a priority. Developers strive to ensure that applications run smoothly, handle user input promptly, and utilize system resources efficiently. One powerful way to achieve this is by optimizing how data is parsed and handled. In this blog post, we'll focus on optimizing the ParseArray
usage in Android development, why it matters, and how to implement it effectively.
What is ParseArray?
ParseArray
is a data structure within Android's framework that is used to store key-value pairs. It's particularly used when working with large datasets. The efficient use of ParseArray
can significantly improve your application’s performance when it deals with the manipulation of complex data types.
Why Optimize ParseArray?
When poorly implemented, ParseArray
can lead to performance bottlenecks, increased memory usage, and a sluggish user experience. Optimizing ParseArray
allows developers to:
- Handle large datasets efficiently.
- Minimize memory overhead, which directly impacts performance.
- Reduce CPU cycles spent on data processing, leading to a more responsive application.
Best Practices for Optimizing ParseArray
Let’s delve into actionable strategies to enhance your usage of ParseArray
.
1. Use SparseArray Instead of HashMap
One of the most critical optimizations for Android applications is preferring SparseArray
over HashMap
.
Why SparseArray?
SparseArray
uses less memory thanHashMap
, especially for primitive data types.- It avoids the overhead associated with boxing/unboxing primitives.
Here's a basic example of how to use SparseArray
:
SparseArray<String> sparseArray = new SparseArray<>();
sparseArray.put(1, "One");
sparseArray.put(2, "Two");
// Accessing the value
String value = sparseArray.get(1); // returns "One"
// Check if key exists
if (sparseArray.containsKey(2)) {
// Do something
}
In this example, SparseArray
allows for efficient memory usage while providing fast access to data through its key-value structure.
2. Minimize Resizing Operations
When adding items to a ParseArray
or SparseArray
, resizing can be a CPU-intensive operation. Thus, you should pre-size your array whenever possible, reducing the frequency of resizing.
Example:
SparseArray<String> sparseArray = new SparseArray<>(100); // Pre-sizing for expected entries
for (int i = 0; i < 100; i++) {
sparseArray.put(i, "Item " + i);
}
By initializing your ParseArray
with a specific size, you can prevent repeated resizing. This simple act can significantly boost performance.
3. Leverage Value Caching
In scenarios where data is processed frequently, consider implementing a caching mechanism within your ParseArray
. This allows you to quickly access already parsed data without needing to reprocess it.
Example:
private SparseArray<MyObject> cachedArray = new SparseArray<>();
public MyObject getObject(int key) {
if (cachedArray.indexOfKey(key) >= 0) {
return cachedArray.get(key); // Return cached object
} else {
MyObject myObject = fetchFromDataSource(key);
cachedArray.put(key, myObject); // Cache the parsed object
return myObject;
}
}
private MyObject fetchFromDataSource(int key) {
// Simulate data fetching logic (could be a database or network operation)
return new MyObject(key);
}
In this case, the caching logic prevents multiple data fetches for the same key, saving on processing time.
4. Avoid Nested Structures
Try not to use nested ParseArray
or other containers within your ParseArray
. This can lead to convoluted code that is hard to manage and poor performance due to increased complexity in accessing and modifying data.
Instead, consider using flat data structures or separating concerns into different classes. For instance, if you are trying to store multiple values associated with a single key, consider redesigning your data model.
5. Profiling and Benchmarking
Lastly, always profile and benchmark your application for performance. Using tools like Android Studio Profiler allows developers to monitor the application’s memory usage and CPU consumption in real time.
Example of Using the Profiler:
- Open Android Studio.
- Navigate to
View > Tool Windows > Profiler
. - Select your device and app.
- Monitor the memory and CPU consumption while interacting with your application.
By identifying the key performance bottlenecks, you can focus your optimization efforts where they matter most.
Final Thoughts
Optimizing ParseArray
usage within an Android application can lead to significant performance improvements. Utilizing SparseArray
, minimizing resizing, implementing caching, avoiding nested structures, and profiling regularly are essential strategies for effective optimization.
With these optimizations, not only will your application utilize resources more efficiently, but users will also enjoy a smooth, responsive experience.
For further reading on performance optimization, consider delving into resources like Android Performance Best Practices.
By prioritizing these best practices, you’re on your way to building high-performing Android applications that are capable of delivering an exceptional user experience, even under heavy loads.
Feel free to share your experiences with ParseArray
optimizations or any challenges you’ve encountered in the comments below!